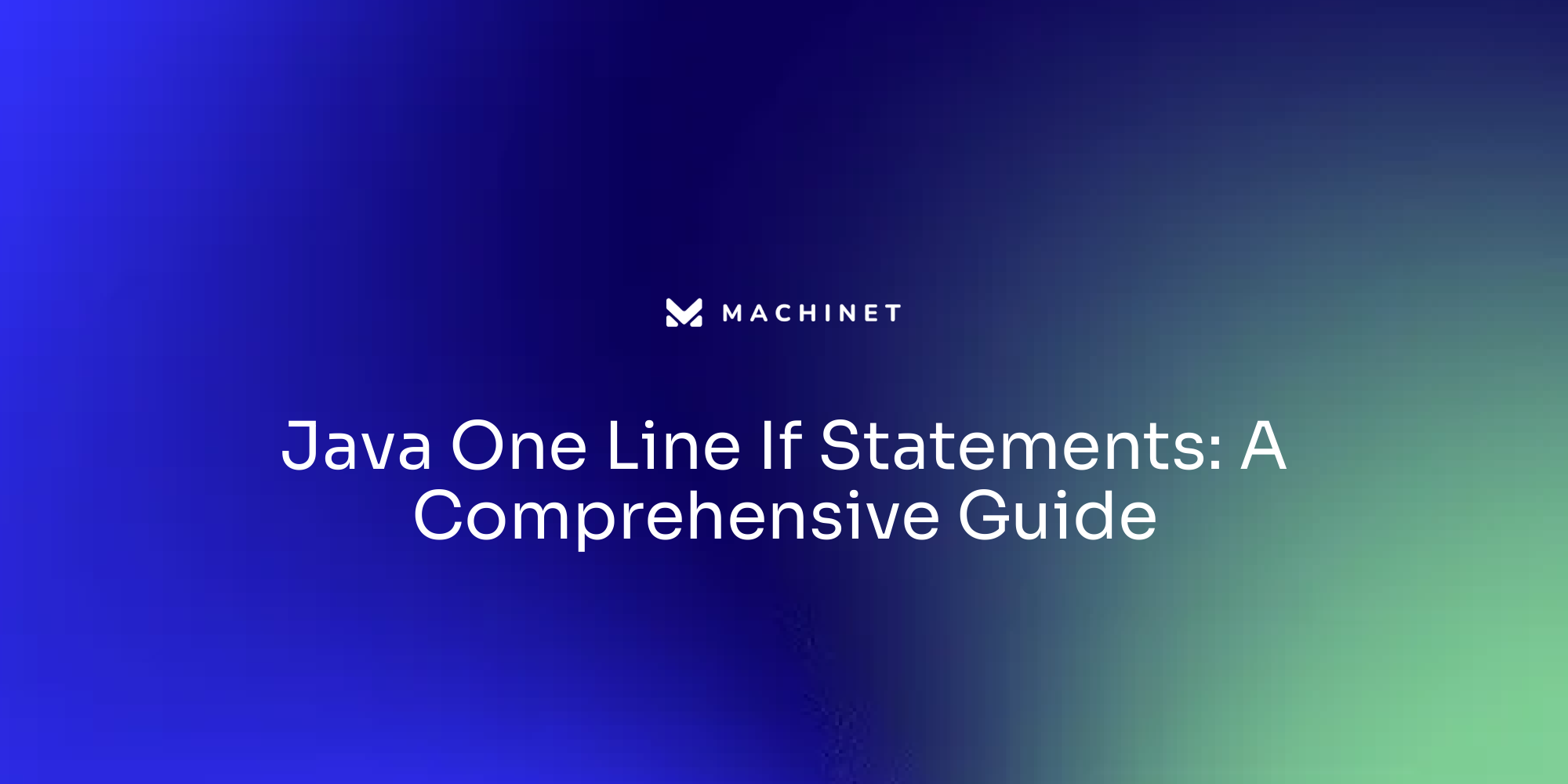
Introduction
The 'if' statement is a fundamental component of Java programming, allowing developers to execute code conditionally based on specific requirements. In this article, we will explore the basics of Java 'if' statements and their role in decision-making within programs. We will also delve into the syntax and usage of one-line 'if' statements, highlighting how they simplify code and enhance maintainability.
Additionally, we will discuss the importance of braces in one-line 'if' statements and explore the ternary operator as an alternative. To ensure best practices, we will provide tips for using one-line 'if' statements effectively and avoiding common pitfalls and errors. By the end of this article, you will have a comprehensive understanding of 'if' statements in Java and how to leverage them for clear and concise code.
Understanding the Basics of Java If Statements
The 'if' structure is a fundamental element of programming in Java, offering a way to execute code selectively depending on whether a specified condition is satisfied. This conditional logic is essential for guiding program flow and making decisions. For instance, consider a Java method that calculates discounts based on a customer's loyalty card type and accumulated points. Before the implementation of switch expressions, developers would utilize a switch coupled with a variable to hold the outcome of a lambda expression. The 'if' structure provides a more straightforward option in comparison to this, enabling direct execution of instructions when specific requirements are satisfied.
To illustrate, let's say the temperature is being monitored, and a specific action should only occur if it exceeds 20 degrees. In Java, this would be expressed as:
java
int temperature = 25;
if (temperature > 20) {
// Execute the code block if the condition is true
}
Here, the if
statement evaluates whether temperature
is greater than 20. If this condition holds true, the subsequent code block is executed. This is a fundamental example of the if
statement in action, showcasing its role in decision-making within programming using the Java language.
Furthermore, the progress of the programming language throughout the previous three decades has experienced noteworthy improvements, with the language adjusting to the requirements of contemporary development while upholding its fundamental features. As the programming language continues to evolve, newer features such as switch expressions have been introduced, offering developers more tools for writing clear and maintainable software. However, the simplicity of one-line 'if' statements remains a valuable asset for developers in the Java programming language, facilitating quick decisions and readable pieces of software.
In a constantly changing tech landscape, the ability of this programming language to remain relevant and cater to the needs of both seasoned professionals and those embarking on their development careers is testament to its enduring design and adaptability. While navigating the intricacies of Java programming, developers should always prioritize the principles of writing clear and sustainable software, making sure that even the most basic constructs like the 'if' condition are fully utilized.
One Line If Statements: Syntax and Usage
Conditional expressions, or one-liner if structures, are a streamlined method of implementing conditional logic in Java. These expressions eliminate the necessity of curly braces to enclose blocks of code, allowing the execution of a single statement dependent on a requirement being fulfilled. The syntax is simple and can be demonstrated as follows: criteria ?' expression_if_true: expression_if_false;
. This ternary operator evaluates the test
, and if it holds true, the expression_if_true
is executed; otherwise, the expression_if_false
is carried out.
Consider the scenario of managing various transportation methods in a delivery company. The strategy to select an appropriate vehicleβbe it a bicycle, motorcycle, or carβdepends on certain conditions, such as distance and connectivity. A concise and readable one-liner if condition beautifully encapsulates this decision-making process, enhancing maintainability and reducing complexity.
java
// Example of using a one-liner if statement for determining delivery mode
String deliveryMode = isShortDistance && isTownConnected ? "bicycle" : "motor vehicle";
In this example, isShortDistance
and isTownConnected
are boolean expressions that, when true, result in the selection of a bicycle for delivery. Otherwise, a motor vehicle is chosen. Itβs a practical illustration of how conditional expressions can simplify decision-making in programming, making it more accessible and easier to debug, an essential practice in software development. The adoption of such concise coding techniques aligns with industry observations, like those by Eleftheria, a seasoned Business Analyst, emphasizing the importance of clarity and simplicity in computer programming.
The Role of Braces in One Line If Statements
When writing, especially in Java, utilizing one line if conditions can simplify your programming, resulting in a cleaner and easier to read code. This technique is particularly helpful when you want to execute a small block of code based on a situation. For example:
java
if (condition) singleStatement;
In this structure, the absence of braces {}
indicates that only the immediately following statement is part of the if
condition. However, it's crucial to keep in mind that excluding braces can sometimes result in confusion and mistakes, especially when the single expression extends across multiple lines or when additional expressions are added later.
Employing braces to establish the scope of an 'if' block, even for a solitary expression, can improve legibility and manageability, which is in line with the idea that programming should be composed for human comprehension, not solely for computer execution.
java
if (condition) {
singleStatement;
}
The use of braces makes it unequivocally clear which statements are executed when the condition is met. This is particularly helpful as codebases grow and become more complex, and as more developers contribute to the same project.
Remember, the objective of writing computer programs is not to exhibit technical expertise with dense, cryptic one-liners, but to produce something that is maintainable, understandable, and robust. Throughout the past three decades, while the Java programming language has evolved, the fundamental principles of writing clear and maintainable software remain unchanged. As developers, we must consider the advantages of concise code versus the potential pitfalls of reduced clarity when choosing to use one line if statements without braces.
Ternary Operator: An Alternative to One Line If Statements
The ternary operator in Java serves as a succinct alternative to one-line if statements, offering a more compact syntax for conditional expressions. It operates on three operands, hence the name 'ternary', and provides a way to evaluate a Boolean expression, then return one of two values depending on the result. The format of the ternary operator is simple: 'expression? Expression_if_true: expression_if_false;. A real-world analogy can be drawn from a delivery company deciding on the mode of transportation: if a destination is accessible by road, use a motorcycle; otherwise, use a bicycle. Similarly, in programming, it might look like
String transportMode = isRoadConnected ?' "motorcycle" : "bicycle";, where
isRoadConnected` is a Boolean statement. Utilizing the ternary operator can result in neater and easier to understand logic, particularly in situations where actions are determined by straightforward conditions. Nevertheless, it's important to use this operator carefully to uphold clarity in programming, as excessively intricate ternary operations can be as confusing as double negatives in writing.
Best Practices for Using One Line If Statements in Java
One-line if statements in Java offer a succinct way to write conditional logic. However, to preserve the clarity and maintainability of your code, it's essential to employ them judiciously. The syntax of a one-line if, also known as a ternary operation, is straightforward: expression ?' 'expression_if_true : expression_if_false;
which evaluates the statement and executes the expression before the colon if true, otherwise, the expression after the colon is executed.'. When utilized correctly, one-line if statements can enhance the efficiency of your programming without compromising readability.
To maintain the comprehensibility of your programming, it is crucial to avoid using double negatives and excessively intricate conditions. Double negatives, much like in spoken language, can unnecessarily complicate the understanding of your logic. Strive for simplicity; your programming should be as easy to read as a book, free from the convolution that hinders readability and invites bugs.
Remember, programming is not just for computers to execute, but for humans to read and maintain. It's a common understanding among seasoned developers that simplicity and readability take precedence over showing off technical prowess. Thus, while it may be tempting to condense your programming into a clever one-liner, consider the future maintainers who will thank you for your clarity and foresight.
Here's an example to illustrate the use of a one-line if statement in a clear and readable way:
java
int max = (a > b) ? a : b;
This line concisely determines the maximum of two variables without introducing complexity. It's a practical application of a ternary operation that remains easily understandable. Embrace such practices, and your programming will not only work well but will also be a prototype of clean coding principles.
Common Pitfalls and Errors in One Line If Statements
One-line if statements in Java provide a concise way to execute actions based on a condition. Nevertheless, their misuse can result in reduced clarity in programming and increased potential for errors. A common issue arises with the use of double negatives -- a practice that can obscure the intention behind the code, making it harder for others to read, modify, or debug. Avoiding double negatives not only enhances readability but also contributes to a more maintainable and less error-prone codebase. Let's think about the effects of double negatives and the significance of clear conditional phrases in Java.
When crafting one-line if statements, it's essential to express the logic in the simplest and most direct form possible. For instance, instead of using a double negative like 'if (!is Not Valid)', it's cleaner and more understandable to use 'if (isValid)'. This direct approach prevents confusion and aligns with best practices in software readability, as emphasized in Rod Johnson's book on J2EE design and development. Additionally, complex business exceptions can clutter your codebase, leading to difficulties in maintenance and understanding. Maintaining a balance between conciseness and clarity is essential to ensure the robustness and security of your programming language, as emphasized by the JDK's focus on security.
In summary, while one-line if statements in Java can streamline your code, they should be used with care. Keeping conditions straightforward and avoiding double negatives can dramatically improve the comprehensibility and reliability of your applications.
Conclusion
In conclusion, the 'if' statement is a fundamental component of Java programming, allowing developers to execute code conditionally based on specific requirements. One-line 'if' statements provide a concise and readable way to implement conditional logic, simplifying code and enhancing maintainability. Using braces to define the scope of an 'if' block improves readability and makes codebases more manageable.
The ternary operator offers a compact syntax for conditional expressions, but it should be used judiciously to maintain code clarity. When using one-line if statements, it's important to follow best practices, such as avoiding double negatives and complex conditions, to ensure code intelligibility. By prioritizing simplicity and readability, developers can create maintainable and robust code.
Improve your code readability and manageability with Machinet's AI-powered plugin!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.