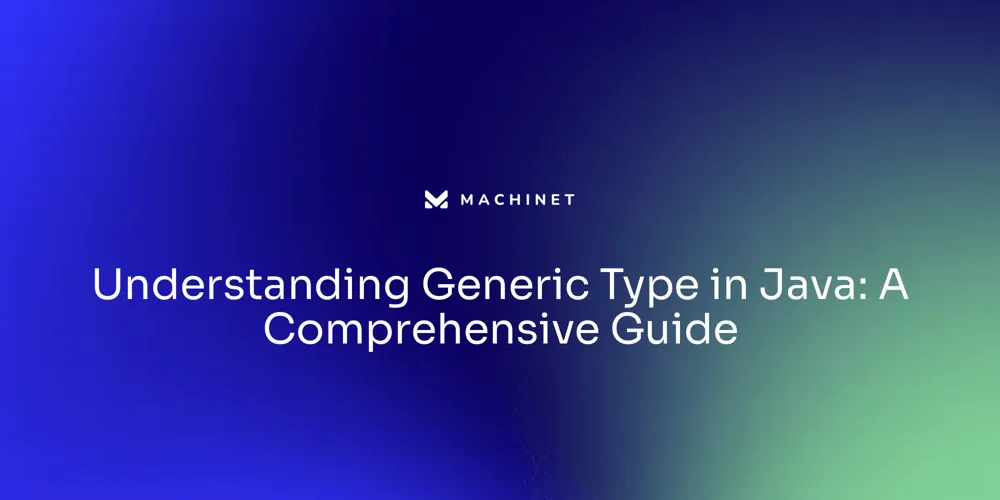
Table of Contents
- Types of Generics in Java
- Advantages of Using Generics
- Type Parameters and Naming Conventions
- Creating Generic Classes and Methods
- Using Generics with Collection Classes
- Type Erasure and Its Implications
- Common Use Cases for Generics
- Best Practices for Using Generics
Introduction
Generics in Java are a powerful feature that enhances code flexibility and type safety. With generics, developers can create classes, methods, and interfaces that can handle different data types, promoting code reusability and reducing redundancy.
This article explores the various types of generics in Java, their advantages, type parameters and naming conventions, creating generic classes and methods, using generics with collection classes, type erasure and its implications, common use cases for generics, and best practices for using generics. By understanding and utilizing generics effectively, developers can write more maintainable, efficient, and robust applications.
Types of Generics in Java
Generics in Java enhance the language's ability to handle different data types, allowing developers to write classes, methods, and interfaces that are type-safe and flexible. With generics, you can create a single method that can process a collection of objects, regardless of their data type, ensuring that the code is not only reusable but also free of common pitfalls like type casting errors.
For example, consider a method that sorts a list of elements. Without generics, you would need to write separate methods to sort lists of integers, strings, etc.
However, with generics, you can write a single, generic method to sort a list of any type, adhering to the Single Responsibility Principle, as it focuses solely on sorting, and the Open-Closed Principle, since it can be extended to handle new types without modifying its existing code. The use of generics also aligns with the philosophy of clear code naming conventions.
Instead of cryptic names that obfuscate the purpose of a class or method, generics allow for descriptive naming that makes the developer's intent clear. For instance, a generic 'List
Moreover, generics play a crucial role in data validation. They ensure that the data being processed conforms to a defined format, which is vital in transactions like user portfolio management. This provides a robust framework for maintaining the integrity of the system and preventing runtime errors. Reflecting on the real-world scenario of a delivery company that expanded from bicycles to multiple modes of transportation, just as the company had to adapt to different vehicles for efficiency, software development requires adaptable code. Generics enable developers to write adaptable code that can handle various data types, akin to how the delivery company uses different vehicles to meet the delivery demands efficiently. In summary, generics are a cornerstone of Java programming, promoting code reusability, type safety, and maintainability, in line with modern software development principles and real-time service demands.
Advantages of Using Generics
Java's generics mechanism is a powerful tool for developers, enhancing both the flexibility and safety of code. By leveraging generics, you can create classes and methods that operate on a variety of data types, enabling code reuse and reducing redundancy. This feature avoids the need for multiple iterations of similar code for different data types, streamlining the development process.
One of the fundamental principles underpinning this is the Single Responsibility Principle (SRP) from the SOLID design principles, which encourages developers to design classes that have one purpose and thus one reason to change. Generics align well with this principle, allowing classes to handle a single responsibility across multiple data types without modification. Moreover, by using clear and descriptive naming conventions, as recommended by industry standards, generics contribute to the readability and maintainability of code.
Generics also play a critical role in compile-time type safety, catching errors early in the development cycle. This early detection is crucial, as it can prevent runtime errors that are often more difficult to debug and can lead to more serious issues, such as security vulnerabilities or application crashes. As the software development lifecycle (SDLC) emphasizes planning, testing, and maintenance, the integration of generics supports these phases by ensuring that code is robust and reliable from the outset.
Type Parameters and Naming Conventions
Generics in Java serve as a cornerstone for creating robust and type-safe code. They allow developers to define classes, methods, and interfaces with placeholders for types, known as type parameters.
These parameters, typically denoted by single uppercase letters such as 'T' for Type or 'E' for Element, follow a naming convention that serves multiple purposes. Not only do they uphold code readability and maintainability, but they also embody the Single Responsibility Principle (SRP) by encapsulating a specific and singular intent.
This practice of meaningful naming is echoed in the sentiment that names should be both descriptive and mnemonic, providing a cognitive anchor to the system's functionality while remaining concise. In the context of real-time services and edge data platforms, where milliseconds matter and clarity is paramount, these conventions in naming and structure ensure that software components are immediately understandable and efficiently navigable. As in financial markets where traders rely on clear, immediate data, software developers depend on well-named generics to swiftly navigate and manipulate code, enhancing the development process and the quality of the end product.
Creating Generic Classes and Methods
In Java, the power of generics enhances code flexibility and reusability, allowing a single class or method to operate on various types of data. To define a generic class, one employs angle brackets (<>) with a type parameter following the class name.
This parameter serves as a placeholder that can be replaced with any class type when an object of the generic class is instantiated, ensuring type safety. For example, instead of creating numerous classes to handle different data types, a single generic class can manage them all.
This mirrors the principle of the Single Responsibility Principle (SRP) from SOLID design principles, where a class encapsulates a single, well-defined task, providing clarity and manageability. Similarly, generic methods are defined with a type parameter preceding the return type, encapsulating a set of related instructions into a cohesive unit.
This aligns with SRP by ensuring that methods have a clear, singular focus, preventing them from becoming too complex. In the realm of software development, where the choice between custom and ready-made solutions is crucial, generics offer a middle ground.
They allow developers to create flexible code akin to custom software that can be adapted for various purposes, similar to the versatility of ready-made software. Adhering to clear naming conventions is paramount when working with generics. Instead of obscure acronyms, descriptive names make the codebase accessible and understandable, avoiding confusion and enhancing maintainability. A recent report highlights the significance of such practices, noting that 77% of developers are embracing tools like Chat GPT, indicating a trend towards leveraging AI to streamline coding tasks. In this evolving landscape, the use of generics in Java is a testament to the industry's direction towards more efficient and intelligent coding practices.
Using Generics with Collection Classes
In Java, the use of generics enhances the robustness of code interactions with collection classes. By defining a type parameter for a collection, developers can ensure that only objects of a predetermined type are permitted within it.
This type safety is essential, particularly when dealing with critical data transactions, such as user portfolios in financial applications. Generics aid in enforcing data conformity to expected formats, thereby safeguarding the system's integrity.
For instance, when handling Data Transfer Objects (DTOs) for operations like creating new portfolios or processing stock transactions, generics provide a clear and strict framework for data validation. Moreover, adhering to the Single Responsibility Principle, generics allow methods within these collections to remain focused on their core purpose without becoming convoluted.
This is in line with best practices in software design, where methods serve as concise building blocks, each with a clearly defined role. Additionally, the clarity brought by generics aligns with the industry's preference for simple and descriptive code names, as it prevents the ambiguity that often arises from the use of acronyms or complex naming conventions. This clarity is not only beneficial for current development but also serves as self-documenting code, assisting future developers in understanding the code's structure and logic. As Eleftheria, an experienced business analyst, highlights, having a solid grasp of technical skills, including generics in Java, is invaluable for creating efficient and maintainable software.
Type Erasure and Its Implications
Java's implementation of generics involves a process known as type erasure, where the compiler essentially removes all generic type information during compilation. This is done to maintain compatibility with older Java code not designed with generics.
The consequence, however, is that developers cannot use the type parameter at runtime, which can pose certain challenges. For instance, in scenarios where no value is available for an object reference, as is often the case in software applications, the absence of a value must be handled appropriately.
The concept of type erasure in Java aligns with the broader discussion of practical type systems in software development, which emphasizes the importance of data structures and the relationships between them over code syntax. A practical type system guides developers to write code that is not only reliable and maintainable but also ensures data integrity, especially in data transactions. In the context of Java generics, understanding type erasure is a step towards writing better code, as it pushes developers to pay closer attention to data structures and how they are manipulated at runtime.
Common Use Cases for Generics
In Java programming, generics are a fundamental concept that enable developers to write flexible, reusable, and type-safe code. By defining generic data structures, one can create containers that work uniformly with any object type.
For instance, a generic List interface can hold any type of object, from Integers to Strings, without the need for casting, which can lead to ClassCastException if not handled properly. Generics also play a crucial role in the design of APIs, allowing for methods that can operate on a wide range of types while ensuring compile-time type safety.
This prevents runtime errors and enhances code readability, as it becomes clear what type of objects are expected. Applying the principles of SOLID design, especially the Single Responsibility Principle (SRP), generics help keep classes and methods focused and manageable.
When a class or method is generic, it can perform its function regardless of the data type, adhering to the Open-Closed Principle (OCP) by being open for extension but closed for modification. This aspect of generics is particularly beneficial for algorithm implementation, where the logic can be applied to any data type, increasing the versatility of your code. Moreover, a recent survey revealed that 77% of developers are utilizing AI tools like ChatGPT, and 46% are using GitHub Copilot, indicating a trend towards tools that can support and enhance coding practices, including the use of generics. As the technology landscape evolves, embracing generics in Java will undoubtedly contribute to writing more maintainable, efficient, and robust applications.
Best Practices for Using Generics
Generics in Java empower developers to write flexible and type-safe code, much like a delivery company choosing the right mode of transportation for their package. Just as a bicycle, motorcycle, or car is selected based on the delivery distance, generics allow for the selection of specific data types at runtime, ensuring that code is robust and adaptable.
Adhering to best practices when utilizing generics, such as using descriptive type parameter names like 'T' for a generic data type or 'E' for an element in a collection, enhances code readability and maintenance. By avoiding raw types, developers prevent the potential for unchecked warnings at compile-time, and instead, ensure that the correct data type is used consistently throughout the codebase.
Moreover, implementing bounded type parameters guides the Java compiler with specific type information, which can be as vital as having clear requirements and effective communication in software development. This approach is aligned with the Single Responsibility Principle, ensuring that each generic type or method is focused and purposeful. The State of Developer Ecosystem report underscores the trend towards high-quality, maintainable code, with a majority of developers adopting modern tools and practices. Embrace generics with these practices in mind, and your code will be as efficient and reliable as the most well-organized delivery service.
Conclusion
In conclusion, generics in Java enhance code flexibility and type safety. They promote code reusability by allowing developers to handle different data types with a single class or method. Generics align with modern software development principles like the Single Responsibility Principle and the Open-Closed Principle.
Advantages of generics include increased code flexibility, improved readability, and compile-time type safety. They streamline development by avoiding repetitive code and catching errors early. Type parameters and naming conventions ensure readability and maintainability.
Clear naming conventions make software components immediately understandable. Creating generic classes and methods enables code adaptability and reuse. Instead of writing separate components for different data types, generics handle various types in a single component.
Using generics with collection classes enhances type safety, enforces data conformity, and maintains system integrity. Generics also promote concise building blocks within collection methods. Understanding type erasure in Java generics is crucial as it affects how generic type information is handled during compilation.
Common use cases for generics include creating flexible containers and designing APIs that operate on various types while ensuring compile-time type safety. To use generics effectively, follow best practices like using descriptive type parameter names, avoiding raw types, implementing bounded type parameters, and embracing modern tools and practices in software development. By understanding and utilizing generics effectively, developers can write more maintainable, efficient, and robust applications that align with industry standards.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.