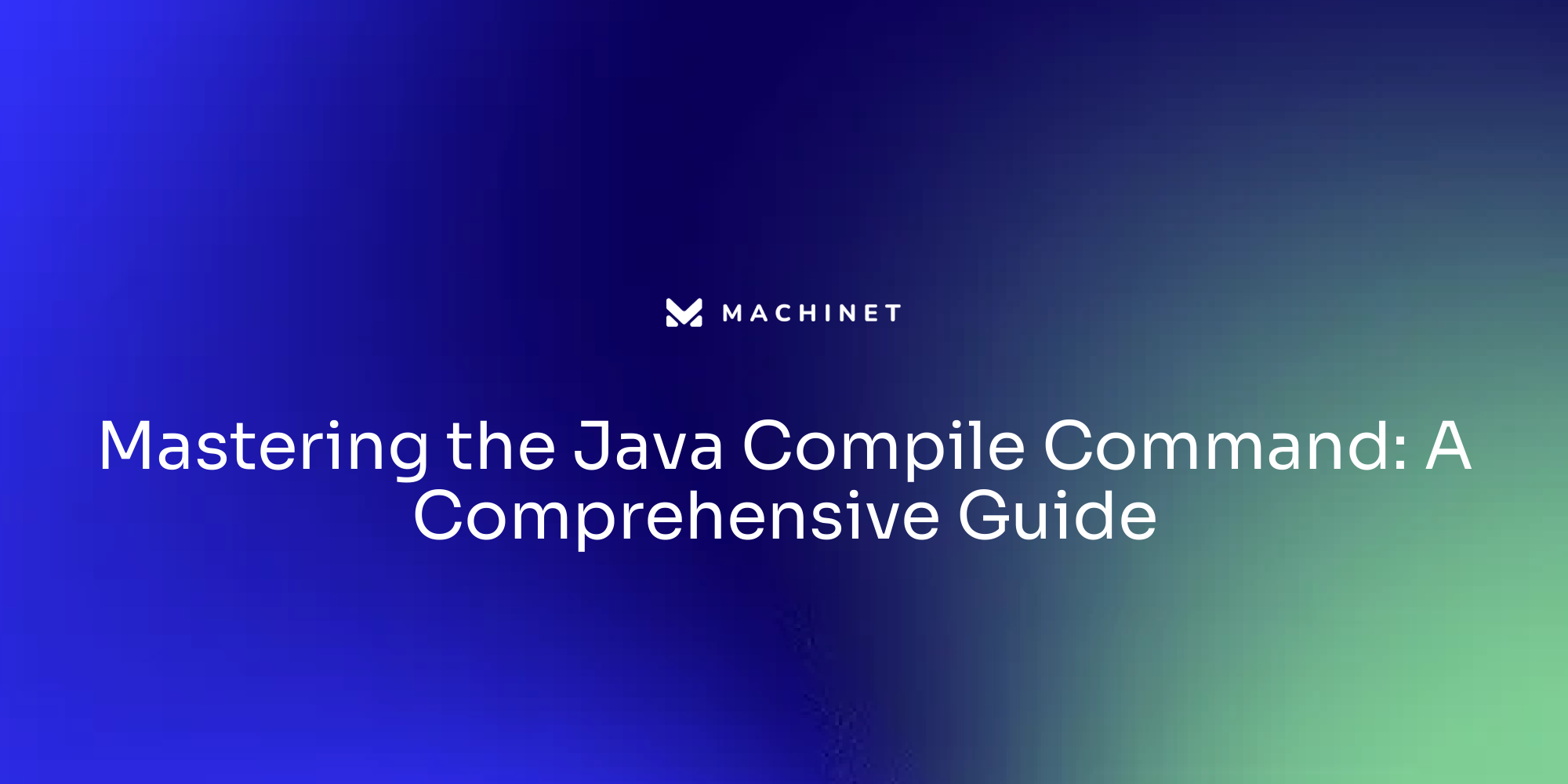
Introduction
Transforming Java source code into bytecode is a fundamental process for running Java applications, as the Java Virtual Machine (JVM) cannot directly interpret the source code. This crucial transformation is performed by the Java compiler, javac
, which is part of the Java Development Kit (JDK). The JDK offers a comprehensive suite of tools and libraries necessary for compiling, debugging, and running Java applications.
Understanding the organization of source files, package structures, and the interaction between the compiler and the JDK is vital for mastering Java compilation. The syntax of Java, derived from C and C++, promotes a clean and organized coding style, which is essential for effective development within the Java ecosystem. The evolving landscape of software development, with its increasing complexity and specialization, underscores the importance of mastering these fundamental processes for efficient and robust Java application development.
Understanding the Java Compile Process
Compilation converts source text into bytecode, which the Virtual Machine (JVM) can execute. This transformation is essential for running applications in that language, as the JVM does not directly understand source code from it. The programming language compiler, javac
, performs this critical task. The role of the Development Kit (JDK) is significant in this process, offering a suite of tools and libraries necessary for compiling, debugging, and running applications. Grasping how source files are arranged, the layout of packages, and the interaction of the compiler with the JDK is essential to mastering compilation. According to James Gosling, the creator of the programming language, its syntax is derived from C and C++, promoting a clean and organized coding style that is both readable and writable. This clean structure is crucial when working with the JDK, which acts as a comprehensive environment for programming development. As emphasized in a recent assessment, the movement towards more precise instruments and methods, such as privacy engineering in design, illustrates the increasing intricacy and specialization within software development, including the ecosystem of this programming language.
Step-by-Step Guide to Compiling a Java Program
-
Write Your Programming Code: Begin by creating a
.java
file using a text editor or an IDE. Ensure your program includes amain
method for execution. This step entails converting your algorithm, a comprehensive outline of actions, into programming language. Algorithms can be described in natural language or pseudocode, helping you design an efficient program before coding. -
Open the Command Line Interface: To compile and run your program, access the command prompt on Windows or the terminal on macOS/Linux.
-
Navigate to the File Location: Use the
cd
command to change directories to where your.java
file is stored. This ensures the compiler can locate your file. -
Compile the Code: Execute the command
javac YourFileName.java
. This compiles your Java program into bytecode, creating aYourFileName.class
file if no syntax errors are present. -
Check for Compilation Errors: If there are errors, the compiler will display messages indicating the issues. Address these errors to ensure your program compiles successfully.
-
Run the Compiled Code: Use the command
java YourFileName
to run the compiled bytecode. This step executes your program, allowing you to see the output of your code.
Using the Command Line for Java Compilation
'Compiling programs via the command line offers a powerful and flexible approach, with java
as the primary command.'. 'java' takes the source file name as an argument, but understanding additional options can greatly improve the building process.
- Destination Directory: Use the
-d
option to specify where the generated class files should be stored. This aids in organizing your output, keeping your project structure clean and manageable. - Classpath: The
-cp
option allows you to set the classpath, ensuring the compiler can locate referenced classes. This is particularly useful in larger projects with multiple dependencies. - Detailed Warnings: Activating
-Xlint
offers thorough alerts during the build process, assisting you in recognizing possible problems in your code at an early stage.
These choices not only simplify the assembling process but also aid in the creation of strong and efficient programming applications. For instance, the recent challenge set by Gunnar Morling involved processing a 1 billion row text file using a programming language, showcasing the importance of efficient building and processing techniques in handling big data.
Common Java Compilation Commands and Tools
Beyond the basic javac
command, several tools and commands enhance Java compilation processes:
java
: This command runs the compiled Java bytecode, enabling the execution of Java applications.jar
: By packaging multiple class files into a single JAR file, this utility simplifies distribution and execution, making it easier to manage and deploy Java applications.- Build instruments: Instruments like Apache Maven and Gradle automate the compilation process, manage dependencies, and streamline project builds. These resources are crucial for modern development, as they enable developers to concentrate on coding rather than manual build management.
The software development sector, with its 27 million developers, is supported by a multitude of resources created to enhance various tasks. 'These resources, such as source management, unit testing, and CI/CD integration, assist in automating processes and allow developers to focus on crafting software.'. Fred Brooks highlighted that a professional developer averages 10 lines of code per day, emphasizing the need for tools that reduce overhead and enhance productivity.
Heather VanCura, Director and Chairperson of the Java Community Process (JCP) program, emphasizes the significance of the technology in the industry. This programming language is not only in high demand but also one of the highest-paid technical skills. Efforts to bridge the gap between education and industry through networking, mentoring, and internships are vital. As the programming language continues to evolve, tools like Maven and Gradle are essential for maintaining stability, security, and performance in applications.
Troubleshooting Common Compilation Issues
The process of translating code can pose numerous difficulties, each demanding particular focus on precision. Here are some common issues and how to address them:
-
Syntax Errors: These occur when misplaced symbols or incorrect keywords disrupt the code structure. The programming language is strict about syntax, and even a small error can prevent successful compilation. Always check compiler error messages, which typically indicate the line number and description of the problem, to quickly locate and fix these issues.
-
Class Not Found: This error often arises when the classpath is not correctly set, especially when using external libraries. Ensure that all required libraries are correctly referenced and that the classpath is properly configured. 'This step is crucial to prevent runtime errors and to maintain the smooth operation of your application.'.
-
Version Incompatibility: The evolution of the programming language has introduced several versions, each with its own set of features and changes. It is crucial to verify that your Java source files version corresponds with the JDK version utilized for building. Mismatched versions can lead to unexpected errors and compatibility issues. Always verify that the development environment is aligned with the requirements of your project to avoid such pitfalls.
Advanced Topics in Java Compilation
Advanced coding methods can significantly enhance the efficiency of your development process. Hereβs a closer look at three such techniques:
-
Incremental Compilation: This technique saves time by recompiling only the files that have changed. By avoiding recompilation of the entire codebase, developers can see their changes reflected more quickly, streamlining the debugging and testing phases.
-
Annotation Processors: Using custom annotation processors during the build phase enables the creation of supplementary scripts. This can automate repetitive programming tasks and enforce programming standards, ultimately reducing manual errors and enhancing consistency.
-
Compiler APIs: The Java Compiler API allows for the programmatic building of Java programs. This capability is particularly useful for developing integrated tools and applications that require dynamic program compilation, such as custom development environments or automated build systems.
By mastering these advanced techniques, developers can optimize their build processes, resulting in faster development cycles and more efficient code management.
Conclusion
The Java compilation process is a cornerstone of effective Java application development, transforming source code into bytecode that the Java Virtual Machine can execute. The role of the Java compiler, javac
, and the Java Development Kit (JDK) is crucial, as they provide the necessary tools and libraries for compiling and running applications. Understanding the organization of source files, package structures, and the interaction between the compiler and the JDK is essential for mastering this process.
A step-by-step approach to compiling a Java program reveals the straightforward nature of the task, from writing the code to executing the compiled bytecode. Utilizing the command line enhances flexibility, with commands like javac
and options for specifying directories and classpaths, which contribute to a more organized development process. Additionally, various tools such as jar
, Apache Maven, and Gradle further streamline compilation and project management, allowing developers to focus on writing code rather than manual build processes.
Common compilation issues, such as syntax errors and classpath misconfigurations, can be effectively addressed with attention to detail and an understanding of Java's strict syntax rules. Advanced techniques like incremental compilation and annotation processors can significantly improve efficiency, facilitating quicker development cycles and reducing manual errors. By embracing these practices and tools, developers can enhance their Java programming capabilities, ensuring robust and efficient application development.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.