.png)
Unit testing is a crucial part of the software development process that ensures the correctness and functionality of individual units of code. It allows developers to catch bugs and errors early, leading to more reliable and robust software. In Java, there are various frameworks and tools available for unit testing, such as JUnit.
However, when it comes to mocking and stubbing dependencies in unit tests, Mockito stands out as one of the most popular and powerful libraries. In this article, we will dive into Mockito, specifically focusing on understanding how it simplifies mocking in Java unit testing.
The Basics of Unit Testing
Before delving into the specifics of Mockito, let's recap the basics of unit testing. At its core, unit testing involves testing individual units of code in isolation to verify their expected behavior. A unit is the smallest testable part of an application, such as a method or a function. Unit testing allows developers to catch defects early, identify and fix issues quickly, and build a solid foundation for the overall system.
The primary purpose of unit testing is to validate that each unit of code behaves correctly according to its expected behavior. This includes checking the inputs and outputs of the unit, as well as any side effects or interactions with other units of code. Unit tests are typically written by developers themselves and executed automatically as part of the build process, providing fast feedback on the correctness of the code.
If you want to learn best practices of writing unit tests for Java read Best Practices for Java Unit Testing: Tips and Techniques
Benefits of Unit Testing
Early Detection of Defects:
Unit tests catch defects early, reducing the cost and effort of fixing them later in the development process or in production.
Improved Code Quality:
Unit tests serve as documentation and examples of code behavior, promoting good coding practices and leading to improved code quality.
Faster Development Cycles:
Unit tests catch bugs early, speeding up the development process and improving team productivity.
Regression Testing:
Unit tests detect regressions, allowing for timely fixes and preventing unintended changes in existing code from reaching production.
Better Collaboration:
Unit tests provide a common understanding of code behavior, facilitating better collaboration among team members in larger projects.
For a more deep understanding, take a look at the basics and benefits of unit testing, read the article "Demystifying Unit Testing: Basics and Benefits".
Understanding Mockito
Mockito is a widely used mocking framework that provides a simple and intuitive way to create mock objects and stub dependencies in unit tests. Mock objects are objects that mimic the behavior of real objects but do not have any real implementation.
They are used to isolate the code being tested from external dependencies, such as databases, APIs, or external services, allowing for isolated and focused testing of individual units of code.
Mockito provides a set of annotations and methods that allow developers to create mock objects and define their behavior during test execution.
Key concepts in Mockito
Mock Objects
Mock objects are created using Mockito's mocking capabilities. They are created with the same interfaces or classes as the real objects they mimic and can be used in place of real objects in unit tests. Mock objects allow developers to define the behavior of external dependencies, such as method return values or exceptions, during test execution.
Behavior Definition
Mockito provides methods for defining the behavior of mock objects during test execution. For example, developers can use Mockito when() method to define the return value of a method call on a mock object, or use the doThrow() method to specify an exception to be thrown when a method is called on a mock object. This allows developers to simulate different scenarios and test the behavior of their code under various conditions.
Verification
Mockito allows developers to verify the interactions between the code being tested and its dependencies. Developers can use Mockito's verify() method to check if certain methods were called on mock objects during test execution, or use the verifyNoMoreInteractions() method to ensure that no unexpected interactions occurred. This helps in ensuring that the code being tested is interacting correctly with its dependencies.
Mocking Annotations
Mockito provides annotations that can be used to create mock objects and define their behavior directly in the test code. For example, the @Mock annotation can be used to create a mock object, and the @InjectMocks annotation can be used to automatically inject the mock objects into the code being tested. This makes the process of creating and using mock objects in tests even more convenient and readable.
Using Mockito in Java Unit Testing
Now that we have a basic understanding of Mockito, let's explore how it can be used in Java unit testing. Mockito follows a simple and intuitive syntax that allows developers to create mock objects, define their behavior, and verify interactions with minimal boilerplate code. Here are some of the key steps involved in using Mockito in Java unit testing:
Add Mockito to the Project
To use Mockito in a Java project, you need to add the Mockito library as a dependency. You can do this by including the Mockito dependency in your project's build configuration, such as Maven or Gradle.
For example, in a Maven project, you can add the following dependency to your pom.xml file:
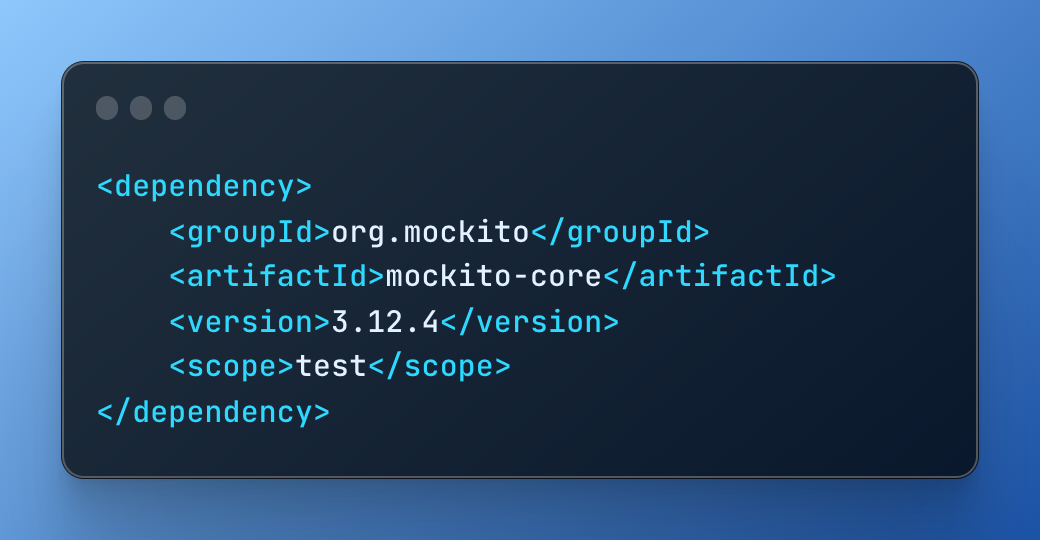
Ask Machinet to add the dependency if it is not already present. Machinet can write appropriate Mockito dependency, making it even more convenient for developers to integrate Mockito into their Java projects.
Create Mock Objects
Once you have added the Mockito dependency to your project, you can start creating mock objects in your unit tests. Mock objects can be created using the Mockito.mock() method, or by using the @Mock annotation. For example:
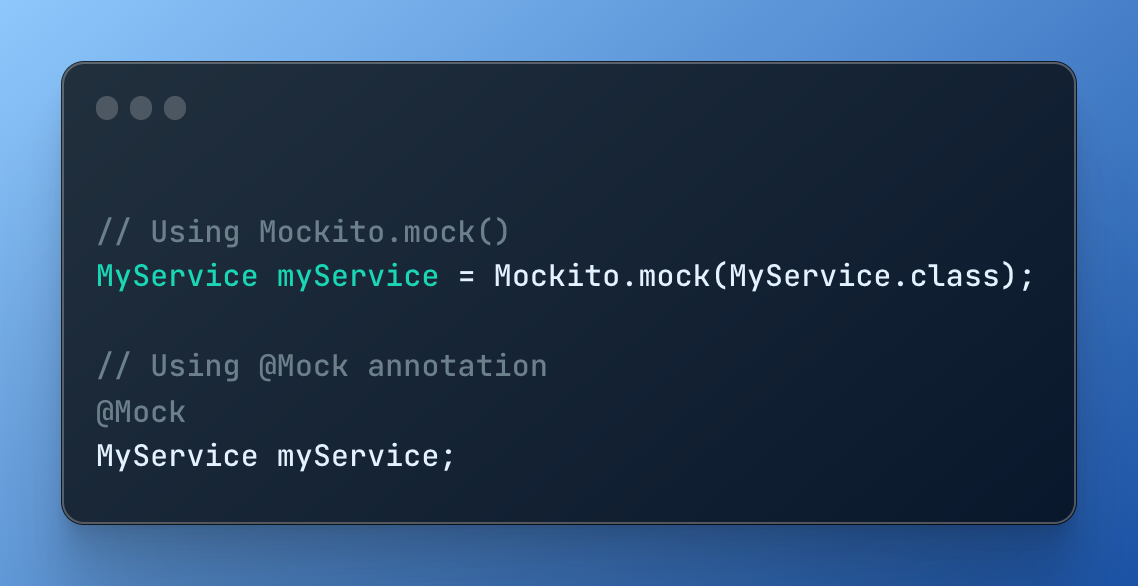
Machinet can automatically generate code that creates mock objects using the Mockito.mock() method or the @Mock annotation. This enables developers to easily create mock objects for their unit tests without having to manually write the code, reducing the chances of errors and ensuring consistent usage of mock objects across tests.
Define Behavior
After creating mock objects, you can define their behavior using Mockito's methods, such as when(), thenReturn(), thenThrow(), etc. For example:
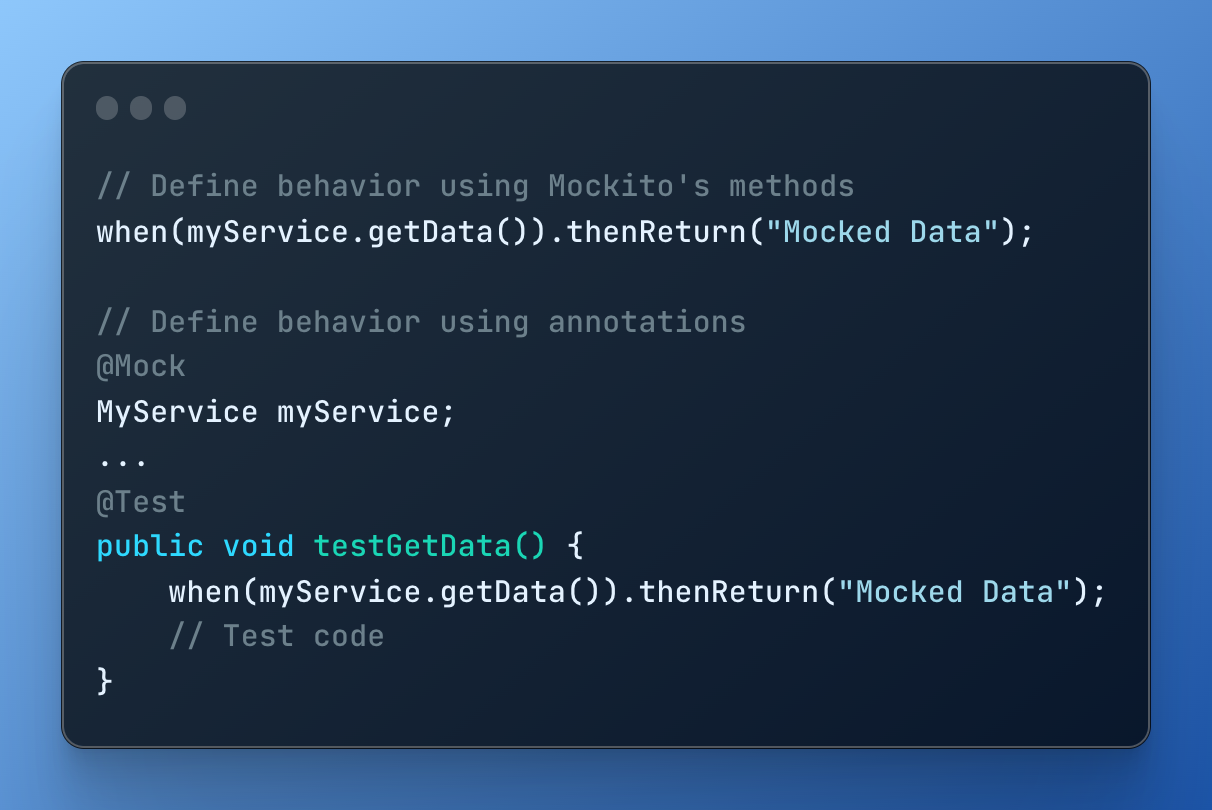
Machinet can automatically generate code that defines the behavior of mock objects using Mockito's methods, such as when(), thenReturn(), thenThrow(), etc. This allows developers to specify the desired behavior of the mock objects during test execution without having to manually write the code, making it more efficient and less error-prone.
Verify Interactions
Mockito allows you to verify the interactions between your code and its dependencies. You can use methods like verify(), verifyZeroInteractions(), verifyNoMoreInteractions(), etc., to check if certain methods were called on mock objects during test execution. For example

Machinet can automatically generate code that uses Mockito's methods, such as verify(), verifyZeroInteractions(), verifyNoMoreInteractions(), etc., to verify the interactions between the code being tested and its dependencies. This helps developers ensure that the expected interactions with mock objects are taking place during test execution, leading to more reliable and robust unit tests.
Use Mockito Annotations
Mockito provides annotations that allow you to create mock objects and define their behavior directly in the test code. You can use annotations like @Mock, @InjectMocks, @Spy, etc., to make the process of creating and using mock objects in tests more convenient and readable.
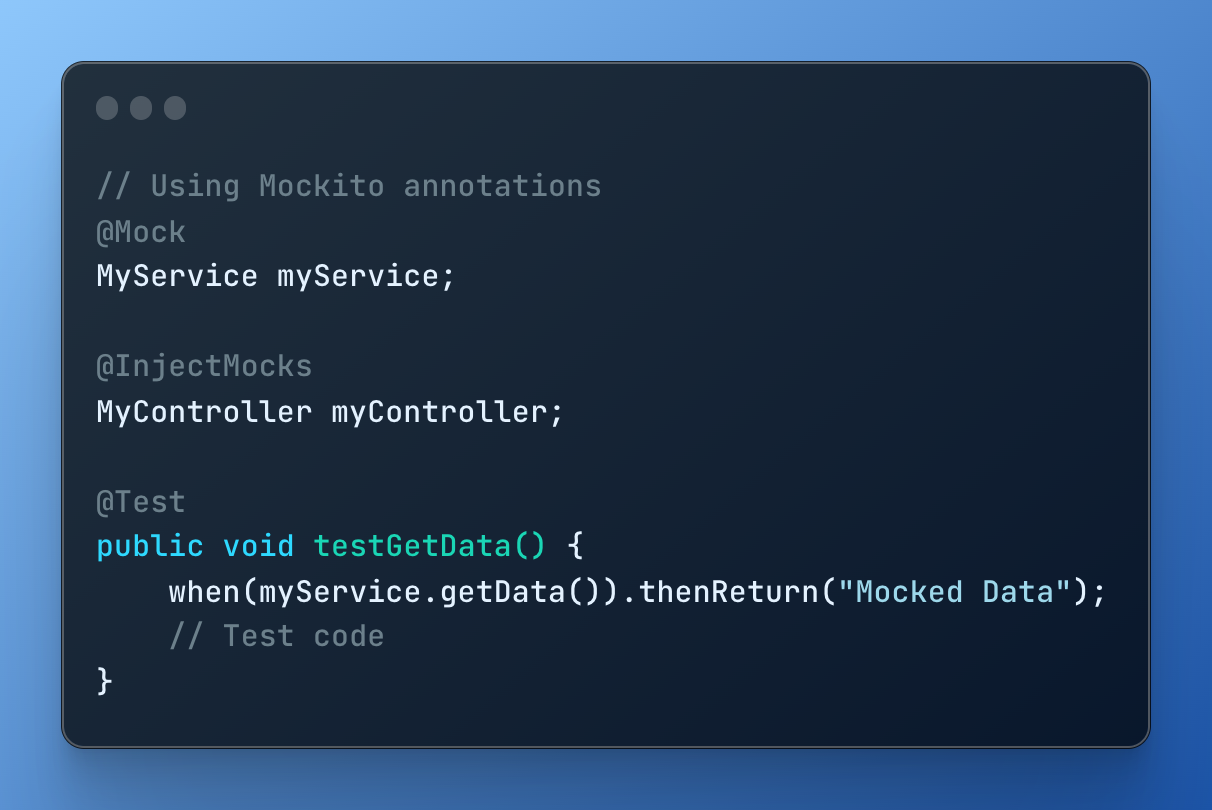
Machinet can automatically generate code that utilizes Mockito annotations, such as @Mock, @InjectMocks, and @Captor, to simplify the setup and usage of mock objects in unit tests. This allows developers to annotate fields or methods with these annotations, and Machinet can generate the corresponding code to properly initialize and inject mock objects into the test class. This helps streamline the process of using Mockito in unit tests and ensures proper configuration of mock objects.
Handle Exceptions
Mockito allows you to handle exceptions that may occur during testing. You can use methods like thenThrow(), doThrow(), etc., to define how mock objects should behave when certain methods are called with specific arguments. For example:
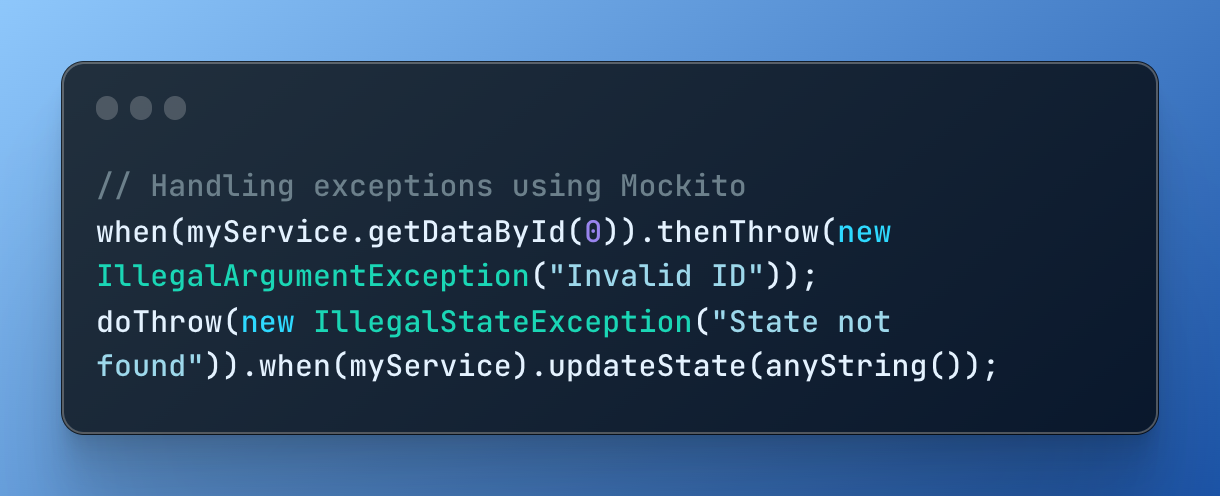
Machinet can generate code that demonstrates how to handle exceptions thrown by mock objects during unit testing. This may include generating code that uses Mockito's doThrow() or thenThrow() methods to specify the exception to be thrown by a mock object when a particular method is called.
Machinet can also generate code that verifies the behavior of the code under test when an exception is thrown by a mock object, such as checking if the code handles the exception appropriately. This helps developers thoroughly test the error handling logic of their code and ensure correct behavior in exceptional scenarios.
Use Mockito with JUnit
Mockito is commonly used in conjunction with JUnit, a popular Java testing framework. You can use Mockito to create and configure mock objects in your JUnit test methods, and then use JUnit's assertion methods to perform assertions on the results.
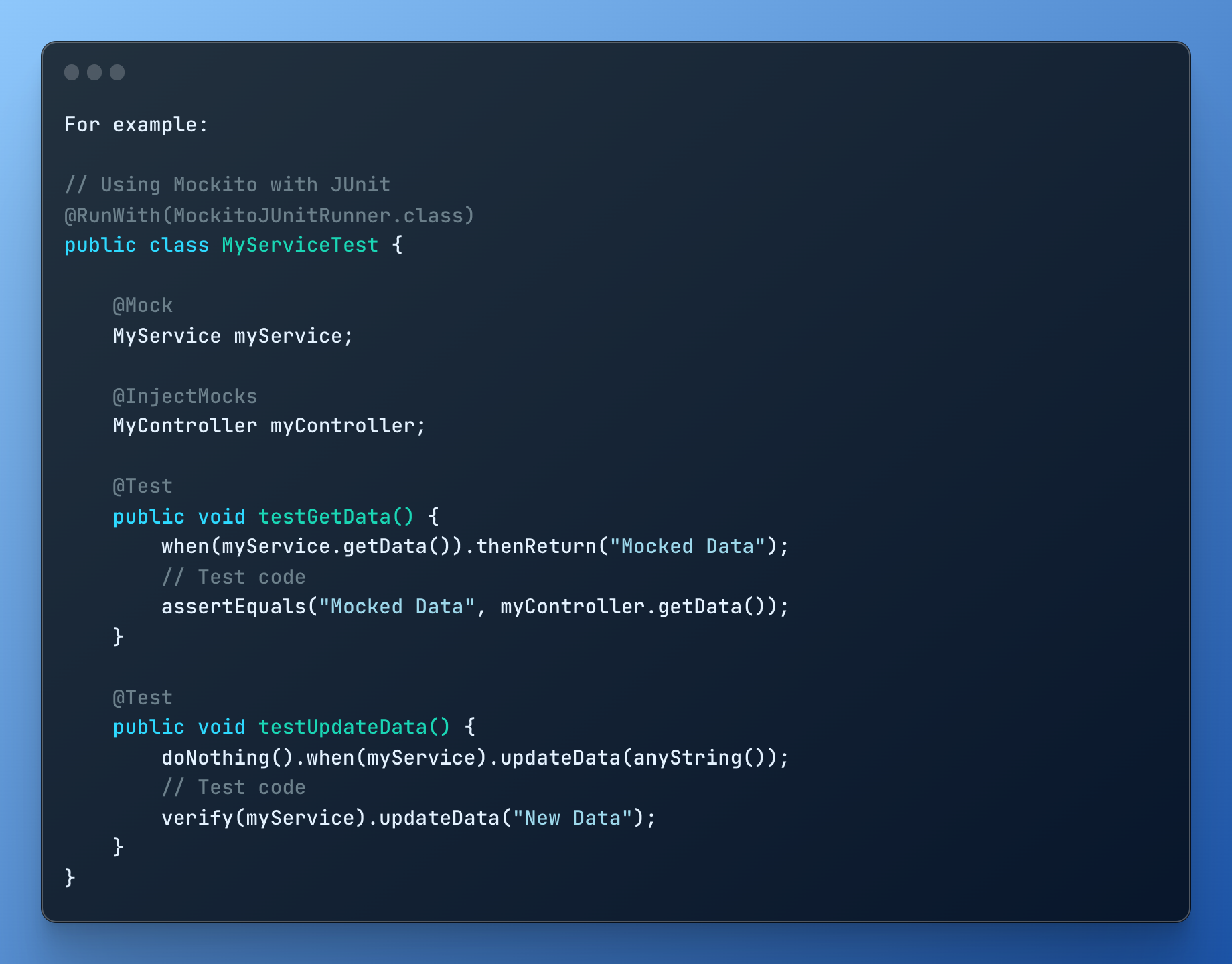
Machinet can automatically generate code that shows how to use Mockito in combination with JUnit, the popular Java testing framework.
Machinet can also generate code that showcases best practices for using Mockito with JUnit, such as organizing tests into test suites, using test runners, and setting up test doubles for different test scenarios. This helps developers effectively integrate Mockito into their JUnit testing workflow and write robust and reliable unit tests.
Conclusion
Mockito is a powerful and widely used mocking framework for Java unit testing. Understanding the basics of Mockito, including its annotations, assertions, and best practices, is essential for writing effective unit tests in Java.
By using Mockito effectively, you can isolate your code from external dependencies, define the behavior of mock objects, handle exceptions, and perform verifications, leading to more robust and reliable unit tests.
To speed up your adoption of Mockito Framework use Machinet, an AI assistant for Java developers, that automates the process of writing unit tests using Mockito. This helps Java developers push productivity and ensure the correctness of their code through automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.