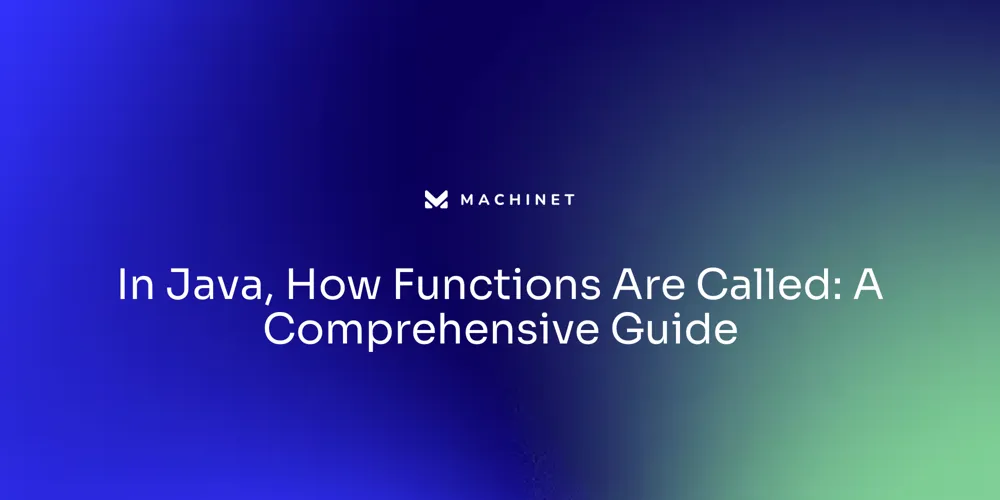
Table of Contents
- Declaring and Calling Methods in Java
- Method Parameters and Return Types
- Types of Methods: Instance and Static Methods
- Method Overloading and Method Overriding
- Best Practices for Using Methods in Java
- Common Errors and Troubleshooting in Method Calls
- Advanced Topics: Abstract Methods and Factory Methods
Introduction
Java methods serve as essential building blocks within the programming language, encapsulating a sequence of statements to perform a specific operation. This article explores the declaration and calling of methods in Java, as well as method parameters, return types, and the distinction between instance and static methods.
The concept of method overloading and method overriding is also discussed, along with best practices for using methods. Common errors and troubleshooting tips related to method calls are addressed, and finally, advanced topics such as abstract methods and factory methods are explored. Whether you're new to Java or looking to deepen your understanding, this comprehensive guide provides valuable insights into the world of Java methods.
Declaring and Calling Methods in Java
Java methods serve as essential building blocks within the programming language, encapsulating a sequence of statements to perform a specific operation. This encapsulation not only promotes code reusability but also aids in structuring the program's logic for better clarity and maintenance.
A method's declaration in Java is a formal process that requires specifying the return type, the method's name, which should be self-explanatory and adhere to Java's naming conventions, and the parameters it accepts, if any. To invoke a method, the syntax involves the method's name followed by parentheses.
Arguments passed within these parentheses must align with the types and sequence outlined in the method's signature. If a method is designed to return a value, its type must be declared, and upon execution, it can be captured in a variable or utilized directly in expressions. Consider the following snippet demonstrating method declaration and invocation:
```java public class MyClass { public int add(int a, int b) { return a + b; }
public static void main(String[] args) {
MyClass obj = new MyClass();
int result = obj.add(5, 10);
System.out.println(result);
}
} ```
In this example, the add
method within the MyClass
class is constructed to take two integers and return their sum. The main
method then invokes add
, passing in values 5 and 10, and proceeds to print out the sum returned by the method.
Method Parameters and Return Types
In Java, method declarations and calls are building blocks of a program, enabling developers to encapsulate related instructions and divide complex tasks into simpler, more focused components. Methods accept input through parameters, which are specified within the method declaration and encased in parentheses.
Each parameter comprises a data type and an identifier, forming the method's signature. For instance, public void printMessage(String message)
is a method that receives a single String parameter named message
and performs an action without returning any value.
On the flip side, return types define the nature of data a method sends back to the caller. When a method concludes its execution, the return type determines what kind of result is produced, if any.
A method like public int calculate Sum(int a, int b)
not only takes two integer parameters but also returns their sum, an integer value. Meanwhile, methods such as public boolean isEven(int num)
evaluate the input parameter and return a boolean value indicating the evenness of the input number. Incorporating multiple parameters and handling complex return types, Java methods are versatile tools that follow the Single Responsibility Principleβeach method is tasked with a single, well-defined function, avoiding the pitfalls of overcomplication. By understanding parameters and return types, developers can create effective, reusable methods that contribute to a program's modularity and maintainability, akin to how a growing delivery company might diversify its transportation methods to handle different delivery scenarios efficiently.
Types of Methods: Instance and Static Methods
In the realm of Java programming, methods are fundamental units that encapsulate specific functionalities. They can be broadly categorized into instance methods and static methods.
Instance methods are tied to an object created from a class and can manipulate the object's instance variables. For instance, using the incrementCount
method in the following code snippet, we can increment the count
variable for a MyClass
object:
```java public class MyClass { private int count;
public void incrementCount() {
count++;
}
} ```
Static methods, in contrast, are associated with the class itself rather than any object instance.
They have access only to static variables and can invoke other static methods. An example of a static method is print Message
, which simply outputs a message to the console, as shown below:
```java public class MyClass { public static void printMessage() { System.out.println("Hello, World!
"); } } ```
To invoke these methods, an instance method requires the creation of an object, such as MyClass obj = new MyClass();
, followed by obj.incrementCount();
. Meanwhile, a static method can be called directly using the class name, like MyClass.printMessage();
. By distinguishing between these two types of methods, Java developers can adhere to the Single Responsibility Principle, ensuring methods have a focused role, thus maintaining simplicity and manageability in their code. Moreover, proper documentation through comments can serve as a quick reference, helping developers understand the structure and purpose of the code, which is particularly useful when dealing with complex codebases.
Method Overloading and Method Overriding
Java, a mainstay in the realm of object-oriented programming (OOP), empowers developers with method overloadingβa robust feature allowing multiple methods to share the same name while differentiating by parameter lists. Imagine a Calculator
class equipped with various 'add' methods, each tailored to operate with distinct types and quantities of inputs. The compiler astutely selects the correct method when invoked, ensuring seamless execution.
Here's a glimpse into this concept:
```java public class Calculator { public int add(int a, int b) { return a + b; }
public double add(double a, double b) {
return a + b;
}
public String add(String a, String b) {
return a + b;
}
public static void main(String[] args) {
Calculator calc = new Calculator();
int sum1 = calc.add(5, 10);
double sum2 = calc.add(3.14, 2.71);
String sum3 = calc.add("Hello", " World! ");
System.out.println(sum1);
System.out.println(sum2);
System.out.println(sum3);
}
} ```
Conversely, method overriding is a key pillar of polymorphism in Java. It allows a subclass to tailor the functionality of an inherited method.
By maintaining the same signature, a subclass method can provide a specific implementation that overrides its superclass counterpart. Employing the @Override
annotation clarifies this intention. For instance, in the Animal
and Dog
classes below, the Dog
class overrides the makeSound
method, offering a specialized behavior:
```java public class Animal { public void makeSound() { System.out.println("Animal is making a sound.
"); } }
public class Dog extends Animal { @Override public void makeSound() { System.out.println("Dog is barking. "); }
public static void main(String[] args) {
Dog dog = new Dog();
dog.makeSound();
}
} ```
These practices are not mere theoretical concepts but are widely applied in various programming domains, including data science and AI, where efficient and maintainable code is paramount. Coupled with the knowledge that Java interlocks with languages like JavaScript, SQL, Python, and HTML/CSS, understanding these OOP tenets is invaluable for a holistic development approach.
Best Practices for Using Methods in Java
When crafting methods in Java, adhering to best practices not only enhances the clarity and maintainability of your code but also aligns with the structured approaches of software development methodologies. Embrace meaningful, descriptive names for methods that convey their functionality, avoiding cryptic acronyms that obscure their intent.
For instance, rather than 'Ctr', opt for 'Customer', which is unequivocally understood. Methods should encapsulate a cohesive set of instructions, adhering to the Single Responsibility Principle (SRP).
This principle dictates that a method should have one well-defined task, avoiding the pitfalls of complexity by breaking down larger tasks into digestible, manageable units. Furthermore, limit the use of global variables, favoring parameters to pass necessary values, which fosters encapsulation and minimizes dependencies.
Robust error handling is crucial; employ exception handling within your methods to gracefully manage errors and ensure the resilience of your applications. To aid in this endeavor, documentation is key. Comments should not only explain the logic and structure of the code but also serve as a guide for other developers, detailing the purpose, parameters, and any side effects of your methods. In summary, by choosing clear names, focusing on single responsibilities, limiting global dependencies, handling exceptions effectively, and providing thorough documentation, you establish a strong foundation for writing clean, efficient, and maintainable Java methods.
Common Errors and Troubleshooting in Method Calls
While using Java, a language celebrated for its robustness and cross-platform capabilities, developers often face method-related errors. To troubleshoot such issues effectively, consider the following common pitfalls:
1.
Verify the method signature: A mismatch in the method name or parameters can lead to errors. Scrutinize the method call for correct spelling and sequence of arguments, aligning them with the method's declaration.
- Data type alignment: Arguments passed must have data types that correspond to those declared in the method.
Mismatched data types can cause the program to falter. 3.
Parentheses balance: Incorrect use of parentheses when invoking a method can lead to syntactical errors. Ensure each opening parenthesis is paired with its closing counterpart.
- Null pointer exceptions: A common issue in Java, these exceptions occur when calling methods on objects that have not been initialized. Check for null references before invoking methods to avoid runtime errors. 5. Method access level: The visibility of a method dictates where it can be called from. Confirm that methods are not inappropriately shielded by private or protected access modifiers when attempting to call them outside of their permissible scope. Incorporating these checks into your Java development routine can significantly reduce method call errors and enhance your program's reliability.
Advanced Topics: Abstract Methods and Factory Methods
Java's extensive features like abstract and factory methods are pivotal in enhancing programming skills and understanding advanced concepts. Abstract methods, declared without a body in an abstract class or interface, require subclasses to provide specific implementations.
This is exemplified by the abstract calculate Area
method in the Shape
class, which is overridden in the Circle
subclass for a concrete implementation. Abstraction, a core principle in software development, emphasizes focusing on essential details while omitting non-essential ones, allowing developers to manage complexity effectively.
Factory methods, part of the design patterns in Java, enable object creation without calling constructors directly. By using a static method within the class, such as create Car
in the Car
class, objects can be instantiated with additional flexibility and customization.
Understanding these patterns is crucial, as they reflect the evolution of software engineering practices over time, from the early days of manual coding to the advanced use of Computer-Aided Software Engineering (CASE) tools. These tools have transformed software creation, guiding developers through design, coding, testing, and maintenance, while adapting to various programming languages. By grasping the concepts of abstraction and factory methods, one can appreciate the systematic approaches embodied in software development methodologies. These methodologies provide structured processes and define roles, fostering an environment for efficient and high-quality software production. Embracing these advanced Java features aligns with the historical evolution of software engineering, where simplifying the complex and enhancing code readability and writability have always been paramount.
Conclusion
In conclusion, Java methods are vital building blocks in the programming language that encapsulate specific operations. By understanding method parameters, return types, and best practices, developers can create reusable and maintainable code.
Java methods can be categorized into instance methods, associated with objects, and static methods, associated with the class itself. Distinguishing between these types promotes simplicity and follows the Single Responsibility Principle.
Method overloading allows multiple methods to share the same name but differentiate by parameter lists, while method overriding enables subclasses to provide specific implementations. These concepts support efficient and adaptable code.
Adhering to best practices, such as using meaningful names, following the Single Responsibility Principle, handling exceptions effectively, and providing documentation, enhances code clarity and reliability. Common errors in method calls, such as mismatched signatures or data types, can be avoided by careful verification.
Understanding and managing method access levels also prevents unexpected issues. Advanced topics like abstract methods and factory methods further enhance code structure and flexibility. Abstract methods require specific implementations in subclasses, while factory methods facilitate object creation without directly calling constructors. By mastering Java methods and applying best practices, developers can create clean, efficient, and maintainable code. Java methods play a crucial role in building robust software solutions that align with established development methodologies.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.