Unit testing is an essential practice in modern software development that ensures the quality and reliability of code by verifying that individual units, or components, of a software system function as expected.
In this article, we will explore the basics of unit testing, including the definition of a unit, the purpose and benefits of unit testing, and commonly used frameworks and tools for Java unit testing, such as JUnit and Mockito.
What is a Unit?
A unit in the context of unit testing refers to the smallest testable part of a software system, typically a single function or method. It can also be a class, a module, or a component, depending on the granularity of the system being tested. The key idea is to isolate and test individual units in isolation, independent of their dependencies, to ensure that they function correctly according to their specifications.
The key idea is to isolate and test individual units in isolation, independent of their dependencies, to ensure that they function correctly according to their specifications.
Purpose of Unit Testing
The primary purpose of unit testing is to catch and fix defects or bugs in the code as early as possible in the development process, before they propagate and cause issues in other parts of the system.
Unit tests serve as a safety net that provides rapid feedback on the correctness of individual units, helping developers identify and fix problems quickly and efficiently.
Additionally, unit testing helps improve the maintainability and modifiability of code by providing a safety net for refactoring, allowing developers to confidently make changes to the codebase without introducing unintended consequences.
Benefits of Unit Testing
Unit testing offers numerous benefits to software development teams, including:
- Early detection of defects: By testing individual units in isolation, unit testing helps catch defects early in the development process, reducing the cost and effort required to fix issues that would have been more challenging to identify and fix in later stages of development or in production.
- Faster development and debugging: Unit tests provide rapid feedback on the correctness of code, enabling developers to identify and fix issues quickly, reducing the time spent on manual testing and debugging.
- Improved code quality: Unit testing promotes good coding practices, such as modular and maintainable code, by enforcing the separation of concerns and encapsulation of functionality in individual units. This helps improve code quality and reduces the likelihood of introducing defects.
- Better collaboration among team members: Unit tests serve as executable documentation that outlines the expected behavior of units, making it easier for team members to understand and collaborate on the codebase. Unit tests also serve as a safety net for catching regressions introduced by changes made by other team members, promoting collaboration and accountability.
- Faster and safer refactoring: Unit tests provide confidence in the correctness of code, making it safer and easier to refactor or modify code without introducing unintended consequences. This promotes maintainability and allows teams to evolve their codebase with confidence.
- Cost-effective bug fixing: Catching defects early with unit tests helps reduce the cost of fixing bugs, as it is typically easier and less time-consuming to fix issues in isolated units compared to identifying and fixing issues in integrated systems.
Commonly Used Java Unit Testing Frameworks and Tools
There are several popular frameworks and tools available for Java unit testing. Let's take a closer look at some of the commonly used ones
JUnit
JUnit is one of the most widely used testing frameworks for Java applications. It provides a rich set of annotations, assertions, and test runners for writing and executing tests. JUnit follows a simple and intuitive syntax for writing tests, making it easy for developers to adopt and use.
Mockito
Mockito is a powerful and widely used mocking framework for Java unit testing. It allows developers to create mock objects to simulate the behavior of dependencies or external components during unit testing. Mockito provides a clean and intuitive API for creating mocks, stubs, and spies, making it easy to isolate units for testing and verify their interactions with dependencies.
Other frameworks and tools
In addition to JUnit, and Mockito, there are other popular Java unit testing frameworks and tools such as AssertJ, PowerMock, and EasyMock, TestNG, among others. These frameworks offer different features and capabilities, and teams may choose the one that best fits their specific testing needs and requirements.
How to write Java Unit Tests Automatically with Machinet AI?
Machinet is an AI assistant for Java developers that helps them push productivity with automated unit test generation. It provides an Intellij plugin for Java developers that generates unit tests for all commonly used Java Unit Testing Frameworks we mentioned above.
TLDR; You need to click on the automatically identified test unit and it creates a test. Simple as that.

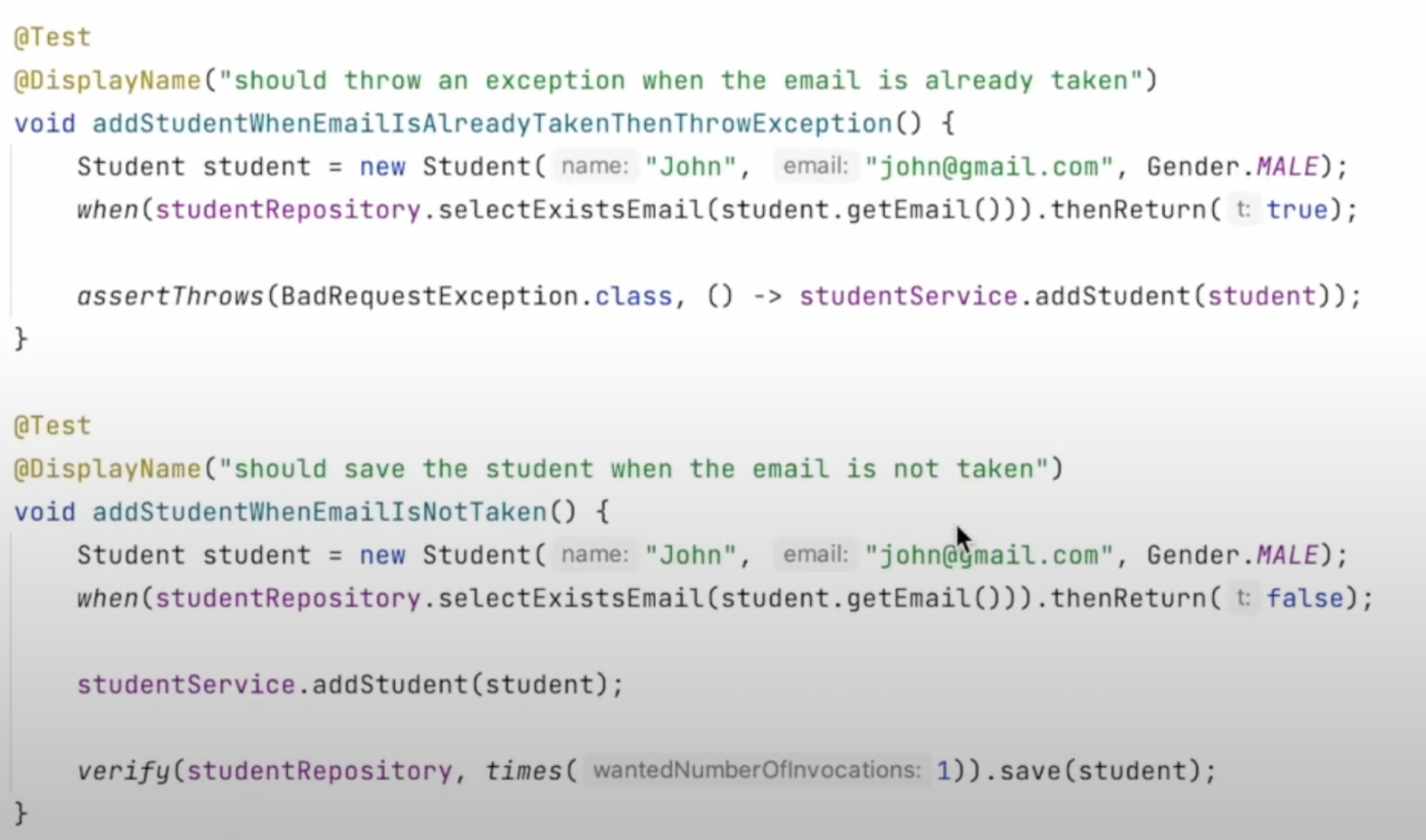
… and this is how Machinet writes unit tests in 3 easy steps:
Step 1. Identify the Unit to be Tested
Machinet's plugin scans the Java codebase in the Intellij IDE and identifies the units, such as methods or functions, that need to be tested.
Step 2. Generate Test Skeleton
Once the units to be tested are identified, Machinet automatically generates a test skeleton for each unit. The test skeleton includes the necessary imports, annotations, and method signatures required for writing unit tests. It also sets up the initial test structure with placeholders for the test code.
Step 3. Generates Unit Tests with Mocks, Assertions
Machinet utilizes its AI capabilities to automatically generate parameterized test cases for each unit, providing comprehensive test coverage. It also generates necessary mock objects for dependencies using popular Java mock frameworks like Mockito, simulating their behavior during unit testing and allowing for isolated testing of the unit under test. Additionally, Machinet automatically adds assertions to verify expected output and verifies interactions between the unit and its dependencies using Mockito's verification features, ensuring correct interaction during test execution.
In conclusion, Machinet's automated unit test generation feature simplifies the process of writing comprehensive and effective unit tests for Java developers. By automatically generating test skeletons, parameterized test cases, and necessary mocks, Machinet empowers developers to quickly and efficiently create reliable unit tests that catch defects early, improve code quality, and promote maintainability, ultimately leading to more robust and reliable software systems.
Conclusion
Unit testing is a fundamental practice in modern software development that helps ensure the quality, reliability, and maintainability of code. By testing individual units in isolation, unit testing catches defects early, improves code quality, promotes collaboration among team members, and enables faster and safer refactoring.
Several popular frameworks and tools are available for Java unit testing, such as JUnit and Mockito, offering different features and capabilities to support effective unit testing. Incorporating unit testing into the development process can greatly enhance the overall quality of software systems and contribute to the success of software projects.