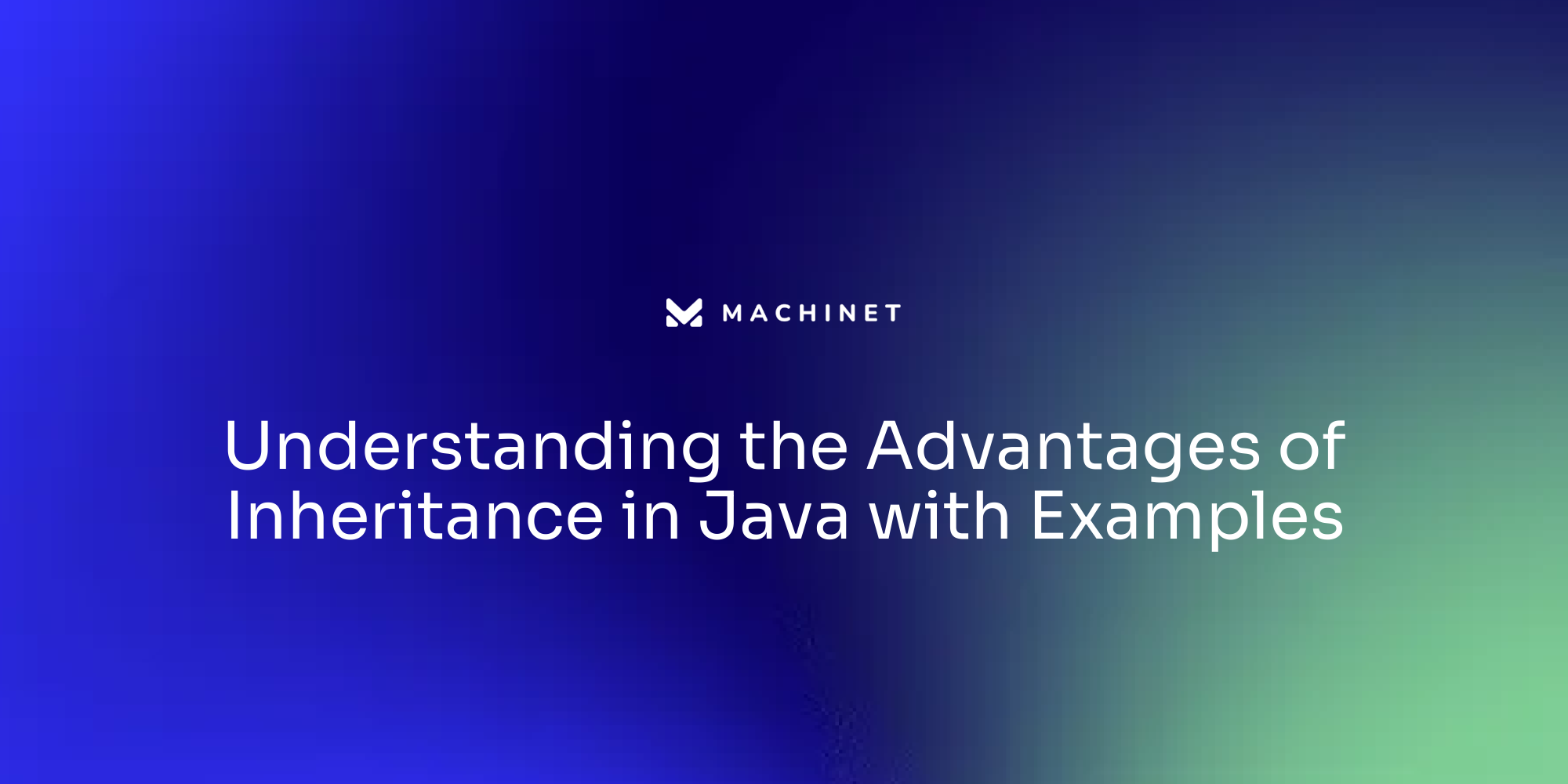
Introduction
Inheritance in Java stands as a fundamental concept within Object-Oriented Programming (OOP), empowering one class to inherit the properties and methods of another. This mechanism supports the creation of a subclass that can utilize and enhance the functionality of a superclass, promoting code reusability and mirroring real-world scenarios. By establishing hierarchical relationships, inheritance simplifies complex systems, making them easier to manage and understand.
This article delves into the essence of inheritance in Java, exploring its various types, such as single, multilevel, hierarchical, and multiple inheritance through interfaces. Additionally, it highlights the significant advantages of inheritance, including code reusability, polymorphism, encapsulation, extensibility, and modularity. Practical examples are provided to illustrate these concepts, along with a discussion on common pitfalls and best practices to ensure effective use of inheritance in software development.
Through this comprehensive exploration, developers can gain a deeper understanding of how to leverage inheritance to build robust, maintainable, and scalable Java applications.
What is Inheritance in Java?
Inheritance in Java is a cornerstone of Object-Oriented Programming (OOP) that allows one type to inherit the properties and methods of another. This powerful mechanism allows the creation of a new class, known as a subclass, which can utilize and extend the functionality of an existing class, referred to as a superclass. The hierarchical connection created through legacy not only encourages reuse of programming but also reflects real-world situations, making intricate systems simpler to comprehend and oversee.
For instance, consider a software development scenario where companies must decide between building custom software or purchasing off-the-shelf (OTS) solutions. Custom software can be tailored to specific needs, much like how subclasses in Java can be customized to add or override methods from their superclasses. On the other hand, OTS software might offer immediate functionality but may lack the adaptability that custom solutions provide.
This concept is akin to the need for a Software Bill of Materials (SBOM) in managing software supply chains. Similar to how legacy features enable the reuse and expansion of programming elements, comprehending the components within your software aids in reducing risks and guaranteeing adherence. Based on the 2024 Open Source Security and Risk Analysis (OSSRA) report, 96% of applications include open-source components, highlighting the significance of understanding what is in your software. By utilizing derived classes, developers can create robust systems that are both efficient and secure.
In summary, the concept of class hierarchy in Java not only simplifies maintenance of programs and enhances reusability but also aligns with best practices in software development, such as those highlighted in the OSSRA report. It provides a structured approach to building complex systems, ensuring that developers can focus on innovation while maintaining high standards of quality and security.
Types of Inheritance in Java
In Java, inheritance is a core concept of Object-Oriented Programming (OOP) that enables a new entity, known as the derived entity, to inherit attributes and behaviors from an existing entity, referred to as the base entity or superclass. This facilitates code reuse and the establishment of hierarchical connections among types, encouraging modularity and extensibility in software design. Java supports several types of inheritance:
-
Single Derivation: This type involves a derived entity inheriting from one base entity only. It is straightforward and allows the derived type to access the functionalities of the single superclass.
-
Multilevel derivation: In this structure, a type is derived from another derived type, forming a multi-layered hierarchy. For example, if Type A is a base type, Type B can be derived from Type A, and Type C can be derived from Type B, creating a chain of inheritance.
-
Hierarchical Inheritance: Multiple derived entities inherit from a single base type. This type enables various subclasses to share common characteristics of the base type while incorporating their unique attributes and methods. For instance, if Type A is a base type, both Type B and Type C can be derived from Type A.
-
Multiple Inheritance (via Interfaces): Although Java does not allow multiple lineage directly through types, it enables this feature through interfaces. A class can implement multiple interfaces, allowing it to inherit the abstract methods of those interfaces. This approach offers the flexibility of multiple lineage without the complexity associated with it.
The use of hierarchies not only streamlines functionality but also represents actual relationships by creating a coherent framework within the system. Understanding these inheritance types is essential for designing robust and maintainable software systems.
Advantages of Inheritance in Java
The use of Java's features provides numerous important advantages that improve both the effectiveness and upkeep of programs.
-
Program Reusability: By deriving from established structures, developers can utilize existing functionality, significantly decreasing repetition. This principle is akin to using pre-built blocks in constructing a large structure, ensuring that common functionalities do not need to be rewritten, thereby saving time and effort.
-
'Polymorphism: The process of deriving enables polymorphism, permitting objects to be regarded as examples of their parent type.'. This capability enhances flexibility in programming as it allows the same operation to perform differently on various types, adapting to specific needs without changing the overall system design.
-
Encapsulation: Inheritance helps in encapsulating related properties and methods within a single class hierarchy. This encourages structured and modular programming, making it simpler to handle and comprehend. Encapsulation ensures that the internal representation of an object is hidden from the outside, only exposing a controlled interface.
-
Extensibility: New functionality can be added to existing code without modifying it, making applications easier to extend and maintain. This modular approach allows for the integration of new features or updates without disrupting existing system operations, much like adding new rooms to a building without changing its fundamental structure.
-
Modularity: The use of parent-child relationships encourages a modular design, making it easier to manage and understand large codebases. By breaking down a complex system into smaller, more manageable parts, developers can isolate and address specific functionalities more efficiently, leading to more robust and scalable applications.
These advantages align with the core principles of Object-Oriented Programming (OOP), promoting a structured and systematic approach to software development. As applications grow in complexity, utilizing hierarchical relationships effectively can lead to more maintainable and scalable solutions, much like the transition from monolithic to more modular architectures in software development.
Examples of Inheritance in Java
-
Single Inheritance Example:
java class Animal { void eat() { System.out.println("Eating..."); } } class Dog extends Animal { void bark() { System.out.println("Barking..."); } }
In this example, theDog
class inherits theeat()
method from theAnimal
class. This illustrates how single lineage permits a type to originate from a sole base type, a fundamental aspect of Java's object-oriented programming model. -
Multilevel Inheritance Example:
java class Animal { void eat() { System.out.println("Eating..."); } } class Dog extends Animal { void bark() { System.out.println("Barking..."); } } class Puppy extends Dog { void weep() { System.out.println("Weeping..."); } }
Here,Puppy
inherits fromDog
, which in turn inherits fromAnimal
. This demonstrates multilevel derivation, where an entity is derived from another entity that is also derived from a different one, enabling a hierarchical arrangement of entities. -
Hierarchical Inheritance Example:
java class Animal { void eat() { System.out.println("Eating..."); } } class Dog extends Animal { void bark() { System.out.println("Barking..."); } } class Cat extends Animal { void meow() { System.out.println("Meowing..."); } }
In this case, bothDog
andCat
inherit fromAnimal
, showcasing a hierarchical structure. This method of passing down traits enables several classes to derive from one base class, encouraging code reuse and a tidy organization.
Common Pitfalls and Best Practices
Although the passing down of properties is a powerful feature in object-oriented programming, it can result in problems if not used wisely. Here are some common pitfalls:
- Overusing Inheritance: Favoring composition over class hierarchy where possible can help avoid tight coupling and maintain flexibility. As Bruce Lee once said, "Simplicity is the key to brilliance." Maintaining cognitive complexity at a minimal level through the use of clear and straightforward design patterns, such as composition, can enhance comprehensibility and upkeep.
- Diamond Problem: The diamond problem arises from multiple parent classes, leading to ambiguity and complexity. Utilizing interfaces can mitigate this issue, ensuring clarity and reducing the chances of hidden bugs. Henry Ford emphasized the importance of simplicity: "As simple as it looks, composing functions can be a turning point for creating neat and beautiful functions."
- Hidden Members: Subclasses can hide superclass members, leading to confusion and potential errors. To avoid this, it is essential to document relationships and responsibilities clearly, ensuring that the hierarchy is easily comprehensible.
Best practices include: - Keep inheritance hierarchies shallow to reduce complexity and improve code readability. - Use interfaces to achieve multiple inheritances while maintaining clarity. - Document the relationships and responsibilities clearly, making the codebase easier to understand and maintain.
A practical example of these principles in action can be seen in the 2024 OSSRA report, which highlights the importance of knowing what's in your code. With 96% of analyzed applications containing open source components, maintaining clarity and simplicity through proper design patterns becomes crucial in managing software supply chains effectively.
Conclusion
Inheritance in Java serves as a foundational pillar of Object-Oriented Programming, enabling classes to inherit properties and methods, thereby promoting code reusability and simplifying complex systems. The article delineates various types of inheritance, including single, multilevel, hierarchical, and multiple inheritance through interfaces, each offering unique advantages for structuring code efficiently. By establishing clear hierarchical relationships, developers can create modular and extensible applications that mirror real-world scenarios.
The advantages of inheritance extend beyond mere code reuse. It fosters polymorphism, encapsulation, and modularity, allowing for flexible and maintainable software design. The practical examples provided illustrate how inheritance can be effectively implemented in Java, showcasing its capabilities to enhance the functionality of derived classes while maintaining a clean codebase.
However, while inheritance is a powerful tool, it is essential to use it judiciously to avoid common pitfalls such as overusing inheritance, encountering the diamond problem, and unintentionally hiding superclass members. Adhering to best practices, such as favoring composition over inheritance where appropriate and maintaining clear documentation, can lead to more robust software solutions. Ultimately, embracing these principles will empower developers to build scalable and maintainable Java applications, ensuring high standards of quality and security.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.