
Java unit testing is a critical aspect of modern software development, allowing developers to verify the correctness and reliability of their code. Well-written unit tests can catch defects early in the development process, leading to higher code quality and reduced maintenance costs. If you want to learn more about the basics and benefits of unit testing, read the article "Demystifying Unit Testing: Basics and Benefits". In this article, we will focus on best practices for Java unit testing, providing tips and techniques to ensure effective and efficient unit testing in your projects.
Follow the Given When Then (GWT) Pattern
The GWT pattern, which stands for Given, When, and Then, is an effective pattern for structuring unit tests. It provides a clear and organized approach to writing tests, making them easy to comprehend and maintain.
Given: In the Given phase, set up the initial state of the system under test (SUT) by arranging the necessary test data and dependencies. This may involve creating mock objects, initializing variables, and configuring the test environment. This phase sets the foundation for the test and prepares the SUT for the actual testing.
When: In the When phase, trigger the action or behavior of the SUT that is being tested. This may involve invoking a method, calling a function, or performing an operation. This phase should be focused and singular, with only one action being tested at a time.
Then: In the Then phase, verify that the expected behavior has occurred. This may involve checking the return value of the method, asserting that certain conditions are met, or verifying interactions with mock objects. This phase ensures that the SUT behaves as expected and meets the requirements of the test.
Organizing tests according to the GWT pattern promotes readability, maintainability, and consistency across tests. It helps in understanding the purpose of the test and the expected behavior of the code being tested, making it easier to spot issues and make necessary changes.
Machinet, an automated testing tool, generates unit tests following the GWT pattern. It arranges the required test data and dependencies, triggers the action on the SUT, and verifies the expected behavior, all while using popular testing frameworks like JUnit and Mockito. Machinet's generated tests have rich parameterization and necessary mocks for side-effects, resulting in well-structured and organized tests for developers to work with.
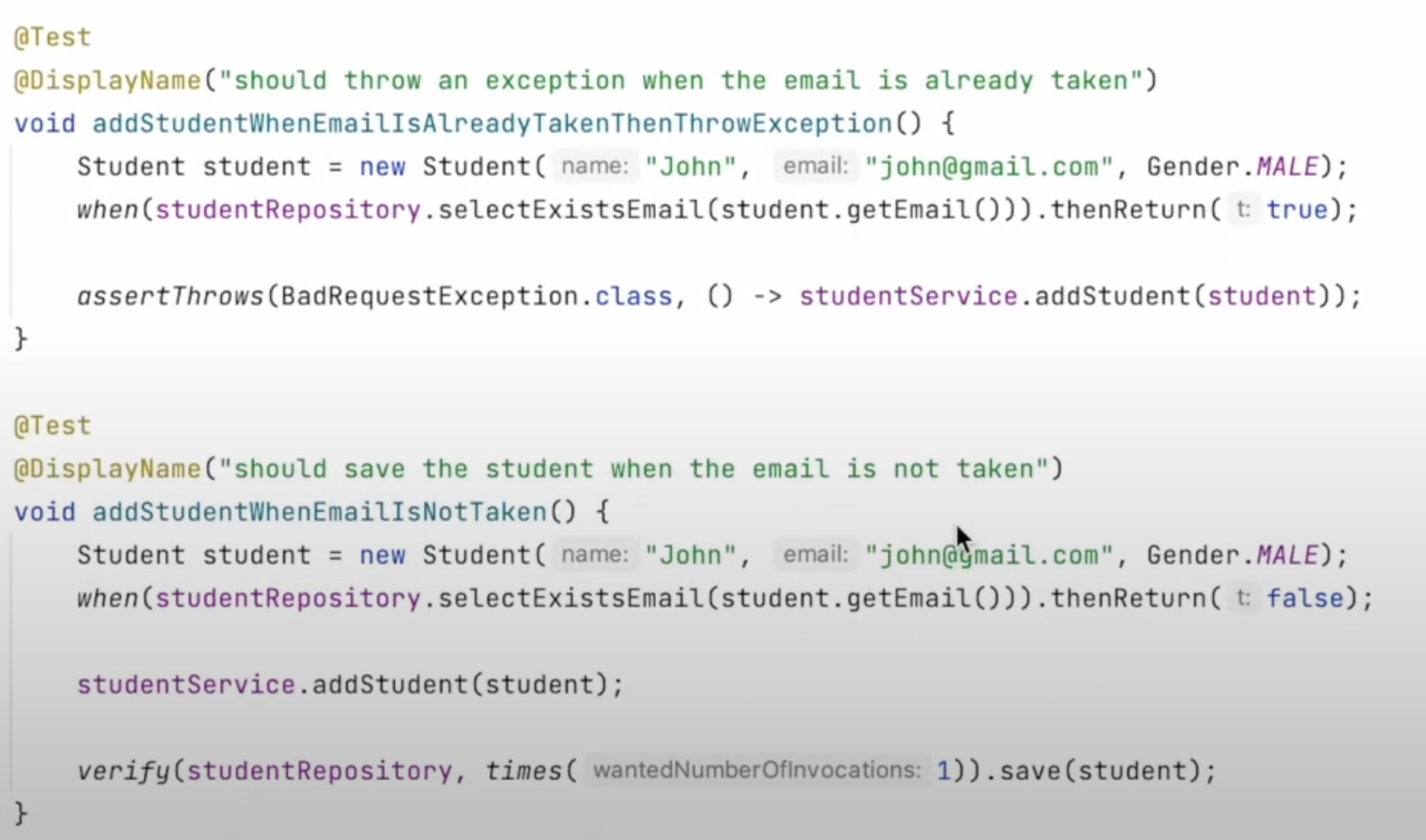
Keep Tests Isolated
One of the key principles of unit testing is to isolate tests from external dependencies, such as databases, web services, or other external systems. This is achieved through the use of mocking frameworks, such as Mockito, which allow developers to create mock objects for dependencies, enabling tests to run in isolation.
Isolating tests from external dependencies has several benefits:
Reliability
External dependencies can introduce variability in test results, leading to unreliable and inconsistent tests. By isolating tests from external dependencies, tests become more reliable, producing consistent results.
Speed
Tests that are not dependent on external systems can run faster, allowing for quick feedback during development. Fast tests enable developers to identify and fix issues more rapidly, leading to faster development cycles.
Flexibility
Isolated tests are more flexible and resilient to changes in external systems. Changes in external systems, such as changes in APIs or database schemas, do not impact the tests, reducing maintenance efforts.
To achieve test isolation, use mocking frameworks, such as Mockito, to create mock objects for dependencies, and configure them to behave as expected during tests. This allows tests to focus on the specific behavior of the SUT, without being affected by the behavior of external systems.
Machinet ensures that tests are kept isolated from external dependencies. It provides an Intellij plugin for Java developers that generates unit tests with mocks for dependencies, allowing tests to run independently without relying on external systems. This helps in achieving reliable and consistent test results, faster test execution for quick feedback during development, and flexibility to handle changes in external systems without impacting the tests.
Test for Both Positive and Negative Scenarios
Unit tests should cover both positive and negative scenarios to ensure comprehensive testing of the code. Positive scenarios test the expected behavior of the code, while negative scenarios test how the code handles unexpected or erroneous situations.
Positive scenarios typically include testing the normal flow of the code, where inputs are within expected ranges and expected results are returned. Negative scenarios, on the other hand, test for edge cases, error handling, and boundary conditions, where inputs are unexpected or out of bounds.
By testing both positive and negative scenarios, you can uncover potential issues, such as unexpected exceptions, incorrect error handling, or unexpected behaviors, ensuring that the code is robust and resilient to unexpected situations.
Machinet generates unit tests that cover both positive and negative scenarios. It automatically writes tests to cover the normal flow of the code with expected inputs and expected results, as well as edge cases, error handling, and boundary conditions with unexpected or out of bounds inputs. This helps in uncovering potential issues and ensuring that the code is robust and resilient to unexpected situations.

Use Descriptive Test Method Names
Choosing descriptive and meaningful names for test methods is important to convey the purpose of the test and the expected behavior of the code being tested. Test method names should be self-explanatory and provide a clear indication of what is being tested. This makes it easier for developers to understand the purpose of the test without having to go through the test code in detail.
Avoid generic or ambiguous names for test methods, as they can lead to confusion and make it difficult to understand the intent of the test. Instead, use descriptive names that clearly indicate what aspect of the code is being tested and what the expected outcome is.
For example, instead of naming a test method "test1()" or "testMethod()", use names like "testCalculateTotalWithValidInputs()" or "testHandleExceptionWithInvalidInputs()". This provides better context and makes it easier to understand the purpose of the test, even without looking at the test code.
Machinet generates test methods with descriptive and meaningful names. It automatically names the test methods based on the aspect of the code being tested and the expected outcome. This makes it easy for developers to understand the purpose of the test without having to go through the test code in detail, improving the readability and maintainability of the tests.
Use Assertions Effectively
Assertions are a powerful tool in unit testing, as they allow you to verify that the expected behavior of the code is met. However, it's important to use assertions effectively to ensure that they provide meaningful feedback in case of test failures.
Here are some best practices for using assertions effectively:
Use specific assertionsΒ
Choose assertions that are specific to the type of data being tested. For example, use assertEquals() for comparing values of primitive types or objects, assertTrue() or assertFalse() for checking boolean conditions, and assertNotNull() or assertNull() for checking object references.
Use descriptive messages
Provide descriptive messages as the last argument in assertions to provide meaningful feedback in case of test failures. For example, assertEquals(expected, actual, "The calculated total is incorrect") provides a clear message indicating the reason for the failure.
Use parameterized tests
Parameterized tests allow you to write tests with multiple input values, reducing the need for duplicate test code. Use parameterized tests to test different scenarios with different input values, and provide descriptive messages for each scenario.
Avoid using too many assertions
Avoid using too many assertions in a single test method, as it can make it difficult to pinpoint the exact cause of a test failure. Keep test methods focused and test only one aspect of the code at a time, with a limited number of assertions.
Keep assertions independent
Ensure that assertions in a test method are independent of each other and do not depend on the order of execution. This allows for better maintainability and makes it easier to identify and fix issues.
Machinet generates assertions effectively in the generated unit tests. It uses specific assertions that are appropriate for the type of data being tested, provides descriptive messages as the last argument in assertions to provide meaningful feedback in case of test failures, and uses parameterized tests to test different scenarios with different input values. This helps in making the generated tests more reliable and informative, aiding in the identification and resolution of issues during testing.
β
Mock External Dependencies
As mentioned earlier, isolating tests from external dependencies is an important practice in unit testing. Mocking frameworks, such as Mockito, allow you to create mock objects for dependencies, enabling tests to run in isolation. Mocking external dependencies has several benefits:
Control the behavior of dependencies
With mocking, you can control the behavior of external dependencies during tests, allowing you to simulate different scenarios and test edge cases. This gives you greater control over the test environment and allows for comprehensive testing.
Reduce test setup complexity
Mocking external dependencies can help reduce the complexity of test setup. For example, if a test requires a database connection or a web service call, mocking those dependencies eliminates the need to set up the actual database or web service during tests, simplifying test setup.
Improve test performance
Tests that rely on external dependencies, such as databases or web services, can be slow and resource-intensive. Mocking those dependencies allows for faster and more efficient tests, as tests do not have to wait for external resources to respond.
When mocking external dependencies, it's important to strike a balance between mocking too much and not mocking enough. Over-mocking can lead to tests that do not accurately reflect the behavior of the real system, while not mocking enough can result in slow, brittle, and unreliable tests. Carefully choose which dependencies to mock and ensure that the mocked behavior is aligned with the expected behavior of the real system.
Some best practices for mocking external dependencies in Java unit testing are:
Use reputable mocking frameworks
There are several mocking frameworks available for Java, such as Mockito, PowerMock, and EasyMock. Choose a reputable and widely used framework that best fits your testing needs and follow the best practices and guidelines provided by the framework's documentation.
Keep mock code simple and focused
Keep the mock code simple and focused on simulating the behavior of the external dependency. Avoid adding unnecessary complexity or logic to the mock objects, as it can lead to confusion and make the tests harder to understand.
Verify interactions with mocks
Use mocking frameworks to verify interactions with mock objects. For example, Mockito provides methods such as verify() to assert that certain methods were called on the mock objects with expected arguments. Verifying interactions with mocks can help ensure that the code under test is interacting correctly with its dependencies.
Use realistic mock behavior
Define realistic behavior for mock objects to accurately simulate the behavior of the external dependencies. Avoid using overly simplistic or unrealistic behavior, as it may not reflect the real-world behavior of the dependencies and can lead to false-positive or false-negative test results.
Update mocks with changes in dependencies
Keep the mock objects updated with any changes in the external dependencies. If the behavior of the external dependency changes, update the mock objects accordingly to reflect the updated behavior in your tests.
Machinet generates unit tests with mocks for external dependencies. It automatically identifies external dependencies in the code being tested and generates mock objects for them, allowing tests to run in isolation without needing to connect to actual external systems. This helps in creating reproducible and consistent tests, preventing interference with external systems during testing, and reducing dependencies on external resources, making tests faster and more reliable.
Conclusion
Java unit testing is a critical part of software development that helps in ensuring the quality, reliability, and correctness of the code. Following best practices for Java unit testing, such as writing effective test cases, using appropriate frameworks and tools, mocking external dependencies, achieving high test coverage, and practicing TDD, can greatly enhance the effectiveness of your tests and improve the overall quality of your code.
By incorporating these best practices into your Java unit testing process, you can catch and fix issues early in the development cycle, reduce the likelihood of introducing new bugs, and ultimately deliver more robust and reliable software to your users.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.