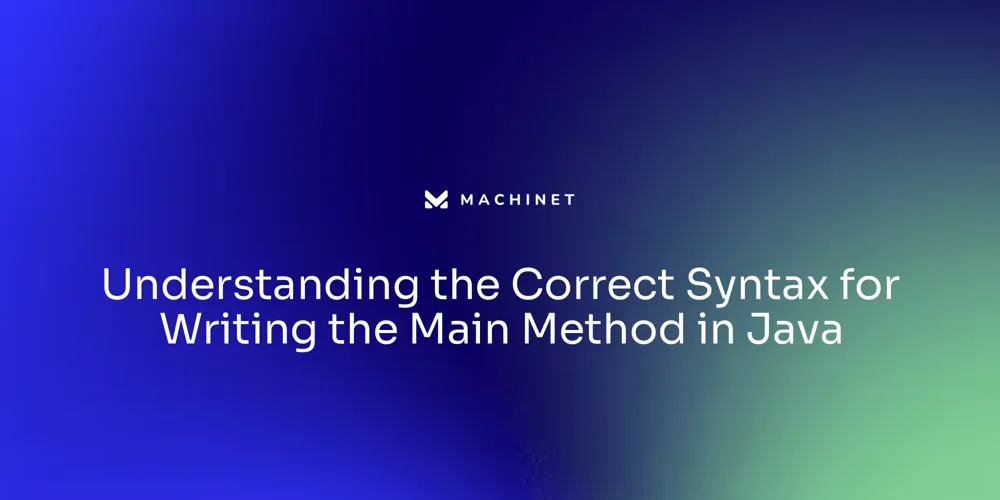
Table of Contents
- Syntax and Structure of the Main Method
- Understanding the Role of Public, Static, and Void Keywords
- The Purpose of the String[] Args Parameter
- Examples of Main Methods in Java Programs
Introduction
Java's main method is the gateway to program execution, serving as the starting point for launching your application. It follows a strict blueprint, with keywords like public
, static
, and void
, making it complex for beginners. However, with the introduction of Java 21, the entry point has been simplified, aligning Java with other languages.
This evolution aims to ease the learning curve and allow developers to focus more on the task at hand. In this article, we will explore the syntax and structure of the main method, understand the role of keywords, and discuss the purpose of the String[] args
parameter. We will also look at examples of main methods in Java programs to illustrate their usage and highlight the recent changes in Java.
Syntax and Structure of the Main Method
Java's main method is the gateway to program execution, a starting point that the Java Virtual Machine (JVM) invokes to launch your application. It follows a strict blueprint, which is mandatory for the JVM to recognize it as the entry point.
The syntax is quite straightforward:
java
public static void main(String[] args) {
// Your code goes here
}
The public
keyword makes the method accessible from anywhere, static
allows it to be called without creating an instance of the class, and void
signifies it returns no value. The String[] args
parameter is an array that stores command-line arguments.
Understanding this method is crucial for Java beginners, but it's also seen as a hurdle due to its complexity. Java 21 introduces a change that simplifies this entry point, making it easier for novices to get started with Java programming.
It aligns Java with other languages where the entry point isn't necessarily a method but a block of code. Now, you can write less verbose code, focusing more on the task at hand.
For instance, to print 'Hello World' in the console, the pre-Java 21 approach required a class with a main method, but with Java 21, you can achieve this with a more concise method signature. This evolution of Java aims to ease the learning curve by gradually introducing new programmers to fundamental concepts before advancing to more complex topics. Methods, encapsulating a set of related instructions, are the building blocks of Java code. They should adhere to the Single Responsibility Principle, ensuring clarity and preventing complexity. Clean and maintainable Java code is essential for effective communication with computers and fellow developers, making it vital to write code that is not only functionally correct but also easy to read and modify.
Understanding the Role of Public, Static, and Void Keywords
In Java, the main()
method traditionally serves as the linchpin for any application, marking the entry point where the Java Virtual Machine (JVM) springs into action. It's crucial to understand the significance of the keywords public
, static
, and void
in its declaration. The public
keyword ensures that the main()
method is universally accessible, bridging the gap between various classes and methods.
With static
, we denote that the method is a class-level attribute, thus eliminating the need for an object instance to invoke it. Lastly, void
communicates that our main()
method is designed to execute actions without returning any value. However, the landscape of Java is evolving with Java 21, where the stringent requirement of the public static void main()
method is now a relic of the past.
This change caters to simplifying Java for newcomers, as the traditional main method often posed as a daunting hurdle. The main method's specific structure—being public
, static
, void
, and accepting a String
array—can be overwhelming for beginners. Moreover, this shift aligns Java with other programming languages where the entry point isn't a designated method but rather an initial block of code.
A fascinating development in Java 21 allows the use of unnamed classes, enabling developers to craft modular programs composed of discrete, functional segments without the formal declaration of a class. This not only streamlines the learning curve but also fosters a more intuitive understanding of Java applications. As Java continues to adapt, it's clear that the language is striving for simplicity, inclusivity, and harmony with broader programming paradigms.
The Purpose of the String[] Args Parameter
In Java, the main method serves as the gateway to program execution. It contains an essential parameter, String[] args
, which is designed to receive an array of command-line arguments when the program is launched.
These arguments, often used for configuration or input, are strings that can be harnessed to tailor the behavior of the Java application. They allow for a level of customization that can be critical in the development of complex systems or during the initial stages of a project, where rapid iteration and frequent changes are common.
This flexibility is also evident in applications like the Apache Commons CLI, where command-line parsing is streamlined for efficiency and ease of use. Moreover, the recent enhancements in Java libraries, such as JobRunr, underscore the ongoing evolution of Java, reflecting its adaptability and the vibrant community that continually enriches it. The args
parameter exemplifies Java's capability to handle large-scale applications while still being accessible for smaller, exploratory projects. As Java progresses, with updates such as Spring Session 3.3.0 and Spring Shell's new features, it remains a language that can start simple but is built to grow with the developer's needs and ambitions.
Examples of Main Methods in Java Programs
The main
method in Java serves as the crucial launchpad for program execution, initiating the sequence of operations when the Java Virtual Machine (JVM) springs into action. A quintessential example of its use is in the classic 'HelloWorld' program, which is the rite of passage for all new Java learners.
It's a simple demonstration of the language's capabilities, where a single line of code outputs the phrase 'Hello, World!' to the console:
```java public static void main(String[] args) { System.out.println("Hello, World!
"); } ```
This example is more than just tradition; it verifies that the Java setup is correctly configured. Furthermore, the main
method is not limited to such straightforward tasks.
It can also handle command-line arguments, providing a dynamic edge to Java applications. Consider the following code snippet that illustrates the processing of command-line inputs, displaying the number of arguments received and echoing them back individually:
java
public static void main(String[] args) {
System.out.println("Number of arguments: " + args.length);
for (String arg : args) {
System.out.println(arg);
}
}
Despite its foundational status, the main
method's complexity has been a stumbling block for beginners.
Its strict signature—public static void main(String[] args)
—requires new developers to juggle multiple concepts simultaneously. However, with the introduction of Java 21, a significant simplification was made: the main
method is no longer a prerequisite for running Java programs. This change aligns Java with other modern languages, where the entry point isn't always a designated method. It also resonates with functional programming styles that favor smaller, reusable functions over a central main
method. This evolution in Java reflects an ongoing commitment to making the language more accessible and consistent with contemporary programming paradigms.
Conclusion
In conclusion, Java's main method serves as the entry point for launching applications. With the simplification introduced in Java 21, the entry point is now more beginner-friendly and aligned with other languages.
Understanding the syntax and structure of the main method is crucial. The keywords public
, static
, and void
ensure accessibility and eliminate the need for object instances.
However, with Java 21, these keywords are no longer mandatory. The String[] args
parameter in the main method allows customization through command-line arguments.
This flexibility caters to both large-scale applications and smaller projects. Examples of main methods demonstrate their usage, from simple output statements to dynamic command-line input processing. These changes make the main method less complex and align it with modern programming paradigms. In summary, Java's main method has been simplified in Java 21 to make it easier for beginners. As Java continues to evolve, it remains a language that can start simple but adapt to developers' needs and ambitions.
Try out Java 21 and experience the simplified main method for yourself!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.