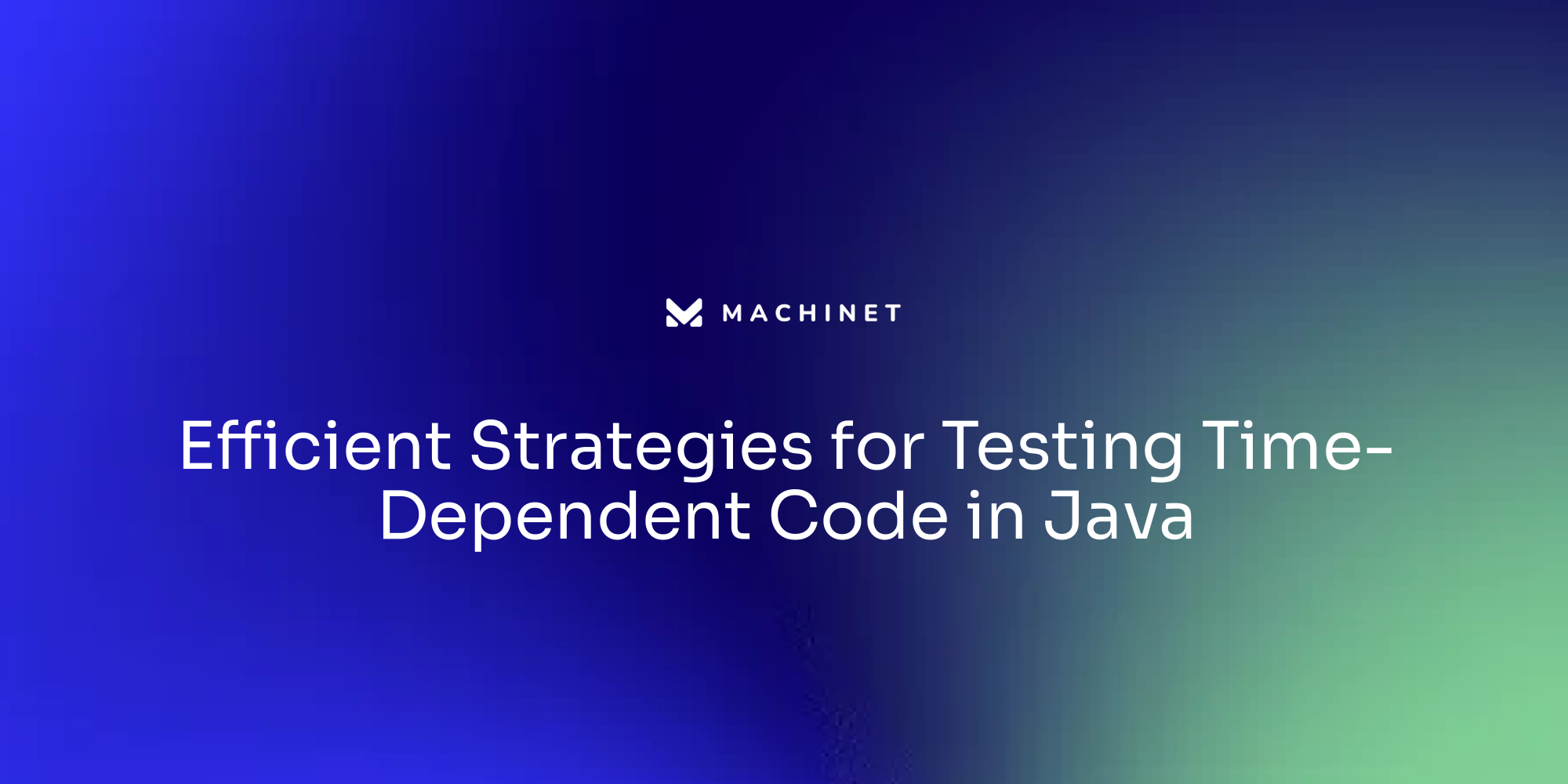
Table of Contents
- Understanding Time-Dependent Code in Java
- Challenges in Testing Time-Dependent Code
- Strategies for Handling Java Timers and Scheduling
- Implementing TimerTask for Unit Testing in Java
- Overcoming Quirks in Java Timers and Scheduling
- Effective Use of Junit for Testing TimerSchedule without Relying on Thread Sleep or Time
- Refactoring Strategies for Legacy Time-Dependent Code
- Balancing Workload and Deadlines in Testing Time-Dependent Code
Introduction
Understanding and effectively managing time-dependent code in Java is crucial for developing and implementing effective unit tests. Time-dependent code, which relies on system clocks or timers, presents unique challenges in testing due to its changing behavior over time. To overcome these challenges, various strategies have been proposed, such as using an alias clock, template specialization access, clock factories, passing time stamps, and more. Each strategy offers different levels of control and precision in unit testing. Additionally, the introduction of the Clock class in Java has revolutionized time manipulation in tests, allowing for custom time providers and enhancing the accuracy of time calculations.
In this article, we will delve into the intricacies of time-dependent code in Java and explore the strategies for handling and testing it effectively. We will discuss the challenges faced in testing time-dependent code and how to overcome them. Furthermore, we will examine the implementation of TimerTask for unit testing, strategies for handling Java timers and scheduling, effective use of JUnit for testing TimerSchedule without relying on thread sleep or real-time, refactoring strategies for legacy time-dependent code, and balancing workload and deadlines in testing time-dependent code. By understanding and implementing these strategies, developers can ensure the reliability and accuracy of their time-dependent code and streamline the testing process
1. Understanding Time-Dependent Code in Java
Rewriting time-dependent code in Java, which leans on system clocks or timers, is a complex task. This code is used for scheduling tasks, managing timeouts, and initiating time-based events. Its behavior changes over time, making it challenging to ensure consistent and reliable testing. To build effective unit tests, it's crucial to understand the nuances of time-dependent code.
Learn how to write effective unit tests for time-dependent code.
There are unique challenges when testing code that is dependent on time in an educational context. Using std::chrono::system_clock::now every time a timestamp is needed can make unit tests nearly impossible, as Björn Fahller points out.
Various strategies have been proposed to address this issue. One approach is to use an alias clock, appclock.now, instead of directly referencing std::chrono::system_clock::now in the code. This strategy provides better control over time in unit tests. Another approach involves using template specialization access, which encapsulates the clock, allowing for distinct clocks to be used in tests and production builds.
There are other strategies as well, like using a clock factory where the program calls clockfactory.getclock to fetch the current time. This method, however, brings up issues with singletons and mocking. Another approach is passing a clock object as a constructor parameter to classes that need to know the time. This eliminates the need for singletons but introduces storage-related complications.
Another strategy is to pass time stamps instead of requesting the current time. This method simplifies testing and provides better control over time. However, it may lead to a loss of precision. As Hubert notes, this strategy aligns with the broader aim of creating testable and deterministic code, eliminating any black boxes like reading the system clock.
The choice of approach largely depends on the required precision and specific needs of the program.
Explore different strategies for handling time-dependent code in Java.
For example, if the loss of precision is acceptable, the fifth approach is a viable option. Otherwise, the second approach, template specialization access, is recommended.
In real-world scenarios like networking systems, timers are often used to request notifications at specific points in future time. This is a practical application where the program's logic is time-dependent. Other examples include the use of clock factories and passing time stamps. While it's recognized that factories are typically singletons with their inherent problems, these approaches demonstrate how time can be managed in a program.
Understanding timezones and timestamps is crucial in coding.
Discover best practices for handling timezones and timestamps in Java.
The Java java.time package, including its classes like LocalDateTime, ZonedDateTime, Instant, and Clock, plays a significant role in this context. The exclusive use of LocalDateTime, however, has drawbacks, and the need to consider timezones is paramount.
The introduction of the Clock class in Java and its application in testing has been transformative. It has paved the way for the creation of a custom time provider interface, enhancing time manipulation in tests. This strategy is particularly useful when testing code that relies on time calculations.
In short, understanding and managing time-dependent code in Java is essential for developing and implementing effective unit tests. With the array of approaches available, it's important to choose the one that best fits the specific needs and precision requirements of the program
2. Challenges in Testing Time-Dependent Code
Testing time-dependent code comes with a unique set of challenges, primarily due to the inherent unpredictability of time-based operations. Tests that rely on timers or schedulers often require waiting for specific durations, resulting in lengthy test execution times. Additionally, isolating time-dependent code for unit testing can be a hurdle as it often interacts with external systems or resources.
Addressing these challenges requires thoughtful design of the time-dependent system and its testability. One method is to use an alias clock, app::clock::now, instead of directly invoking std::chrono::system_clock::now. This provides enhanced control over time during unit tests.
Another strategy involves template specialization access. This technique creates an encapsulation using templates to define different clocks for tests and production builds. However, this approach comes with its own difficulties. When a program needs to know the current time, it calls clockFactory::getClock, which returns an encapsulation of std::chrono::system_clock in production code. This clock factory usage can lead to issues with singletons and mocking during tests.
An alternate solution suggests that classes requiring time information should have a constructor that accepts a clock as a parameter. This eliminates the need for singletons but adds an additional storage requirement. Another approach involves passing timestamps to actions that depend on time. This simplifies testing and allows for precision measurement of timers.
The choice of approach depends on the specific requirements of the program and the level of precision required. While passing timestamps is generally preferred, template specialization access may be a better choice if precision is of concern.
The traditional methods of testing time-dependent classes in unit tests often result in slow and unreliable tests. The NodaTime and NodaTimeTesting packages can help address these issues. For instance, the limitations of traditional unit testing methods for time-dependent classes become evident when using xUnit to write unit tests for a video game combat skill with a 5-second cooldown period.
The introduction of the NodaTime package and the use of the IClock interface to inject the concept of time as a dependency is a significant advancement. The FakeClock class from NodaTimeTesting allows for time manipulation in unit tests, changing the current time and controlling the speed at which time advances. Unit tests using the FakeClock class to test time-dependent methods highlight the importance of NodaTime in making tests more consistent and reliable. Therefore, the NodaTime and its FakeClock class are highly recommended for working with DateTime in projects
3. Strategies for Handling Java Timers and Scheduling
When dealing with Java timers and scheduling, unit tests can present a unique set of challenges. However, there are several strategies that can be employed to mitigate these challenges and ensure accurate and effective testing.
Abstraction is a prevalent method used in handling time-dependent behavior in unit tests. This approach can be achieved through the use of interfaces or wrapper classes which allow for the replacement of the time source during the testing phase. For instance, Mockito, a known mocking framework, can be utilized to create mock objects. These mock objects can simulate the behavior of real objects, including timers, and allow you to control their behavior during testing. This makes the testing process more deterministic and reliable, focusing solely on the logic of the code.
In addition to Mockito, there are several wrapper classes in Java that are useful for managing timers in unit tests. These classes, such as the TimerWrapper
and ScheduledExecutorWrapper
, provide methods for managing time-related operations, making the testing process more predictable and repeatable. They allow for the simulation of different time scenarios and verification of the behavior of time-dependent code.
This approach of using wrapper classes and mock objects extends beyond just unit testing. It draws parallels with techniques used in other fields. For instance, Nader Zeid, a software engineer at OnSIP, used a similar technique to improve the performance of a large-scale web application. He minimized server overload by establishing determinacy in creating timed events using JavaScript functions.
In the field of professional digital audio, accurate playback is of utmost importance. This heavily relies on the precision of audio timing clocks. The Java Sound API has been discussed in relation to the accuracy of these clocks. To achieve single-frame accuracy for digital audio, timers more accurate than Java's System.currentTimeMillis() are required. This further emphasizes the importance of precise scheduling and timing in software development.
In conclusion, effectively handling Java timers and scheduling in unit tests not only improves the reliability of the tests but also has significant implications in other fields such as web application performance and digital audio accuracy. The key lies in understanding the underlying principles of these strategies and effectively applying them in unit tests
4. Implementing TimerTask for Unit Testing in Java
Java's TimerTask, an abstract class implementing Runnable, offers a robust mechanism for scheduling tasks for future execution in a background thread. This functionality is crucial when unit testing time-dependent code that relies on TimerTask. Ensuring the task's execution within the expected time frame is reliable and consistent is paramount.
To ensure reliable execution of TimerTask, countdown latches or similar synchronization mechanisms can be utilized. These mechanisms provide a signal to indicate the task's completion, thus streamlining the testing process. Additionally, the run method of the TimerTask class can be tailored during testing to include assertions or other test-specific logic.
The Timer class in Java is thread-safe, facilitating multiple threads to share a single Timer object without the need for external synchronization. It effectively schedules tasks using object wait and notify methods. Tasks can be scheduled to run once or at regular intervals using the Timer class. The Timer object waits for a task to finish before initiating the next task in the queue, ensuring orderly execution.
The Timer class also has the capability to create Timer objects that run tasks as daemon threads. The Timer cancel method can be invoked to terminate the Timer and discard any scheduled tasks. To avoid an ever-increasing task queue, it is recommended to set the time interval greater than the regular thread execution time when scheduling tasks using Timer.
Given its wide range of scheduling options and functionalities, the ScheduledThreadPoolExecutor class from the java.util.concurrent package can be a more flexible choice for complex applications. It provides a versatile replacement for the Timer class.
For testing time-dependent code that uses TimerTask, a test framework that allows you to control the timing can be utilized. This can be achieved by using a mocking or stubbing framework to simulate the TimerTask behavior and control the timing in your unit tests. Alternatively, you can refactor your code to use a more testable design, such as using dependency injection to inject a mock or stub TimerTask implementation for testing purposes.
To schedule tasks for future execution using TimerTask in Java, create a class that extends TimerTask and override the run() method. This method will contain the code that you want to execute at the scheduled time. Create an instance of Timer and schedule the task using the schedule() method. This method takes the TimerTask object and the delay before the task should be executed. Optionally, you can also specify the period at which the task should be repeated using the scheduleAtFixedRate() method.
In unit tests, if you want to add assertions and test logic to a TimerTask's run method, you can do so by creating a separate test method and invoking the run method of the TimerTask within that test method. Inside the test method, you can add assertions to verify the expected behavior of the TimerTask's run method. This allows you to test the logic and assertions separately from the actual execution of the TimerTask.
Testing TimerTask functionality in Java can be made more effective by using a mocking framework to mock the Timer class, using a test scheduler that allows you to control the timing of events during testing, testing edge cases, and using assertions to verify the expected behavior of your TimerTask.
To test time-dependent code with TimerTask, you can create a TimerTask that executes the code you want to test and schedule it to run at the desired time. By controlling the scheduling of the TimerTask, you can simulate different time scenarios and observe the behavior of your code.
In your testing code, you can create an instance of the TimerTask and schedule it using a Timer. You can then advance the time using the Timer's schedule method with a delay, and observe the effects of the code being tested
5. Overcoming Quirks in Java Timers and Scheduling
Java's built-in timekeeping and scheduling mechanisms, while robust, can introduce a degree of complexity when unit testing. One such complexity arises when a TimerTask throws an uncaught exception, leading to the termination of the timer thread and, consequently, the cancellation of subsequent tasks. This issue can be mitigated by incorporating exception handling within the run method of the TimerTask. Best practices suggest catching and handling exceptions within the TimerTask itself, logging any exceptions that occur, and considering the impact of exceptions on the overall Timer schedule. Unit testing TimerTask with exception handling can be achieved by using a try-catch block within the run() method of the TimerTask.
The Timer class in Java, while useful, is not thread-safe. This can trigger problems when tasks are scheduled or cancelled concurrently. A viable solution to this problem is the utilization of a thread-safe alternative like ScheduledExecutorService from the java.util.concurrent package. This interface provides a more flexible and efficient way to schedule tasks for execution in a background thread. It allows for the scheduling of tasks to run once or at fixed intervals, and also provides methods for cancelling and stopping the execution of tasks.
In professional digital audio, precise timing is paramount. However, Java's millisecond-accurate timers can pose limitations. This underscores the importance of accurate timing in digital audio and the necessity for performance tuning in Java.
There are also challenges associated with the use of Thread.sleep in Java tests, including time wastage or sporadic test failures. Alternatives to Thread.sleep, such as synchronization techniques like wait, notify, or a semaphore, can be employed. Refactoring the tested code to introduce a listener interface can facilitate the use of wait and notify for synchronization.
Synchronization with a semaphore involves the testing thread acquiring the semaphore and waiting for the counting thread to release it. It's also recommended to add a timeout to tests to avert indefinite blocking if the counting thread fails to signal completion.
Another alternative is polling, where the testing thread regularly verifies if the counter has finished counting before checking the result. The choice between these alternatives depends on requirements, personal preferences, and familiarity with different synchronization APIs
6. Effective Use of Junit for Testing TimerSchedule without Relying on Thread Sleep or Time
JUnit in Java brings a plethora of functionalities to the table for testing time-dependent code, reducing the reliance on Thread.sleep or real-time. One remarkable feature is the Timeout rule. This rule ensures tests don't run indefinitely, consuming precious resources by causing a failure if a test runs longer than specified.
To utilize the JUnit timeout rule, you would need to annotate your test method with the @Test
annotation and specify the timeout
parameter. This parameter indicates the maximum allowed time for the test to complete. If the test execution exceeds this limit, it's marked as a failure. For instance, consider the following code snippet:
```java import org.junit.Rule; import org.junit.Test; import org.junit.rules.Timeout;
public class MyTestClass { @Rule public Timeout timeout = Timeout.seconds(5); // Set the timeout to 5 seconds
@Test
public void myTestMethod() {
// Your test code here
}
}
``
In this example, the
myTestMethod` will fail if it takes longer than 5 seconds to complete.
Further, JUnit provides support for mock objects and stubs, which can be used to simulate the behavior of timers or schedulers. Mockito, a framework for Java unit testing, allows creation of mock objects and defining their behavior, including mocking timers and schedulers. By controlling the flow of time and simulating different scenarios, we can increase the reliability of the tests and their efficiency.
Consider creating an alias clock instead of directly referring to std::chrono::system_clock::now in the code. This allows different clocks to be used in unit tests and system builds, providing flexibility and control during testing.
Another strategy could be the use of template specialization to create an encapsulation for the clock. This could facilitate the use of a test clock in unit tests without the need for separate compilations, thus reducing the complexity and time consumption of the testing process.
Alternatively, implementing a clock factory returning an encapsulation of std::chrono::system_clock could allow different clocks to be used in unit tests and production code. This ensures that the testing environment closely mimics the production environment.
One could also consider passing a clock object to classes that need to know the time. This strategy eliminates the need for singletons but adds extra storage. However, this strategy could be beneficial in situations where the time is a crucial factor for the class.
A more preferred approach, if the loss of precision is acceptable, would be to pass time stamps as input to actions that depend on time. This simplifies testing and allows for control over the time stamps. This strategy makes the code testable and deterministic, eliminating the need for a black box. It provides a clear and straightforward method to handle time dependencies in unit tests, making the testing process more efficient and manageable.
The choice between these approaches depends on the requirements of the program. Using a single consistent timestamp versus specific timestamps for different steps of processing is a question of requirements. The most common case is to use a single time stamp for the whole event, indicating when the event was processed. This gives a clear indication of the event processing time, helping in analyzing the efficiency and speed of the code
7. Refactoring Strategies for Legacy Time-Dependent Code
Refactoring legacy time-dependent code can undeniably be a complex task. However, with the right strategies and techniques, it can be effectively managed, resulting in improved maintainability and testability of the codebase.
The first step in this process is to identify the parts of the code that are directly dependent on the current time or other time-related factors. Once these areas have been identified, the next step is to extract the time-dependent logic into separate functions or classes, which helps in isolating and testing the time-dependent behavior.
The use of abstractions is another effective method to refactor time-dependent code. By replacing direct time-dependent calls with abstractions, such as interfaces or utility classes, the code can be decoupled from specific time implementations. This not only enhances the code's clarity but also simplifies the process of identifying and isolating specific behaviors during testing.
Alongside this, consider replacing static method calls with non-static counterparts. The non-static methods can be overridden in subclasses, making it easier to use mocking frameworks for testing. The static methods, in contrast, cannot be overridden, making testing a challenge.
Furthermore, introducing dependency injection for time-related dependencies can be of tremendous help. Injecting these dependencies, rather than instantiating them directly within the time-dependent code, makes it easier to replace the time implementation with a mock or stub during testing.
The process of refactoring should be incremental, starting with the most critical and time-sensitive parts of the code. This approach involves creating a temporary branch and making small, incremental changes, or "micro commits," to the code. Each change is then tested to ensure it doesn't break the existing functionality.
Moreover, using Git for replaying the refactoring commits on top of the test branch can be a valuable tool. Running tests at each step ensures that the refactoring process is successful and that no regressions are introduced.
In addition to the above, when dealing with legacy code, utilizing fake implementations and snapshot tests can be beneficial. These techniques can simplify the task of verifying interactions and can be particularly useful when the number of non-provable transformations in the refactoring process is significant.
Finally, remember to write comprehensive unit tests for the refactored code, covering all possible time scenarios. This allows for more reliable and deterministic unit tests, as the behavior of the time-dependent code can be controlled and manipulated.
In summary, the refactoring process, while complex, can be effectively managed through careful identification and extraction of time-dependent logic, the use of abstractions, dependency injection, and rigorous testing. All these steps contribute to improving the maintainability and testability of legacy time-dependent code, ultimately leading to a more robust and reliable codebase
8. Balancing Workload and Deadlines in Testing Time-Dependent Code
When it comes to managing the demands of testing time-dependent code, strategic prioritization of test cases is crucial. Time-dependent code often presents unique challenges due to specific requirements and dependencies. Hence, test cases should be prioritized based on the critical paths and functionalities that are time-sensitive. A careful analysis of the code to identify areas where time plays a significant role in the outcome or behavior can be beneficial.
In addition to this, prioritizing test cases that cover edge cases and scenarios where time-related issues are likely to occur can ensure the code performs as expected under different time conditions and helps uncover any potential bugs or performance issues. Also, considering the input values and parameters that affect the time-dependent behavior of the code can help prioritize test cases. A focus on test cases that cover a wide range of input values and time scenarios can bolster confidence in the robustness and accuracy of the code.
Automation in testing procedures can be another significant factor in optimizing the process of balancing workload and deadlines. The use of mocking frameworks, such as Mockito or PowerMock, can simulate the passage of time during the test, allowing control and manipulation of the behavior of time-related functions and dependencies. Techniques such as using a virtual clock or time-traveling library, like Joda-Time or Timecop, can manipulate the system clock during tests, proving useful for testing scenarios that involve specific time intervals or dates.
In the context of continuous testing, the implementation of continuous integration servers can be a significant asset. These servers can be programmed to run the tests at predetermined intervals or in response to each modification in the code, thereby further enhancing the efficiency of the testing process. The use of continuous integration and delivery (CI/CD) pipeline can automatically run tests at predefined intervals or trigger them based on specific events. This ensures that any changes to the codebase are automatically tested, and any issues are detected early on.
Testing time-dependent code can be a time-consuming affair, particularly when it comes to comprehensive system testing of embedded software. Therefore, it's important to reduce the workload as much as possible. Using mock objects to simulate the behavior of time-dependent components, such as clocks or timers, during testing, allows control and manipulation of time-related scenarios. This makes it easier to test different code paths without having to wait for specific time intervals to pass.
Ensuring the reliability and correctness of software in time-dependent code is paramount. This can be achieved by following best practices for unit testing, implementing proper unit testing techniques, utilizing annotations and assertions, and using frameworks like JUnit. This structured approach to unit testing makes it easier to write and execute tests for time-dependent code.
In conclusion, the process of testing time-dependent code requires careful planning and consideration to ensure that tests are reliable, provide meaningful coverage, and maintain the balance between workload and deadlines
Conclusion
In conclusion, understanding and effectively managing time-dependent code in Java is crucial for developing and implementing effective unit tests. The article explores various strategies for handling and testing time-dependent code, such as using alias clocks, template specialization access, clock factories, passing time stamps, and more. Each strategy offers different levels of control and precision in unit testing. The introduction of the Clock class in Java has revolutionized time manipulation in tests, allowing for custom time providers and enhancing the accuracy of time calculations.
The ideas discussed in the article have broader significance in the field of software development. By implementing these strategies, developers can ensure the reliability and accuracy of their time-dependent code. This leads to streamlined testing processes, improved maintainability of codebases, and enhanced overall software quality. Time-dependent code is prevalent in various domains, such as networking systems or professional digital audio applications, making these strategies applicable across industries.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Click here to learn more
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.