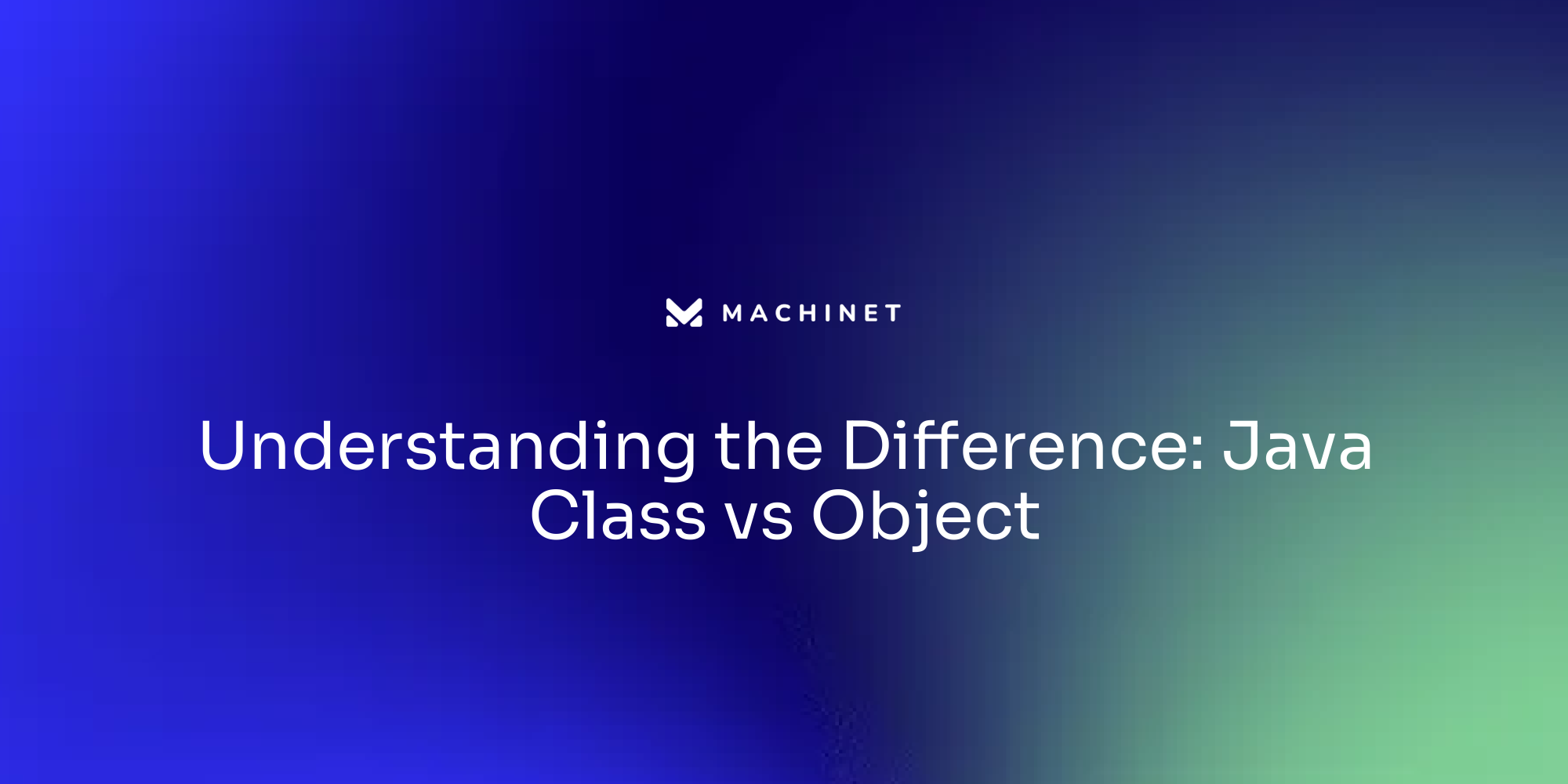
Introduction
Java, with its object-oriented paradigm, provides developers with a powerful toolset to create robust and efficient applications. In this article, we explore the concepts of Java classes and objects and their key differences. We also discuss the process of creating Java classes and objects, as well as how to access their methods and attributes.
Through practical examples, we showcase how classes and objects work together to model real-world entities and build complex applications. Whether you're a beginner or an experienced programmer, understanding Java classes and objects is essential for mastering this versatile language and creating well-structured code. So let's dive in and explore the world of Java classes and objects.
What is a Java Class?
In Java, a structure serves as a fundamental construct that encapsulates data and behavior into a single unit, much like a blueprint for constructing entities. Every category is characterized by particular features and functions that establish the characteristics and capabilities of the entities it generates. For instance, consider a parking lot system application. The class for the parking lot would define attributes such as a unique ID, the number of floors, and slots per floor. Furthermore, it would include methods to allocate slots to different vehicle types like cars, bikes, and trucks. This structure allows for the organized representation of complex real-world entities in a manageable and reusable way.
Classes in programming language X are more than just templates; they are cornerstones of the object-oriented paradigm, embodying the principles of encapsulation, abstraction, and modularity. By adhering to standard naming conventions, developers can create clear and meaningful code, as suggested by industry experts who recommend descriptive names over cryptic acronyms, enhancing readability and maintainability.
With over 26,000 developers surveyed globally, trends show a significant shift towards embracing new technologies and programming paradigms. Despite the advent of more recent programming languages, the industry continues to rely on Java, with tools like IntelliJ IDEA leading the way for Java and Kotlin development. This shows Java's lasting significance and flexibility in the midst of swiftly changing technological environments.
The object-oriented nature of this programming language is not just a theoretical concept but also a practical approach to solving real-world problems. By decomposing intricate systems into manageable classes and entities, developers can systematically address complex requirements, building robust and efficient applications. As this programming language continues to play a crucial role in education and professional development, the community and User Groups (Jugs) worldwide are actively engaging and supporting junior developers in mastering this versatile language.
What is a Java Object?
In the domain of Java, entities are the foundation of any application, embodying real-world elements with their own state and behaviors. When you instantiate a structure, you're essentially creating a construct in memory that houses the attributes and actions that the class defines. Think of a class as a blueprint for a house, and each entity is a house constructed from that blueprint, with its own distinct address and character.
Objects are akin to Lego blocks in the vast structure of a software program, each with a distinct role and capable of interacting with one another. This modular approach is fundamental to Object-Oriented Programming (OOP), which is essential to the language. The four pillars of OOP—abstraction, encapsulation, inheritance, and polymorphism—guide developers in creating objects that represent complex real-world concepts in a manageable and scalable way.
The ongoing development of Java, as highlighted by industry experts such as Brian Goetz, emphasizes its dedication to contemporary development practices. The language's object-oriented nature is supplemented by concepts like Data-Oriented Programming (DOP), encouraging developers to focus on data structuring for clarity and efficiency.
Amidst rapid technological advancements, this programming language has maintained its relevance and continues to be a stronghold in the developer community. As emphasized in industry reports, the technology sector is undergoing exponential growth, and the programming language, Java, is leading the way, adapting to meet the constantly evolving landscape. Tools like IntelliJ IDEA demonstrate the adaptability and lasting appeal of the programming language to both experienced developers and newcomers to the field.
Comprehending entities in the Java programming language involves more than just identifying their structure; it also entails understanding their role within the wider scope of concurrent and asynchronous programming paradigms. Asynchronous requests, for example, are non-blocking operations that allow a thread to continue execution while a request is being processed. This exemplifies the adaptability and effectiveness that the object-oriented programming language can bring to designing applications, guaranteeing quick and efficient response and optimal performance even as the underlying technology environment evolves.
Key Differences Between Java Class and Object
Comprehending the subtleties of programming with Java is vital for effective programming. A blueprint in a programming language is a design that specifies the structure and functionality of a data type, containing the characteristics and capabilities that the instances, also referred to as objects, will possess. When a structure is instantiated, it's similar to a real-world entity being brought into existence with its own distinct condition, determined by the values of its characteristics.
In Java's object-oriented paradigm, a template provides the structure for entities, much like a blueprint for a house, while a tangible manifestation is a concrete realization of this blueprint, akin to a house built from the design. Every entity possesses its individual condition, which pertains to the characteristics or qualities unique to that entity. Even though they are derived from the same category, each entity has the ability to preserve its unique condition regardless of others.
Courses can also have static variables and methods, which belong to the course itself rather than any one entity. Static members are shared across all instances of the category, making them useful for representing properties or behaviors that are common to all things of that category, rather than individual states.
This difference between categories and instances is demonstrated by the ongoing development of the programming language. Almost thirty years since its beginning on May 23, 1995, the programming language has demonstrated its resilience by incorporating new features like var support within methods, improving readability and reducing verbosity in declaring variables. Moreover, improvements such as JEP 458 indicate a commitment to simplifying the developer experience, from small programs to large, complex applications.
As Language Architect at Oracle Brian Goetz expressed in his article on Data-Oriented Programming, it's essential to 'model the data, the whole data, and nothing but the data.' This method complies with object-oriented principles by concentrating on the representation and processing of information as entities, providing a clear and structured approach to manage intricate software development tasks.
When thinking about entities as the essential foundation of a program in Java, one can find similarities with Lego blocks. Each object, or 'Lego piece,' can be used to construct intricate structures (programs) by assembling them in various configurations. This modular approach allows for both flexibility and specificity in software design, enabling developers to tailor their applications to precise requirements while maintaining a structured codebase.
Through object-oriented design, developers are equipped to model real-world entities and concepts within their software, facilitating a methodical and intuitive development process. The Development Kit (JDK) further supports this process by providing the essential tools and libraries for application development, ensuring that programmers have access to the resources they need to bring their object-oriented designs to fruition.
Creating a Java Class
Developing a Java category is a simple process, but following best practices is crucial for clarity and maintainability. Start by stating your category with the 'class' term, followed by a distinct and descriptive title that avoids perplexing acronyms. For example, prefer 'Customer' over 'Ctr' to avoid ambiguity. Inside the category, establish properties for information storage and procedures for functionality. For example, when creating a basic parking lot system, your structure may consist of fields for parking lot ID, number of floors, and slots per floor, with functions designed to manage parking logistics.
Consider the following example of a class that models a basic Java object:
```java public class ParkingLot { private String parkingLotID; private int floors; private int slotsPerFloor;
public ParkingLot(String parkingLotID, int floors, int slotsPerFloor) { this.parkingLotID = parkingLotID; this.floors = floors; this.slotsPerFloor = slotsPerFloor; }
// Additional methods to manage parking operations } ```
When crafting methods, remember the basic syntax that includes an access specifier, return type, method name, and parameters. For example, a method to add a vehicle could look like this:
java
public void addVehicle(String vehicleType) {
// Method body
}
By adhering to these conventions and focusing on clear, descriptive naming, the resulting codebase will be easier to understand and maintain. This approach aligns with the ongoing momentum in the programming community towards simplicity and readability, as emphasized by industry leaders.
Creating a Java Object
Generating a programming element is a clear-cut procedure that includes three essential actions: declaration, instantiation, and initialization.
Firstly, define a variable of your specified class type to prepare the scene for your entity. This is akin to laying the foundation for a building before actual construction begins. Similar to how a strong base is important for a stable structure, a clear declaration is essential for a robust entity.
Next, bring your entity to life by using the 'new' keyword, which serves as the cornerstone of your entity creation. This step is analogous to the actual construction of a building, where materials are assembled to create something functional. In the programming language, the 'new' keyword assigns memory for your instance, effectively building it in the memory area.
If you choose to, you can bring vitality to your entity by invoking the constructor, which initializes the entity with specific values. This is similar to giving a newly constructed building its unique features, such as paint and fixtures, making it ready for use. Constructors in a programming language set the initial state of an instance, adapting it to your particular needs.
To illustrate, consider the following code snippet, which demonstrates the creation of a Java object within the context of a 'Main' class:
java
public class Main {
public static void main(String[] args) {
// Declare and instantiate an object of the MyClass class
MyClass myObject = new MyClass("John", 25);
// Invoke methods on the object
myObject.sayHello();
}
}
This code captures the essence of Java's object-oriented nature, as it creates an instance of 'MyClass' with attributes and behaviors, similar to assigning specific roles and capabilities within a parking lot system. Every element in the programming language can be considered a building block in a bigger software application, similar to a space within a parking area, fulfilling a specific role and collaborating with other elements to accomplish the desired functionality of the entire system.
Comprehending the formation and administration of objects in the programming language is not just crucial for building applications such as parking systems, but also for understanding the wider environment, which is constantly developing and growing. As the technology of programming progresses, it continues to be a preferred language for developers globally because of its durability, safety, and ability to work on multiple platforms. The 'Write Once, Run Anywhere' philosophy ensures that programming applications are versatile and adaptable, mirroring the dynamic needs of modern software development.
Accessing Class Methods and Attributes Through Objects
In Java, the formation of a entity is the initial stage in utilizing the functionalities encapsulated within it. A thing is an occurrence of a category, and once created, it acquires entry to the category's operations and features. This is accomplished through the utilization of the dot operator, which links the entity's name with the desired procedure or characteristic. For example, imagine a class MyClass
with attributes name
and age
, and a method sayHello()
:
java
public class Main {
public static void main(String[] args) {
// Declare and instantiate an object of MyClass
MyClass myObject = new MyClass("John", 25);
// Invoke methods on the object
myObject.sayHello();
// Access and modify object attributes
myObject.name = "Alice";
myObject.age = 30;
// Invoke methods again
myObject.sayHello();
}
}
In this example, myObject
is an object of MyClass
. We change its state by modifying the values of name
and age
. This is reflective of the real-world entities that OOP aims to model, providing both structure and behavior to our code, which is essential for creating modular and reusable software.
It's crucial to comprehend that while the programming language has primitive data types like int
, char
, and double
for simple values, objects enable us to depict intricate entities with state (attributes) and behavior (methods). This differentiation is essential for junior developers exploring the realm of OOP, which serves as a basis for programming and is extensively employed in the business sector, as demonstrated by its widespread use by most Fortune 500 companies.
Additionally, the language continues to be a pivotal language in education, with User Groups (Jugs) worldwide propelling the in Education initiative, aimed at helping new developers and students grasp technology. As the tech sector evolves, the demand for skilled developers in this programming language remains high, with the number of professional developers reaching approximately 13.4 million globally by 2023. This emphasizes the significance of grasping object-oriented principles, as they are at the core of programming in Java and its extensive use in professional software development.
Practical Examples of Java Classes and Objects
Let's explore the realm of Java by analyzing functional examples that mimic real-world entities and demonstrate how objects and their functions collaborate to construct powerful applications.
Consider a ParkingLot structure for a parking lot system application. This category might include attributes such as parkingLotID, floors, and slots, where each slot is designated for a specific vehicle type, such as a car, bike, or truck. Methods might include parkVehicle() and removeVehicle(), allowing each ParkingLot to manage its own state and behavior.
Envision a Vehicle category, which could portray the different kinds of vehicles that use the parking lot. Attributes might include make, model, and vehicle Type, while behaviors might entail turn On() and turn Off(). This grouping of properties and functions into entities, such as the Vehicle, aids in handling intricacy and encourages a well-organized coding approach.
Lastly, take the User class, representing a user of the parking lot system, with attributes such as userID, name, and vehicleOwned. Here, methods could include check In() and checkOut(), individualizing each User's interactions within the system.
This modeling reflects the essence of object-oriented programming, where objects—like building blocks in a structure—serve as the foundation for applications. These examples underscore the importance of clear naming conventions, as seen in the JavaBeans naming convention, and the encapsulation of data and behaviors within objects, a principle that has been integral to the programming language's design since its inception by James Gosling. As Java continues to evolve, features like 'var' support demonstrate its adaptability, ensuring that the language remains relevant and user-friendly for developers.
Conclusion
In conclusion, Java classes and objects are fundamental elements in the object-oriented paradigm. Classes serve as blueprints, encapsulating data and behavior, while objects are instances of classes that embody real-world entities with their own state and behaviors.
Understanding the differences between classes and objects is crucial. Classes define structure and behavior, while objects have unique states. Classes can have static variables and methods, while objects maintain independent states.
Creating Java classes involves declaring, defining, and following best practices. Creating Java objects involves declaring, instantiating, and optionally initializing.
Accessing class methods and attributes through objects is done using the dot operator. Objects gain access to functionalities once instantiated, allowing for attribute manipulation and method invocation.
Practical examples demonstrate how classes and objects work together to model real-world entities. Examples like ParkingLot, Vehicle, and User classes showcase encapsulation and promote clean coding.
Understanding Java classes and objects is essential for mastering the versatile language. By leveraging their power, developers can model complex systems, create reusable components, and adapt to the changing technological landscape.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.