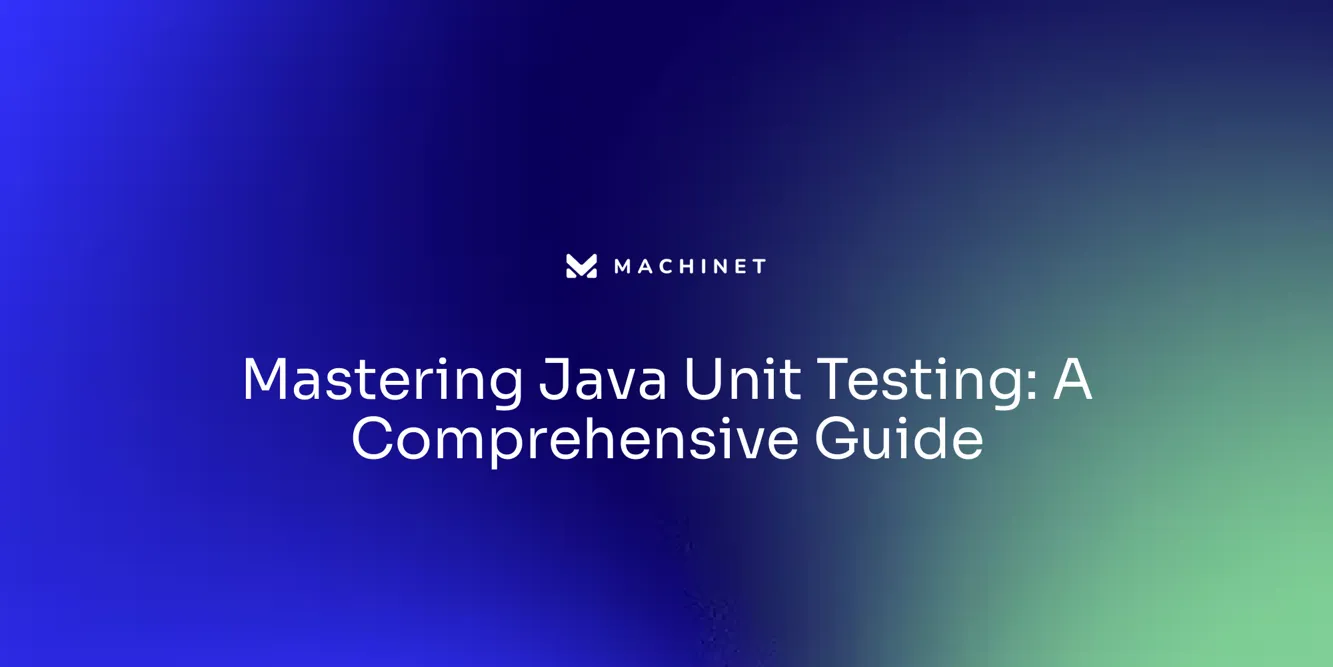
Table of Contents
- Why Unit Testing is Important
- Choosing the Right Testing Framework: An Overview of JUnit
- Setting Up JUnit in Your Java Project
- Writing Effective Unit Test Cases with JUnit
- Advanced JUnit Features: Annotations and Assertions
- Integrating Mockito with JUnit for Mocking Dependencies
- Best Practices for Java Unit Testing with JUnit
Introduction
Unit testing is a crucial practice in software development, especially in the realm of Java. It involves examining individual code components to verify their functionality in isolation. Unit tests play a vital role in catching bugs, confirming code behaviors, and improving overall code quality.
This article will explore the importance of unit testing, the benefits of using JUnit as a testing framework, setting up JUnit in a Java project, writing effective unit test cases, utilizing advanced JUnit features such as annotations and assertions, and integrating Mockito for mocking dependencies. We will also discuss best practices for Java unit testing and how platforms like Machinet.net are revolutionizing the unit testing process. Get ready to enhance your testing skills and deliver robust software products.
Why Unit Testing is Important
Unit testing is a foundational practice in software development, especially within the realm of Java. This testing method involves closely examining individual components of code, such as classes and methods, to verify their functionality in isolation. Unit tests play a crucial role in catching bugs, confirming code behaviors, and enhancing the overall quality of the code.
Each unit test case has a specific goal, designed to evaluate certain aspects of the software's functionality or performance. These tests greatly aid in continuous integration and deployment pipelines, providing a robust base for automated testing, and enabling organizations to uphold the quality of code throughout the development process. Unit testing, including tests for pipelines and lambdas, is an integral part of the integration process.
It helps ensure that new code integrates smoothly with the existing codebase, leading to the development of a stable and deployable application. Furthermore, the practice of unit testing fosters the creation of cleaner, more maintainable code, ultimately improving the quality of the software. Anyone with access to the source code, such as developers, testers, and QA engineers, can perform unit testing.
This process is particularly important for regulatory compliance in some industries, where extensive testing is required to guarantee software accuracy and safety. Libraries like ArchUnit can assist in maintaining architectural control within the codebase. ArchUnit provides a domain-specific language (DSL) that enables the expression of architectural rules in a clear and concise manner, ensuring compliance with design principles and standards.
Machinet.net is a platform dedicated to unit testing in software development, with a particular focus on Java. It provides tips, techniques, best practices, and information on frequently used Java frameworks and tools for unit testing. Machinet.net promotes the benefits of unit testing, including automated test generation via Mockito, to enhance code quality and productivity.
It also covers the use of JUnit annotations and assertions in Java unit testing. In summary, unit testing goes beyond just verifying code correctness. It is a crucial component of a comprehensive testing strategy, working in tandem with other testing methods to produce robust, high-quality software products.
Choosing the Right Testing Framework: An Overview of JUnit
JUnit, a widely recognized testing framework, serves as the bedrock for unit testing in Java applications. It offers a streamlined approach to devising, running, and validating test cases.
Its user-friendly yet powerful features have made it a staple among Java developers. It is noteworthy that a recent survey indicated that 33% of participants use JUnit, contributing to unit tests being a major component of 63% of software projects.
This framework forms a part of an extensive testing infrastructure, encompassing tools, resources, and processes to enable efficient software testing. Key features of JUnit include a zero-configuration testing experience, saving developers from lengthy environment setup.
It presents easy installation and straightforward mock functions. The framework is compatible with various platforms such as Node, React, Angular, Vue, and more.
Its snapshot feature simplifies tracking of large objects, proving beneficial for developers handling substantial JavaScript projects. Testing goes beyond merely identifying bugs; it involves performance evaluation and data integrity verification.
JUnit provides developers with the tools to assess the performance of the new system under real-world conditions and optimize its behavior prior to broad deployment. It also facilitates data integrity verification by comparing the results of the new system with the existing one, ensuring accuracy in data migration and transformation processes.
Additionally, JUnit's inbuilt reporter delivers a detailed report from the crafted tests. These tests serve as a concrete representation of the developer's work, spotlighting both their coverage and the precision of the developer's understanding of the feature or product. This viewpoint enhances the understanding of this developmental phase's importance, solidifying JUnit's position as a vital tool in every Java developer's toolkit. Moreover, platforms like Machinet.net are revolutionizing the unit testing process by automating the creation of unit tests using Mockito. They aim to boost code quality and productivity through automated unit test generation, offering features like mockito mocks and best practices for unit testing. By catching and fixing issues early in the development cycle, they contribute to delivering robust and reliable software. With comprehensive strategies using JUnit annotations and assertions, platforms like Machine.net are shaping the future of AI and software development.
Setting Up JUnit in Your Java Project
To begin your unit testing journey with JUnit, a well-prepared environment is paramount. Integrating JUnit into your Java project is the first step, which involves adding JUnit as a dependency and configuring your preferred build tool.
This sets the foundation for a robust testing environment, crucial for validating the functionality of specific code units. Setting up an efficient Java development environment is crucial and involves installing the Java Development Kit (JDK).
This package includes the Java Runtime Environment (JRE) and development tools such as the Java compiler and debugger. JUnit tests, a type of Java unit test, utilize the JUnit framework to verify the correctness of specific code units, usually methods or classes.
The accuracy of JUnit tests is vital in preserving the application's overall integrity and reliability. Platforms like Machinet.net can automate the process of writing unit tests using Mockito, a mocking framework for Java.
It provides features like automated unit test generation and understanding of JUnit annotations and assertions. This can significantly improve code quality, productivity, and correctness by enabling the early detection and resolution of defects. When writing your test cases and asserting expected results, always aim for clarity and maintainability. This will make your code not only functional but also easy to read, understand, and modify, benefiting all project stakeholders. With Machinet.net's best practices and tips for Java unit testing, including how to structure tests, isolate dependencies, and generate effective assertions, you'll be well-equipped to create a fully operational JUnit setup in your Java project.
Writing Effective Unit Test Cases with JUnit
Writing effective unit tests is a crucial skill for Java developers. This involves understanding and implementing best practices to create comprehensive and meaningful tests using JUnit.
Test structure is vital, and so is generating test data that provides the necessary inputs for the tests. Test coverage ensures all code has been tested, enhancing the reliability of the software application.
Test-Driven Development (TDD) principles can further improve the effectiveness of unit tests. Regular updates, as seen from the Gradle Build Tool team's experience with TeamCity for its CI/CD process, ensures the use of the most feature-rich and current version of the product.
They use Git and GitHub as their version control system, further streamlining their development process. The quality and reliability of applications are highly valued in software development.
Test infrastructure, including tools, resources, and processes, plays a crucial role in achieving these goals. Unit tests, though quick and easy to write, run, and debug, require time to set up, especially if they're not automated.
Automation tools can help by recording, saving, and replaying tests without manual effort. Keeping test cases simple and focused on a single expected result is key.
Understanding the purpose, objectives, and components of a test case is crucial for the robustness and effectiveness of the testing process. Machine.net is a platform that automates the process of writing unit tests for Java developers. It uses Mockito, a mocking framework, to help increase productivity and ensure code correctness. The platform provides features like automated unit test generation, Mockito mocks, and AI assistance for developers. It also offers resources like ebooks and blog articles on Java unit testing, code quality, and best practices. Ultimately, effective unit testing involves a blend of best practices, understanding the purpose and components of tests, and the judicious use of automation tools. These elements contribute to creating robust and reliable unit tests for Java code.
Advanced JUnit Features: Annotations and Assertions
JUnit, a renowned testing framework, harnesses a suite of features that enhances the effectiveness of your unit tests. It uses annotations to manage your tests' lifecycle, set preconditions, and organize test suites. Assertions, another integral feature, confirm expected results and juxtapose actual with anticipated values.
Each test case is centered around a goal, which delineates the functionality or performance it seeks to evaluate. Test cases also encompass a unique identifier for tracking, a succinct title, preconditions, input data, and steps to replicate. In the fast-paced world of software development, unit tests play a crucial role in ensuring smooth performance and integration of code components within the larger application.
They ward off bugs, simplify code refactoring, and serve as living documentation, among other advantages. Unit tests form the backbone of continuous integration and deployment workflows, establishing a benchmark for code quality and aiding in regulatory compliance. Proficient unit testing necessitates a comprehensive grasp of the unit's anticipated behavior, spanning various scenarios such as standard use cases, edge cases, and boundary conditions.
It proves beneficial to assign your test cases significant and descriptive names. As the software progresses, unit tests act as a reference point, facilitating maintenance and mitigating the chance of unforeseen impacts during alterations. Machinet.net, a software development platform, offers extensive unit testing strategies.
It equips Java developers with tools and frameworks to craft effective and efficient unit tests. Leveraging features like tags, annotations, and assertions, Machine.net empowers developers to identify and rectify defects early in the development cycle, thereby ensuring code quality and delivering superior software that caters to end-user requirements. A key tool offered by Machinet.net is Mockito, which automates the unit test writing process, aiding Java developers in enhancing productivity and guaranteeing code correctness.
The platform also provides resources like books, demos, and articles to help developers hone their unit testing skills. To summarize, judicious use of JUnit's features can amplify the expressiveness and maintainability of your unit tests, leading to sturdy, top-notch software products. With the added resources from platforms like Machine.net, developers can further refine their unit testing strategies and deliver quality software.
Integrating Mockito with JUnit for Mocking Dependencies
In the realm of complex Java projects, unit test creation often necessitates mocking external dependencies to hone in on the code under scrutiny. This is where Mockito, a potent mocking framework, comes into play.
It meshes well with JUnit, allowing for efficient dependency mocking. Mockito facilitates the creation of mock objects, defining their responses, and verifying their interactions.
This enables a more focused approach to testing Java code, lessening the dependency on real external elements. However, using Mockito is not without its challenges.
Understanding its syntax and concepts can be a steep learning curve, particularly for those new to the framework or unit testing. Moreover, overuse of Mockito can lead to over specification of the code under test, resulting in fragile tests susceptible to breakage when adjustments are made to the implementation.
A delicate balance must be struck between verifying behavior and concentrating on the outcome. Mockito broadens test coverage, allowing developers to mimic a variety of scenarios.
It ensures tests cover all relevant paths through the code by controlling dependency behavior, thereby overcoming any real-world constraints. However, Mockito can become intricate when testing complex areas of the application.
In such instances, architecture reassessment might be necessary. That being said, Mockito remains an invaluable tool for maintaining code integrity and enhancing software quality and reliability. To ease these challenges, platforms like Machine.net can be instrumental. It automates the process of writing unit tests using Mockito, striving to boost productivity and ensure code correctness. It simplifies mocking with Mockito and aids in understanding its role in Java unit testing. Furthermore, it provides an exploration of JUnit annotations and assertions. Machine.net also offers a wealth of best practices and tips for Java unit testing, including test structuring, dependency isolation, and effective assertion usage. Despite any complexities, Mockito, especially when used in conjunction with resources like Machinet.net, remains a potent tool in ensuring the overall quality and reliability of software systems.
Best Practices for Java Unit Testing with JUnit
To master unit testing in Java with JUnit, a structured approach that includes adhering to best practices is essential. This requires a thorough grasp of naming conventions, test organization, data management, and understanding test coverage.
A well-constructed test infrastructure, including a dedicated test environment, specific objectives for every test case, and effective test data management, can significantly enhance software testing quality. Unit tests are a fundamental component of automated testing in continuous integration and deployment pipelines, establishing a code quality benchmark and playing a crucial role in regulatory compliance.
Utilizing unit testing frameworks can expedite defect detection, providing swift feedback to developers, reducing bug-fixing costs, and improving overall software quality. Machinet.net is a platform that offers a comprehensive unit testing strategy for Java developers, including automating the process of writing unit tests with Mockito.
This automation helps developers enhance productivity and ensure code correctness. A systematic execution of tests can promptly identify issues, enabling quick resolution before they develop into more complex problems.
This not only boosts software quality but also enhances the efficiency of the testing process. Shadow testing, a technique where a new or modified system is deployed alongside the existing production system, can be used to assess the behavior of the new system in a real-world setting before official release.
This approach minimizes risks associated with system changes or updates, ensuring a smoother deployment process. Test smells are a sign that tests could be simplified or better organized. As suggested by Marco Tulio Valente in his book 'Modern Software Engineering', it's crucial to pause and consider if there's a simpler way to approach testing. By implementing these best practices, your unit tests will not only become more effective but also significantly contribute to the overall quality of your Java codebase. Machinet. Net also offers resources for learning about AI software and exciting career opportunities.
Conclusion
In conclusion, unit testing is a crucial practice in Java software development. It catches bugs, confirms code behaviors, and improves overall code quality.
JUnit serves as the foundation for unit testing, offering user-friendly yet powerful features. Platforms like Machinet.net automate the process of writing unit tests using Mockito to enhance code quality and productivity.
Effective unit test cases require best practices and understanding of test coverage. Advanced JUnit features like annotations and assertions improve the expressiveness and maintainability of tests. Integrating Mockito with JUnit allows efficient mocking of external dependencies. By adhering to best practices and utilizing platforms like Machinet.net, developers can create robust software products through effective unit testing.
Start revolutionizing your unit testing with Machinet.net now!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.