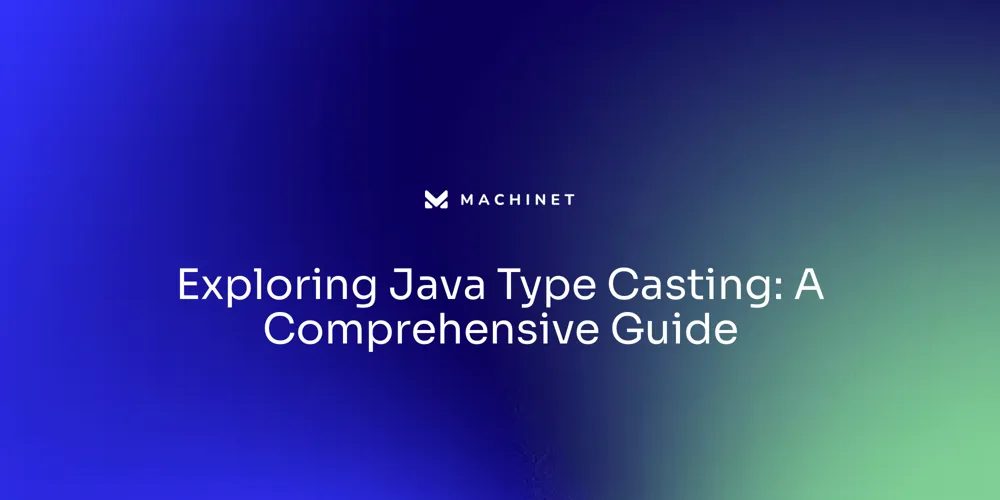
Table of Contents
- What is Type Casting in Java?
- Primitive Type Casting
- Reference Type Casting
- Understanding Upcasting and Downcasting
Introduction
Type casting in Java is a powerful feature that allows developers to convert variables from one data type to another, providing flexibility in programming. This article explores the two primary forms of type casting in Java: primitive and reference.
It discusses how primitive type casting enables the conversion between simple types and how reference type casting allows for interaction between objects within a class hierarchy. Additionally, the article highlights the advancements in type casting brought by pattern matching, a modern Java feature that combines safety and expressive power. With the recent release of JDK 21 and its advancements in virtual threads and garbage collection, Java continues to evolve as a robust platform for enterprise-level software development.
What is Type Casting in Java?
Java's type casting is a pivotal feature that enables developers to convert variables from one data type to another, enhancing flexibility in programming. There are two primary forms of type casting: primitive and reference.
Primitive type casting involves converting between simple types like int, char, and float, using syntax where the target type is specified in parentheses, followed by the variable name. For instance, casting a double to an int might look like (int) myDoubleVar
, a necessary step when dealing with smaller type ranges to prevent compile errors and information loss.
Reference type casting, on the other hand, pertains to objects and classes, allowing for the interaction between different objects within a class hierarchy. This form of casting ensures that methods and properties of one class can be accessed by another, under specific hierarchical conditions.
Pattern matching, a modern Java feature, has enriched type casting by merging the safety of traditional casting with the expressive power of instance pattern matching. This approach simplifies the process of extracting and operating on data, making the code more intuitive and readable. For example, instanceof
now checks both value and type, eliminating the need for manual range checks, a task developers have been meticulously coding for years. The recent release of JDK 21 has brought advancements such as virtual threads and a new garbage collector, indicating Java's ongoing evolution to meet the demands of modern software development. These enhancements are designed to streamline the creation of scalable, concurrent applications, further solidifying Java's role as a robust platform for enterprise-level software.
Primitive Type Casting
Type casting in Java is a fundamental concept for manipulating different primitive data types. When casting from a type with a smaller range to one with a larger range—such as an int to a long, known as widening conversion—this process occurs implicitly, without the need for explicit syntax, as it preserves precision. Conversely, narrowing conversion, like converting a long to an int, requires explicit casting due to the potential loss of information.
This ensures that the programmer is aware of the potential for precision loss, a critical aspect when striving for clean Java code that is not only correct but also expressive and maintainable. The recent advancements in Java, such as the introduction of pattern matching in JDK 21, underscore the language's evolution towards more intuitive and readable code. Pattern matching provides a more elegant approach for operations like type checks and casts, combining the convenience of an assignment statement with the safety of pattern matching, which inherently checks for both value and type.
This modern feature simplifies the tedious range checks that developers have been manually coding for nearly three decades. With the incorporation of pattern matching, Java code becomes more concise and aligned with the principles of writing clean, maintainable code. As Java continues to evolve, features like pattern matching and sealed classes contribute to the language's robustness and developer-friendly nature.
Reference Type Casting
In Java programming, understanding the interplay between reference types and their casting is pivotal. Casting is the process of transforming one reference type into another, often necessitated when dealing with a superclass reference variable and a subclass object.
To achieve this, one must explicitly declare the desired subclass type in parentheses preceding the reference variable. A common misconception about Java's mechanics is its parameter passing behavior.
Clarifying the concept, Java operates on a 'pass-by-value' basis, meaning it passes a copy of the value for primitive types and a copy of the reference's value for objects. This distinction is crucial for junior developers to grasp as it underlines that while object properties can be modified within a method, the reference itself remains unaltered.
Pattern Matching in Java further enhances code readability and conciseness, particularly when dealing with complex data structures. It involves matching a value against a pattern comprising variables and conditions, allowing for intuitive code and easier data extraction and operation on data structures. The number of reachable methods in Java applications is a metric that reflects the scope of analysis and compilation workload. For example, the Spring Petclinic application demonstrates a significant saving in analysis time when applying a rapid type analysis (RTA) instead of a more traditional approach. This illustrates the efficiency gains possible with a deep understanding of Java's syntax and semantics.
Understanding Upcasting and Downcasting
Type casting in Java is a crucial concept, especially when dealing with object-oriented programming. Upcasting is straightforward, where a subclass object is safely assigned to a superclass reference without the need for explicit casting. It's akin to setting a specific detail within a broader category.
For instance, a Dog, which is a subclass, can naturally fit into the category of an Animal, the superclass. Conversely, downcasting is more nuanced. It involves casting a superclass reference back to a subclass reference, which requires an explicit type cast.
This can be risky, as it presupposes that the object indeed belongs to the specific subclass, and failing to ensure this leads to a ClassCastException. The evolution of Java continues to introduce features that enhance code readability and efficiency. With the advent of JDK 21, Java developers have been given tools like virtual threads and a generational Z garbage collector to improve application performance.
Moreover, Java's adoption rates are telling of its progressive nature. Post the release of Java 21, 1.4% of applications monitored started using it—a significant jump compared to the uptake of earlier versions. This illustrates a growing confidence in the newer Java releases among developers.
In the realm of pattern matching, Java's approach simplifies the handling of complex data structures by allowing values to be matched against patterns, which can include variables and conditions. When a match is found, the corresponding parts of the value are bound to the variables in the pattern, leading to more intuitive and readable code. This modern feature contrasts with traditional methods, offering a more streamlined way to work with data structures.
Logging frameworks are essential in identifying and resolving bugs in software applications. A staggering 91% of Java applications utilize logging frameworks to troubleshoot issues, with Log4j, JBoss Logging, and Logback being the most prevalent. The widespread use of these frameworks underlines their importance in maintaining application health and performance.
Conclusion
In conclusion, type casting in Java is a powerful feature that allows developers to convert variables from one data type to another, providing flexibility in programming. There are two primary forms of type casting: primitive and reference. Primitive type casting involves converting between simple types like int, char, and float.
It ensures that the programmer is aware of potential precision loss and prevents compile errors and information loss. On the other hand, reference type casting pertains to objects and classes, allowing for interaction between different objects within a class hierarchy. Pattern matching, a modern Java feature introduced in JDK 21, has enriched type casting by merging safety with expressive power.
It simplifies the process of extracting and operating on data, making the code more intuitive and readable. With pattern matching, Java code becomes more concise and aligned with the principles of writing clean and maintainable code. The recent advancements in Java, such as virtual threads and a new garbage collector in JDK 21, highlight its ongoing evolution to meet the demands of modern software development.
These enhancements streamline the creation of scalable and concurrent applications, solidifying Java's role as a robust platform for enterprise-level software. Overall, type casting in Java empowers developers to manipulate different data types effectively. The incorporation of pattern matching and other advancements showcases Java's commitment to providing intuitive and readable code while maintaining its position as a leading language for enterprise-level software development.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.