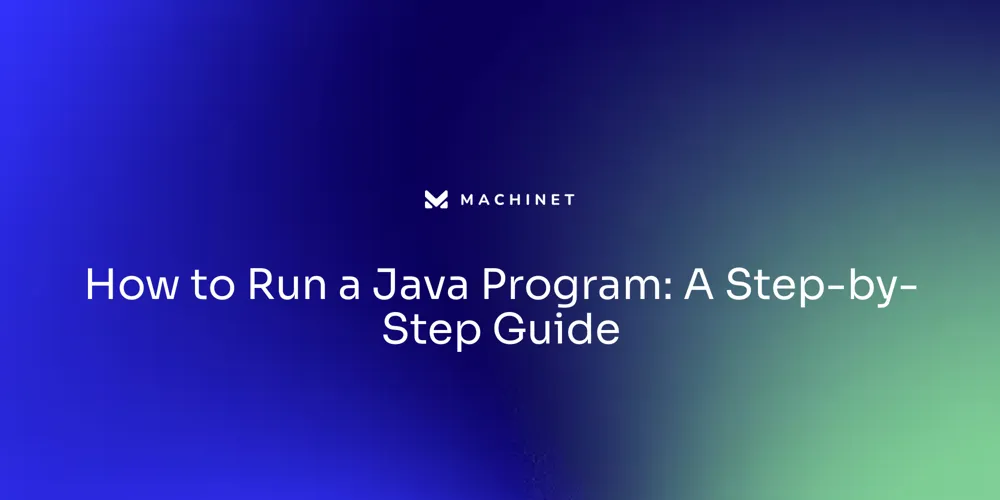
Table of Contents
- Setting Up Your Environment
- Creating a Java Program
- Compiling a Java Program
- Executing a Java Program
- Understanding the Compilation and Execution Process
- Common Errors and Troubleshooting
- Best Practices for Writing and Running Java Programs
Introduction
Setting up your Java development environment is essential to start coding and running Java applications. In this article, we will guide you through the process of setting up your environment, creating a Java program, compiling and executing it, understanding the compilation and execution process, troubleshooting common errors, and following best practices for writing and running Java programs.
By the end of this article, you will have a solid foundation for developing Java applications that are efficient, maintainable, and reliable. Let's get started!
Setting Up Your Environment
Setting up your Java development environment is essential to start coding and running Java applications. First, you'll need to install the Java Development Kit (JDK), which provides the necessary tools for developing Java programs.
The JDK can be downloaded from the official Oracle website. After installation, it's crucial to configure your system's PATH environment variable to include the JDK's bin directory, ensuring that the Java commands are available from the command line.
Next, an Integrated Development Environment (IDE) will significantly streamline your development process. Popular choices include IntelliJ IDEA, Eclipse, and NetBeans, each offering a range of features to aid in writing, testing, and debugging Java code.
IntelliJ IDEA, developed by JetBrains, is particularly noteworthy, as it is regarded as a leading IDE for Java and Kotlin development. This IDE helps developers stay current with the latest industry trends and caters to the evolving needs of modern businesses.
Once your IDE is installed, you can create a new Java project, which serves as a structured environment for your code files and resources. This step is critical as it organizes your work and allows for efficient management of complex codebases. Java's enduring popularity and the principle of 'Write Once, Run Anywhere' make it a staple in the tech industry, with many applications and websites relying on its presence. As highlighted in the State of Developer Ecosystem report, Java continues to be relevant amidst the rapid technological advancements and the growing interest in AI, with tools like ChatGPT and GitHub Copilot being widely used by developers. By following these steps, you'll be well on your way to developing Java applications that can run seamlessly across different platforms, embodying Java's fast, secure, and reliable nature, suitable for everything from mobile apps to enterprise-level solutions.
Creating a Java Program
To craft a Java program, such as one calculating the area of a circle, begin by establishing the foundation of your class. In your IDE, create a new Java class, giving it a name that reflects its purpose.
Within this class, carve out the main method, which is the gateway for your program's execution. Here you'll translate your algorithm—a step-by-step plan for solving your problem—into executable code.
This could involve pseudocode, a blend of natural language and programming syntax, to outline the logic before diving into Java specifics. When writing your code, remember to follow standard naming conventions for clarity; 'Customer' is far more descriptive than 'Ctr', for example.
Ensure your code is not only functional but also adheres to best practices for maintainability and readability, aligning with the adage to 'make it work, make it right, then make it fast.' This approach underscores the importance of clear and methodical development, from initial concept to final optimization.
Once your code is written, encapsulate your efforts by saving the file with a .java extension, sealing your program's logic within. As the tech industry evolves with trends like the integration of Artificial Intelligence tools—77% of developers use ChatGPT and 46% use GitHub Copilot—your commitment to quality and adherence to the latest practices will be paramount in maintaining relevance in the ever-changing landscape of software development. In line with these advancements, Machine, an AI plugin available exclusively as a JetBrains plugin, enhances the Java programming experience. It uses OpenAI and in-house validation models trained on high-quality, community-approved source code and natural language to generate code and unit tests automatically. By simply describing the desired outcome or selecting a method, Machine can create context-aware chat responses or unit tests, streamlining the development process significantly.
Compiling a Java Program
Compiling your Java program is the first step towards transforming your high-level, user-friendly code into bytecode, the lower-level code that machines can understand. Here's how to do it effectively:
1.
Open the Java file in your IDE: Ensure the file you intend to compile is open in your Integrated Development Environment (IDE). This is where you write your code and initiate the build process.
- Compile the project: Utilize the build or compile feature in your IDE.
This critical step translates your Java code into bytecode, which is represented by .class
files. It's a process akin to crafting a detailed blueprint before commencing the actual construction of a building.
- Examine the compilation output: After you've compiled the code, it's vital to inspect the Ide's console for errors or warnings. This is similar to conducting a risk analysis in software development, where identifying and addressing potential issues early on can save time and prevent complications down the line. Remember, just like how clear communication and requirements are essential in high-quality software development, compiling your code with precision sets the foundation for a successful application. Moreover, adhering to principles such as the Single Responsibility Principle during coding ensures that your methods are concise and maintainable, simplifying the compilation process.
Executing a Java Program
Executing a Java program involves a few key steps after compilation. Firstly, ensure the presence of the .class bytecode file in your development environment.
If you're using an Integrated Development Environment (IDE) like PyCharm, which is favored by Scieneers for its robust refactoring capabilities and comprehensive support for programming tasks, you'll find it streamlines the process. Once located, you can initiate the program's execution with the Ide's run feature.
This triggers the JVM to process the bytecode and produce output. Following the execution, it's crucial to inspect the output in the console.
This step verifies that the program's behavior aligns with the expected results. As you assess the output, consider the principles of good software design like the Single Responsibility Principle (SRP), ensuring each method in your program has a distinct and clear purpose.
This approach prevents complexity and maintains manageability, especially in extensive codebases. Additionally, proper commentary can elucidate the rationale behind code segments, serving as invaluable documentation and guiding you and others through the logic and structure of the program. The importance of these practices is underscored by the findings from the State of Developer Ecosystem 2023 report, which reflects responses from over 26,000 developers worldwide. The report highlights trends in technology, indicating a significant growth in the tech sector and the integration of AI in development tools. With 77% of developers using AI tools like ChatGPT, and 46% utilizing GitHub Copilot, it's clear that developers are increasingly leveraging AI to streamline coding-related activities.
Understanding the Compilation and Execution Process
In the realm of software development, understanding the intricacies of how a Java program comes to life is essential. At its core, this involves two primary stages: compilation and execution.
During compilation, your human-readable Java source code is transformed into bytecode—a form of instruction set for the Java Virtual Machine (JVM). This bytecode is a universal language for the JVM, enabling it to execute the program on any platform that supports Java.
As for the execution phase, the JVM breathes life into the bytecode. It interprets each instruction, manages memory allocation, and carries out the necessary computations.
This stage is where the program's logic comes into play, and the JVM's role is to ensure that everything runs smoothly. By gaining a firm grasp of these stages, you equip yourself with the know-how to diagnose and resolve any hitches encountered during the running of your Java programs. A common adage in software engineering is to 'make it work, make it right, then make it fast.' This highlights the progression from getting your code to function, refining its structure, and finally, optimizing its performance. In the context of Java, this translates to writing clear and concise code, adhering to principles like the Single Responsibility Principle to avoid complexity, and ultimately ensuring that your Java programs are both efficient and maintainable.
Common Errors and Troubleshooting
Navigating Java program errors can be a daunting task, but with the right approach, it's manageable. Common issues include the 'ClassNotFoundError' which surfaces when the JVM can't locate a class.
To resolve this, ensure the class exists in the right package and verify the classpath's accuracy. Another typical error, 'NoClassDefFoundError', arises when a class definition isn't found at runtime.
Check that the class is in the classpath and dependencies are included. The 'NullPointerException' occurs when there's an attempt to use a null object reference.
Confirm that objects are initialized as expected. A technique known as rubber duck debugging, coined by Andrew Hunt and David Thomas, can be particularly helpful. By explaining your code to an inanimate object (like a rubber duck), you can clarify your thought process and uncover hidden bugs. This method encourages meticulous review of each code line, often leading to insights on the issue at hand.
Best Practices for Writing and Running Java Programs
In the journey of software development, the clarity of requirements and effective communication with stakeholders are pivotal. They form the blueprint that guides the purpose, functionality, and scope of the software, preventing a descent into a maze of confusion.
Adhering to these principles is essential for crafting high-quality, impactful software. When writing Java programs, it's vital to embrace standard code naming conventions.
Opt for simple, descriptive names rather than cryptic acronyms. For instance, 'Customer' is far more intuitive than 'Ctr', which could stand for multiple terms.
Such clarity in naming not only aids in understanding but also aligns with established programming language conventions. Methods are the cornerstone of organized code, encapsulating related instructions into cohesive units.
This segmentation allows for the management of complex tasks by breaking them down into smaller segments. Moreover, the Single Responsibility Principle (SRP) dictates that a method should focus on a single task, thereby avoiding unnecessary complexity and ensuring each method has a clear, well-defined purpose.
Proper commenting is another pillar of sound development practices. Comments should elucidate the reasoning behind code segments, particularly when dealing with intricate algorithms or data structures.
They act as in-line documentation that can clarify the structure and usage of the code, making it accessible for future reference and collaboration. Embracing modern tools can also significantly enhance the coding experience. Machinet, an AI-powered plugin for JetBrains, streamlines code and unit test generation. It uses context-aware AI to understand your project and generate the necessary code based on your descriptions. The AI unit test agent within Machine simplifies the creation of unit tests by analyzing the selected method and generating tests accordingly. This technology accelerates development by enabling you to code faster with fewer errors, and it helps bring your team up to speed with context-aware chat features. Ultimately, these best practices, coupled with innovative tools like Machinet, foster the creation of clean, maintainable code. Through meaningful naming, methodical structuring, insightful commenting, and the integration of AI-assisted coding, Java programs become models of efficiency and reliability.
Conclusion
In conclusion, setting up your Java development environment is crucial for coding and running Java applications. This involves installing the JDK, configuring the system's PATH variable, and using an IDE like IntelliJ IDEA.
Creating a Java program requires writing clear code, following best practices for maintainability, and saving the file with a .java extension. Compiling a Java program involves opening the file in the IDE, compiling it to bytecode, and examining the output for errors or warnings.
Adhering to principles like the Single Responsibility Principle simplifies compilation. Executing a Java program requires locating the bytecode file and initiating execution with the IDE's run feature.
Inspecting the output verifies expected behavior. Good software design principles and proper commenting enhance maintainability.
Understanding the compilation and execution process helps troubleshoot issues in Java programs. Writing clear code, adhering to principles like the Single Responsibility Principle, and optimizing performance ensure efficiency and maintainability. To write and run Java programs effectively, follow best practices such as standard naming conventions, organizing code into clear methods, and using proper commenting. Embracing tools like Machinet enhances coding experience. By following these guidelines, you can develop efficient, maintainable, and reliable Java applications across platforms. Embracing innovative tools and best practices ensures efficiency in today's tech industry.
Supercharge your Java coding with Machinet's AI-powered plugin!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.