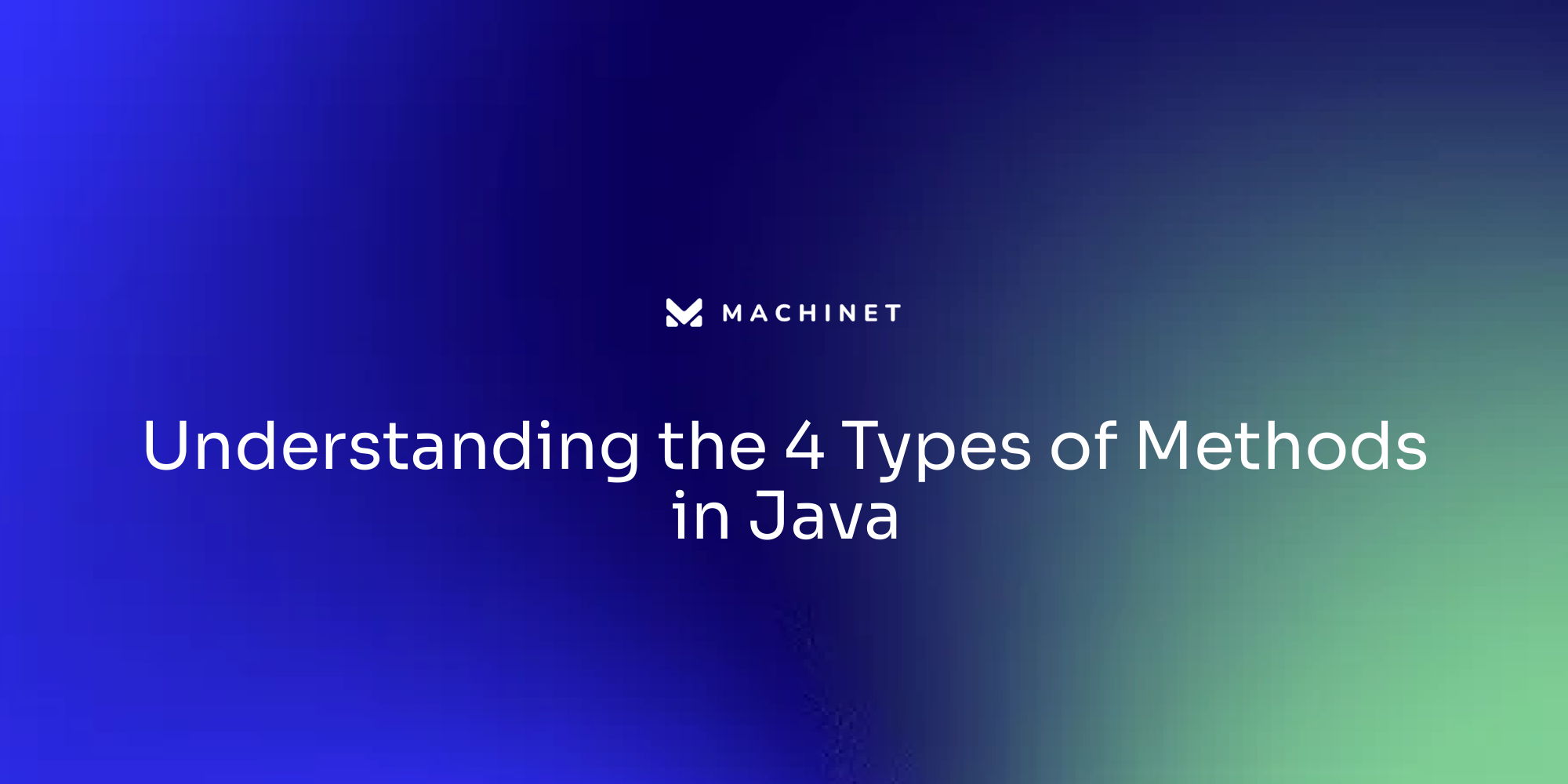
Introduction
Java methods are essential constructs that encapsulate code to perform specific tasks, fundamentally supporting code reusability and modular programming. This design allows developers to call the same code multiple times without needing to rewrite it, significantly enhancing code maintainability and readability. By organizing code logically, methods simplify debugging and testing, promoting collaboration among team members.
Additionally, Java supports various types of methods, such as predefined, user-defined, instance, static, abstract, and final methods, each serving unique purposes. Understanding these methods and adhering to best practices, such as keeping methods focused and using meaningful names, ensures clean, maintainable, and scalable code, crucial for both individual and collaborative development projects.
What are Java Methods?
Programming functions are crucial elements that enclose instructions to carry out particular activities, fundamentally aiding in the reuse of programming components and modular development. This design enables developers to invoke the same functionality multiple times without needing to rewrite it, significantly enhancing maintainability and readability. A technique in the programming language is made up of several essential elements: a name, which identifies the method; a return type, which indicates what kind of value, if any, the method will return; parameters, which are inputs that the method can accept; and a body, which contains the executable instructions. Adopting modular programming in a programming language not only simplifies easier management of scripts but also conforms to best practices for creating high-quality, maintainable software.
Benefits of Using Java Methods
Methods in Java significantly enhance readability and maintainability by organizing logic, which simplifies debugging and testing. This logical structure aids developers in clearly understanding the system's functionality, reducing the time and effort needed to make modifications. Employing techniques also encourages teamwork among group members, as various individuals can operate on distinct approaches simultaneously, ensuring smoother integration and minimizing conflicts.
The significance of maintainable and readable programming cannot be overstated. Code is read far more often than it is written, and significant function names make it easier for developers to comprehend the logic and intent, reducing the likelihood of errors. This readability is crucial for collaborative environments where team members need to quickly understand and communicate about the codebase.
Additionally, techniques enable the reuse of programming, an essential ability for any developer. Utilizing well-organized approaches across various sections of a project conserves time and effort, guaranteeing consistency and dependability. This is especially crucial in extensive projects or microservices, where sustaining high-quality programming is vital despite the smaller module sizes.
By following programming guidelines and optimal techniques, teams can guarantee that procedures are consistently executed, further improving the overall quality of the software. Instruments that oversee databases, produce programming fragments, and manage version control are essential in upholding this consistency. They enable seamless collaboration by providing a unified work environment and tracking changes made by different team members, thus fostering effective communication and integration.
In conclusion, utilizing techniques in a programming language not only arranges scripts but also enhances teamwork and script upkeep, rendering it an essential practice for any development initiative.
Types of Methods in Java
The programming language supports various kinds of functions, each serving distinct purposes. Understanding these types is essential for effective programming and code organization. Techniques in the programming language can be broadly categorized into predefined and user-defined functions. Predefined functions, such as System.out.println() and Math.max(), are already available in the Java Class Library and can be used directly. User-defined functions, on the other hand, are created by developers to carry out specific tasks within their programs, enhancing code reusability and encapsulation.
Within user-defined procedures, there are further classifications based on their functionality. Instance functions are linked to an object of a type and can access and modify instance attributes. For example, in the Dog class, instance functions like bark and ageOneYear interact with the object’s state, demonstrating how instance functions encapsulate behavior related to an object's state.
The programming language also features limited functions in the Foreign Function & Memory API that necessitate the --enable-native-access=module-name command-line option for use. These techniques connect native functions or data and are labeled as limited due to their intrinsic dangers. Adapting to such changes is crucial as access might be forbidden in future JVM versions.
Instance Methods
Instance functions are integral to object-oriented programming in Java. These techniques are linked to a particular example of a type, enabling them to reach instance variables and other instance functions directly. To utilize an instance function, you must first create an object of the type, which can then call the function. 'This is distinct from fixed functions, which are associated with the structure itself and can be invoked without generating an instance.'. The flexibility of instance functions supports a more maintainable and testable code structure, as opposed to the tightly coupled nature of static functions.
Static Methods
Static functions are procedures that are associated with the type itself rather than any instance of the type. This means they can be invoked without creating an instance of the type. These techniques are especially beneficial for utility functions that do not depend on instance variables. However, static functions can only directly interact with static variables and other static functions within the class.
While static functions have their benefits, overusing them can lead to maintenance and testing challenges. Tight coupling is one such issue, making it difficult to mock or stub static functions during unit testing. This complication arises because static functions are bound to their class, which prevents the substitution of mock objects.
Furthermore, static functions can introduce statefulness if they manipulate static properties. This shared state can lead to hard-to-diagnose bugs, as changes in one part of the application can have unintended effects elsewhere. Furthermore, static functions cannot be overridden, which restricts flexibility and hinders the capacity to enhance functionality through inheritance.
Employing static functions wisely, such as for constants or utility purposes, is recommended. However, favor instance functions and properties to promote a more maintainable and adaptable codebase. This approach aligns with best practices in software development, emphasizing scalability, maintainability, and ease of testing.
Abstract Methods
Abstract functions in Java are described without offering an implementation within abstract types. This design decision requires that any subclass deriving from the abstract type must execute these functions. By enforcing this requirement, abstract functions promote polymorphism and ensure consistency across different subclasses. This way, all subclasses adhere to a specific contract, thereby facilitating a structured and predictable development process. Utilizing abstract techniques efficiently can result in more modular and maintainable code, as they enable developers to establish a common interface for a set of related types.
Final Methods
When a function is declared as final in Java, it can't be overridden by any subclass. This guarantees that the approach's execution stays the same, preserving the original behavior established in the parent class. This feature is particularly useful for preserving the integrity and security of essential processes. By preventing subclasses from altering these functions, developers can avoid unintended side effects and ensure consistent performance across different parts of an application. This consistency is crucial in large-scale systems where unpredictable changes could lead to significant issues.
Method Parameters and Return Types
Methods in Java can accept parameters, allowing them to perform more flexible and dynamic operations. For instance, a technique that calculates the sum of two numbers can accept those numbers as parameters, rather than using fixed values. Parameters allow functions to handle various data inputs with each call, improving reusability and efficiency.
Furthermore, functions have return types that indicate the kind of value they will return. This is crucial for ensuring that the expected output type is clearly defined and understood. For example, a technique that performs a calculation might return an int
or double
type, depending on the precision required. If a procedure does not return any value, it is declared with a void
return type. This indicates that the process performs its operations without providing any output, such as a function that prints information to the console.
Method Overloading and Overriding
Function overloading and function overriding are essential ideas in Java programming that improve the adaptability and capability of functions within a structure. Function overloading enables a class to possess several functions with the identical name, distinguished by their parameter lists. This capability allows developers to utilize the same function name for various types of input, enhancing code readability and reusability. For instance, an approach to add numbers can be overloaded to handle both integers and floats, providing a versatile solution for different data types.
Conversely, function overriding happens when a subclass offers a particular implementation for a function already defined in its superclass. This enables the subclass to customize or enhance the behavior of the inherited function, ensuring that the subclass can operate properly within its context. By overriding functions, developers can create more precise and specialized functionalities, adhering to the principles of polymorphism and inheritance in object-oriented programming.
Both techniques play a crucial role in creating robust and maintainable code. Overloading provides flexibility by enabling functions to manage different kinds of inputs effortlessly, while overriding guarantees that inherited functions can be tailored to satisfy the particular requirements of subclasses. These practices contribute to the overall efficiency and adaptability of software systems, making them essential tools in a developer's toolkit.
Best Practices for Using Java Methods
To maximize the effectiveness of methods, follow best practices like keeping methods focused on a single task and using meaningful names. Based on a project execution of Clean Architecture utilizing Spring Boot, following such principles guarantees tidy, manageable, and expandable programming. Avoid excessive parameters to maintain simplicity and readability. Proper documentation, as shown in the release notes for Java utilities such as JReleaser, and consistent programming styles also enhance quality and maintainability. Clean Architecture principles, as highlighted in a case study, integrate various patterns and methodologies to reinforce learning and practice of maintainable coding standards. A study has shown that adopting SOLID design principles significantly enhances code understanding, which is crucial for both individual and collaborative work environments where clear communication and effective collaboration are essential.
Conclusion
Java methods play a critical role in software development by promoting code reusability, maintainability, and readability. They encapsulate specific tasks, allowing developers to call upon the same code multiple times without redundancy. This modular approach not only simplifies debugging and testing but also supports effective collaboration among team members, making it easier to integrate different parts of a codebase developed by various individuals.
The benefits of using Java methods extend beyond organization; they enhance code clarity and facilitate teamwork. By employing meaningful method names and adhering to best practices, developers can create a code structure that is both easy to understand and modify. This is particularly important in collaborative environments where clear communication about the codebase is essential.
Furthermore, methods allow for code reuse, which is especially valuable in larger projects or microservices, ensuring consistent and reliable functionality throughout the application.
Understanding the different types of methods—such as instance, static, abstract, and final methods—equips developers with the knowledge needed to implement Java effectively. Each type serves a unique purpose, and recognizing when to use each is vital for maintaining a clean and efficient codebase. Additionally, concepts like method overloading and overriding enhance flexibility and functionality, allowing developers to tailor methods to specific needs while adhering to object-oriented principles.
By following best practices, such as focusing methods on single tasks and maintaining consistent documentation, developers can ensure that their code remains scalable and maintainable. Employing principles like Clean Architecture and SOLID design further reinforces the importance of structured coding practices, ultimately leading to the creation of high-quality software that meets the demands of modern development projects.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.