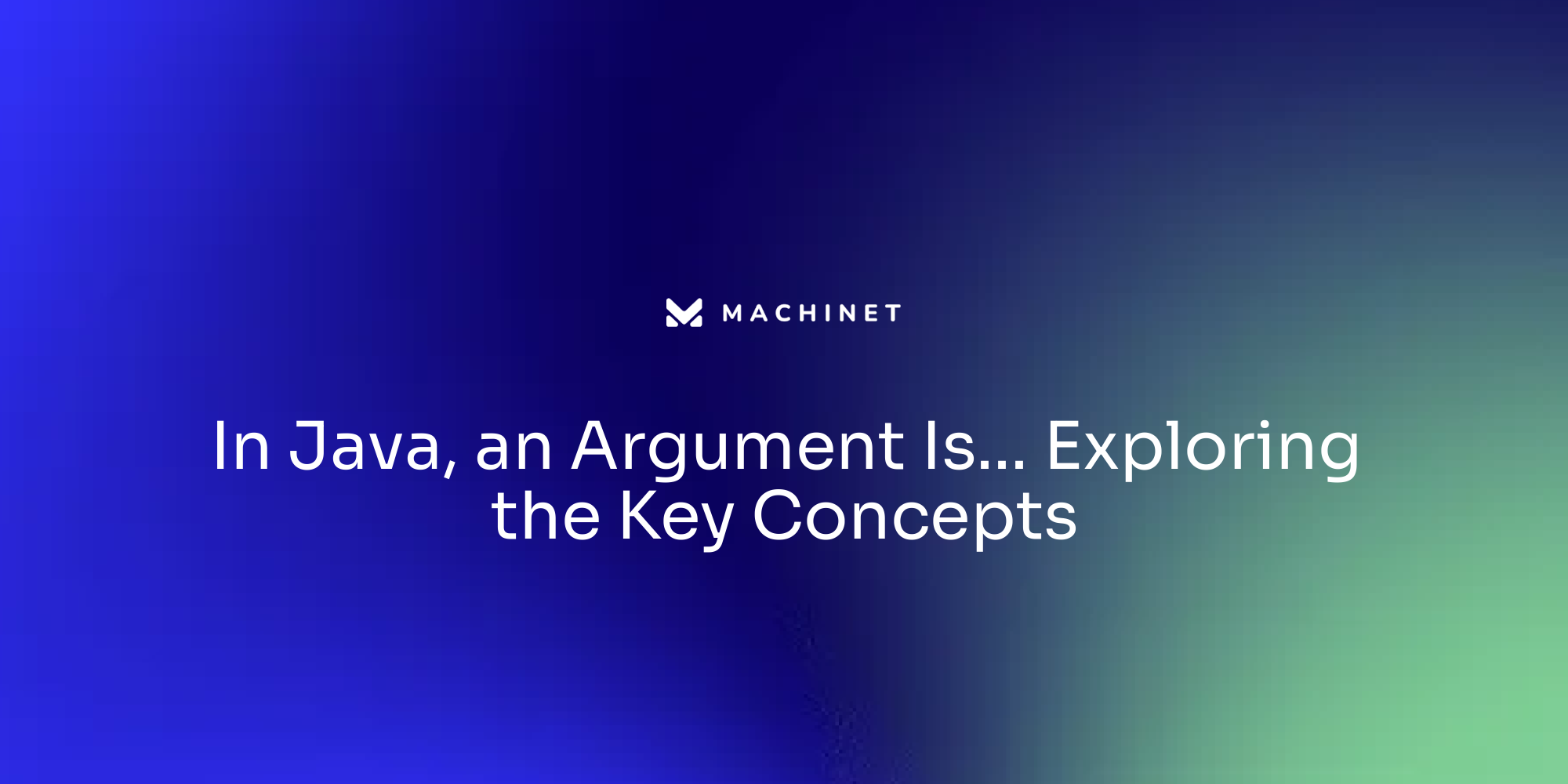
Introduction
Java's method invocation relies on the proper understanding and usage of arguments and parameters. While arguments are the actual values passed into a method, parameters act as placeholders within the method's definition. This distinction is crucial in Java's approach to synchronous and asynchronous operations, allowing developers to optimize for different execution contexts.
In this article, we will explore the difference between arguments and parameters, how to effectively use arguments in Java methods, working with multiple parameters, and the use of varargs and command line arguments. By grasping these concepts, Java developers can enhance their code's clarity, maintainability, and performance. So let's dive into the world of Java method invocation and discover the power of arguments and parameters in building robust and efficient applications.
Understanding the Difference Between Arguments and Parameters
The successful execution of Java's function call depends on the correct utilization of parameters and inputs. A parameter is the real value that you provide to a function when you invoke it. For example, if you possess a function that computes the total of two numbers, the numbers themselves serve as the parameters. In contrast, a parameter is similar to a placeholder within the definition of a function; it is the variable that receives the value of the input and is utilized within the function to carry out its operations.
Consider a scenario where a function processes a request. If the request is synchronous, the calling thread will wait for the response before moving on to the next line of code. This is like giving an argument to a function and waiting for the function to give back a result before continuing. On the other hand, with an asynchronous request, the calling thread continues with other tasks while the request is being processed, akin to a non-blocking function call that allows for concurrent task execution.
The robustness and adaptability of the programming language over the past three decades have seen it evolve from a simple language to a complex ecosystem, accommodating both native development and a multitude of frameworks. This adaptability extends to the language's invocation mechanisms, which support both synchronous and asynchronous operations, providing developers with the flexibility to optimize for different execution contexts. Understanding the approach of Java to arguments and parameters is crucial, highlighting the language's dedication to performance and developer productivity.
How to Use Arguments in Java Methods
To effectively use inputs in methods written in Java, it's crucial to align them with the specified parameters of the function. For example, consider an application developed using the Java programming language for a parking lot system, where each parking slot is designated for a specific vehicle type. When invoking a method in a programming language like Java that assigns a vehicle to a parking slot, the values passed should match the necessary inputs, including the parking lot ID and the type of vehicle. The provided inputs can assume different forms, such as literals, variables, or more complex expressions. By ensuring the argument types align with the argument types, developers can maintain clarity in their code, as emphasized by the widely-accepted naming convention.
As we navigate through the rich history of this programming language, from its inception as 'Oak' nearly 30 years ago to its present-day status as a foundational software language, understanding these nuances becomes even more crucial. Arguments are not only values passed to functions but must also be suitable with the argument types, supporting the language's ability to adjust and stay significant amidst the introduction of newer programming languages. By understanding these important differences, developers can write code that is easier to debug, maintain, and extend, achieving the ultimate objective of code: to be comprehended and maintained.
Working with Multiple Parameters in Java Methods
In the domain of programming language, creating functions with numerous arguments is similar to selecting the appropriate means of transportation for a shipment considering the size of the package and the distance to be covered. Similar to a truck being chosen for a significant quantity delivery to efficiently maneuver through urban areas, in Java, we establish function designations with the suitable types and names for each input to manage different intricacies of tasks. When calling these procedures, it's crucial to provide arguments that correspond with the procedure signature, both in type and sequence. This precision ensures that the code operates as intended, much like how using the correct mode of transportation ensures a package reaches its destination efficiently.
For example, in order to perform tasks simultaneously, such as handling asynchronous requests, it is essential to organize your method to accept multiple inputs that correspond to the different aspects of the task. This could involve inputs for the data to be processed, callbacks for task completion, and error handlers. Adhering to standard naming conventions, such as those suggested by JavaBeans, further clarifies the purpose of each parameter, making the code more intuitive to read and maintain. As the programming language keeps progressing, adopting straightforward, lucid, and descriptive code remains a fundamental aspect of sound development methodologies, guaranteeing the codebase is reachable and controllable.
Using Varargs (Variable Arguments) in Java
The evolution of this programming language over nearly three decades has seen it incorporate features that ease development while maintaining its core principles. The introduction of variable parameters (varargs) with Java 5 is one such feature that exemplifies this balance. Varargs enhance the language's functionality by allowing developers to pass an arbitrary number of parameters to a function, thereby improving code flexibility and readability. This is accomplished using the ellipsis (...) syntax following the parameter's type, indicating that the function can accept a range of values without having to specify the exact number in advance.
The implementation of the var
keyword, a more recent update, reflects Java's continuous modernization. While the programming language is traditionally known for its strong typing, var
introduces a degree of type inference within methods. This means developers can declare variables without specifying their types, and the compiler deduces the type based on the context. This feature has been embraced by the community for its ability to streamline code and reduce verbosity, further simplifying the lives of developers.
'The tooling for this programming language has also kept pace, with improvements aimed at facilitating a smoother development process.'. JEP 458 is one such initiative that aims to enhance the application launcher, allowing programs to be run from multiple source code files. This incremental approach to building applications serves to ease the transition from small to larger projects, recognizing the varied needs of developers at different stages of application complexity.
In accordance with these advancements, it is noteworthy that the language continues to be favored by developers, not only due to its strength but also due to its compatibility with other widely used languages and technologies like JavaScript, SQL, Python, and HTML/CSS. This interoperability is crucial for developers who often work across various platforms and frameworks to create diverse software applications.
Command Line Arguments in Java: Basics and Examples
While running a Java application, you can provide command line inputs to customize its behavior or supply required data. This feature is similar to preparing the stage before a performance, where each input provided on the command line serves as a distinctive prompt for the application to proceed. For example, when creating a parking lot system, command line parameters could define a distinct parking lot identification, the amount of levels, or even the distribution of parking spaces by vehicle category, such as automobile, bicycle, or truck.
Passing values through the command line is not only about transferring data; it also aligns with the main aspects of building a Command Line Interface (CLI) such as user-friendliness, uniformity, and verification. Developers aim for a CLI that is intuitive for users and guards against errors by validating inputs. This is evident in the Apache Commons CLI library, which offers robust tools for defining and processing command line options, ensuring that applications like parking lot systems are both flexible and user-friendly.
Additionally, command line parameters are a component of the diverse history of Java's development. Celebrating nearly three decades since its inception, Java has adapted to the ever-changing landscape of software development, maintaining its core tenets while integrating new features to stay relevant.
In practice, utilizing command line parameters efficiently can save developers time and prevent unintended actionsβa concept paralleled in Linux commands, where the selection of exiting with ':x' versus ':wq' in vi or vim editors can influence file timestamps based on recent edits. This level of control and precision is what makes command line parameters a powerful asset in a developer's toolkit. As we continue to explore the programming language, from its native capabilities to the various frameworks that enhance its ecosystem, the ability to utilize command line arguments remains a fundamental skill for creating efficient and responsive applications.
Practical Examples: Passing Arguments to Java Methods
While exploring the extensive ecosystem of Java, we come across different approaches and their distinctive styles, ranging from those that don't necessitate any inputs to those that can handle a wide range of intricate types. The structure of a programming procedure typically encompasses an access specifier, indicating the visibility within the program, a return type that denotes the data type of the output, the method name that follows Java naming conventions, and parameters that the method can accept to influence its behavior. For instance:
java
public int multiply(int a, int b) {
return a * b;
}
This example demonstrates a method that accepts two integers and returns their product. The method's simplicity belies the depth and flexibility inherent in the design language, which has underpinned its sustained relevance in the software industry for nearly three decades.
As the programming language has matured, it has retained its core functionality while continuously integrating new features that maintain its modernity. This evolution demonstrates the programming language's capacity to adjust to the constantly shifting software development environment. Whether dealing with legacy code or the latest framework, understanding how to effectively use the parameters of Java's methods is fundamental to creating robust and efficient applications.
In the context of function parameters, the adaptability of the programming language is further demonstrated by the notion of varargs, which enable programmers to transmit a variable number of values to a function, enhancing versatility. Moreover, command line arguments offer a way to interact with a program during its execution, providing dynamic input that can lead to more versatile applications. By delving into these examples and internalizing the principles of Java's method architecture, you equip yourself with the knowledge to leverage Java's strengths in various development scenarios, from the granular level of individual methods to the broader context of entire software systems.
Conclusion
In conclusion, understanding the difference between arguments and parameters is crucial in Java method invocation. Arguments are the values passed into a method, while parameters act as placeholders within the method's definition. This distinction allows for optimizing synchronous and asynchronous operations.
To effectively use arguments in Java methods, developers must match them with the defined parameters, ensuring their types align. This improves code clarity and maintainability.
Working with multiple parameters in Java methods is akin to selecting the right vehicle for a delivery based on size and distance. Defining method signatures with appropriate types and names ensures code operates as intended. Adhering to naming conventions enhances code readability and maintainability.
The introduction of varargs in Java 5 allows passing an arbitrary number of arguments to a method, improving code flexibility and readability. The var
keyword simplifies code and reduces verbosity, streamlining development.
Command line arguments in Java tailor applications' behavior or provide necessary input. They offer control and precision, similar to Linux commands, and are a powerful asset for developers.
By understanding Java's method architecture and internalizing argument and parameter concepts, developers can leverage Java's strengths in various development scenarios. Java's adaptability, versatility, and compatibility with other languages make it a favorite for creating diverse software applications.
In conclusion, grasping the power of arguments and parameters enhances code clarity, maintainability, and performance. Java's commitment to performance and productivity is evident in its method invocation mechanisms, supporting synchronous and asynchronous operations. Effective use of arguments and parameters is fundamental for building robust and efficient Java applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.