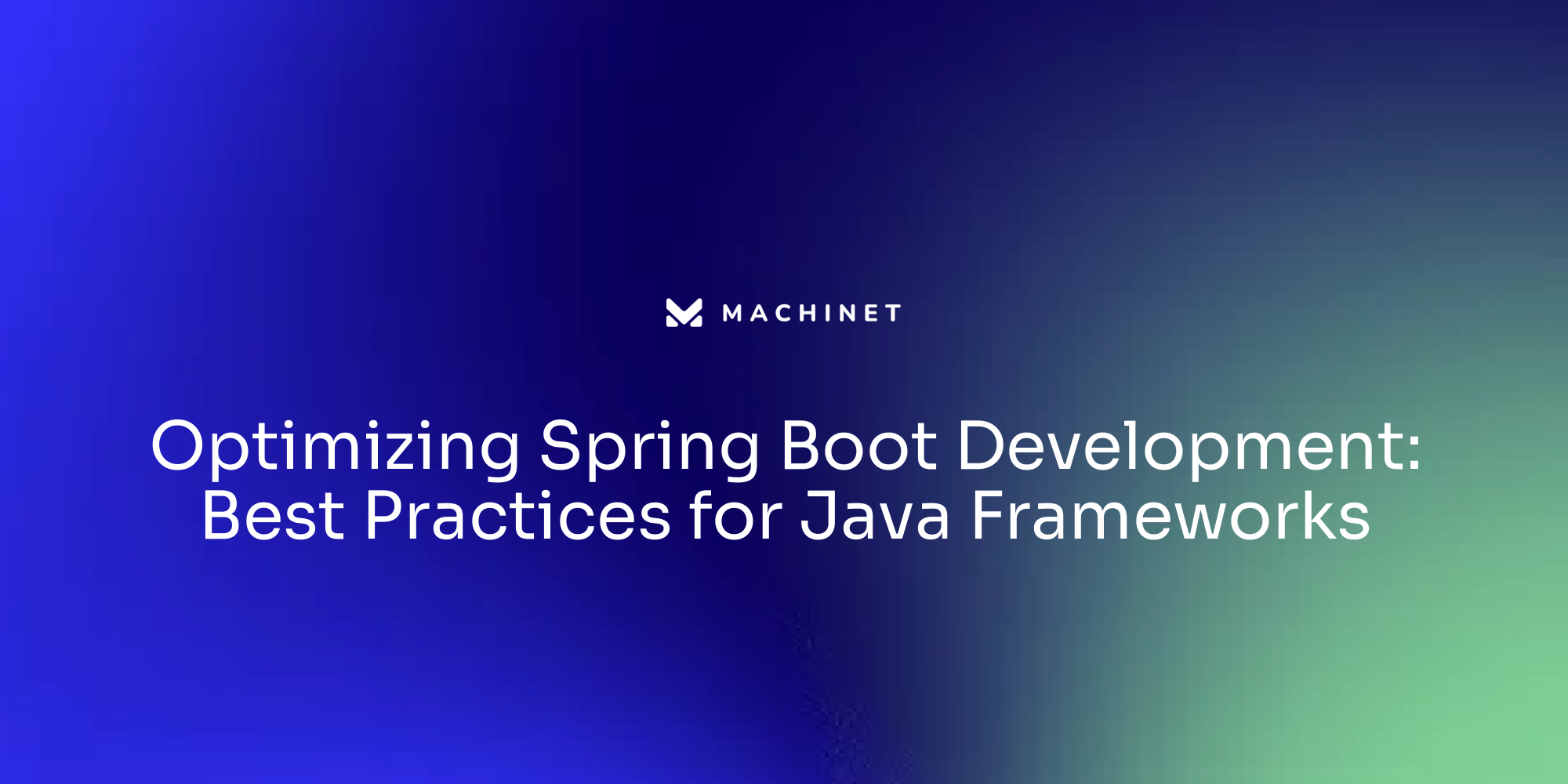
Table of contents
- Understanding the Basics of Spring Boot
- The Role of Test-Driven Development in Java Frameworks
- Setting Up an Efficient Unit Testing Environment in Spring Boot
- Implementing Automated Unit Testing in Java with Spring Boot
- Strategies for Managing Technical Debt and Legacy Code in Spring Boot
- Balancing Workload and Deadlines: Optimizing Test Efforts without Compromising Quality
- Case Study: Successful Implementation of Automated Unit Testing in a Spring Boot Project
Introduction
Automated unit testing is a crucial aspect of software development, especially in the context of Spring Boot projects. By prioritizing testing based on code complexity and risk, developers can optimize their testing efforts without compromising code quality. Automation tools like JUnit and Mockito simplify the testing process, saving time and reducing the risk of human error. Test-driven development (TDD) can also accelerate the development process by minimizing debugging and maintenance time. Balancing workload and deadlines requires a strategic approach, including the use of delivery metrics, dedicated bug days, and a pragmatic collaboration between teams. Real-world case studies highlight the success of automated unit testing in improving code quality, reducing debugging time, and enhancing overall development processes. By implementing automated unit testing in Spring Boot projects, developers can ensure code correctness, early bug detection, and code reusability, ultimately delivering high-quality software solutions
1. Understanding the Basics of Spring Boot
Spring Boot, an integral module of the Spring Framework, is highly favored among Java developers for architecting self-contained, production-ready applications. It provides a simplified approach to application setup and development by offering out-of-the-box configurations, thereby reducing the need for manual setup and boilerplate code.
One of the key features of Spring Boot is its auto-configuration capability that configures beans and settings based on classpath and dependency configurations. This dynamic wiring of beans and settings at the start of the application minimizes the need for extensive manual configuration, thereby reducing the likelihood of errors.
To set up a Spring Boot application, it is necessary to have Java Development Kit (JDK) installed on your system. Then, create a new Maven or Gradle project and add the necessary dependencies for Spring Boot by adding the Spring Boot starter dependencies to your project's build file. Then, make a main class with the @SpringBootApplication
annotation, which serves as the entry point for your application. From here, you can start building your Spring Boot application by adding controllers, services, and repositories according to your needs.
The Spring Initializr tool, an essential component of Spring Boot, aids in initializing a Spring Boot project with necessary dependencies and configurations. This tool simplifies the creation of a web application with Spring Boot, which necessitates the creation of a web controller class. The main application class, marked with the @SpringBootApplication annotation, enables auto-configuration and component scanning, thereby making the development process more efficient.
Spring Boot applications can be executed using the Gradle or Maven build tools, providing flexibility to developers. Unit tests for Spring Boot applications can be written using the Spring Test framework, ensuring the quality of the software.
Spring Boot also boasts of an Actuator module, offering management services, such as health checks and bean audits. The Actuator module can be added to a Spring Boot project to expose these management services, crucial for monitoring and maintaining the application's health.
Furthermore, Spring Boot supports both traditional WAR file deployments and executable JAR files, offering flexibility in deployment options. For more detailed information about its features and capabilities, developers can refer to Spring Boot's comprehensive online documentation.
Spring Boot, being an open-source enterprise-level framework, is ideal for creating standalone production-grade applications that run on the Java Virtual Machine (JVM). It requires minimal or zero configuration and is easy to get started with. Thanks to its built-in support for various tasks such as data binding, type conversion, validation, exception handling, resource and event management, and internationalization, Spring Boot is a robust tool for all kinds of applications, including web applications, RESTful APIs, microservices, batch processing applications, and command-line applications.
Key features of Spring Boot, such as auto-configuration, dependency injection, starter dependencies, embedded servers, and production-ready features, make it a potent tool for Java developers. With its support for embedded servers like Tomcat, Jetty, and Undertow, Spring Boot eliminates the need for external web servers and war file deployment. Moreover, Spring Boot includes production-ready features such as metrics, health checks, and external configuration management, making it an excellent choice for enterprise-level applications.
The choice between Spring and Spring Boot depends on specific requirements, with Spring being suitable for complex applications and Spring Boot being suitable for rapid application development. However, it's noteworthy that Spring Boot has a faster setup time compared to Spring. The simplicity and efficiency of Spring Boot make it a compelling choice for Java developers, significantly enhancing the development process
2. The Role of Test-Driven Development in Java Frameworks
Test-Driven Development (TDD) is a software development approach that emphasizes the importance of testing before the actual code construction. This method is particularly significant within Java frameworks such as Spring Boot, where it ensures code quality and functionality.
TDD encourages developers to think about the expected outcomes before writing the code, fostering a more calculated and efficient design process. This practice ensures that each segment of code has a corresponding test, bolstering code coverage and reducing the risk of bugs in production.
However, it is worth noting that TDD may slow down the initial stages of the development process, as writing tests before the code requires additional time. Yet, this perceived disadvantage is often offset by reduced debugging and maintenance time in the long run.
In the context of compliance standards like Autosar, C 14 Cert, CWE, DO 178BC, IEC 62304, ISO 26262, ISO 21434, MISRA, and OWASP, TDD offers a robust solution for maintaining code quality. These standards demand rigorous testing, efficiently managed through a TDD approach.
When it comes to testing services like performance testing, API security testing, reporting analytics, requirements traceability, application security testing, TDD provides a solid foundation. Writing tests before code helps enhance these testing services, ensuring that all code is thoroughly tested and validated against defined requirements.
TDD plays a significant role in industries such as embedded systems, automotive, civil aviation, industrial automation, medical devices, military defense, rail, enterprise, finance, healthcare, insurance, hospitality, travel, public sector, retail, e-commerce, and telecommunications. These industries often have stringent quality and safety requirements, and TDD ensures that all code meets these standards.
For products like CCTest for static analysis and unit testing in C/C++ code, JTest for Java unit testing and static analysis, DotTest for static analysis in C#/.NET software, and Soatest for managing test suites, API load testing, and security testing, TDD provides a solid foundation for ensuring high-quality code.
Machinet, a platform offering resources related to software development, including blog posts on unit testing and TDD, supports the TDD process. The resources provided by Machinet can help developers gain a better understanding of TDD principles and techniques, enabling them to write tests before the actual code. This approach aids in designing applications in a more modular and testable manner, leading to improved code quality and easier maintenance.
Integrating Machinet with TDD frameworks like Spring Boot can enhance the testing process, leveraging the benefits offered by Machinet for efficient and effective TDD. It can be done by configuring the Machinet URL in your Spring Boot application and creating test cases using the TDD framework of your choice. The Machinet API can interact with the TDD framework in your test cases, running tests, retrieving test results, and performing other actions supported by Machinet.
In essence, while TDD may initially slow down the development process, it ultimately leads to higher-quality code, reduced debugging and maintenance time, and better alignment with compliance standards and testing requirements. This makes it an invaluable methodology for software development in a vast range of industries and applications
3. Setting Up an Efficient Unit Testing Environment in Spring Boot
Unit testing in Spring Boot applications necessitates a meticulous approach to ensure optimal efficiency and effectiveness. The journey towards this goal commences by establishing a well-structured project, adhering to best practices. This involves segregating source code and test code into distinct directories or packages, thereby maintaining an organized and clean codebase. Creating a dedicated directory for unit tests facilitates easier management and location of test classes. Moreover, adhering to naming conventions for test classes and methods by prefixing them with "Test" or "IT" (Integration Test) enhances the ease of identification and execution of tests.
The next critical step involves the selection of a suitable testing framework. JUnit and Mockito are highly recommended choices for unit testing within the Spring Boot framework due to their compatibility and ease of use. JUnit facilitates the testing of individual code units in isolation, a fundamental aspect of unit testing. It provides a clear and standardized way of organizing and executing tests and integrates well with Spring Boot's testing infrastructure. JUnit's vast range of assertion methods and support for parameterized tests simplifies the process of writing test cases.
On the other hand, Mockito, a popular mocking framework, can be employed for stubbing dependencies, thereby isolating the code under test. Mockito allows the creation of mock objects, defining their behavior during unit testing, particularly useful for testing code with dependencies on external systems or services.
To further enhance the testing process, utilizing a build tool such as Maven or Gradle is advisable. These tools automate the build and test processes, significantly reducing manual effort and potential for errors. The Spring Boot Maven Plugin, for example, provides a set of goals to help with building and testing your Spring Boot application, while Gradle can streamline unit testing by including necessary dependencies in the build file and configuring Gradle to run unit tests as part of the build process.
Configuring your Integrated Development Environment (IDE) to support unit testing is the final step in the setup. Most modern IDEs, including IntelliJ IDEA, come with built-in support for unit testing, making this step straightforward. IntelliJ IDEA, for instance, allows adding the appropriate testing framework dependency such as JUnit or TestNG to your project, enhancing your unit testing experience in Spring Boot.
When testing Spring Boot applications, adhering to the principles of fast, independent, repeatable, self-validating, and timely (FIRST) tests is recommended. Achieving this necessitates isolating the functionality under test by limiting the context of loaded frameworks and components. The @SpringBootTest
annotation can be used to limit the application context to just the set of Spring components that participate in the test scenario. Coupling this approach with the use of the @DataJpaTest
annotation to load only repository Spring components can significantly enhance the performance of your unit tests.
For testing REST APIs exposed through controllers without running the server, the @WebMvcTest
annotation can be utilized. Database interactions can also be streamlined by mocking beans and turning off Spring Boot test database initialization.
The setup and execution of an efficient unit testing environment in Spring Boot require a strategic approach that includes the selection of appropriate testing frameworks, the use of build automation tools, the right IDE configuration, and adherence to the FIRST principles of testing. With these in place, the challenges of unit testing can be effectively managed, ensuring the high quality of your Spring Boot applications
4. Implementing Automated Unit Testing in Java with Spring Boot
In the modern landscape of software engineering, automated unit testing is a fundamental element. Spring Boot provides a fertile environment for its application, offering a plethora of libraries and tools that streamline the process. JUnit, an established testing framework in the Java community, is fully supported by Spring Boot, making the creation of comprehensive unit tests a straightforward operation. Mockito, another essential tool, simplifies the testing process by aiding in the creation of mock objects within tests.
When it comes to examining the interaction between various components of a Spring Boot application, integration testing is the method of choice. Spring Boot Test, a utility purpose-built for this task, is an indispensable ally. It helps confirm that the individual parts of your application function harmoniously when combined.
A crucial aspect of automated testing is the automatic execution of tests. This is where Continuous Integration (CI) servers, such as Jenkins or Travis CI, enter the picture. These tools can be configured to build your project and execute your tests automatically whenever changes are pushed to your repository. This ensures quick identification and resolution of any issues or bugs, thereby maintaining the integrity of your codebase.
Let's explore the specifics of setting up a basic Spring Boot REST service and writing unit tests for it. The process kicks off with initializing a Spring Boot project using Spring Initializr. Subsequently, a business service for the API, termed 'StudentService', is created. This service employs an ArrayList as an in-memory data store and provides methods for retrieving student and course information.
Next, we introduce the 'StudentController' class. This class exposes GET services for retrieving student and course information. Execution of a GET operation can be performed using Postman, a widely used tool for API testing. For the purpose of unit testing, security can be disabled using the 'spring-security-test' dependency. The '@WebMvcTest' annotation, specifically designed for unit testing Spring MVC components, can be used in conjunction with the 'MockMvc' class to perform requests and receive responses.
The HTTP POST operation is then added to the REST service. This operation is vital for creating new resources. The URL for the POST request is mapped, and the '@RequestBody' annotation is used to bind the request body to an object. The expected response status and location header are set, and the process of executing the POST operation can be demonstrated using Postman. A unit test for the POST operation is then written, with the focus on checking the response status and location header.
In the broader perspective of application development, managing technical debt is of utmost importance. One way to ensure this is through the implementation of automated unit testing in your Spring Boot projects. By harnessing the power of JUnit, Mockito, and Spring Boot Test, you can establish a robust and efficient testing environment. Paired with CI servers like Jenkins or Travis CI, you can keep your codebase in optimal health and robustness, paving the way for the delivery of high-quality software solutions
5. Strategies for Managing Technical Debt and Legacy Code in Spring Boot
Technical debt, that accumulation of suboptimal or outdated code, is a reality that software developers must contend with. Legacy applications, often built on outdated technology stacks, are a common source of technical debt, leading to slower, less responsive, and challenging to scale systems. Over time, ignoring technical debt can lead to resource-intensive complications.
In the realm of Spring Boot development, several strategies can be employed to manage technical debt and legacy code. A primary strategy involves regular code refactoring. This process involves simplifying complex methods, ridding the code of duplication, and updating outdated libraries. By doing so, the code's quality and maintainability are significantly enhanced.
An effective way to simplify complex methods in Spring Boot is through techniques such as refactoring, code modularization, and applying design patterns. By breaking down complex methods into smaller, more manageable pieces, code readability, maintainability, and testability are improved. By leveraging Spring Boot features such as dependency injection and aspect-oriented programming (AOP), complex methods can be further simplified.
To remove duplicate code in Spring Boot applications, various techniques can be used. One approach is to use inheritance or composition to extract common code into reusable components or base classes. This approach helps to eliminate redundancy and improve code maintainability. Another approach is to use aspect-oriented programming (AOP) with Spring's AspectJ framework, which helps in reducing code duplication and improves modularity.
Updating outdated libraries in a Spring Boot application involves identifying the outdated libraries, updating their version numbers, resolving compatibility issues, and thoroughly testing the application to ensure everything works as expected. Once confident in the application's functionality with the updated libraries, it can be deployed to the production environment.
Another crucial strategy is ensuring that legacy code is adequately covered by tests before making any changes. This process helps to identify any regressions introduced by the changes, preventing them from causing errors or malfunctions. Identifying critical functionality within the legacy code that needs to be covered by tests can be done by analyzing the codebase and understanding the key features and business logic. Once the code has been refactored, unit tests can be written to cover the critical functionality. Unit tests should focus on testing individual methods or components in isolation to ensure that they are functioning correctly.
A third strategy involves the gradual migration of legacy code to Spring Boot. This can be done module by module, reducing the risk of breaking your application. By leveraging Spring Boot's tools and features, developers can gradually refactor and modernize the existing codebase while benefiting from the advantages of the Spring Boot framework.
Managing technical debt requires the involvement of senior engineers who have a deep understanding of the entire system and can make informed decisions. Continuous assessment of the current status versus the risk of migrating to newer systems is crucial. Temporary code can be written as an interim solution, and success in reducing technical debt should be rewarded and celebrated.
A software development company, AppsFlyer, has implemented effective strategies for managing technical debt. These include continuously considering it in technological decisions and emphasizing code craftsmanship and engineering excellence. They've taken risks, such as switching core data stores in production and rewriting mission-critical services to improve functionality.
Managing technical debt is crucial for maintaining developer velocity and sanity, especially during periods of rapid growth and change. It's essential to have a company-wide culture that values and prioritizes the management of technical debt to prevent cascading failures and ensure the system's resilience.
To sum up, managing technical debt and legacy code is a common challenge in software development. However, with the right strategies and a proactive approach, it's a challenge that can be effectively managed
6. Balancing Workload and Deadlines: Optimizing Test Efforts without Compromising Quality
As a software engineer, the challenge of juggling workload, deadlines, and maintaining code quality is a feat akin to tightrope walking. The core issue lies in optimizing your testing efforts without compromising the quality of the product. One prominent strategy is to prioritize testing based on the inherent risk and complexity of the code. The more vulnerable and intricate sections of the code should be addressed first.
Automation is a crucial player in this balancing act, especially for tests that are repetitive and time-consuming. These tests are perfect candidates for automation, which not only saves valuable time but significantly mitigates the risk of human error.
Test-Driven Development (TDD) is another viable strategy. While TDD may initially seem to decelerate the development process, it can potentially quicken later stages by minimizing the time dedicated to debugging and maintenance.
However, maintaining engineering health and delivery speed is a common stumbling block for engineering leaders. Teams can easily fall into patterns of firefighting and getting bogged down. To counter this, strategies such as dedicated bug days, engineering health sprints, and incidents and support processes can be useful.
Delivery metrics, such as cycle time, PR review time, and velocity per sprint, can assist in tracking and enhancing delivery speed. Additionally, the 60-30-10 strategy provides a framework for time allocation towards maintenance, improvements, and delivery, respectively, to balance competing priorities.
The balancing act should not just be a top-down approach. The goal should be to empower teams to take ownership and make informed decisions on achieving balance and adjusting as needed.
When managing this balance, it is crucial to remain pragmatic. Upon receiving a request for a bug fix or feature delivery, three possible responses emerge: technical rigidity, blind acceptance, and pragmatic collaboration.
Technical rigidity, a focus purely on the technical aspect that ignores the impact on the product and organization, is the least appropriate response. Conversely, blind acceptance, which involves promising quick workarounds and allowing the product manager to have full ownership of the technical aspect, can lead to untested errors, immense pressure, and a lack of trust.
Pragmatic collaboration is the ideal approach. This involves understanding the urgency and impact of the request, estimating the overall impact on the product, team, and code infrastructure, and making a collaborative decision on whether to do a workaround or deliver the proper solution.
Remember, workarounds should not be left in the code indefinitely. Long-term maintainability of the codebase should always be the focal point.
Consider a real-life example of collaborative decision-making in a company. A workaround was used to deliver a feature quickly, followed by working on the proper solution afterward. This emphasizes the importance of learning from experience and avoiding past mistakes in balancing business needs and engineering excellence in software development.
As Mariusz Soltysiak, a software engineer with more than 15 years of experience, aptly put it, "Some people say it's impossible, some people say it's hard, I say it's not easy but achievable." This philosophy embodies the spirit of pragmatic collaboration and long-term maintainability in software development
7. Case Study: Successful Implementation of Automated Unit Testing in a Spring Boot Project
Drawing from real-world case studies, we can appreciate the transformative potential of automated unit testing. Let's delve into a couple of examples that highlight its practical application.
Heart Test Laboratories, creators of MyoVista, a heart disease screening tool, found their development process revolutionized by SmartBear Collaborator. This tool, which facilitates code reviews and document reviews, became a linchpin in their testing and code review process. Notably, it reduced a projected three-month review process to just three weeks, marking a significant 70% reduction in the review timeline.
Similarly, Stack Overflow, the ubiquitous platform used by over 100 million developers each month, utilized Mabl, a test automation solution, to enhance their software development organization and product quality. Mabl's low-code test automation platform allowed Stack Overflow to create reusable testing flows and scale their quality engineering strategy.
But how can these experiences be mirrored in a Spring Boot project? Consider a case study involving the use of Mockito, a widely-used mocking framework for Java unit testing. Mockito allows developers to create mock objects, simulating the behavior of dependencies for isolated and focused testing of individual components. Using Mockito in a Spring Boot project, a development team was able to substantially improve their code quality, reduce bug numbers, and get faster feedback on code changes.
Automated unit testing, as demonstrated by these examples, has multiple benefits. It ensures the correctness and functionality of individual units of code, allows for early bug detection, serves as documentation for the code, and promotes code reusability and modularity. Furthermore, it provides a safety net during refactoring or code changes.
Let's consider how to implement automated unit testing using JUnit and Mockito. The process involves setting up your project with JUnit and Mockito as dependencies, writing test cases, mocking dependencies, asserting expected outcomes, and running the tests using a JUnit-supported testing framework or IDE.
Automated unit testing has a proven impact on bug detection and debugging time. By running tests frequently and easily, developers can catch bugs early in the development process, reducing debugging time and effort. Furthermore, automated tests serve as a safety net for future code changes, ensuring existing functionality isn't inadvertently broken. This leads to more efficient bug detection and faster debugging.
In a Spring Boot application, automated unit testing can significantly improve code quality. By testing individual components, developers can ensure each part of their code functions correctly and as expected. Additionally, unit tests provide documentation and examples of how to use the code, making it easier for other developers to understand and work with the codebase.
To measure automated unit testing's effectiveness in Spring Boot projects, consider factors such as code coverage, test execution time, and test failure rates. These metrics can provide insights into the effectiveness of automated unit testing and guide necessary improvements.
In conclusion, Heart Test Laboratories, Stack Overflow, and a Spring Boot project using Mockito demonstrate the potential of automated unit testing to enhance code quality, reduce debugging time, and expedite feature delivery. Other development teams can adopt similar practices and tools to achieve improved code quality and more efficient development processes
Conclusion
The main points discussed in this article include the basics of Spring Boot, the role of test-driven development (TDD) in Java frameworks, setting up an efficient unit testing environment in Spring Boot, implementing automated unit testing in Java with Spring Boot, strategies for managing technical debt and legacy code in Spring Boot, balancing workload and deadlines, and a case study on the successful implementation of automated unit testing.
In conclusion, automated unit testing is a crucial aspect of software development in Spring Boot projects. By prioritizing testing based on code complexity and risk, developers can optimize their testing efforts without compromising code quality. Automation tools like JUnit and Mockito simplify the testing process, saving time and reducing the risk of human error. Test-driven development (TDD) can also accelerate the development process by minimizing debugging and maintenance time. Balancing workload and deadlines requires a strategic approach, including the use of delivery metrics, dedicated bug days, and a pragmatic collaboration between teams. Real-world case studies highlight the success of automated unit testing in improving code quality, reducing debugging time, and enhancing overall development processes. By implementing automated unit testing in Spring Boot projects, developers can ensure code correctness, early bug detection, and code reusability, ultimately delivering high-quality software solutions.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.