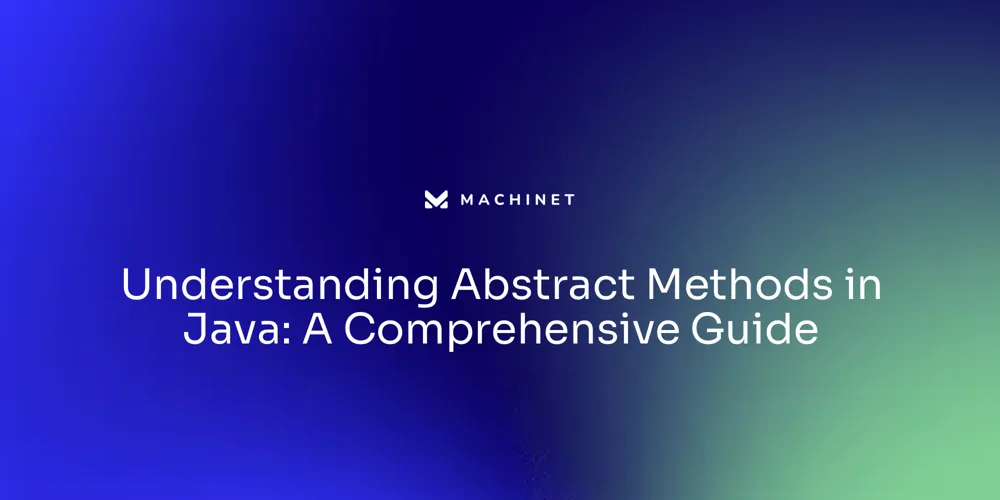
Table of Contents
- What are Abstract Methods?
- Declaring Abstract Methods
- Implementing Abstract Methods in Subclasses
- Using Abstract Methods in Real-World Scenarios
Introduction
Abstract methods play a crucial role in Java's object-oriented approach, providing a blueprint for methods that subclasses must implement. They are declared within an abstract class or an interface and serve as a clear contract for defining behaviors.
This article explores the concept of abstract methods, their declaration, implementation in subclasses, and their real-world applications. By understanding abstract methods, developers can enhance the readability, maintainability, and efficiency of their Java code.
What are Abstract Methods?
In Java, abstract methods are the blueprint for methods that subclasses must implement. They are declared within an abstract class or an interface, and they do not have a body; instead, they provide a signature that the inheriting classes must define.
This concept is pivotal for Java's object-oriented approach, promoting a clear contract for what behaviors a subclass should have. For instance, in the context of the Java Development Kit 23 (JDK), the introduction of the Vector API and the preview of primitive types in patterns, such as instanceof and switch, have been notable enhancements.
These features, which build upon the abstract methods' foundation, facilitate more efficient vector computations and pattern matching, leading to more readable and intuitive code. One of the overarching goals in evolving Java is ease of learning and use.
Enhancements like instance main methods, which do not require the static keyword or public visibility, make the language more accessible to novices. Meanwhile, the Foreign Function & Memory API introduces restricted methods, which necessitate specific command-line options to access due to their inherent risks.
These advancements underscore Java's commitment to safety and control while interacting with native functions and data. The significance of writing clean, maintainable Java code cannot be overstated. As one expert encapsulates, clean Java code is 'expressive, concise, well-organized, and easy to understand.' It is essential for the longevity of software projects and facilitates collaboration among developers. The Single Responsibility Principle (SRP) is a key tenet in this regard, ensuring that methods have a singular focus and do not become overly complex. By embracing these principles, developers can craft Java code that is not just functional, but also a joy to work with and maintain.
Declaring Abstract Methods
In Java, when you want to create a method that is declared but not implemented, you use the abstract
keyword in its signature. This signifies that the method does not contain an implementation (a method body) at this point but instead defers the implementation to its subclasses. It ends with a semicolon, indicating the absence of a body.
For instance, abstract void moveTo(double deltaX, double deltaY);
defines an abstract method without any code enclosed in curly braces. Such an approach is particularly useful in scenarios where a method's behavior is intended to vary depending on the subclass, thereby enforcing a contract for the subclasses to provide a concrete implementation. This concept is part of clean code principles, promoting code that is not only error-free but expressive and easy to understand, thus enhancing maintainability and collaboration.
Pattern Matching in Java exemplifies how abstract methods can be leveraged for more readable code. By matching a value against a pattern, parts of the value are bound to variables within the pattern, enabling operations on complex data structures with clarity and conciseness. The introduction of the Vector API in JDK 23 demonstrates the ongoing evolution of Java, as it incorporates new ways to express computations efficiently, which can be seen as an extension of the principles behind abstract methods and clean codeβwriting code that clearly communicates its intent and operates optimally across various CPU architectures.
Implementing Abstract Methods in Subclasses
In Java development, adherence to the Single Responsibility Principle (SRP) is critical, as it ensures that each class and method handles a single aspect of the functionality. For instance, in an application designed to connect doctors with patients, the Practitioner
and Event
models should encapsulate distinct responsibilities. The Practitioner
model would manage doctor-related information, while the Event
model would handle scheduling details.
When such classes implement an interface or extend an abstract class, they must conform to the contract by providing concrete implementations for all abstract methods. This is akin to defining a 'strategy' in design patterns where each strategy must implement the interface's methods in its own way. The beauty of this approach is its maintainability; introducing a new strategy or subclass doesn't require changes to existing code, as long as it fulfills the interface contract.
The purpose of abstract methods is to set a common API for derived classes, much like a game of chess where the rules are fixed but the game play is dynamic. Abstract methods act as a promise that subclasses will provide specific functionalities. In Java, methods are either predefined, available in the Class Library, or user-defined, written to perform specific tasks.
Instance methods, in particular, have access to the class's instance variables and can manipulate the object's state. Overriding a method in a subclass changes the behavior inherited from the parent class, providing a new implementation. As a result, when a class fails to implement all abstract methods from its parent class or interface, it leads to a compilation error, ensuring that the class adheres to the predefined structure and behavior expected of it.
Using Abstract Methods in Real-World Scenarios
Abstract methods in Java serve as a blueprint for subclasses to follow, ensuring that each subclass provides a specific implementation of the method. Take, for example, the concept of shapes in a class hierarchy.
Each shape, such as a rectangle or circle, has its own unique formula for calculating its area. By declaring the calculateArea()
method as abstract, we enforce a contract that obliges each subclass to furnish its own specialized method for area calculation, thereby achieving a polymorphic behavior.
This approach aligns with the Single Responsibility Principle, as it assigns a focused task to each method, avoiding complexity and making the code easier to maintain and understand. The introduction of virtual threads, or vthreads, in Java is a testament to the language's evolving concurrency model.
Threads, which are lightweight and managed by the JVM, underscore Java's commitment to innovation and performance. They can be suspended during blocking I/O operations, allowing for more efficient use of system resources.
This advancement is a leap forward from the traditional model that relied solely on platform threads. In the realm of software development, pattern matching and sealed classes have enhanced Java's capability to process data structures with clarity and precision. Pattern matching simplifies code by directly binding parts of a value to variables if the value fits a specified pattern. Meanwhile, sealed classes provide a way to define a restricted hierarchy of classes, which can lead to more robust and maintainable code. Ultimately, a deep understanding of Java's syntax and semantics, including abstract methods and the latest concurrency and data handling features, empowers developers to write cleaner, more efficient, and scalable code.
Conclusion
Abstract methods in Java provide a blueprint for subclasses, enhancing code readability and maintainability. By using the abstract
keyword, developers can define clear contracts for behaviors. Implementing abstract methods in subclasses adheres to the Single Responsibility Principle (SRP), allowing for flexibility without changing existing code.
Real-world scenarios benefit from abstract methods, defining specific functionalities. Java's advancements, like virtual threads and pattern matching, improve concurrency and simplify code. Understanding abstract methods empowers developers to write readable and maintainable Java code, creating efficient and scalable projects.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.