.png)
Unit testing is an essential practice in software development that ensures the quality and correctness of code at the smallest functional level, known as a unit. It involves testing individual units of code in isolation to identify and fix bugs, validate functionality, and improve code maintainability.
This article is part of Introduction to Java Unit Testing:
- If you want to learn more about the basics and benefits of unit testing, read the article "Demystifying Unit Testing: Basics and Benefits"
- If you want to learn best practices of writing unit tests for Java read Best Practices for Java Unit Testing: Tips and Techniques
What is JUnit?
Java, being one of the most widely used programming languages, offers several frameworks and tools for unit testing, and one of the most popular and widely used ones is JUnit.
JUnit is a powerful and flexible testing framework for Java applications that provides a set of annotations and assertions to write effective and efficient unit tests. In this article, we will dive deep into JUnit and explore its various annotations and assertions that help developers write effective unit tests for Java applications.
Understanding JUnit Annotations
Annotations in JUnit are special markers that provide additional information to the testing framework and control the behavior of tests. They are used to define the setup, teardown, and test methods in a test class, and they guide the JUnit framework on how to execute the tests.
@Test
The @Test annotation in JUnit 5 works similarly to the one in JUnit 4 and is used to mark a method as a test method. JUnit 5 supports different test execution modes, including running tests in parallel, running tests based on tags, and running tests conditionally using the new @Enabled and @Disabled annotations.

@BeforeEach
The @BeforeEach annotation is used to mark a method that should be executed before each test method in the test class. It replaces the @Before annotation in JUnit 4 and is used to set up the preconditions for the tests.
.png)
@AfterEach
The @AfterEach annotation is used to mark a method that should be executed after each test method in the test class. It replaces the @After annotation in JUnit 4 and is used for cleanup tasks that need to be performed after each test method.
.png)
@BeforeAll
The @BeforeAll annotation is used to mark a method that should be executed once before any test method in the test class. It replaces the @BeforeClass annotation in JUnit 4 and is used for setup tasks that need to be performed once for the entire test class.
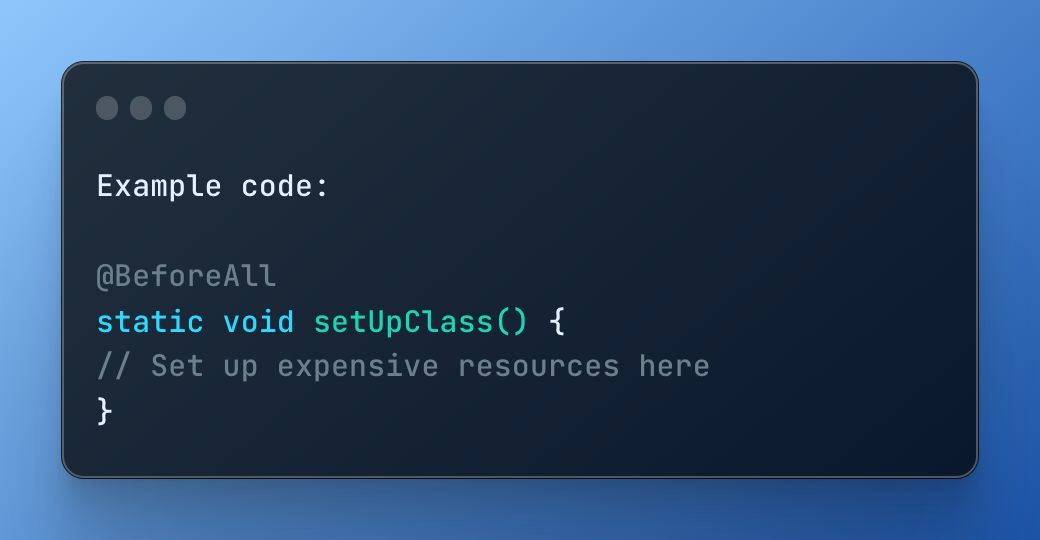
@AfterAll
The @AfterAll annotation is used to mark a method that should be executed once after all the test methods in the test class have run. It replaces the @AfterClass annotation in JUnit 4 and is used for cleanup tasks that need to be performed once for the entire test class.
.png)
@Disabled
The @Disabled annotation is used to mark a test method or a test class that should be disabled or ignored during test execution. It replaces the @Ignore annotation in JUnit 4 and is useful when a test is not ready for execution or needs to be temporarily skipped.
.png)
Understanding JUnit Assertions
Assertions in JUnit are used to validate the expected behavior of code under test. They allow developers to specify the expected output or state of the code and compare it with the actual output or state during test execution. If the actual output or state matches the expected output or state, the test passes; otherwise, it fails, indicating that there is an issue with the code being tested. JUnit provides a rich set of assertion methods that can be used to perform various types of validations.
assertEquals(expected, actual)
This assertion method checks if the expected value is equal to the actual value. It is commonly used to compare two values for equality, such as comparing the result of a method call with an expected value.
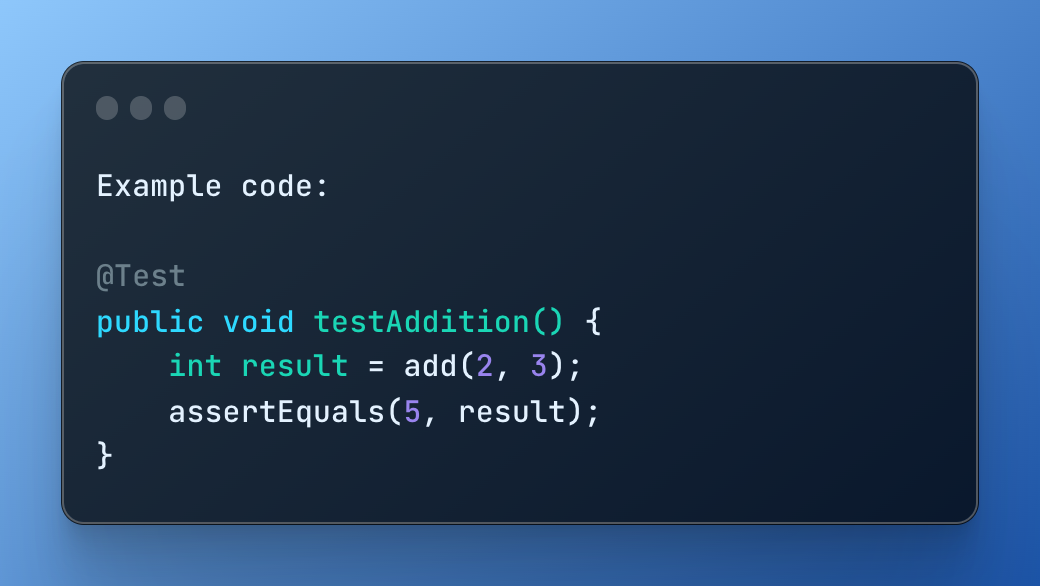
assertTrue(condition)
This assertion method checks if the given condition is true. It is commonly used to verify if a certain condition holds true during test execution, such as checking if a boolean flag is set to true.
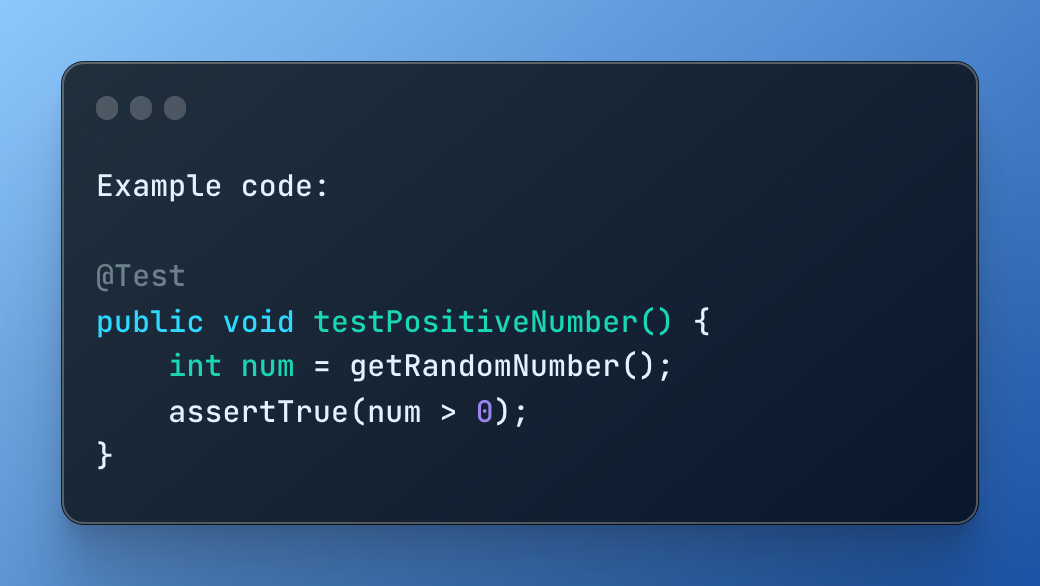
assertFalse(condition)
This assertion method checks if the given condition is false. It is commonly used to verify if a certain condition does not hold true during test execution, such as checking if an error flag is not set to true.

assertNull(object)
This assertion method checks if the given object is null. It is commonly used to verify if a method returns a null value when it is expected to.
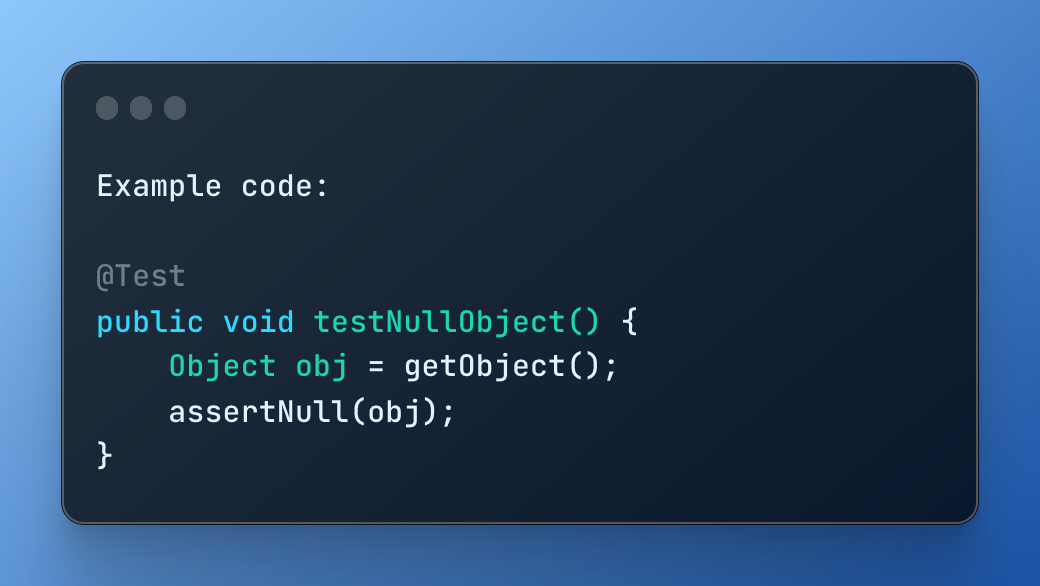
assertSame(expected, actual):
This assertion method checks if the expected and actual values refer to the same object in memory. It is commonly used to compare object references, such as checking if a method returns the same instance of an object.

assertNotSame(expected, actual):
This assertion method checks if the expected and actual values do not refer to the same object in memory. It is commonly used to verify if two object references are not pointing to the same instance.
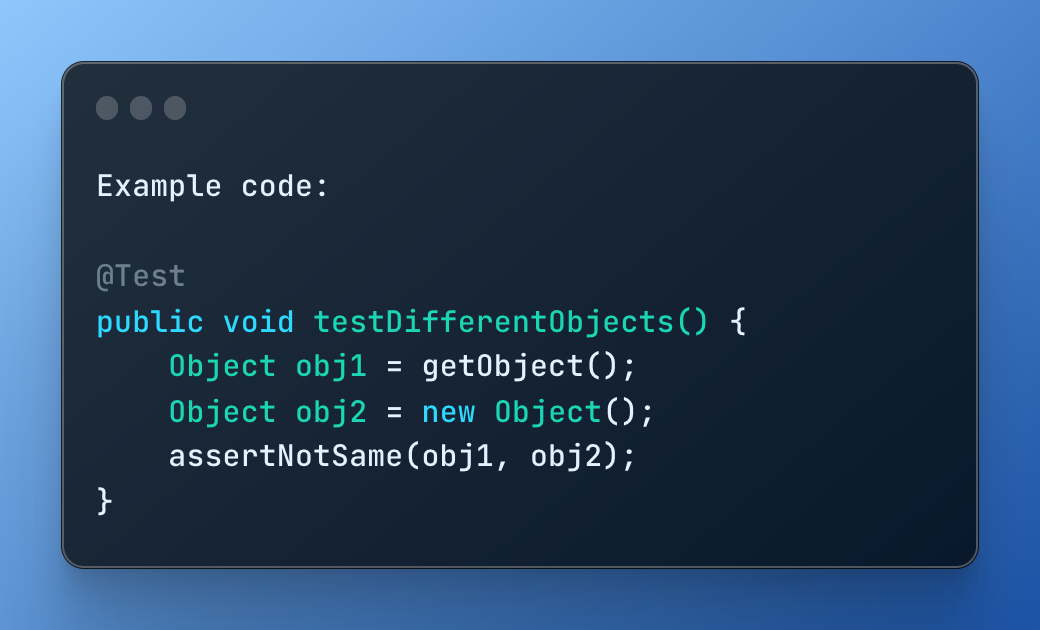
assertArrayEquals(expected, actual):
This assertion method checks if the expected and actual arrays are equal. It is commonly used to compare arrays for equality, such as checking if the elements in an array are as expected.
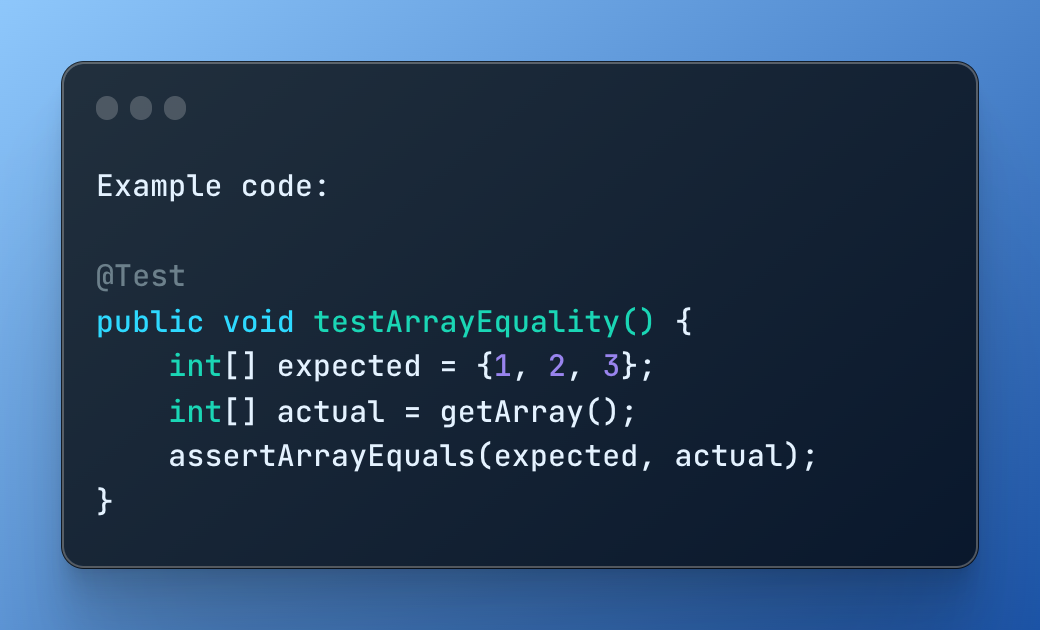
Machinet can also generate other types of assertion statements such as assertTrue, assertFalse, assertNull, and assertNotNull to perform various types of validations based on the code under test. With the help of JUnit annotations and assertions, Machinet's AI assistant can automatically generate comprehensive unit tests for Java applications, helping developers improve code quality, identify and fix bugs, and validate functionality more efficiently and effectively.
Conclusion
In conclusion, understanding annotations and assertions in the JUnit framework is crucial for writing effective unit tests in Java. Annotations provide a way to define and configure test methods, test classes, and test suites, while assertions allow for validations and comparisons of expected and actual values.
By leveraging these powerful features of JUnit, developers can write robust, reliable, and efficient unit tests that help ensure the quality and reliability of their Java applications.
Incorporating JUnit, along with other popular testing frameworks like TestNG and Mockito, into a comprehensive unit testing strategy can greatly contribute to the overall success of a software development project, enabling developers to catch and fix defects early, improve code quality, and deliver high-quality software that meets the needs of end-users.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.