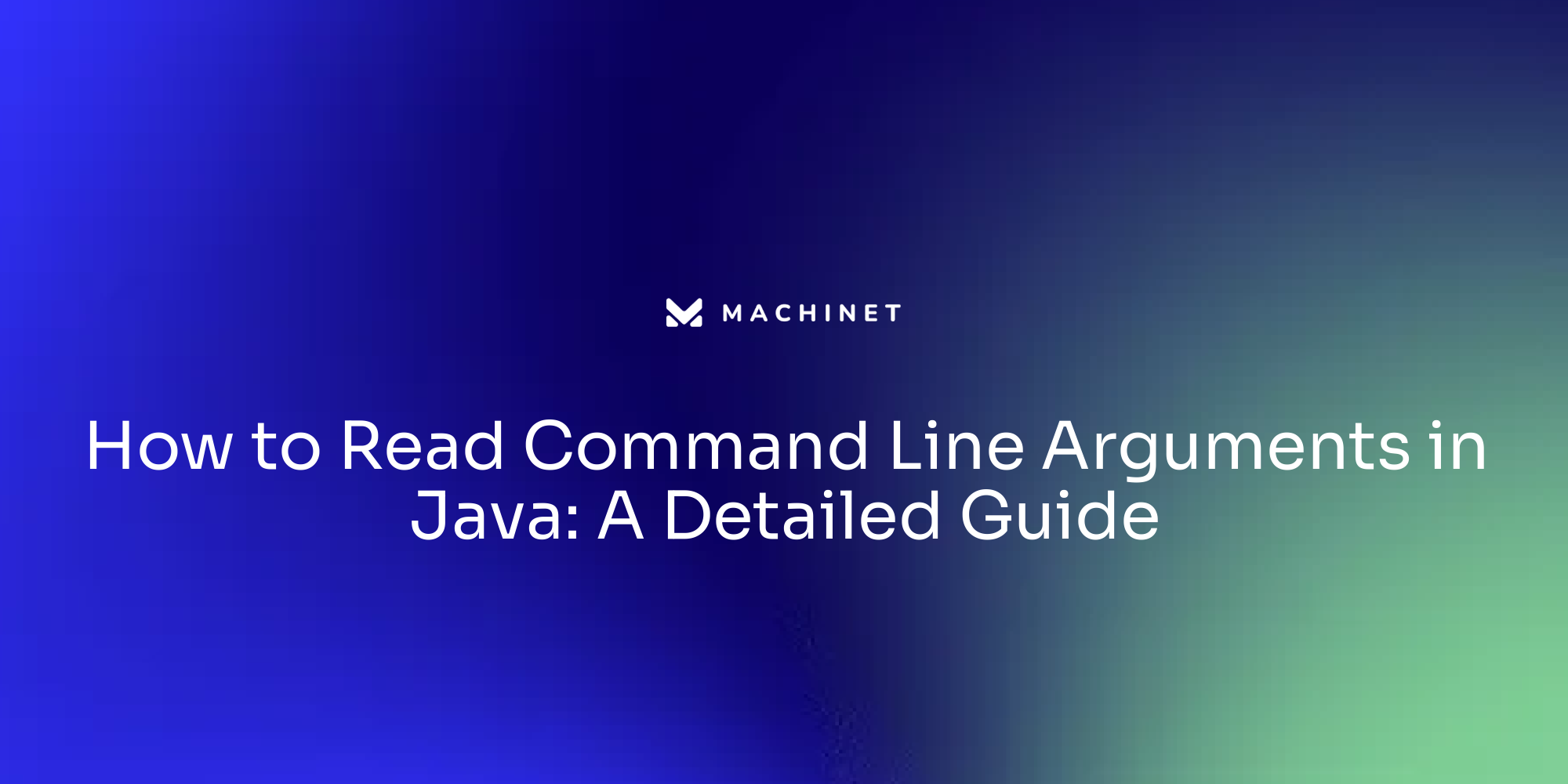
Introduction
Java command line arguments provide a powerful way to pass data to applications at runtime, enabling greater flexibility and control over program execution. Command line interfaces (CLI) are essential for building robust and user-friendly applications, allowing developers to create dynamic and adaptable software solutions. This article delves into the syntax and usage of command line arguments in Java, demonstrating how to pass, access, and process these arguments efficiently.
It also covers best practices for handling errors and validating inputs to ensure program stability. Additionally, the article explores advanced topics, such as using third-party libraries like Apache Commons CLI and JCommander, to simplify argument parsing and enhance the overall user experience. By understanding and implementing these techniques, developers can significantly improve the functionality and maintainability of their Java applications.
Understanding Command Line Arguments Syntax
Java console inputs enable you to transmit information to your application during execution. These statements are provided as strings in an array named args
, which is a parameter of the main
method. The syntax for the main
method is:
java
public static void main(String[] args) {
// code to process command line arguments
}
When you execute a Java program, any arguments specified on the command line are passed into the args
array. For example, if you run java MyProgram arg1 arg2
, args[0]
will contain arg1
and args[1]
will contain arg2
. This feature is fundamental for creating command-line interfaces (CLI), which need to be user-friendly and robust. By utilizing libraries that make easier the analysis of input parameters, like those created in Apache projects such as Kafka and Maven, developers can improve the usability and effectiveness of their applications. Ensuring maintainability and clear documentation through meaningful variable names and comments is essential for collaboration and future code modifications.
Passing Command Line Arguments to a Java Program
To provide parameters to a Java program, you utilize the interface of your operating system. First, navigate to the directory where your compiled Java class resides. Then, run the following command:
bash
java MyProgram argument1 argument2
Replace MyProgram
with your actual class name, and argument1
, argument 2
with the values you want to pass. The program will then run, and the designated parameters will be accessible in the args
array within the main
method.
For more sophisticated terminal processing, Java developers frequently utilize libraries such as Apache Commons CLI. This library streamlines the interpretation and handling of input parameters, facilitating the definition of options and executing validation. Widely used in Apache projects like Kafka, Maven, Ant, and Tomcat, Apache Commons CLI streamlines the development of robust and user-friendly CLIs.
Accessing Command Line Arguments in Java
Retrieving parameters in your Java program is simple. Within the main
method, you can reference the args
array to obtain the inputs. For example:
java
public static void main(String[] args) {
for (String arg : args) {
System.out.println(arg);
}
}
This code snippet will print each command line argument passed to the program. You can utilize indexing to reach particular inputs, such as args[0]
for the first input. Utilizing command line arguments is essential for configuring your programs dynamically, especially when working in different environments like development, testing, or production. Using the System.getenv
method, you can also read environment variables to adjust the software's behavior based on the execution context. This capability is crucial for handling different configurations seamlessly, ensuring your application can operate efficiently across various setups.
Processing Command Line Arguments: Converting Strings to Numbers
Frequently, terminal inputs require conversion from text to different data formats, like whole numbers or floating-point values. Java provides wrapper classes like Integer
and Double
for this purpose. For example:
java
public static void main(String[] args) {
if (args.length > 0) {
int number = Integer.parseInt(args[0]);
System.out.println("Number: " + number);
}
}
In this example, the first command line argument is converted to an integer using Integer.parseInt()
. However, it's crucial to handle potential exceptions that may arise from invalid input. Assertions validate your assumptions. Use them to protect your code from an uncertain world. Where possible, the function or object that allocates a resource should be responsible for deallocating it.
Handling Errors and Invalid Command Line Arguments
Verifying command line inputs is essential for avoiding mistakes during program execution. The initial action is to verify the size of the args
array to confirm the necessary quantity of inputs is supplied. You can enhance this validation by using try-catch blocks to handle any exceptions that might occur during parsing:
java
public static void main(String[] args) {
try {
if (args.length < 1) {
System.out.println("Please provide an argument.");
return;
}
int number = Integer.parseInt(args[0]);
System.out.println("Number: " + number);
} catch (NumberFormatException e) {
System.out.println("Invalid number format.");
}
}
This approach helps in maintaining program stability by gracefully handling invalid inputs. For instance, if a user provides a value that cannot be parsed into an integer, the program will catch the NumberFormatException
and inform the user of the invalid input. This approach to validating and managing inputs ensures that your program can handle unexpected situations without crashing, enhancing overall reliability and user experience.
Best Practices for Command Line Argument Handling
To ensure robust command line argument handling in your Java programs, consider the following best practices to enhance functionality and user experience:
-
Validate the number of arguments before processing: Ensuring the correct number of arguments are passed can prevent runtime errors and unexpected behavior. This step is crucial for maintaining the integrity of your program's execution flow.
-
Use descriptive error messages to guide users: Clear and informative error messages help users understand what went wrong and how to fix it. For instance, instead of a generic message, indicating which point is missing or wrong can save users time and frustration.
-
Handle exceptions gracefully to avoid program crashes: Implementing try-catch blocks and custom exception handling can prevent your program from crashing due to unexpected input. This approach ensures that your program can recover and provide meaningful feedback to the user.
-
Think about offering assistance guidelines when invalid inputs are detected: Providing a help feature or showing usage instructions when invalid inputs are entered can greatly enhance user experience. This practice helps users quickly learn how to interact with your program correctly.
-
Utilize significant titles for parameters to improve clarity: Selecting clear and detailed names for your input options makes your code simpler to comprehend and sustain. This practice is especially beneficial for collaborative projects or when revisiting your code after some time.
For example, the Apache Commons CLI library is a superb resource for developing terminal interfaces. It aids in defining CLI options, conducting basic validation, and streamlining the parsing of command inputs. This library is widely utilized in various Apache projects, such as Kafka, Maven, Ant, and Tomcat, showcasing its reliability and effectiveness in practical scenarios.
Example Use Cases: Reading and Processing Command Line Arguments
Command line arguments can significantly enhance the flexibility and functionality of Java applications in several key ways:
- Configuration Settings: Command line arguments allow for dynamic configuration of applications by passing file paths or specific configuration values required for execution. This flexibility means that the same software can be tailored to different environments or user needs without altering the source code.
- Input Data: By specifying input files directly through command line arguments, programs can handle data more flexibly and efficiently. This is especially beneficial for programs that handle various data sets, as it removes the requirement for hardcoding file paths.
- Flags and Options: Using flags (e.g.,
-verbose
) enables users to toggle features or modes within a program easily. This functionality not only simplifies the user experience but also enhances the discoverability of features within the software.
Command prompt interfaces (CLI) are crucial in attaining automation and remote access. Automation with CLI can drastically reduce time and errors in repetitive tasks, as highlighted by the efficiency of scripting in software development and system administration. Moreover, CLI provides a direct link to system resources, making it indispensable for managing remote systems and enhancing overall developer productivity.
Using Command Line Arguments to Pass Files and Configuration Settings
To manage files or configuration settings through command line arguments in Java, you can provide the file paths as inputs to your program. This method allows users to specify which file the software should process at runtime, enhancing flexibility. For instance:
java
public static void main(String[] args) {
if (args.length > 0) {
String filePath = args[0];
// Code to read the file
}
}
This approach is particularly useful because it simplifies the process of specifying input files during execution, making your application more adaptable to different environments and use cases. By doing so, you empower users to dynamically choose the files they want the software to process, which is essential for creating versatile and user-friendly tools. This method is essential for numerous Java programs, allowing efficient and effective file management directly from the terminal.
Advanced Topics: Using Third-Party Libraries for Command Line Argument Handling
Handling input parameters for intricate applications can be difficult. To streamline this process, leveraging third-party libraries like Apache Commons CLI or Jcommander can be highly beneficial. These libraries provide sophisticated capabilities for parsing input options, managing flags, and producing assistance messages automatically. This not only simplifies user input management but also enhances the overall efficiency of your program. For instance, Apache Commons CLI is widely used in projects such as Kafka, Maven, and Tomcat, demonstrating its reliability and effectiveness. By adopting such libraries, developers can focus on core functionalities while ensuring robust and user-friendly command line interface operations.
Conclusion
Java command line arguments are a vital component for enhancing application flexibility and user interaction. By utilizing the args
array within the main
method, developers can easily pass and access data at runtime, facilitating dynamic configurations and input management. The syntax is straightforward, and with the right practices, command line interfaces can be both robust and user-friendly.
Effective handling of command line arguments requires thorough validation and error management. By implementing best practices such as checking argument counts, providing clear error messages, and gracefully managing exceptions, developers can significantly improve the reliability of their applications. Additionally, libraries like Apache Commons CLI offer advanced parsing capabilities, making it easier to manage more complex command line interactions.
The potential applications of command line arguments extend beyond simple data input; they can also enhance configuration management and streamline file processing. By allowing users to specify settings and file paths at runtime, applications become more adaptable to various environments and user needs. Overall, mastering command line arguments in Java not only boosts functionality but also contributes to a more efficient and engaging user experience.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.