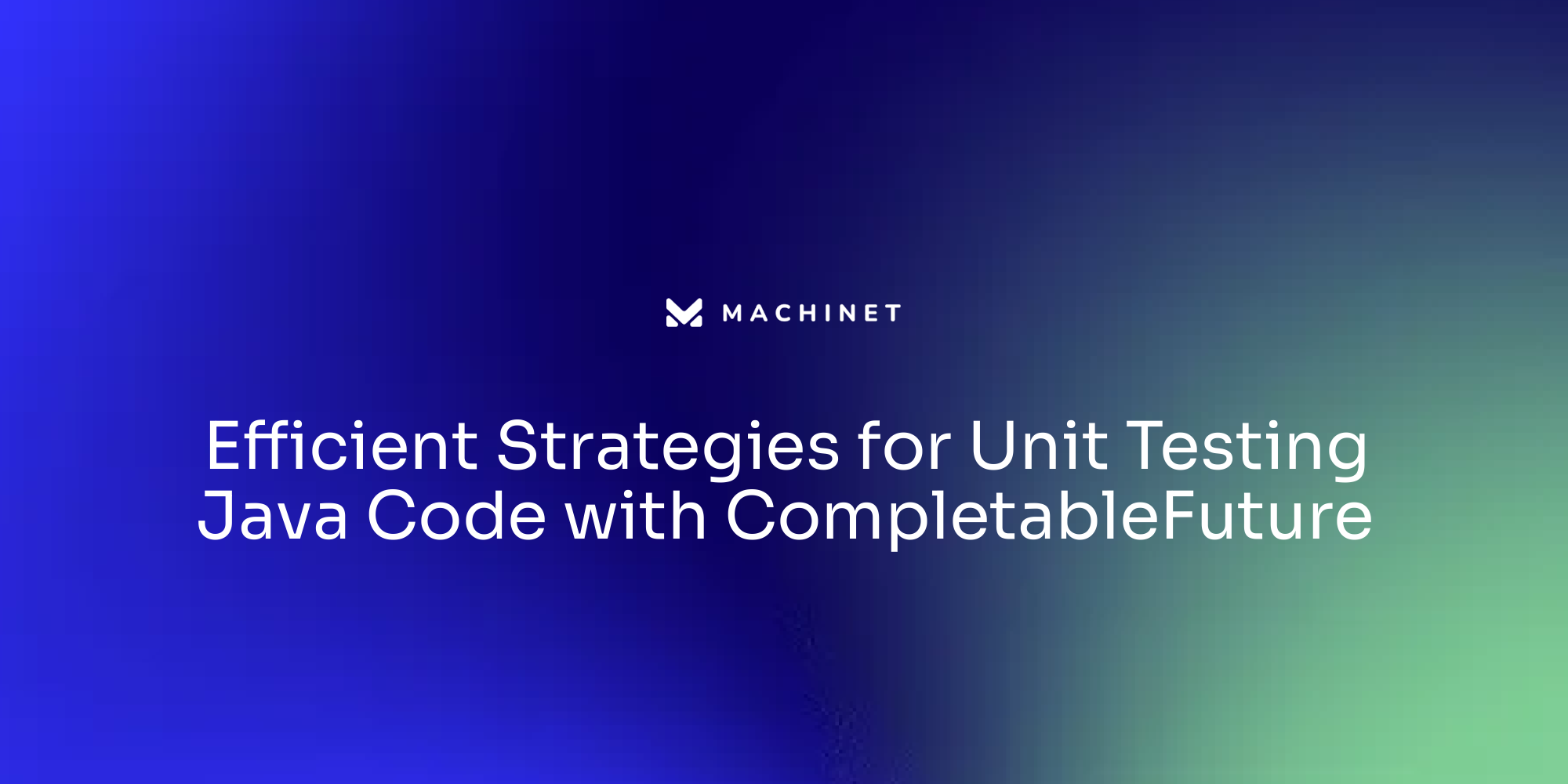
Table of contents
- Understanding CompletableFuture in Java
- The Importance of Unit Testing CompletableFuture
- Strategies for Effective Unit Testing with CompletableFuture
- Mocking Dependencies in CompletableFuture Unit Tests
- Handling Exceptional Cases in CompletableFuture Tests
- Testing Asynchronous Behavior with CompletableFuture
- Implementing Robust and Flexible Testing Frameworks for CompletableFuture
- Balancing Workload and Deadlines in the Unit Testing Process
Introduction
CompletableFuture is a powerful class in Java that facilitates asynchronous programming and non-blocking code execution. It offers significant advancements over the traditional Future interface, providing robust tools for managing and controlling asynchronous operations. With features such as callback functions, chaining, and exception handling, CompletableFuture addresses the limitations of Futures and offers a more modern and streamlined approach to asynchronous programming in Java. In this article, we will explore the functionalities and benefits of CompletableFuture, as well as strategies for effective unit testing and exception handling in CompletableFuture tasks. By understanding and harnessing the power of CompletableFuture, developers can enhance the efficiency, reliability, and quality of their software solutions
1. Understanding CompletableFuture in Java
Java's CompletableFuture, a class representing a future result of an asynchronous computation, is an advanced iteration of the Future interface. It is used to optimally manage asynchronous computations, making it a robust tool for crafting non-blocking code. This code operates by running tasks on a separate thread while the main work continues uninterrupted until the results from the auxiliary thread are ready. As such, it represents a significant advancement in managing and controlling asynchronous operations in Java.
The key to CompletableFuture lies in its facilitation of asynchronous programming in Java. This asynchronous paradigm allows for non-blocking code execution, where tasks are distributed across separate threads. A significant advantage of CompletableFutures is their ability to notify the main thread about the progress, completion, or even failure of a task.
CompletableFutures can be manually completed using the complete
method, a feature absent in Futures. This characteristic, coupled with capabilities such as callback functions, chaining, and exception handling, allows CompletableFutures to effectively address the limitations of Futures.
CompletableFutures, which implement both the Future and CompletionStage interfaces, extend the functionality of these interfaces by providing convenience methods for creating, chaining, and combining multiple futures. The creation of a CompletableFuture is facilitated through the CompletableFuture
constructor, while asynchronous tasks can be executed using the runAsync
method. If a background task yields a result, it can be run using the supplyAsync
method.
Callback functions can be attached to CompletableFutures through methods such as thenApply
, thenAccept
, and thenRun
. These callback functions can be executed asynchronously using thenApplyAsync
, thenAcceptAsync
, and thenRunAsync
methods. Additionally, CompletableFutures can be combined using thenCompose
and thenCombine
methods.
In situations where multiple CompletableFutures need to be combined, methods such as allOf
and anyOf
come into play. Exception handling, a vital aspect of robust software, is addressed in CompletableFutures using the exceptionally
method. This method allows a function to be invoked if an exception occurs during the computation, taking the exception as an argument and returning a value that will serve as the result of the CompletableFuture.
Overall, CompletableFutures provide a comprehensive API for asynchronous programming in Java, making them an invaluable tool for software engineers. As a result, they offer a more modern and streamlined approach to asynchronous programming in Java, with better readability, composability, and error handling compared to other options. However, the choice of which option to use ultimately depends on the specific requirements and constraints of the application
2. The Importance of Unit Testing CompletableFuture
Unit testing is a crucial part of the software development lifecycle, particularly when it comes to asynchronous programming in Java using CompletableFuture.
The role of unit testing extends beyond a mere procedural task; it serves as an integral part of the development cycle, ensuring the robustness and reliability of the software under development.
Testing for CompletableFuture specifically aims to ascertain that asynchronous tasks function as anticipated. These tests are designed to verify proper task execution and alignment of the produced results with expectations. The importance of these tests becomes more pronounced in the context of CompletableFuture, as they provide a means to validate the correct execution of asynchronous tasks.
The benefits of unit tests are manifold. They enable developers to detect bugs and issues early in the development cycle, thereby significantly reducing the cost and effort required to fix them later. Early identification of issues not only conserves time and resources, but also contributes to a more stable and reliable software product.
Moreover, unit tests for CompletableFuture play a pivotal role in ensuring that the code adheres to its design and functions as intended. It's one thing to design and code a software product, but ensuring that it behaves as expected is where the real challenge lies. Unit tests help in overcoming this challenge by providing a means to verify the intended behavior of the code.
In essence, unit tests for CompletableFuture contribute significantly to enhancing the overall quality of the software. They provide a means to ensure that the software is not just functional but also reliable and robust. By ensuring the correct execution of asynchronous tasks and the production of expected results, these tests play a crucial role in the development of high-quality software solutions.
In the realm of asynchronous programming with CompletableFuture in Java, the importance of unit testing cannot be overstated. It is a critical aspect that ensures the robustness, reliability, and quality of the software being developed.
To write effective unit tests for CompletableFuture, a few best practices can be followed. Ensuring that your unit tests are deterministic and reliable is crucial. This means that the test results should be consistent every time the test is run, regardless of external factors. Methods such as CompletableFuture.join()
or CompletableFuture.get()
can be used to wait for the completion of the CompletableFuture and obtain its result.
Handling exceptions and error cases in your unit tests is another important aspect. CompletableFuture provides methods such as CompletableFuture.exceptionally()
or CompletableFuture.handle()
that allow you to handle exceptions or errors that occur during the execution of the CompletableFuture. These methods can be used in your unit tests to verify that the CompletableFuture behaves as expected in error scenarios.
Furthermore, methods such as CompletableFuture.thenCompose()
or CompletableFuture.thenCombine()
can be used to chain multiple CompletableFutures together. This allows you to test complex scenarios where multiple asynchronous operations are combined. By testing these scenarios, you can ensure that the CompletableFuture behaves correctly in different situations.
Unit tests for CompletableFuture can be written to test various scenarios and functionalities. For instance, you can create a test for successful completion, exceptional completion, combining multiple CompletableFutures, and handling timeouts. These tests can help ensure that the CompletableFuture behaves correctly under different circumstances.
Avoiding common pitfalls while unit testing CompletableFuture can ensure the reliability and accuracy of your tests. These include proper handling of exceptions, testing for completion, proper use of the get()
method, and not relying on specific timing in unit tests.
In summary, unit tests for CompletableFuture play a key role in software development, ensuring the robustness, reliability, and quality of the software. By following best practices and avoiding common pitfalls, you can write effective unit tests for CompletableFuture, contributing to the development of high-quality software solutions
3. Strategies for Effective Unit Testing with CompletableFuture
The process of unit testing CompletableFuture in Java is multifaceted and requires a strategic approach. One strategy is to test the functionality of CompletableFuture tasks independently from their asynchronous behavior. To achieve this, tasks can be isolated in unit tests to allow for more precise and focused testing.
Isolating CompletableFuture tasks can be accomplished by employing a mocking framework like Mockito, which enables the creation of mock implementations of the CompletableFuture class and the specification of the desired behavior for each task. This approach allows control over how tasks complete, what values they return, and any exceptions they should throw. It's also possible to use CompletableFuture's own methods for controlling task execution, such as complete
, completeExceptionally
, and cancel
. By manually invoking these methods on the CompletableFuture instances in unit tests, different scenarios can be simulated, verifying the behavior of your code under different conditions.
Another aspect to consider when testing CompletableFuture is the proper handling of exceptions within the tests. CompletableFuture provides the exceptionally()
method for this purpose, which handles exceptions and returns a new CompletableFuture that has already been completed exceptionally. This method allows for consistent handling of exceptions in line with the asynchronous nature of CompletableFuture. When testing scenarios where exceptions are expected, it's possible to use the exceptionally()
method to define a fallback action that verifies the expected exception is thrown, thus asserting the correctness of the code.
One of the most beneficial strategies for unit testing CompletableFuture is the use of timeouts. Timeouts prevent tests from waiting indefinitely for a task to complete, a common issue when testing asynchronous code. To add timeouts to CompletableFuture unit tests, the CompletableFuture.orTimeout()
method can be used. This method specifies a timeout duration, after which the CompletableFuture will complete exceptionally with a TimeoutException
. This approach ensures that unit tests do not hang indefinitely and fail gracefully if a timeout occurs.
The implementation of these strategies can be improved by using certain tools and techniques. Test doubles, such as fakes or mocks, can be used to create deterministic tests and eliminate uncertainties. This approach allows for testing specific scenarios that may be difficult to replicate in a live environment. Additionally, embracing the inherent uncertainty of testing asynchronous code can be beneficial. This can involve testing in an environment that mirrors the production environment, exposing the implementation to a wider range of scenarios and providing greater confidence in the robustness of the code.
Overall, effective unit testing with CompletableFuture in Java involves a combination of strategies that focus on isolating task functionality, handling exceptions, and using timeouts. By incorporating these strategies and using appropriate testing tools and techniques, a thorough and effective testing process can be ensured
4. Mocking Dependencies in CompletableFuture Unit Tests
The effectiveness of unit testing often hinges on isolating code units, which necessitates the creation of mock dependencies. This becomes more complex when dealing with CompletableFuture due to its asynchronous nature. However, with the aid of tools like Mockito, it's possible to create mock methods that return a CompletableFuture, allowing for the simulation of various scenarios and the testing of how CompletableFuture tasks handle them.
One way to mock CompletableFuture with Mockito is by using the CompletableFuture.mock()
method provided by the Mockito framework. This method allows for the creation of a mock instance of the CompletableFuture class, which can then be used to define the behavior of the mock object. Once you have created the mock CompletableFuture object, you can use Mockito's when()
method to specify the behavior of the mock object when certain methods are called. You can also use Mockito's thenReturn()
method to specify the return value of the mock object's methods.
In addition to using Mockito to create mock objects for the dependencies of the CompletableFuture and define the desired behavior for each method call, you can isolate the CompletableFuture and test its functionality without relying on the actual implementation of the dependencies. This allows for the creation of controlled test scenarios and verification of the expected behavior of the CompletableFuture object.
While using mocks in testing can be complicated, it's important to remember the concept of using an adapter. Code should not directly rely on a third-party API, but should depend on that API through an adapter. This strategy simplifies the code and makes it easier to write tests.
The use of adapters enables the separation of concerns into separate specialized objects, making the design simpler. Writing tests with mocks can be complicated and low value. Mocking static methods in Java requires a specialized library like PowerMockito. Mocking the external API directly leads to mixed business logic and API calls, making the code difficult to test. Instead of mocking the external API, it is better to mock your own adapter.
Focused integration tests are more effective than broad integration tests because they can be made small, precise, and relatively fast. Focused integration tests for an adapter that sends email messages can be done by pointing it to a test SMTP server and checking if the message is received. These tests become even more important when targeting a fast-changing dependency. External APIs are best used through the medium of an adapter. Mocks are a useful way to design adapters.
In sum, the adapter pattern simplifies the design by separating concerns into separate specialized objects. There are alternative ways to solve the dependencies problem, such as using contract tests or the logic sandwich pattern. The Ultratestable coding style and the object theatre pattern are similar patterns to the logic sandwich pattern. The birthday greetings kata is a small exercise that explores refactoring and the "ports and adapters" architecture
5. Handling Exceptional Cases in CompletableFuture Tests
When working with CompletableFuture in Java, especially in unit testing, handling exception scenarios is a key aspect to consider. The CompletableFuture API provides several methods, including exceptionally()
, handle()
, and whenComplete()
, which can be utilized in tests to simulate exceptional cases and validate the correctness of CompletableFuture task handling in such situations[^0^][^1^][^2^][^3^].
Moreover, it's crucial to test the code for both checked and unchecked exceptions to strengthen its resilience[^4^]. This can be achieved using the handle()
method in a CompletableFuture
, which allows you to catch and assert both types of exceptions[^4^].
However, a closer look at the CompletableFuture API reveals an issue with the CompletableFuture.applyToEither
method. This method is intended to apply a function to the value of the first CompletableFuture that completes normally. But, when exceptions are thrown, it has been found to lack proper exception handling[^5^].
Consider a scenario where the CompletableFuture.applyToEither
method is used with two futures. Ideally, swapping the futures should yield the same result. However, when exceptions are involved, the method does not function as expected. The exception propagates to the joint future, causing an unexpected halt despite the completion of the other future[^5^].
To navigate this issue, a solution is proposed. The idea is to create a new method that instigates a race between two completions, using the CompletableFuture.allOf
method. This method ensures all futures are completed, even if they completed exceptionally[^5^]. The approach is exemplified with code samples, which are available on GitHub.
While the CompletableFuture API provides multiple methods for handling exceptions, it's vital to be aware of the limitations of methods like CompletableFuture.applyToEither
. This method has been found to inadequately handle exceptions, making it largely unsuitable for production[^5^]. Implementing a utility method that initiates a race between two completions can be a practical solution to this problem. This approach ensures all futures are completed, thus providing a robust and reliable mechanism for exception handling in CompletableFuture tasks[^5^]
6. Testing Asynchronous Behavior with CompletableFuture
Dealing with asynchronous operations in Java, particularly utilizing CompletableFuture, can indeed be challenging due to their unpredictable nature. However, there are numerous strategies that can effectively manage the execution of CompletableFuture tasks within a testing environment.
The use of Countdown Latches, for instance, can control the execution of CompletableFuture tasks. This can be achieved by using CompletableFuture methods like thenRun
, thenAccept
, thenApply
, etc. These methods allow you to specify actions that should be executed after the CompletableFuture completes. By creating a CountdownLatch with an initial count of 1, and calling latch.countDown()
after the action is completed in the CompletableFuture, the latch is released only after the action is completed. latch.await()
can then be used to wait for the CompletableFuture to complete before proceeding with the next steps in your code. This allows you to control the flow of execution and synchronize different parts of your code.
Testing CompletableFutures using CyclicBarriers is another strategy. This can be achieved using the CompletableFuture.thenAcceptBothAsync() method along with a CyclicBarrier. By creating the CompletableFutures that you want to combine and a CyclicBarrier with the desired number of parties, each CompletableFuture will call the CyclicBarrier.await() method before completing. This ensures that all CompletableFutures reach a point where they can be combined.
One of the key features of CompletableFuture is the thenAccept() method, which allows for assertions within asynchronous tasks, thereby ensuring the accuracy of the results. By using methods such as thenApply
, thenAccept
, and thenRun
, you can chain together multiple CompletableFuture instances to create a pipeline of asynchronous tasks. Additionally, methods like join() or get() can be used to wait for the completion of the CompletableFuture and retrieve its result.
To perform unit testing on CompletableFutures in Java, frameworks like Mockito and JUnit can be used. Mockito allows you to mock dependencies and simulate the behavior of external components. JUnit provides annotations and assertions for writing unit tests. By creating a mock object for any dependencies and defining the expected behavior using Mockito, you can use JUnit to write test cases that verify the expected behavior of the CompletableFuture.
The default executor for CompletableFuture is the ForkJoinPool, which can create a new thread for each task if the parallelism level is set to 1. This parallelism level can be configured using system properties or environment variables, and a lower level can be beneficial for short tasks.
In environments like Kubernetes, CPU shares and CPU quotas play a significant role. CPU shares determine the amount of CPU resources allocated to a pod, while CPU quotas limit the CPU usage of a process within a cgroup. The use of CPU shares and CPU quotas can be a critical factor in the performance of CompletableFuture tasks.
Asynchronous programming allows for the simultaneous execution of multiple tasks, leading to improved performance and task decoupling. However, debugging and testing can be more intricate in an asynchronous context.
In conclusion, testing the asynchronous behavior of CompletableFuture is a complex task that requires a deep understanding of CompletableFuture and asynchronous programming. However, with the right strategies and understanding, it is possible to effectively test and validate the asynchronous behavior of CompletableFuture tasks
7. Implementing Robust and Flexible Testing Frameworks for CompletableFuture
Asynchronous programming in Java, facilitated by the CompletableFuture class, necessitates a flexible and well-structured testing environment. CompletableFuture, a significant upgrade to Java's Future API, enables parallel and non-blocking code execution in separate threads, thereby enhancing performance.
JUnit, a widely-adopted Java unit testing framework, is instrumental in this testing process. Coupled with Mockito, it enables the simulation of dependencies, effectively mocking them to create a controlled testing environment.
A thorough understanding of the CompletableFuture API is essential for effective testing. Functions such as join(), get(), and getNow() are critical to the testing process. These methods offer diverse ways to retrieve computation results, enabling comprehensive testing of various scenarios.
The join() method is similar to the get() method but without throwing checked exceptions. It waits for the CompletableFuture's completion and delivers the result when available. If the CompletableFuture completes exceptionally, the join() method throws an unchecked exception. This method is particularly useful in testing scenarios where you need to wait for a CompletableFuture's completion and retrieve its result.
The get() method is used to retrieve a CompletableFuture's result. It blocks the current thread until the CompletableFuture completes and returns the result. However, using the get() method to block the current thread undermines the purpose of using CompletableFuture for asynchronous programming, which is to perform computations asynchronously and efficiently utilize available resources.
The CompletableFuture API provides methods for creating, chaining, and combining multiple futures. These methods are useful for manually completing a future or executing tasks asynchronously. Functions such as runAsync and supplyAsync are used to run tasks, with the latter returning a result upon completion.
In addition, CompletableFuture allows attaching callbacks using methods such as thenApply, thenAccept, and thenRun. These methods enable further actions on the future's result without blocking. The API also provides methods for combining dependent futures using thenCompose and independent futures using thenCombine. It also allows for combining multiple futures with allOf and anyOf methods. Exception handling is catered for with the exceptionally and handle methods.
The use of assertion libraries like AssertJ or Hamcrest is recommended. These libraries increase the readability of assertions in the tests, making them more expressive and intuitive. For example, Hamcrest provides a wide range of matchers that can be used to assert various conditions on the values returned by the CompletableFuture.
Real-world examples and case studies provide insights into the functionality and application of CompletableFuture. For instance, the use of CompletableFuture in a framework to avoid external dependencies is a practical application. The default CompletableFuture pool's behavior of creating a new thread for each task, and the use of the ForkJoinPool and its parallelism level based on available processors, are examples of how CompletableFuture operates in real-world scenarios.
Lastly, the configuration of CPU resources in a Kubernetes deployment, including CPU shares and CPU quotas, and the JVM's option to prefer CPU shares over CPU quotas, illustrate the complex interplay between system resources and JVM ergonomics. These examples underscore the importance of understanding the entire system environment when implementing robust and flexible testing frameworks for CompletableFuture
8. Balancing Workload and Deadlines in the Unit Testing Process
In the realm of software development, the challenge of juggling between workload and deadlines while performing unit testing is a prevalent issue. The key to overcoming this hurdle lies in the strategic prioritization of tests based on the complexity and criticality of the code. High-risk areas within the code should be the primary focus of testing.
To save time and reduce manual effort, the unit testing process can be automated. For instance, Machinet, a platform known for providing valuable resources related to software testing and development, can be employed to generate unit tests automatically. This not only accelerates the software development process but also enhances the quality of the code.
However, even with automation in place, one must be wary of test flakiness, a common challenge in automated testing. This can lead to false positives and negatives, rendering the test results unreliable. To navigate this issue, developers can resort to mutation testing, a method where the source code is tweaked to ensure the tests can detect these changes.
The significance of testing in the contemporary software development landscape is growing by the day. Consequently, it's imperative to ensure that the tests are executed swiftly. Slow test times can hinder the development process and potentially lead to code rot, a state where the code turns obsolete or unusable. As tests take longer to run, they are executed less frequently, which can further exacerbate the issue of code rot. Thus, maintaining fast test times is not merely a requirement, but a design challenge.
The FitNesse project is a stellar example of a system that boasts fast test times. Developers can adopt strategies like decoupling architectures and stubbing to maintain swift test times. Stubbing out slow components in tests can dramatically reduce test times and boost efficiency. Over-testing slow components can result in unnecessary time expenditure, hence, it's crucial for developers to prioritize fast test times to ensure efficient development.
A pertinent quote from the context underscores the importance of speed in testing, stating, "Fast tests need to run fast." To uphold this, it's crucial to use a unit testing tool that doesn't decelerate the tests. If a tool is slowing down the tests, it might be time to consider a new tool. Another quote from the context underlines that "Keeping the tests running very fast is a design challenge." Therefore, it's not just about having the right tool, but also about designing the tests in a way that they run quickly.
Ultimately, striking a balance between workload and deadlines in the unit testing process is a critical aspect of software development. It calls for meticulous planning, efficient design, and the right tools to ensure that the tests are executed swiftly and effectively. It's also crucial for developers to stay updated with the latest trends and challenges in automated testing to ensure the development of high-quality software
Conclusion
CompletableFuture in Java is a powerful class that revolutionizes asynchronous programming and non-blocking code execution. It offers significant advantages over the traditional Future interface, providing robust tools for managing and controlling asynchronous operations. With features like callback functions, chaining, and exception handling, CompletableFuture addresses the limitations of Futures and offers a more modern and streamlined approach to asynchronous programming in Java.
The functionalities and benefits of CompletableFuture discussed in this article demonstrate its importance in enhancing the efficiency, reliability, and quality of software solutions. By utilizing CompletableFuture, developers can leverage its capabilities to optimize performance, improve code readability, compose complex tasks, and handle exceptions effectively. Additionally, the strategies for effective unit testing with CompletableFuture highlight the crucial role of testing in ensuring the robustness and reliability of software. Unit tests allow developers to detect bugs early in the development cycle, reduce costs of fixing issues later on, validate correct task execution, and ensure proper exception handling.
To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation. Visit Machinet today
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.