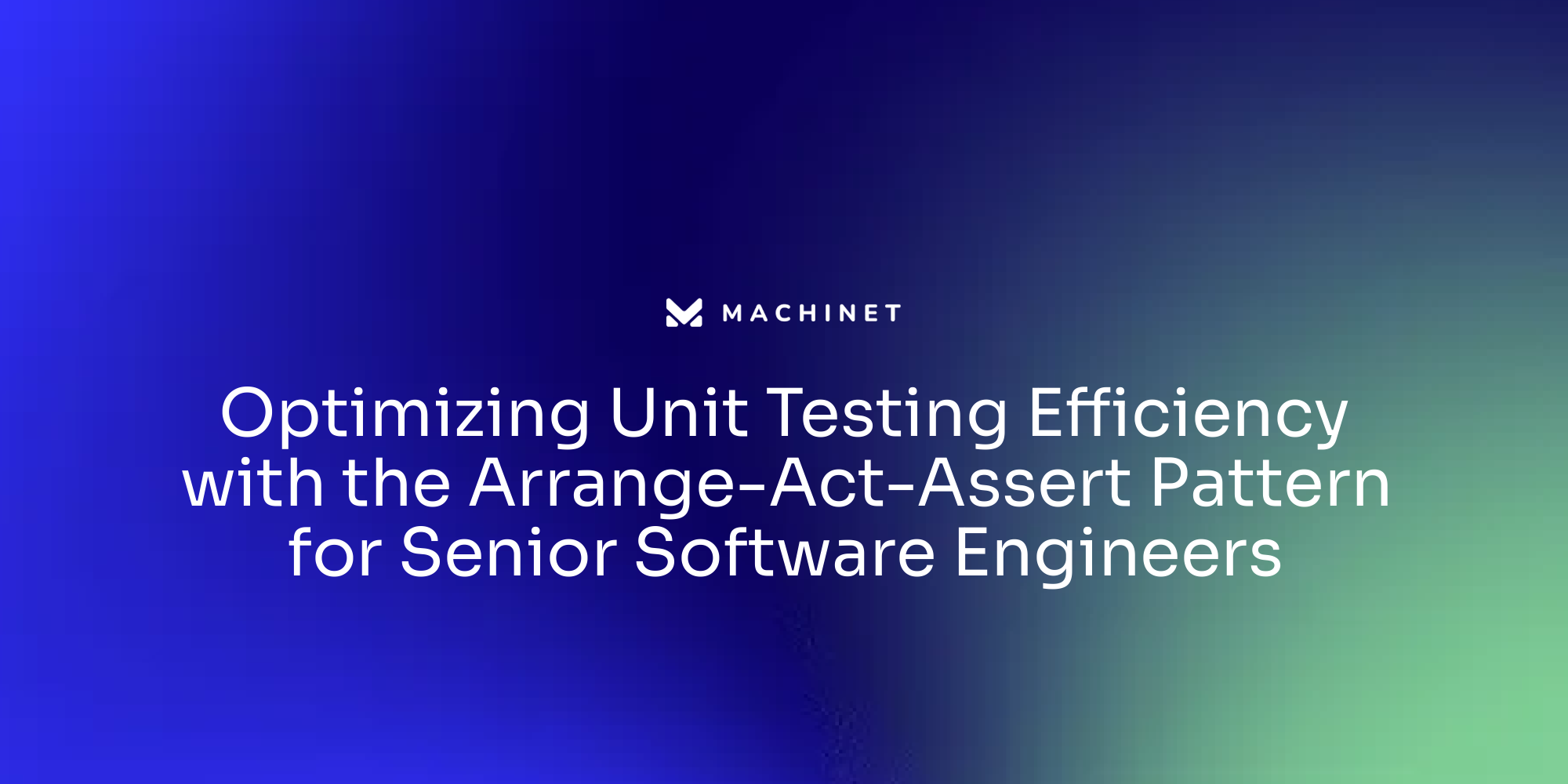
Table of Contents
- Understanding the Arrange-Act-Assert (AAA) Pattern in Unit Testing
- Implementing the AAA Pattern: Step-by-step Tutorial
- Best Practices for Unit Testing with AAA Pattern: Tips for Senior Software Engineers
- Addressing Common Challenges in Unit Testing: Changing Requirements and Workload Management
- The Role of Automated Testing Tools in Optimizing AAA Pattern Implementation
- Case Study: Successful Application of AAA Pattern in Unit Testing
- Refactoring and Improving Existing Test Suites Using the AAA Pattern
- Balancing Deadlines and Test Quality: Strategies for Efficiently Implementing the AAA Pattern
Introduction
The Arrange-Act-Assert (AAA) pattern is a structured approach to unit testing that offers clarity and simplicity to developers. This pattern divides the testing process into three phases: Arrange, Act, and Assert. In the Arrange phase, the test environment is set up by initializing objects and preparing the necessary data. The Act phase involves executing the code being tested, while the Assert phase verifies the expected outcomes and compares them to the actual results.
The AAA pattern has gained popularity among developers due to its effectiveness in creating readable and maintainable tests. By following this pattern, developers can easily understand and modify tests, leading to improved test coverage and software stability. Additionally, integrating automated testing tools into the AAA pattern can further enhance the efficiency and reliability of unit tests. In this article, we will explore the benefits and best practices of implementing the AAA pattern in unit testing, as well as address common challenges and strategies for improving existing test suites
1. Understanding the Arrange-Act-Assert (AAA) Pattern in Unit Testing
The Arrange-Act-Assert (AAA) pattern is a widely recognized strategy in unit testing, offering a clear and structured approach to scripting tests. This pattern is broken down into three distinct segments, each of which plays a crucial role in the testing process.
The 'Arrange' stage sets the foundation for the test. It includes initializing objects, identifying inputs, and setting up mock data. This stage is vital as it ensures that the testing environment is optimally prepared for the execution phase. The Arrange phase is integral to unit testing as it ensures that the test is conducted in a controlled and predictable setting. By appropriately arranging the test data and setting up the necessary dependencies, you can isolate the code under test and verify its behavior accurately.
The 'Act' phase follows, serving as the heart of the testing process. It involves executing the functionality undergoing testing. This phase is where the actual testing takes place, with the system under test being called upon to execute the function being tested. The Act phase is where the behavior being tested is carried out. This could involve invoking a method, calling a function, or performing some action.
The 'Assert' phase is the final step. It is responsible for validating the results, checking if the outcomes produced by the 'Act' phase align with expectations. This stage is crucial as it determines the success or failure of the test. In the Assert phase, the outcome of the test is verified. This typically involves making assertions on the actual results against the expected results, checking if the behavior was as expected.
The AAA pattern's popularity in unit testing stems from its simplicity and clarity.
Try Machinet to simplify your unit testing process and save time!
It deconstructs the testing process into manageable segments, making it easier for developers to write and comprehend tests. The simplicity of this pattern also contributes to reducing complexity in test cases, making them easier to manage and maintain.
Software development expert Roy Osherove, author of "The Art of Unit Testing" among other works, endorses this pattern. He is a respected figure in the software development community, with extensive contributions including training on topics such as Test-Driven Development (TDD) and unit testing, and sharing insights on effective software development practices.
Moreover, numerous real-world applications of the AAA pattern have demonstrated its effectiveness. For instance, Osherove has successfully tackled technical challenges related to popular applications such as Skype for Business on Mac, Pi-hole, and CPU usage during screen sharing in Zoom, using the AAA pattern. These examples serve as evidence of the pattern's practicality and robustness in diverse testing scenarios.
In essence, the AAA pattern offers a structured, clear, and effective approach to unit testing. Its simplicity and clarity make it a preferred choice among developers, leading to more manageable and maintainable tests. It is a powerful tool that can enhance the quality and reliability of software applications
2. Implementing the AAA Pattern: Step-by-step Tutorial
The AAA pattern, standing for Arrange, Act, Assert, is widely recognized as an effective strategy for structuring unit tests. This pattern not only enhances the readability of the test code but also its maintainability, playing a crucial role in the software development process.
The Arrange phase forms the bedrock of the test, laying the groundwork for test execution. This phase might encapsulate object creation, variable initialization, and system configuration, thus preparing the system for the test run.
Following the Arrange phase is the Act phase, where the actual execution of the code under test occurs. This phase might involve calling a single method or executing a series of actions on the system, marking the actual testing of the code's functionality.
The Assert phase is the final stage, focusing on verifying the test results. This could involve checking return values or the state of the system post-test execution. It's essential to note that each test should ideally contain a single assert to ensure that the tests remain atomic and independent, preventing any interference between them.
A practical demonstration of the AAA pattern in action can be found in a GitHub repository named "jest test practice", maintained by a user "cynthiainga". This repository showcases the application of the AAA pattern in testing JavaScript functions with the Jest library.
As echoed by industry professionals like Jay Cruz, who states, "Unit testing is an essential part of building software", and Rich Edwards, who emphasizes, "All testing needs to follow this pattern, everything from unit tests through automation and up to manual testing", the importance and acceptance of the AAA pattern in unit testing are evident. This further reinforces its importance in building robust and reliable software
3. Best Practices for Unit Testing with AAA Pattern: Tips for Senior Software Engineers
As a senior software engineer, one of the key aspects of enhancing the quality of your software is unit testing. The Arrange-Act-Assert (AAA) pattern is a proven method that can be employed to structure your unit tests. However, the effectiveness of this pattern is not only dependent on its application but also the practices that are followed.
Each test you design should focus on validating a singular functionality or condition. This principle of simplicity and focus in your tests allows for precise error detection, and in case of a test failure, the source of the problem can be easily identified.
Another important aspect to consider is the independence of your tests. When tests are interdependent, it can lead to a cascade of failures making it challenging to pinpoint the root cause. To avoid this, each unit test should be independent and capable of running in isolation, not influenced by the results of other tests.
The naming of your tests should be expressive. The name should clearly indicate what functionality or condition the test is validating, which provides clarity and understanding of the test's purpose and the impact of its result.
Ignoring a failing test is not an option in the AAA pattern. A failed test is a signal of a potential issue in your application's functionality. It's critical to investigate and address these issues to ensure the reliability of your software.
Refactoring your tests is just as important as refactoring your production code. Keeping your tests clean, well-structured, and maintainable is integral to the long-term effectiveness of your testing strategy. Organizing your tests according to the AAA pattern - arranging the test data, acting on the system under test, and asserting the expected outcomes - makes your unit tests more readable and easier to understand, which in turn helps to identify and isolate any issues or failures.
Utilizing patterns like builder and custom assert, coupled with these practices, can contribute to creating clean, readable, and maintainable code. Libraries such as Fluent Assertions can enhance the readability and meaningfulness of your assertions. However, remember, while these additional layers of abstraction have benefits, they also require extra maintenance.
In essence, by following these best practices while using the AAA pattern in unit testing, you can significantly enhance the quality of your tests and, consequently, the software you're developing
4. Addressing Common Challenges in Unit Testing: Changing Requirements and Workload Management
Unit testing, an integral part of the software development life cycle, can sometimes be challenging due to the volatile nature of requirements and the task of managing workloads. The Arrange-Act-Assert (AAA) pattern offers a structured methodology to alleviate these issues.
The AAA pattern's adaptability becomes a significant asset when dealing with evolving requirements. Tests built on this pattern can easily be modified to reflect these changes, ensuring the uninterrupted functionality of the software. This adaptability proves crucial in a setting where non-determinism could give rise to flaky tests, i.e., tests that sporadically fail, shaking the trust in the test suite. For example, in Elixir, eight common causes can lead to flaky tests, including certain aspects like using application.put_env
in asynchronous tests, misconfiguring Ecto.SQL.Sandbox
, non-unique data, and more.
The AAA pattern also promotes efficient and effective testing, which is vital to prevent testing from becoming a bottleneck in the development process. A strategy to enhance efficiency is to run tests asynchronously, which can expedite the test suite. However, this method may also increase the likelihood of flaky tests due to the previously mentioned reasons. Therefore, it becomes necessary to weigh the pros and cons and make well-informed decisions based on the specific context and requirements.
To ensure effective testing using the AAA pattern, follow these steps: 1. Arrange: Set up the test environment properly, which includes initializing any necessary objects and setting the system under test's initial state. 2. Act: Perform the action or method call you want to test. This could involve invoking a specific method or simulating user interactions. 3. Assert: Verify the expected outcome or behavior of the system under test. Check whether the actual results match the expected results using appropriate assertions.
In addition to these steps, it's essential to keep tests independent, test all possible scenarios, use meaningful test names, maintain good code coverage, and regularly review and update tests.
Moreover, through the utilization of linting rules for tests, software engineers can identify common mistakes and ensure consistency in the test code. This practice, along with avoiding code repetition by using beforeEach/afterEach blocks and utility functions, can contribute to more efficient and reliable testing.
Incorporating the AAA pattern, together with best practices and careful considerations, can significantly enhance the testing process, culminating in reliable and high-quality software
5. The Role of Automated Testing Tools in Optimizing AAA Pattern Implementation
Embedding automated testing tools into the software development workflow is key in the effective execution of the Arrange-Act-Assert (AAA) pattern. These tools automate the setup and execution of tests, reducing the time and effort required, while offering a plethora of features to enrich the testing process. These features may encompass test reporting and integration with development environments, significantly smoothing the testing process.
More sophisticated tools, like Machinet, can automatically generate unit tests, thus enabling comprehensive test coverage. This feature is especially beneficial for software engineers who often grapple with writing automated tests due to time or resource constraints.
Automated testing tools also present a solution to a frequent software engineering issue - regression errors. These tools can detect such errors, permitting developers to concentrate on their primary tasks. Furthermore, automated testing can cover aspects of the application that are not addressed by unit tests, elevating the overall software quality.
Automated testing tools extend beyond unit testing. They can also be employed for performance testing, aiding in identifying memory or resource leaks. This feature is particularly advantageous as it allows for the same tool and scripts to be utilized for various types of testing.
The advantages of automated testing tools extend beyond the technical domain. These tools also foster collaboration between developers and product managers, enhancing cooperation and feedback loops.
Nevertheless, it's critical to use these tools judiciously. Overdependence on pixel coordinates in GUI testing can lead to problems, as tests can easily and frequently fail, necessitating constant re-recording. To circumvent this, it's advised to use tools that allow for simple test modifications and that support continuous integration.
Automated testing tools can substantially augment the efficiency and effectiveness of the AAA pattern implementation.
Explore Machinet's automated testing capabilities and enhance your AAA pattern implementation!
Whether it's amplifying code coverage, reducing test maintenance, or providing quicker feedback loops, these tools offer a plethora of benefits that can significantly elevate the quality of software products.
By integrating these tools with development environments, developers can effortlessly run tests and receive feedback within their familiar workspace. This assists in identifying bugs and issues early in the development cycle, making the overall development process more efficient and reliable. Moreover, integration with development environments facilitates easier collaboration between developers and testers, enabling them to work together seamlessly within the same environment.
While Machinet.net provides resources and information on unit testing, it is unclear whether they offer automated unit test generation specifically in the AAA pattern. It would be best to navigate the Machinet.net website or reach out to their support team for more information on automated unit test generation in the AAA pattern.
Based on the available context information, there is no direct reference to Machinet being used for automated testing in the AAA pattern. Therefore, it is not possible to provide specific documentation on using Machinet for automated testing in the AAA pattern based on the given context
6. Case Study: Successful Application of AAA Pattern in Unit Testing
The AAA (Arrange-Act-Assert) pattern has been a game-changer for development teams grappling with the complexities of their unit testing processes. This structured approach to testing has simplified the comprehension of tests for developers, emphasizing the importance of single-focus tests. As a result, test maintenance became less daunting. This, in turn, allowed for better test coverage and enhanced the stability of the software applications under development.
In the realm of development tools, GitHub stands out for its comprehensive suite of features and solutions tailored to developers and teams. It provides a robust platform for workflow automation, package hosting, and vulnerability detection. Among its offerings are Codespaces, which offer instant development environments, and Copilot, an AI-powered tool that assists in producing high-quality code and managing code changes. Additionally, GitHub promotes non-code collaboration through discussions and provides an extensive range of documentation and learning resources. GitHub serves a diverse range of users, from enterprise teams and startups to educational institutions, fostering an open-source developer community and offering sponsorship programs to fund open-source initiatives.
An example of a project that has benefited from these tools is "The Diamond Kata." This project utilized property-based testing in the F# programming language and is hosted on GitHub. It has garnered five stars and has not had any forks or pull requests.
Similarly, the Adobe Experience Manager (AEM) project showcases the use of JUnit, AEM mocks, and Mockito for writing integration tests. This project, licensed under the Apache 2.0 license, is written in Java, HTML, and Less. It has attracted two stars and two forks and is maintained by two contributors. At the time of writing, there are no published releases or packages. These real-world examples highlight the potential of the AAA pattern to enhance the quality of unit tests and, consequently, the reliability of software
7. Refactoring and Improving Existing Test Suites Using the AAA Pattern
In the realm of software development, the Arrange-Act-Assert (AAA) pattern is a powerful tool for enhancing the effectiveness of existing test suites. With this pattern, tests are logically divided into three distinct phases - setting up the test (Arrange), executing the code under test (Act), and verifying the outcome (Assert). This clear structure aids in pinpointing areas that can benefit from simplification or optimization, thereby augmenting the maintainability and reliability of tests.
Integrating the AAA pattern into test suites can also streamline the process of identifying and rectifying software issues. This is particularly relevant in the context of Test-Driven Development (TDD), a programming technique that emphasizes writing tests before the actual code. TDD often employs the concept of partitions, dividing a set into subsets to ensure comprehensive testing. This technique can be further enriched by integrating the AAA pattern, which offers an organized approach to handling tests for each partition.
Moreover, the AAA pattern can be leveraged to improve approval testing, an approach that focuses on capturing and storing system behavior. Approval testing is designed with the understanding that tests will inevitably need to change, and thus, it makes the process of updating them straightforward and safe. With the AAA pattern, this process can be further streamlined, as the clear demarcation of different phases of a test makes it easier to identify and approve behavior changes.
It's worth mentioning that approval testing is particularly beneficial for legacy code, and the AAA pattern can be instrumental in retrofitting tests to such code. There are several tools available for approval testing, such as TextTest, Approvals, Retest, and Jest, which can be effectively used in conjunction with the AAA pattern to enhance the testing process.
In conclusion, the AAA pattern is a powerful tool in software development, proving instrumental in refining existing test suites, facilitating Test-Driven Development, and optimizing approval testing. By integrating this pattern into testing processes, developers can better manage and enhance the quality of their software products
8. Balancing Deadlines and Test Quality: Strategies for Efficiently Implementing the AAA Pattern
Maintaining a balance between the urgency of deadlines and the quality of testing is a persistent struggle in software development. The Arrange-Act-Assert (AAA) pattern can be a valuable ally in this battle by promoting a more streamlined and effective testing process. It organizes tests into distinct stages, which not only simplifies their creation and comprehension, but also reduces the time required to write them.
In the typical software development life cycle, delivering features within a two-week sprint is a common scenario. During the first week, while the development team is coding, the Quality Assurance (QA) team prepares test data and artifacts. Ideally, the build should be delivered in the second week. However, delays can occur, leaving the QA team with a limited testing window from Wednesday afternoon until Friday. This is akin to attempting to fit "two gallons of water into a one-gallon jug" in terms of time constraints.
The AAA pattern proves to be a valuable tool in such time-critical situations. It promotes testing one aspect at a time, which ensures that tests are comprehensive and reliable. This, in turn, aids in preserving the quality of tests, even when faced with tight schedules.
Implementing the AAA pattern in testing involves three steps:
- Arrange: Establish the necessary preconditions and initialize any objects or variables required for the test.
- Act: Execute the specific action or operation that is being tested. This could include calling methods, interacting with the system under test, or simulating user actions.
- Assert: Confirm the expected outcome or behavior of the operation. Check if the actual result matches the expected result and manage any assertions or checks accordingly.
By following the AAA pattern, tests become well-structured, easy to read, and maintainable. It aids in separating the different phases of a test, making it clear what is being arranged, acted upon, and asserted.
The article also discusses two approaches to building a test suite - priority-based and context-based. The former entails selecting test cases based on their criticality and priority. In contrast, the latter emphasizes including test cases related to the affected functionality, even if they have a lower priority.
Unforeseen circumstances such as illness or unexpected delays in build delivery can affect the available testing time. However, the AAA pattern can help lessen the impact of these challenges. Failing to address these challenges can have severe consequences, including an increase in production bugs, dissatisfied customers, and burnout among the QA team.
In the ever-changing landscape of software development, where delays and time deficiencies are a reality, the AAA pattern serves as a sturdy tool to navigate these challenges. By promoting efficient testing practices, it ensures the delivery of high-quality software products within the set deadlines
Conclusion
The Arrange-Act-Assert (AAA) pattern in unit testing offers a structured and effective approach to writing tests. By dividing the testing process into three phases - Arrange, Act, and Assert - developers can create more readable and maintainable tests. The Arrange phase sets up the test environment by initializing objects and preparing data, the Act phase executes the code being tested, and the Assert phase verifies the expected outcomes. This pattern has gained popularity among developers due to its simplicity and clarity, leading to improved test coverage and software stability. Integrating automated testing tools with the AAA pattern further enhances the efficiency and reliability of unit tests.
The AAA pattern's benefits extend beyond just improving individual tests. It promotes good testing practices such as focusing on single-functionality tests, ensuring test independence, using meaningful test names, and regularly reviewing and updating tests. Following these best practices while implementing the AAA pattern can significantly enhance the quality of tests and contribute to building robust and reliable software products. To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation here
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.