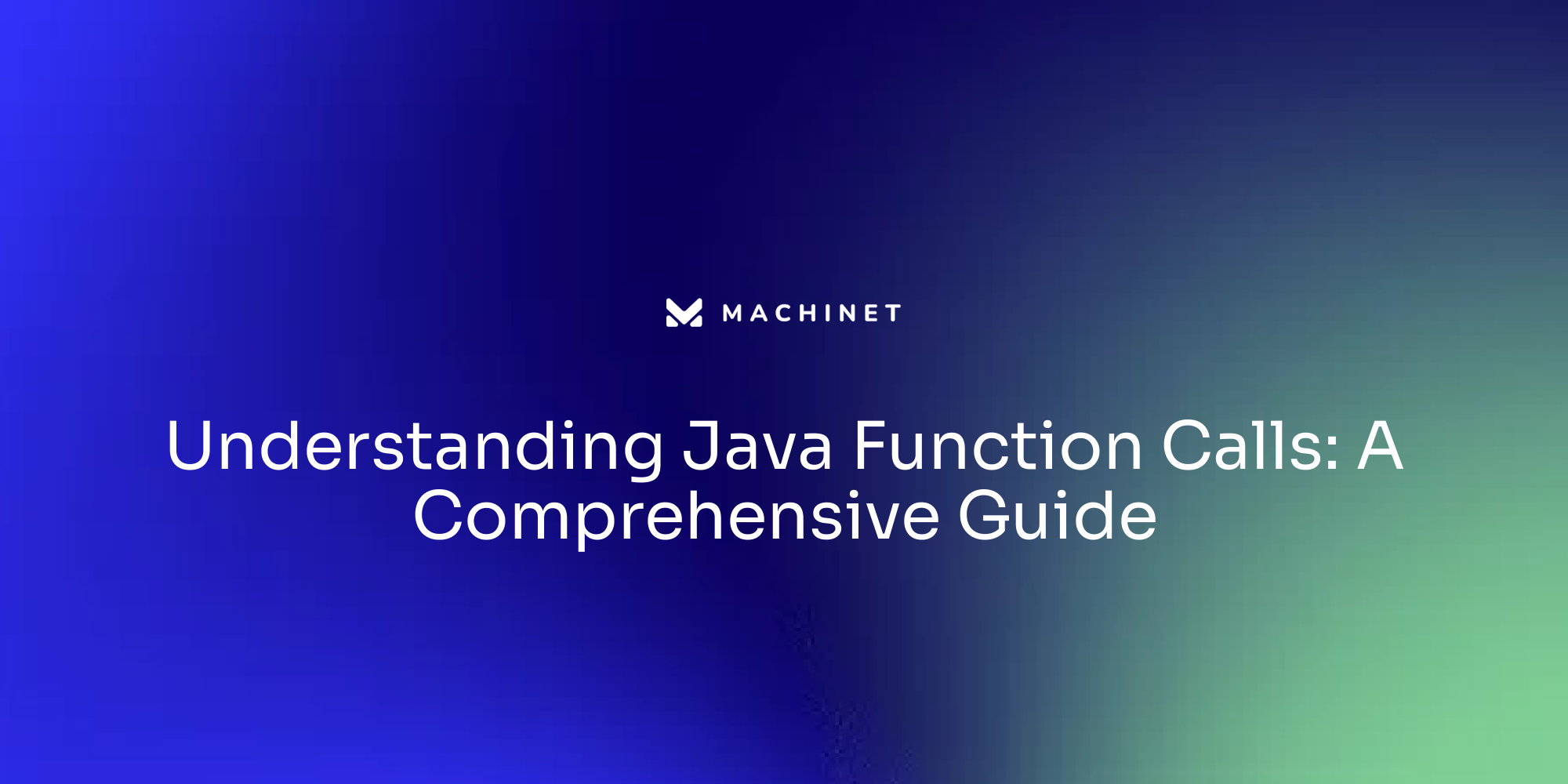
Introduction
Java methods serve as the backbone of any Java application, enabling developers to encapsulate code into reusable and organized units. This modular approach simplifies complex programming tasks by breaking them down into manageable pieces. By understanding the various types of Java methods—instance methods and static methods—developers can effectively manage an object's state and class-level operations.
Differentiating between these types of methods is crucial for writing maintainable code.
Further insight into method signatures and calls reveals how methods are uniquely identified and executed within a class. Access modifiers, such as public, private, and protected, play a pivotal role in controlling method visibility, enhancing both security and encapsulation. Additionally, the mechanics of method execution and invocation by the Java Virtual Machine (JVM) highlight the efficiency of Java's runtime environment.
Leveraging method references and adhering to best practices in method usage, such as focusing on single tasks and proper documentation, can significantly improve code readability and maintainability. This comprehensive understanding of Java methods is essential for any developer aiming to write clean, efficient, and scalable Java code.
What are Java Methods?
'Java functions are fundamental building blocks within a class, designed to perform specific tasks.'. By enclosing functions within procedures, programmers can attain enhanced reusability and structure. This modular approach is essential for breaking down complex programs into smaller, more manageable pieces. Methods can accept inputs through parameters, enhancing their flexibility and functionality. Additionally, they can return values to the caller, facilitating efficient data processing and manipulation. This practice not only improves code readability and maintenance but also aligns with the principles of good software engineering, such as those highlighted in version control and collaborative development scenarios.
Types of Java Methods
Java functions are classified into two primary categories: object functions and static functions. Object functions are linked to a particular object of a type, enabling them to access and alter the object attributes and invoke other object functions. For instance, in a Dog type, functions like bark
and ageOneYear
can engage with properties such as name
and age
, offering a means to encapsulate and handle an object's state efficiently.
Conversely, unchanging functions are associated with the type itself instead of any specific instance. They function at the class level and can only reach static variables and other static functions. This distinction makes static functions suitable for utility or helper tasks that do not depend on the state of individual objects.
Understanding these concepts is crucial for writing reusable and maintainable code in Java, as it helps in organizing functionality and managing state appropriately.
Static vs. Instance Methods
'Static functions, designated with the 'static' keyword, can be called without requiring the creation of an instance of the type. These techniques are perfect for utility or helper functions that do not depend on object state. For instance, mathematical operations in the Math course are usually carried out as static functions. Static functions can also act as factory functions, providing adaptable and contained approaches to generate objects of a type.
Conversely, class functions necessitate an object of the class to be invoked. These techniques can alter object properties and establish the behavior of separate entities. While static functions can be viewed as a micro-optimization by avoiding object instantiation overhead, this is not a significant factor in modern programming. Rather, the decision between static and instance functions should be directed by the design and functionality needs of the application.
Method Signature and Method Calling
A function signature in Java includes the function's name and its parameter list, which together serve to uniquely identify the function within a class. This is vital for differentiating techniques, particularly when overloading is utilized, where several functions share the same title but have distinct parameters. The function body contains the statements that define the actions performed by the function.
When calling a function, you invoke it by referencing its name and passing the appropriate arguments that match its parameters. This process is essential for executing the code of the approach. It is important to ensure that each function call is clear and concise to avoid confusion, which can be further enhanced by practices such as chaining in some programming languages.
Grasping the elements of a technique is essential. The access Specifier defines the visibility of the operation, return Type indicates the type of value it provides (or 'void' if it provides nothing), methodName follows Java naming conventions, and parameters are the values accepted. This structure allows developers to write clean, maintainable, and efficient code.
Understanding Method Access Modifiers
Access modifiers in Java, such as public, private, and protected, play a crucial role in controlling the visibility and accessibility of functions, thereby enhancing encapsulation and security.
- Public Functions: These functions can be accessed from anywhere in the application, making them part of the public interface of the structure.
- Private Functions: Limited to the structure in which they are created, private functions prevent outside structures from accessing or altering sensitive information directly.
- Protected Functions: These functions can be accessed by subclasses and entities within the same package, providing a balance between accessibility and encapsulation.
Encapsulation, a fundamental concept of object-oriented programming, entails grouping data and functions within a structure, limiting direct access to an object's internal state. By using access modifiers, developers can control how data is accessed and modified, ensuring data integrity and security. For instance, a class may encapsulate a private attribute and offer public getter and setter functions to manage access and validate modifications, thereby preventing unintended alteration of the internal state.
How Java Methods are Executed
When a function is invoked in Java, the Java Virtual Machine (JVM) allocates memory for the function's parameters and local variables on the call stack. 'This allocation is part of the JVM's function call mechanism, where each function invocation leads to a new frame being pushed onto the stack.'. This frame includes the procedure parameters and local variables. As the procedure executes, it operates within this frame, utilizing the allocated memory space.
Upon the procedure's completion, control returns to the caller, and the frame is popped off the stack. If the procedure has a return value, this value is passed back to the caller. This process is essential for communication between different parts of a program. The efficiency of this mechanism is maintained by the JVM's Just-In-Time (JIT) compilers, C1 and C2, which optimize the execution during runtime.
Method Invocation and the JVM
Method invocation in Java is a multi-step process managed by the Java Virtual Machine (JVM). When a procedure is invoked, the JVM first locates the procedure's definition and then prepares the call stack, which is essential for keeping track of procedure calls and their corresponding data. During the execution of the procedure, the JVM handles the procedure's context, including managing local variables and parameters. Upon finishing the procedure, the JVM cleans up the call stack to ensure that all resources are properly freed, maintaining optimal performance and memory management.
Using Method References
References in Java provide a streamlined approach to point to functions without calling them directly. This feature is frequently combined with functional programming constructs, such as lambdas, enhancing clarity and succinctness. By permitting functions to be passed as arguments, function references enhance functional programming principles and boost maintainability. For example, utilizing function references instead of unnamed inner classes or lambda expressions can greatly minimize repetitive elements, resulting in a cleaner and more comprehensible codebase. This corresponds with refactoring strategies such as Extract Function, which seeks to enhance the clarity and maintainability of programming by decomposing intricate scripts into more straightforward, reusable functions. As Java continues to evolve with advancements like JEP 431, which enhances the collections framework, developers can leverage these features to write more efficient and maintainable programs.
Best Practices for Java Method Usage
To uphold effective and sustainable programming, it is crucial to adhere to best practices for function usage. Begin by making sure each approach is concentrated on one specific task, which not only makes the programming easier to comprehend but also streamlines troubleshooting and evaluation. Use meaningful names for functions, as this significantly enhances code readability and maintainability. It is also beneficial to limit the number of parameters in procedures to keep them manageable and reduce complexity.
Consistently applying access modifiers is another crucial practice. Utilizing the suitable access level for each function improves security and encapsulation, guaranteeing that functions are only reachable where needed. Moreover, thorough documentation for each method is vital. Adequate documentation not only assists other developers in comprehending the programming but also supports future maintenance and updates.
Incorporating these best practices into your coding routine will lead to cleaner, more efficient, and more maintainable code, ultimately facilitating better software development outcomes.
Conclusion
Java methods are integral to the structure and functionality of any Java application, allowing developers to encapsulate tasks into manageable units. By understanding the distinctions between instance methods and static methods, developers can effectively manage both object states and class-level operations. This modular approach not only enhances code reusability but also simplifies complex programming tasks, aligning with best practices in software engineering.
The importance of method signatures, access modifiers, and the mechanics of method execution cannot be overstated. Method signatures uniquely identify methods within a class, facilitating clarity and preventing confusion, especially in cases of method overloading. Access modifiers enhance encapsulation and security, ensuring that methods are accessible only where appropriate.
Furthermore, the role of the Java Virtual Machine (JVM) in method invocation underscores the efficiency of Java's runtime environment, which is crucial for optimal performance.
By leveraging method references and adhering to best practices—such as focusing on single tasks, using meaningful names, and maintaining thorough documentation—developers can significantly improve code readability and maintainability. These practices are essential for writing clean, efficient, and scalable Java code, ultimately leading to better software development outcomes. Understanding and implementing these concepts is vital for any developer striving to excel in Java programming.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.