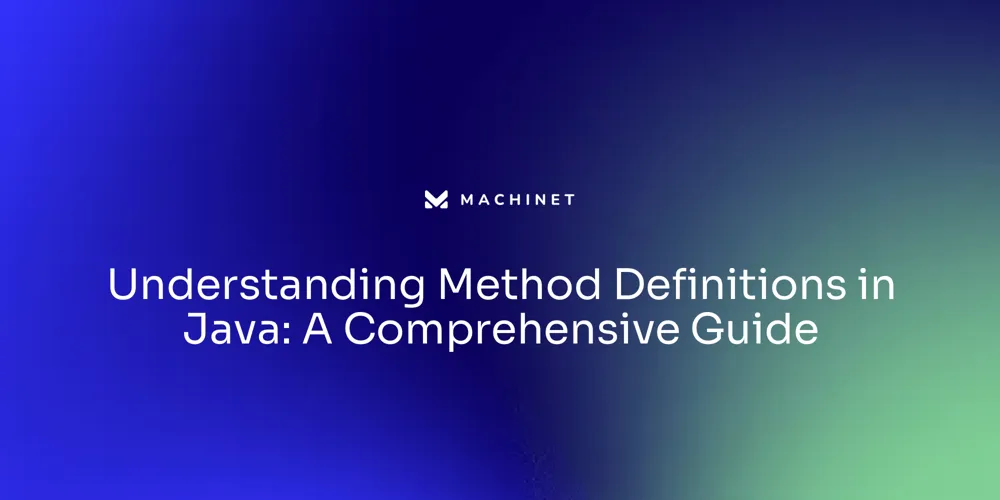
Table of Contents
- Method Declaration and Syntax
- Method Types: Instance, Static, and Abstract
- Method Parameters and Return Types
- Method Overloading and Overriding
Introduction
In the realm of Java programming, methods serve as the backbone, providing structure and facilitating the execution of specific tasks within a class. But what exactly is a method and how does it work?
This article explores the world of Java methods, from their declaration and syntax to their types, parameters, and return types. Whether you're a beginner or an experienced Java developer, understanding methods is essential for crafting robust and efficient applications. So, let's dive in and explore the ins and outs of Java methods.
Method Declaration and Syntax
In the realm of Java programming, methods serve as the backbone, providing structure and facilitating the execution of specific tasks within a class. A method's declaration encompasses its signature, which is composed of the access modifier, return type, name, and an optional list of parameters.
Here's a concise breakdown of these elements:
-
Access Modifier: This component, such as
public
,private
, orprotected
, dictates the method's visibility scope. - Return Type: It indicates the type of value that will be returned by the method, which could bevoid
if no value is returned,int
,String
, or other data types. -
Method Name: Following Java's naming conventions, this identifier allows the method to be invoked. - Parameter List: This is where input values are specified, each declared with its data type and name.
Java 21 introduced streamlined approaches to launching Java programs, emphasizing simplicity. For instance, a main
method no longer needs to be static, public, nor require a String[]
parameter.
A source file can implicitly declare a class, simplifying the typical Hello World
program to a mere few lines of code:
java
// HelloWorld.java
System.out.println("Hello, World! ");
This change reflects the principles of Single Responsibility and Non-static Context, where methods are designed to handle a singular task and are invoked in the context of an object, excluding the need for the static
keyword. Such advancements in Java's syntax and semantics not only enhance clarity and maintainability but also bring a sense of accomplishment to developers, recalling the joy of running their first Java program. The method body, enclosed in curly braces, contains the set of statements that perform the action, and if a return value is expected, a corresponding return statement is included. Unit testing, a critical aspect of software development, is also shaped by method design, as it focuses on testing individual methods in isolation to ensure they function as intended. By understanding and implementing these principles, developers can craft robust and efficient Java applications.
Method Types: Instance, Static, and Abstract
Java methods are the fundamental building blocks of any Java application, enabling developers to encapsulate a set of related instructions into a single, coherent unit. By dividing complex tasks into smaller, more focused methods, you can adhere to the Single Responsibility Principle (SRP), which dictates that a method should have one well-defined task. This principle is crucial for maintaining clean, understandable, and manageable code.
Java offers several types of methods to cater to different needs within your applications:
- Instance Methods: These methods are tied to a specific instance of a class and have the ability to interact with instance variables and other instance methods. They are typically invoked using the object they belong to, followed by the dot operator. - Static Methods: Instead of being associated with an instance, static methods are class-level and can be called without creating an object.
They have access only to static data members and other static methods within the class. - Abstract Methods: These are special methods that have no implementation in the abstract class or interface they are declared in. It is the responsibility of the subclasses or classes that implement the interfaces to provide concrete implementations for these methods.
Moreover, Java methods can be predefined or user-defined. Predefined methods, such as System.out.println()
for output and Math.max()
for calculating the maximum of two numbers, are readily available in the Java Class Library. User-defined methods, on the other hand, are crafted by developers to perform specific tasks within a program, enhancing reusability and modularity.
A well-named method is essential for software maintenance and comprehension. For instance, a study on Java methods revealed a considerable number of stable methods (13,137) compared to test oracles (400), highlighting the importance of consistent naming conventions that align with a method's behavior. Furthermore, with the release of Java Development Kit 23 (JDK), developers can look forward to new features like the Vector API and Stream Gatherers, which are set to enhance the language's capabilities and performance on different CPU architectures.
Method Parameters and Return Types
Java methods serve as essential building blocks, encapsulating a suite of related instructions into a cohesive unit, thus allowing complex tasks to be segmented into smaller, more digestible components. Each method in Java can take inputs known as parameters, which are specified within the parentheses of the method's declaration and can vary from zero to many. For instance, a method designed to add two integers would include two parameters representing the numbers to be summed.
This is exemplified in the simple addNumbers
method, which takes two integers, computes their sum, and returns the result. Parameters in Java adhere to a 'pass-by-value' mechanism, meaning that for primitive types, a copy of the actual value is passed, leaving the original value unaltered. Conversely, when object references are passed, it is the reference's value - the memory address - that is copied, not the object itself.
This subtle distinction allows for the modification of the object's properties within the method but prevents altering the reference to the object. Methods must also have a return type, indicated by the return Type
in the method signature. If no value is returned, void
is used to denote this.
The method's body, enclosed in curly braces, carries out the task at hand, and if a value is to be returned, it must conclude with a return
statement. Staying up-to-date with Java's evolution is crucial, as new features and changes, such as the transition from the break
keyword to yield
in switch expressions, can impact the way methods and parameters are used. Java 20, for example, introduces advancements like virtual threads and pattern matching for switch statements, which continue to refine and expand Java's capabilities in handling methods and their parameters.
Method Overloading and Overriding
Java's method overloading and overriding are key features that enhance the language's object-oriented nature. Method overloading enables defining multiple methods with identical names within a class, differentiated by their parameter lists.
This is not merely about convenience; it serves a practical purpose by allowing similar operations on different types of data. For instance, a method might be overloaded to handle both integers and floating-point numbers, thus maintaining code clarity and consistency.
Overriding, on the other hand, is a concept where a subclass offers a specific implementation of a method already present in its parent class. The subclass's method must match the parent's method in terms of name, return type, and parameters.
Overriding is a cornerstone of polymorphism, permitting a subclass to tailor a method to its needs or to respond differently to the same method call. These techniques are not without their challenges.
As noted in a case study involving Java 21's virtual threads, method overloading can inadvertently lead to complex issues such as unpredictable deadlocks, which may occur deep within the libraries used in a project. This highlights the importance of careful design and debugging practices. Moreover, when leveraging these features, developers must consider the Single Responsibility Principle to ensure methods remain focused and maintainable, typically aiming for 10 to 20 lines of code per method to avoid unnecessary complexity. The recent Java 20 release, and subsequent updates like Spring Boot 3.2.0, reflect an ongoing evolution of Java, emphasizing the need for developers to stay current with the language's capabilities and best practices. As methods for the smallest unit of program behavior, careful naming and implementation of methods are essential for software maintenance and comprehension. This is underscored by research suggesting that a significant portion of methods in Java class files remain stable and unmodified, indicating the importance of stable and well-considered method names and bodies.
Conclusion
In conclusion, Java methods are the backbone of Java programming, providing structure and facilitating the execution of specific tasks within a class. They are declared with an access modifier, return type, name, and optional parameters.
The introduction of streamlined approaches in Java 21 simplifies method declaration and enhances clarity and maintainability. Java offers different types of methods, including instance methods tied to a specific instance of a class, static methods associated with the class itself, and abstract methods without implementation.
These methods can be predefined or user-defined, allowing for reusability and modularity. Parameters in Java methods allow for inputs to be passed into the method's declaration.
They follow a 'pass-by-value' mechanism where primitive types pass a copy of the value while object references pass the reference's value. Methods must also have a return type, indicated by the returnType
in the method signature.
Method overloading and overriding are key features that enhance Java's object-oriented nature. Overloading allows for multiple methods with identical names but different parameter lists, while overriding enables subclasses to provide specific implementations of methods already present in their parent classes. It is important for developers to stay up-to-date with Java's evolution as new features and changes can impact method usage. Careful design and debugging practices are crucial when leveraging method overloading and overriding to avoid complex issues. Overall, understanding Java methods is essential for crafting robust and efficient applications. By adhering to best practices such as using consistent naming conventions and following the Single Responsibility Principle, developers can create maintainable code that allows for scalability and comprehension.
Master Java methods and level up your coding skills with Machinet!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.