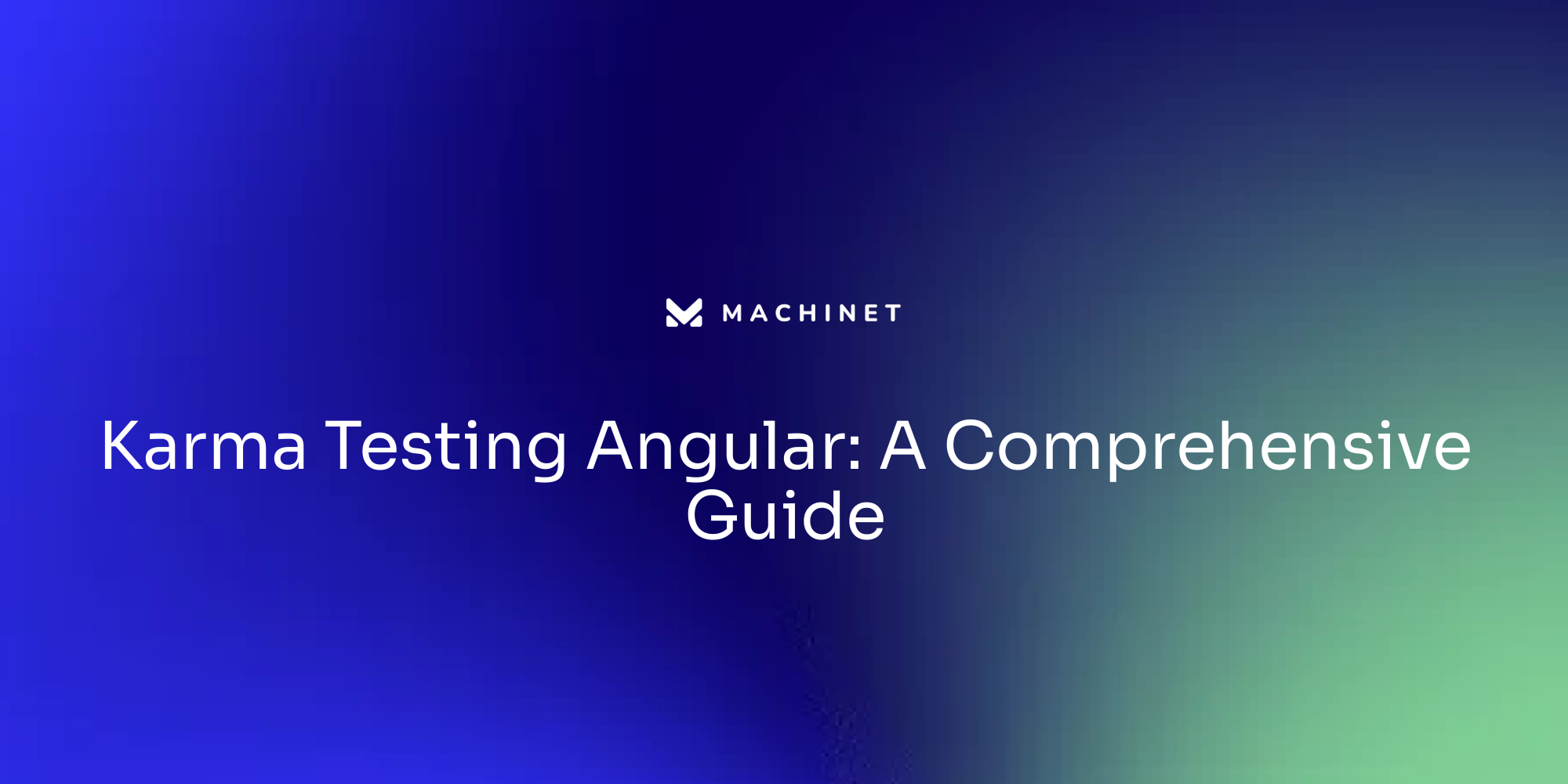
Introduction
Setting up a robust testing environment is essential for ensuring the quality and stability of your Angular application. In this article, we will guide you through the process of setting up your Angular testing environment using Karma, a powerful test runner that seamlessly integrates with Angular. You will learn how to install Node.js and npm, set up Karma configuration, write and execute tests, and incorporate best practices for unit testing.
We will also explore advanced testing scenarios, troubleshooting common issues, and the importance of continuous integration in Angular testing. By following these steps and strategies, you can enhance the reliability and maintainability of your Angular application, keeping up with the latest practices in the dynamic landscape of Angular development.
Setting Up Your Angular Testing Environment
Establishing a strong testing environment is crucial for guaranteeing the quality and stability of your application. Here's a stepwise guide to get you started with Karma, a powerful test runner that works seamlessly with Angular:
- Begin by installing Node.js and npm, the fundamental building blocks for modern JavaScript development.
- Move to the directory of your project, or create a new one if you're just getting started.
- To install Karma and its related packages, enter the command below in your terminal:
bash
npm install karma karma-cli karma-jasmine karma-chrome-launcher --save-dev
- Next, initialize a Karma configuration file with:
bash
karma init
- Follow the configuration prompts, selecting Jasmine as your testing framework, as it's specifically designed to work with the framework called Angular.
- Adjust the generated Karma configuration file to correctly represent the file paths of your Angular project's source and examination files.
- With the configuration finished, you can now start writing and running evaluations, utilizing Karma's features to improve the quality of your code.
Remember, well-structured unit tests are a testament to high coherence within your application's domains, ensuring that changes are isolated and don't adversely affect other parts of the system. Embracing this approach not only streamlines your deployment process but also significantly lowers the mean time to production (MTTP).
In the evolving landscape of modern web development, staying updated with the latest practices is crucial. The recent release of Angular 17.3 introduces significant changes that could impact your testing strategy. Incorporating these updates into your workflow can keep your skills sharp and your applications cutting-edge.
Understanding Jasmine and Karma
Jasmine is a popular framework for behavior-driven development (BDD) that aids in writing clean and understandable evaluations for JavaScript, utilizing a rich set of features to define and perform assessments. By employing a readable syntax, it simplifies the testing process, making it more approachable for developers.
Karma, on the other hand, functions as a versatile runner. It enables the performance of assessments across various browsers, establishing a setting that closely duplicates user engagement with the software. Its command-line interface is designed for efficiency, reporting results in a detailed manner to help identify issues swiftly.
The partnership between Jasmine and Karma is particularly effective for projects utilizing the Angular framework. Jasmine establishes the groundwork with its framework, while Karma focuses on test execution, providing real-browser environments and detailed reports. This combination enables comprehensive examination of applications developed with Angular, guaranteeing strength and dependability.
Furthermore, the testing capabilities of this framework are being enhanced with emerging tools. The web-test-runner, currently in experimental stages, shows promise for future integration with Jasmine, allowing developers to run tests without modifying existing test suites.
With the rapid evolution of the JavaScript framework, staying updated on the latest tools and frameworks is crucial. The most recent release of version 17.3 introduces new features that improve the development experience. Evaluating remains a crucial part of this process, and developers are encouraged to explore new advancements in this area, such as those highlighted by experts like Rainer Hahnekamp in discussions about Angular's examination future.
In a landscape where assessment is integral to software development, with 80% of professionals acknowledging its importance, tools like Jasmine and Karma play a critical role. They not only back the creation of high-quality apps, but they also follow industry trends where automated checking and effective design are more and more embraced by developers.
As technology progresses, particularly with the integration of AI in development workflows, tools like Jasmine and Karma will continue to evolve, assisting developers in delivering exceptional software that meets the high standards expected by users worldwide.
Creating Test Files with Angular CLI
Generating files for testing using the CLI of a popular JavaScript framework is a critical step to ensure the proper functionality of your application's components and services. To generate a file for a specific element, like a component, just open your terminal, go to your project directory, and run ng generate component-name --spec=true
, replacing component-name
with the name of your component. This command creates a .spec.ts
file in the component's folder, providing a foundational testing setup.
After the Angular CLI has created the file, you can start writing your assessments using the Jasmine framework. The command line interface conveniently imports necessary modules and provides initial trial cases to kickstart your evaluation process.
Take into account the scenario of evaluating a Product Component
that relies on a ProductsListComponent
and utilizes a service to retrieve product data. The default tests have already been generated by the framework, but you will need to enhance them to encompass the interactions between the components and service.
In view of the rapid evolution of Angular, exemplified by the recent release of Angular 17.3, staying current with practices for evaluation is more important than ever. The new features and capabilities introduced in the framework can significantly impact how you write and manage your tests.
Furthermore, according to the 2023 Developer Ecosystem Survey with insights from over 26,000 developers, JavaScript and TypeScript remain dominant in the industry. Since applications developed using these languages are mainly constructed, it becomes even more crucial for developers to grasp the process of evaluating in this scenario.
Embrace the process of learning testing for the framework by utilizing the tools and resources available to you. Share your progress and insights with the community to promote collaborative learning and growth in the framework.
Running Tests with Karma
Karma, as a crucial component of your development toolkit, allows you to run assessments effortlessly. To set it in motion, follow these simple steps:
- Using your terminal or command prompt, navigate to your Angular project's root directory.
- Start the examination procedure by inputting the 'ng examination' directive. This action ignites Karma into motion, launching browsers as set or defaulting to the system's primary one to perform your experiments.
- Observe the outcomes directly in your terminal; they will inform you about which assessments have succeeded and which have faltered.
- Karma's default behavior is to keep a vigilant watch over your source and trial files, rerunning trials automatically at the slightest change, ensuring your program remains bug-free.
With the recent Angular 17.3 release, incorporating such tools and practices is more relevant than ever, considering the enhancements and new features aimed at improving development efficiency. Additionally, adopting test-driven approaches such as BDD and TDD—where creating tests is intertwined with creating code—can result in more resilient and regression-proof applications. In the realm of automated examination, Test distinguishes itself as a flexible framework accommodating a range from unit to integration evaluation, emphasizing the significance of annotation-driven examination organization.
Statistics indicate that 80% of developers recognize the crucial role of software validation in software development, with 58% utilizing automated assessments. It's clear that a well-structured testing strategy is not merely a formality but a cornerstone of quality software.
Best Practices for Angular Unit Testing
To craft effective and maintainable unit tests for your Angular application, consider these refined strategies:
- Emphasize Test Specificity: Each test should target a distinct behavior or scenario to prevent overlap and confusion.
- Descriptive Naming: Use clear, descriptive names for your assessments to convey their purpose at a glance.
- Comprehensive Scenarios: Test the expected behaviors as well as the edge cases to ensure your code is robust against various conditions.
- Isolate with Test Doubles: Employ mocks or stubs for dependencies when examining components or services to focus on the unit under examination.
- Autonomy is Crucial: Develop your assessments to operate autonomously from each other to prevent cascading breakdowns and streamline problem-solving.
- Sustain and Modify: Continuously assess and improve your assessments to match the changing application, guaranteeing their ongoing efficacy.
By implementing these techniques, you can improve the strength and dependability of your unit assessments, resulting in early bug identification and a codebase of better quality. Furthermore, embracing testability as a core principle—characterized by modularity, clarity, and independence—can significantly improve your testing efforts and code quality.
Testing Asynchronous Code
It is crucial to assess asynchronous code in applications, and there are various approaches to guarantee the durability and dependability of your unit assessments. Angular's built-in async
and fakeAsync
utilities are invaluable for handling asynchronous operations like HTTP requests or timers in a way that mimics synchronous execution. This makes your assessments simpler to compose and comprehend.
Another technique is using Jasmine's done
callback, which is essential for testing code dependent on callbacks or promises. By utilizing done
, you can indicate when an asynchronous examination is finished, guaranteeing examinations run to completion before proceeding.
Mocking asynchronous dependencies is another powerful strategy. By using mocks or stubs, you can simulate the behavior of dependencies like HTTP services, providing you control over the timing and responses in your evaluation environment.
For codebases that employ Promises, the modern async
and await
syntax is your ally, allowing you to write more readable tests. Wrap your asynchronous code with async
functions and use await
to pause execution until Promises resolve.
Incorporating these techniques into your strategy not only enhances the dependability of your code but also aligns with the recommended practices observed in the recent Angular 17.3 release, which emphasizes improved evaluation capabilities. As emphasized by developers, the ability to test code is crucial, and these methods contribute to a codebase that is modular, clear, and independent, as reaffirmed by 80% of developers who recognize the essential role of assessment in software projects. With 58% of those involved in evaluation also developing automated evaluations, it's clear that adopting these approaches can significantly streamline your evaluation process.
Configuring Karma for Angular Testing
Customizing Karma to meet the testing requirements of your Angular project is an essential measure in sustaining a strong and effective testing environment. By customizing Karma's configuration, you can ensure that your software is tested in a manner that reflects its real-world usage and your team's specific requirements.
-
Browsers: Karma's flexibility allows you to specify the browsers where your evaluations will execute. Although Chrome is the default option, adjusting the settings to include browsers like Firefox or Safari can ensure cross-browser compatibility and a better understanding of how your application behaves across different platforms.
-
File Patterns: Managing your files and source code is simplified with Karma's ability to recognize file patterns. This feature is especially helpful for excluding irrelevant files or directories from your suite, ensuring that only pertinent code is considered for coverage.
-
Reporters: The quality and readability of reports are paramount, and Karma supports a variety of reporters to suit your needs. From the standard
progress
report to the more comprehensivecoverage
report, you have the option to select the one that best fits your project's requirements for code coverage analysis. -
Code Coverage: Speaking of coverage, Karma's code coverage reports are crucial in evaluating the comprehensiveness of your assessments. By configuring settings such as coverage thresholds and report generation locations, you gain valuable insights into potential gaps in your test suite.
-
Plugins: Extending Karma's functionality is straightforward with the use of plugins. These extensions enhance the evaluation experience by introducing characteristics like additional browser starters or customized journalists, enabling you to create an evaluation structure that aligns flawlessly with your app's requirements.
By thoughtfully configuring Karma, you pave the way for a testing process that is not only tailored to your web framework but also adaptable to the evolving landscape of software development, as evidenced by the recent advancements in Angular versions and the integration of AI tools in the development process.
Using TestBed for Unit and Integration Tests
The Testbed of AngularJS is a dynamic testing utility that simplifies the setup of modules for unit and integration testing. It simplifies the process of creating an environment similar to that of your application running in a browser. Here are the steps to effectively utilize TestBed in your Angular testing:
- Begin by importing the necessary modules from
@angular/core/testing
and@angular/platform-browser-dynamic/testing
. - Utilize
Testbed.configureTestingModule()
to set up your testing module. This versatile method allows you to specify dependencies, declarations, and imports, thereby replicating the module's actual environment. - Create an instance of the component or service under examination with
TestBed.createComponent()
or obtain the instance usingTestbed.get()
. These instances provide you with aComponent Fixture
which gives you access to the component instance and the ability to interact with its properties and methods. - Invoke
fixture.detectChanges()
to initiate change detection, which updates the component's view. This step is crucial for components that depend on Angular's data binding or other features necessitating change detection. - Frame your tests using Jasmine's straightforward syntax, leveraging
fixture.componentInstance
to delve into the component's properties and methods.
By incorporating TestBed, developers can create a controlled evaluation environment that closely mirrors actual component behavior, fostering high-quality, reliable software. This aligns with the principles of testable code, which emphasize modularity, clarity, and independence—attributes that permit efficient defect detection.
Furthermore, recognizing the importance of low coupling and high coherence, Testbed aids in isolating components, allowing developers to verify that changes within a domain do not adversely affect others. This approach to testing is a testament to the evolving practices in web development, as it supports the latest enhancements in the framework without necessitating a complete overhaul.
The ecosystem of Angular keeps flourishing with updates like version 17.3, which brings in new features that highlight the framework's dedication to contemporary, sturdy, and scalable applications. With the growing intersection of Angular and AI-assisted development, tools like Testbed are instrumental in ensuring that developers can keep pace with these rapid advancements, as noted by industry experts and community leaders.
Statistics validate the importance of assessment, with 80% of developers recognizing its crucial function in development projects. Automated tests are developed by 58% of those engaged in test activities, and 46% include test case design in their process. Testbed, in this context, serves as an essential tool in the examination arsenal of AngularJS, aligning with modern examination practices that drive the creation of reliable and sustainable codebases.
In summary, TestBed offers a robust framework for assessing Angular, ensuring that your components and services are thoroughly verified in an environment that mirrors real-world scenarios, thereby contributing to the overall stability and maintainability of your applications.
Advanced Testing Scenarios
Mastering advanced testing scenarios in JavaScript framework ensures that as your application complexity increases, the stability and maintainability of your codebase remain intact. Consider the following strategies for intricate test cases:
-
Component Input/Output Testing: Testing Angular components with input and output properties can be achieved by manipulating the
component Instance
property of theComponentFixture
. This simulates user interactions, allowing you to set input values and subscribe to output events, ensuring that the component reacts as expected. -
Routing-Related Component Testing: For components tied to routes within Angular's Router, configure the testing environment to mimic navigating to these routes. This strategy verifies that upon route changes, components are properly rendered, reflecting a real user's journey through the application.
-
Form-Driven Component Testing: Components utilizing Angular forms benefit from testing with the
FormsModule
andReactiveFormsModule
. Create form controls and simulate user input to evaluate the correctness of form validations and the form submission behavior. -
Service Dependency Testing: Angular services frequently depend on other services or external APIs. Take advantage of Angular's strong dependency injection system to replace actual dependencies with mocks or stubs, isolating the service being evaluated and focusing exclusively on its functionality.
These techniques draw inspiration from the forensic code analysis methods described by Adam Tornhill, which use source code management history to identify complex, frequently changed 'hotspots.' These hotspots, indicative of areas with architectural weaknesses, can lead to instability and long-term maintenance challenges. By understanding the relationships within your codebase, such as the coupling between changes and the coherence within domains, you can craft more effective tests that reflect the intricacies of your application's architecture.
Recent progress in Angular and the development tools that surround it, including updates to official documentation and functionalities that utilize the newest browser features, have further simplified the evaluation process. Extensive learning materials, frequently involving practical coding exercises, offer a blueprint for implementing these strategies in your projects.
Statistics from industry surveys highlight the growing focus on quality assurance, with organizations dedicating significant portions of their development budget to quality control. For example, 40% of large-scale companies allocate more than 25% of their budget to quality assurance, highlighting the importance placed on quality in digital experiences.
Embrace these advanced evaluation scenarios to fortify your web framework, ensuring that it remains robust, maintainable, and high-quality, even as it scales and evolves.
Continuous Integration with Angular Testing
Establishing Continuous Integration (CI) for your application improves code quality and stability by guaranteeing regular and automated testing. To begin, choose a CI platform that is compatible with Angular evaluations, such as Jenkins, Travis CI, or CircleCI. Afterwards, set up the selected platform to replicate your project repository, install necessary components, and run assessments using Karma by specifying required instructions in a build script or pipeline. It's crucial to set the CI to initiate a build and evaluation sequence with each code push to the repository, allowing for immediate detection of any integration issues or bugs. CI platforms often include interfaces to review reports and coverage metrics provided by Karma, aiding in quick identification and resolution of problems.
Continuous Integration is more than a set of practices; it's a synergy of automated integration, frequent commits, and collaborative efforts that underpin modern software development. Embracing CI means adopting a culture where quality and efficiency are paramount, and every team member's contribution is vital. The automated execution of tests upon each integration not only accelerates development cycles but also reinforces the reliability of the software. With CI, you can detect defects early, significantly reducing the cost and effort needed for fixes. As technology advances quickly, incorporating continuous evaluation into your CI/CD pipeline is crucial to sustain the rate of innovation and guarantee the delivery of high-quality software.
Troubleshooting Common Testing Issues
Navigating common challenges during Angular testing can be a critical skill for software engineers. For example, if scenarios are not passing, it is crucial to examine the error messages and stack traces from Karma, as they may reveal problems like inaccurate assertions or flaws in the code. Additionally, addressing configuration issues necessitates a thorough examination of karma.conf.js
to validate the correctness of syntax and settings, ensuring that source code and file paths are accurately specified.
Browser-specific failures necessitate the installation and proper configuration of the required browser launchers within Karma. This step is crucial to address compatibility issues, which may also involve extra dependencies. When facing sluggish execution, optimizing the suite is advisable, possibly by curtailing superfluous dependencies or employing doubles to focus on the specific code under examination. Modifying Karma to run assessments simultaneously or on various browsers could greatly enhance performance too.
Lastly, generating code coverage reports correctly is a matter of configuring Karma with the appropriate settings, including coverage thresholds and report directories. Overcoming these common testing hurdles ensures the smooth operation of Angular tests, leading to reliable outcomes that reflect the software’s quality.
Conclusion
In conclusion, setting up a robust testing environment using Karma is crucial for ensuring the quality and stability of your Angular application. By following the stepwise guide provided, you can easily install Node.js and npm, configure Karma, write and execute tests, and incorporate best practices for unit testing.
Staying updated with the latest practices in Angular development is emphasized throughout. The recent release of Angular 17.3 introduces significant changes that can impact your testing strategy. By incorporating these updates, you can keep your applications cutting-edge.
The article also highlights best practices for Angular unit testing, such as emphasizing test specificity and using descriptive naming. Testing asynchronous code is also covered, providing strategies for robust and reliable testing.
Advanced testing scenarios, including component input/output testing and service dependency testing, are discussed. These strategies help maintain the stability and maintainability of your codebase.
Continuous integration is emphasized as an essential aspect of Angular testing. By setting up continuous integration using platforms like Jenkins or Travis CI, you can ensure regular and automated testing, enhancing code quality and stability.
Finally, the article provides troubleshooting tips for common testing issues, enabling you to address challenges effectively.
By following the steps, best practices, and strategies outlined in the article, you can create a robust testing environment for your Angular application. This will enhance the reliability and maintainability of your codebase, keeping up with the latest practices in Angular development.
Start creating a robust testing environment for your Angular application today!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.