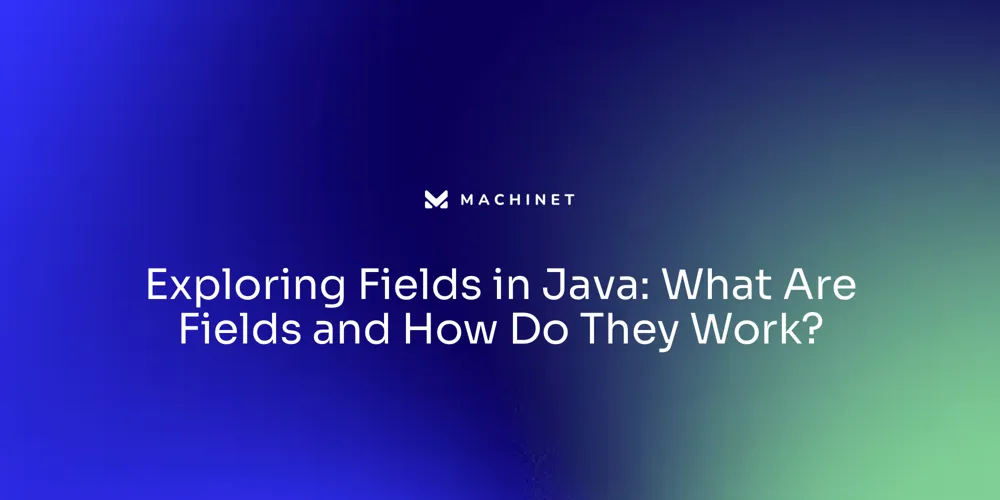
Table of Contents
- What Are Fields in Java?
- Types of Fields in Java
- Declaring Fields in Java
- Access Modifiers for Fields
- Field Initialization and Default Values
- Field Hiding and Overriding
- Using Fields in Java Programs
- Common Issues with Fields in Java
- Best Practices for Using Fields in Java
Introduction
Java, the popular object-oriented programming language, offers a rich structure that promotes clean coding practices and organized development. One important concept in Java is fields, which are like variables tailored specifically for classes or objects. Fields serve as containers for values and define the state and behavior of instances in Java.
They encapsulate data, allowing easy access and modification within the program, contributing to its dynamic nature. In this article, we will explore the different types of fields in Java, how to declare and initialize them, the use of access modifiers, common issues to be aware of, and best practices for using fields effectively. By understanding and utilizing fields in Java, developers can write clean and maintainable code that fosters efficient and organized software development.
What Are Fields in Java?
Java, an object-oriented programming language created by James Gosling in 1990, comes with a rich structure that facilitates clean coding practices and organized development. To articulate the architecture of Java, let's consider fields, which are akin to variables tailored for classes or objects. Fields serve as specific containers that hold values and define the state and behavior of instances in Java.
Fields encapsulate data, ensuring that each bit of information can effortlessly be accessed and modified within the program, thus contributing to its dynamic nature. For instance, in the context of a Person class, fields such as name and age store data pertinent to individual objects or 'instances' of that class, while also outlining potential actions (methods) such as walk() and talk().
James Gosling's design philosophy, rooted in readability and writability, is reflected in the seamless way fields allow data storage and retrieval - a foundation for the robust and maintainable code that typifies Java. This paradigm is underscored by the use of the Java Development Kit (JDK), a comprehensive suite that provides the indispensable tools for crafting Java-based applications.
The Single Responsibility Principle (SRP) aligns with the idea that a method, much like a field, should have a focused role. This principle contributes to an application’s maintainability and prevents complexities that could arise from multi-functional methods. This attribute is critical in object-oriented programming (OOP), where the creation of objects from classes, and the capable handling of their associated data and methods, is of paramount importance.
Adherence to such principles is what produces 'clean Java code,' lauded for its ease of comprehension and effectiveness, as expressed by experts: 'Clean code... is a set of best practices and a commitment to excellence in software craftsmanship.' Fields, in this respect, embody the union of data and functionality, each class defining the data it houses while the JDK offers the tools to manipulate it effectively.
Types of Fields in Java
Java, as a programming language, is underpinned by both art and science in the way it handles the structure and elements of code. Fields in Java can be categorized into different types, each serving a unique role in organizing data and behavior in an application. Instance fields define characteristics unique to an object and ensures every object has its own values, encapsulating the object's properties.
Static fields, however, are consistent across all instances of a class — a singular data point shared among various objects, ideal for constants or utility values. Local fields have a transient life; they come into play within the boundaries of a method, providing temporary storage for intermediate computations or values. Lastly, parameter fields act as conduits for data, channeling inputs into methods or constructors to influence the behavior of an object or a process.
By strategically applying these field types, developers can design Java code that is not only effective but also adheres to the principles of 'clean code' - efficient, readable, and maintainable over time. Clear and consistent utilization of fields aligns with the Single Responsibility Principle, ensuring each component of the codebase is tasked with a clear and singular purpose - a premise echoed in the belief that writing clean Java code is an indispensable facet of quality software development.
Declaring Fields in Java
Defining fields in Java is a fundamental aspect of structuring your code. This starts with specifying the data type of the variable, such as int
, boolean
, or String
, to clearly communicate what sort of information will be stored. For instance, an integer variable count
would be declared as private int count;
.
Here, private
serves as the access modifier, which is essential in encapsulating the visibility of your fields. In Java, the access modifiers such as public
, private
, protected
, or default (no explicit modifier) play a critical role in object-oriented programming by controlling access levels to various parts of your code.
While the concept of algebraic data types from type theory may seem distant from day-to-day Java programming, their underlying philosophy is ever-present. It's the idea of assigning a specific, logical type to every piece of data we deal with, ensuring that the behavior of our code aligns with the principles of good software design. By adhering to these ideas and writing clean, maintainable code, you anchor your work in best practices that champion performance and simplicity.
When dealing with fields in Java, it’s crucial to remember the significance of clear, well-structured code as emphasized by clean code advocates. Each declaration is a commitment to precise, understandable, and functional software. According to recent statistics, technologies such as JavaScript, SQL, and Python are frequently utilized alongside Java by many developers.
This highlights the importance of robust fundamentals like field declaration, as they are the building blocks in a diverse technological landscape where integrating multiple languages and frameworks is commonplace.
Access Modifiers for Fields
In the realm of Java, an object-oriented programming language with its syntax influenced by C and C++, understanding the rules of accessibility is pivotal for maintaining clean, readable code. This is where access modifiers come into play. There are four tiered levels of access control for classes, methods, and fields:
-
Public: This modifier allows the member to be accessed from any context within the Java environment. Utilizing public means that fellow developers can interact with the field from any class.
-
Private: A private modifier ensures that the member is only accessible within its own class. It represents a strong level of encapsulation, safeguarding against unintended interactions from outside the declaring class.
-
Protected: This provides a middle ground, allowing access within its own class, to subclasses, and to classes within the same package. Its use reflects a balance between accessibility and encapsulation.
-
Package-private (default): Without an explicit modifier, Java defaults to package-private access, permitting visibility only within its own package, helping preserve a streamlined project structure.
Encapsulation, a core concept of object-oriented programming in Java that conceals the internal state of objects, is reinforced through these access levels by controlling visibility in alignment with the Single Responsibility Principle. Each field and method are encapsulated, embodying a single, defined purpose. Remember, as advocated by experts in software development, 'clean code' isn't just an ideal to strive for; it's integral to the sustainability of projects.
By meticulously choosing the right access modifier, you uphold the principles of clean code — crafting not only functional but also sustainable Java applications. Moreover, as you learn and grow in Java development, these principles help you manage complex codebases effectively, easing your understanding and maintenance of robust software systems.
Field Initialization and Default Values
In Java, one of the pillars of software construction is initializing variables with appropriate values. When a field is declared within this robust language, often coveted for its performance and flexibility, the default value is automatically set based on the type of the field if no initialization is explicitly provided. For numeric types such as 'int' and 'double', this value is 0; 'boolean' fields begin as 'false'; characters start as the null character '\u0000', and object references are initiated to 'null'.
To cater to the unique needs of your application or to comply with the principle of clean code, it's possible—and sometimes necessary—to override these defaults. You can explicitly set values at the point of declaration or within a constructor, laying down a clear, reliable foundation for the field's lifecycle. This initial assignment is not just about avoiding errors; it’s about embedding the software with your intentions, making it readable and understandable—qualities that frameworks can sometimes obscure compared to the transparency of native Java.
This decision will depend heavily on the context of your project and the balance you wish to achieve between the control native Java grants and the accelerated development that frameworks offer. Understanding Java’s default behaviors and the depth of control you have over them is the first step towards crafting code not just for its function, but for its role in the larger tapestry of your application’s architecture and maintainability.
Field Hiding and Overriding
In the dynamic world of Java programming, a core concept of Object-Oriented Programming (OOP) comes into play when dealing with fields within classes and subclasses. In the Java language, a field in a subclass that bears the same name as one in its superclass enters a relationship of either 'hiding' or 'overriding'. Hiding happens when the subclass's field simply occupies the same name space, obscuring the superclass's field.
This hidden field can only be accessed through explicit reference, typically by employing the 'super' keyword.
Overriding, by contrast, is a more deliberate act where the subclass not only shares the same name for the field but also assigns it a new value, effectively replacing the content of the superclass's field when accessed through instances of the subclass. This nuanced approach allows Java developers to finely tune the attributes and behaviors of objects, embracing the principles of encapsulation and abstraction that are pivotal in OOP.
OOP, as an architectural framework, is vested deeply in the way Java operates, with its goal of mirroring real-world entities and their interactions at a conceptual level. A Java class, embodying this principle, serves as a template that encapsulates attributes (fields) and actions (methods) to fabricate objects. For instance, imagine a 'Person' class, possessing fields for 'name' and 'age', as well as methods like 'walk()' and 'talk()'.
In this way, Java’s syntax and semantics intertwine to form the backbone of creating robust and highly adaptable software systems.
Using Fields in Java Programs
Handling fields in Java, one can employ dot notation to access or alter a field's value. To put it simply, fields store data within your Java classes, much like how a parking lot system requires specific features, such as parking lot ID, floors, and slots—all fundamental elements for the system's infrastructure. Similarly, fields in Java contribute to the structure and functionality of a program.
Let's dissect field usage with an example:
```java public class ParkingLot { private int parkingLotID; private int numFloors; private int slotsPerFloor; // Initialization of a ParkingLot object with required fields public static void main(String[] args) { parking lot = new parking(); lot.parking lot = 101; lot.num floors = 5; lot.slotsPerFloor = 20; System.out.println("Parking Lot ID: " + lot.parkingLotID); System.out.println("Number of Floors: " + lot.numFloors); System.out.println("Number of Slots per Floor: " + lot.slotsPerFloor); } }
```
In this structure, 'parkingLotID', 'numFloors', and 'slotsPerFloor' are akin to the slots in our envisioned parking lot—they form the basic requirements and allow for data storage and manipulation. It is imperative that as we delve into programming, we adhere to the axiom of writing clean code, celebrated for its ease to read and maintain. As underscored by industry experts, clean, maintainable code is not optional but essential, serving as the 'lifeblood of our creations'.
In the realm of Java, where fields and classes interlock to build the architecture of a program, maintaining a clear and concise codebase is crucial, allowing for robust and secure software solutions. By applying the Single Responsibility Principle, as with fields, each segment of code in Java should perform a singular, focused task, reducing complexity and enhancing clarity.
Common Issues with Fields in Java
While tackling the intricacies of Java fields, developers should be mindful of pitfalls that can hinder code quality. One such pitfall is shadowing, where a field declared within a nested scope bears the same identifier as a field in an outer scope. This may lead to unintended behavior, hence the importance of distinct naming conventions to deter shadowing.
Speaking of conventions, adherence to consistent naming protocols like camelCase or UPPER_CASE_WITH_UNDERSCORES is fundamental. It bestows order and readability, qualities that are essential in the poetic science of code craftsmanship.
Moreover, accidental field modification is a symptom akin to the dreaded 'code smells' that seasoned developers warn against—it signals the code might be harder to maintain or evolve. To forestall such accidental modifications, encapsulation is crucial. Limiting accessibility through proper use of access modifiers guards against unwelcome changes, thereby solidifying the integrity of your code.
Ultimately, the quest for clean Java code extends beyond the immediate task—it underpins a commitment to the evolution and longevity of our software endeavors. As put by veteran developers, methods should encapsulate distinct functions, embracing the Single Responsibility Principle to avoid overwhelming complexity. By internalizing these principles, you contribute to the creation of code that's not just operational, but lucid, maintainable, and poised for future enhancements.
This approach echoes the best practices that transform rudimentary code into a mature, stable product, as evidenced by experienced programmers who elevate legacy systems to reliable, self-sustaining applications.
Best Practices for Using Fields in Java
In Java development, crafting clean code is akin to an art form that underpins the long-term health of software projects. It's not merely about writing code that executes tasks but about doing so in a manner that fosters readability and maintainability. When handling fields within Java, here are several best practices to adhere to:
-
Encapsulation: To protect the integrity of the data within your program, like in a parking lot system where unique identifiers and various parameters for spots and vehicles are needed, encapsulate fields with private modifiers. Access and modify these values via getter and setter methods. This not only safeguards information but also simplifies future modifications and debugging.
-
Naming Conventions: Always opt for clear, descriptive names over cryptic acronyms. For instance, instead of 'Ctr', use 'Customer'. This approach, consistent with naming conventions like camel case for instance fields or all-uppercase with underscores for constants, enhances code readability and reduces misunderstandings.
-
Access Modifiers: Choose the most restrictive access level necessary for fields. Restricting access helps maintain control over data flow within the program and prevents unintended alterations. This echoes the philosophy that security should be integrated into the design process, rather than being an afterthought.
-
Initialization: Initialize fields preemptively to steer clear of null references and unexpected behaviors. Just as you would carefully lay the foundation for a parking lot system with specific requirements, ensure fields are appropriately set before bringing them to use.
-
Avoid Public Static Fields: Favor instance fields where possible and convey information via method parameters or return values. This encourages stronger encapsulation and reduces interdependencies within your code, mirroring the ideal that clean code should be simple and coherent.
Indeed, security by design is paramount; integrating it from the onset prevents vulnerabilities from deeply embedding themselves into the code. This promotes not just security but also efficiency, preventing the need for overhauling code later on. By embracing these principles, developers can achieve clean Java code—code that is seamless, effectively communicates intent, and stands as a testament to their software craftsmanship skills.
Conclusion
In conclusion, fields in Java are essential for clean and organized coding practices. They encapsulate data and define object state and behavior, contributing to the dynamic nature of Java programs. By understanding the different types of fields and their role in organizing data, developers can design efficient and maintainable code.
Proper declaration and initialization of fields, along with the use of appropriate access modifiers and adherence to naming conventions, ensure code readability and security. By leveraging concepts like field hiding and overriding, developers can fine-tune object attributes and behaviors in Object-Oriented Programming.
However, it is important to be mindful of common issues such as shadowing and accidental field modification, which can impact code quality and maintainability. By following best practices like encapsulation, proactive initialization, and avoiding public static fields, developers can create code that is clean, readable, and maintainable.
In summary, fields in Java are powerful tools that contribute to organized and efficient coding. By utilizing them effectively and adhering to best practices, developers can write clean and maintainable code that fosters robust software development.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.