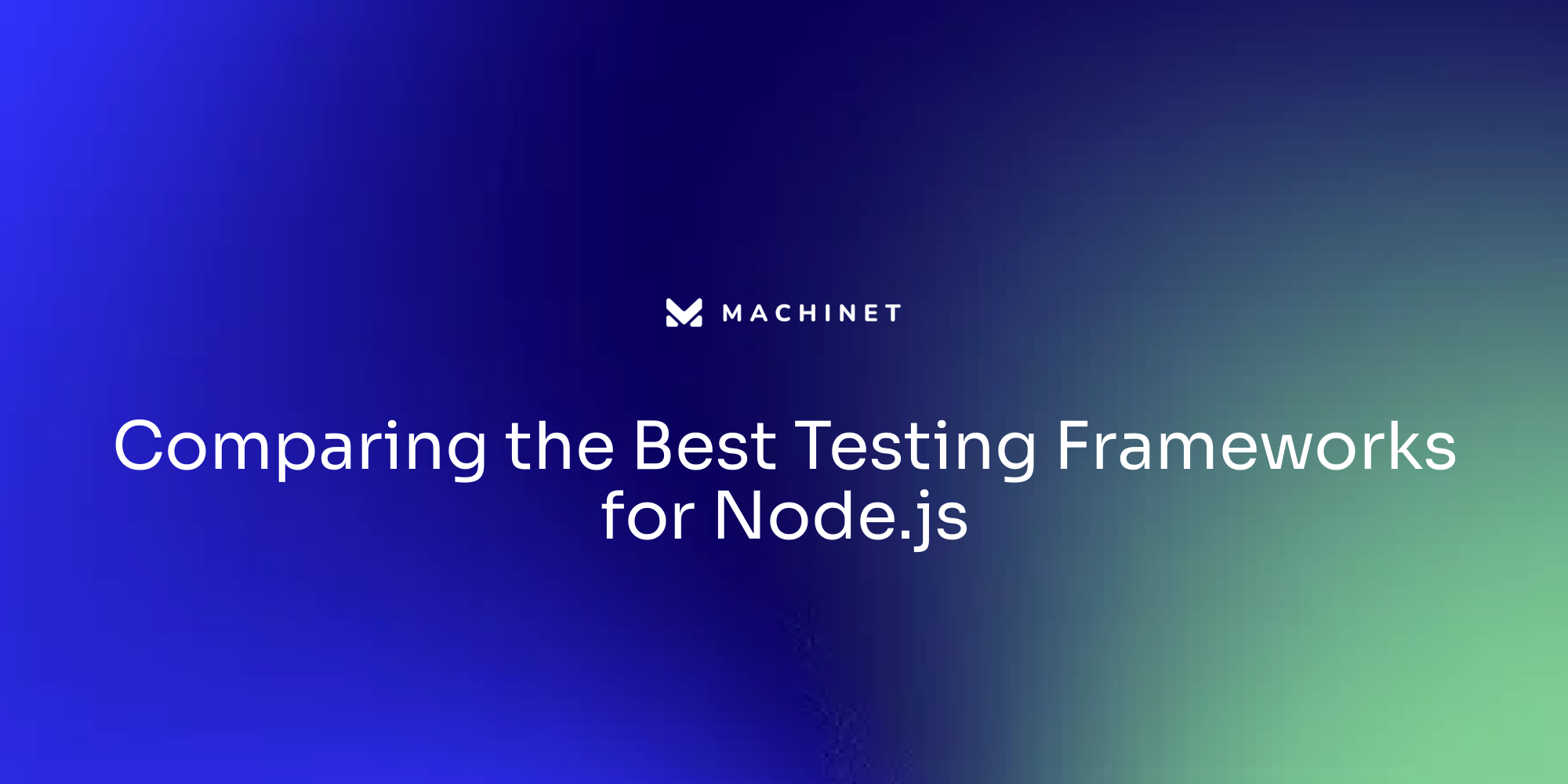
Table of Contents
- Understanding the Importance of Testing Frameworks in Node.js
- Overview of Popular Node.js Testing Frameworks
- Detailed Analysis: Chai
- Detailed Analysis: Testdouble
- Detailed Analysis: Istanbul
- Comparing Mocha, Ava and Jest for Unit Testing in Node.js
- Factors to Consider When Choosing a Node.js Testing Framework
- The Role of Automated Unit Testing in Streamlining Development Process
Introduction
Automated unit testing has become an essential practice in software development, providing developers with a safety net to catch bugs and ensure code stability. By integrating testing frameworks like Mocha, Ava, and Jest into the development process, teams can streamline their workflows, improve code quality, and minimize technical debt. These frameworks offer a range of features, from assertion libraries to mocking utilities and code coverage reporting. Choosing the right testing framework depends on factors such as project requirements, preferred testing paradigm, and the need for specific functionalities like concurrent testing or integrated mocking support.
In this article, we will explore the importance of automated unit testing in the Node.js ecosystem and compare popular testing frameworks like Mocha, Ava, and Jest. We will discuss the advantages and use cases of each framework, as well as considerations when selecting a testing framework for your Node.js projects. Additionally, we will delve into the role of code coverage tools like Istanbul in assessing the effectiveness of your tests and ensuring comprehensive test coverage. By understanding the benefits and capabilities of these testing frameworks, developers can enhance the reliability and efficiency of their Node.js applications
1. Understanding the Importance of Testing Frameworks in Node.js
The importance of testing frameworks in software development, particularly when dealing with an asynchronous and event-driven environment like Node.js, is a widely acknowledged fact among software engineers. These frameworks provide the necessary tools to ensure that the code functions as expected. An appropriate testing framework doesn't only streamline the process of writing tests, but it also generates insightful error messages and integrates smoothly with other tools for comprehensive code coverage reports.
The asynchronous nature of Node.js makes testing frameworks an essential tool. Asynchronicity can lead to complicated scenarios that are tough to test manually. Understanding the significance of writing tests for a Node.js application can be a daunting task, especially when the project's complexity increases. This is where testing frameworks prove to be beneficial.
Gaining knowledge from popular web frameworks and libraries enhances understanding of testing patterns, thereby boosting confidence in writing tests. Testing patterns such as object deep equal and snapshot testing are crucial for validating that the structure of objects or responses matches a known valid state. Asynchronous tests are particularly helpful in replicating real-world scenarios, making them a vital part of the testing process.
Over time, writing tests becomes a habit, and the perspective towards testing evolves. The code-test-fix loop becomes an integral part of development, saving significant time that would have otherwise been spent on debugging and fixing bugs. It also serves as an indicator of code complexityβif tests are difficult to write, it might suggest that the code is overly complex.
Among the various testing frameworks available, Jest is a standout. It is mature and offers extensive guides covering a wide range of common use cases. Moreover, Jest is supported by a large testing community and numerous libraries that extend its functionality. Two particularly useful testing patterns are mocking inner functions and mocking external dependencies. These patterns allow you to ensure that internal functions are being called by creating mock versions, and to test dependencies without actually running them using package mocks, such as Shelfjest MongoDB.
Adopting a testing framework in the development process is a game-changer, especially in an environment like Node.js. It simplifies the process, saves time, and increases efficiency. As a software engineer, it is advised to embrace the culture of testing and make it an integral part of the development process.
In the context of Node.js, several code coverage tools are available for testing, including Istanbul, nyc, and Jest. These tools help developers identify which parts of their code are being tested and provide insights into the overall test coverage. They can be used to generate coverage reports and highlight areas of code that are not adequately tested.
To test asynchronous code in Node.js, various techniques can be used. A common approach is to use callback functions or the Node.js built-in Promise object. Libraries like Mocha and Jasmine are also available, offering testing frameworks specifically designed for Node.js applications. These frameworks provide features such as support for asynchronous testing, test runners, and assertion libraries. The async/await syntax, introduced in newer versions of Node.js, can also be used to handle asynchronous code in a more synchronous manner
2. Overview of Popular Node.js Testing Frameworks
The Node.js ecosystem is teeming with testing frameworks, each with its distinct set of capabilities and constraints. Among the prevalent ones are Mocha, Jest, Ava, Chai, Testdouble, and Istanbul. These frameworks cater to a broad range of needs, offering everything from fundamental assertion libraries to comprehensive testing solutions. They are equipped with functionalities like mocking, stubbing, and code coverage. The choice of a framework is typically a matter of the team's specific needs and preferences.
Automated testing plays a pivotal role in software development, ensuring that the software behaves as expected and aids in detecting regressions. For instance, Mocha, a commonly used JavaScript testing framework, operates on Node.js and in the browser. Chai, on the other hand, is an assertion library that complements test frameworks like Mocha, making the writing of tests more straightforward. Both Mocha and Chai can be installed via npm, and there are numerous examples of tests using these frameworks available on GitHub.
Mocha structures tests using the describe
function, and individual tests are written inside the it
function. Mocha also provides hooks such as beforeEach
and afterEach
, allowing for the execution of setup and teardown tasks before and after each test.
Node.js also features a built-in test runner, empowering developers to write tests without depending on third-party libraries like Mocha, Jest, or Ava. The test runner searches for tests in a test directory or co-located with the code they are testing. Test files should have extensions ending in .js, .mjs, or .cjs. Tests can be written using the describe/it syntax or the test function. The test runner can be executed using the command 'node test'. Specific tests or test suites can be run using the "test only" flag or the only
method. Hooks such as before
, after
, beforeEach
, and afterEach
can be used for setup and teardown code.
When it comes to integrating Istanbul code coverage with Mocha, the istanbul-middleware package can be used. This package enables you to generate code coverage reports for your Mocha tests using Istanbul.
First, install the istanbul-middleware package by running the following command:
npm install istanbul-middleware --save-dev
Once the package is installed, you can include it in your test setup file or test runner script. You will need to configure the middleware to instrument your code and collect coverage data. Here's an example of how to set it up:
```javascript const istanbulMiddleware = require('istanbul-middleware');
// Configure the middleware istanbulMiddleware.hookLoader({ verbose: false });
// Start the coverage reporting istanbulMiddleware.createHandler({ // Specify the path where the coverage reports will be saved dir: './coverage', // Set the reporters you want to use (e.g., text, html, lcov) reporters: ['text', 'html', 'lcov'], }); ```
Once the middleware is configured, you can run your Mocha tests as usual. The coverage data will be collected and saved to the specified directory. You can then generate reports using the Istanbul command-line tools or any other tools that support Istanbul. It is crucial to note that this is just one way to integrate Istanbul code coverage with Mocha, and there may be other approaches depending on your specific setup and requirements.
Platforms like Endpts streamline the process of building and deploying Node.js apps, providing features like automatic preview deployments, serverless functions, CI/CD, multi-region deployments, and SSL certificates. This showcases the continuous evolution and versatility of the Node.js ecosystem, catering to the diverse needs of software development teams
3. Detailed Analysis: Chai
Chai stands out as an esteemed assertion library that is compatible with Node.js and browsers, serving as a valuable companion to any testing framework. Its API is straightforward and expressive, enabling developers to create tests that are easily understood and interpreted. Chai's versatility is another of its strengths, supporting both Behavior-Driven Development (BDD) and Test-Driven Development (TDD) styles. It provides a wide range of assertions, from simple equality checks to complex object property and type checks.
However, it's important to note that Chai, being an assertion library, does not inherently encompass functionalities like test runners or mocking utilities. These features require the integration of other tools. Chai's core strength resides in its assertion abilities, offering three primary APIs: assert, expect, and should. Each of these APIs presents its unique style for writing assertions, but the 'expect' interface generally gets the spotlight due to its readability and natural language assertions.
Chai offers an extensive assortment of getters and assertion methods, which can be chained together to create intricate assertions. This library is applicable to any JavaScript project, as exemplified in the courses provided by Applitools. These courses, powered by Add AI, demonstrate the use of Chai with Mocha and WebdriverIO. The library's capabilities, however, are not confined to these frameworks.
The courses focus on the practical application of Chai, such as integrating the library into existing projects and centralizing assertions. They delve into the differences between 'should', 'expect', and 'assert' styles, as well as their extras and configurations. The course content is open to all, only necessitating an IDE that supports JavaScript programming.
Chai's value is further accentuated by its compatibility with Mocha, a solid testing framework for Node.js. Mocha facilitates the execution of both synchronous and asynchronous code sequentially. The framework provides the 'describe' and 'it' methods for structuring and organizing test cases. It also includes hooks like 'before', 'after', 'beforeEach', and 'afterEach' for carrying out setup and teardown tasks required for tests, such as setting up database connections.
Executing Mocha tests is done using the 'mocha' command, with options like '-g' and '-f' available to run specific tests or patterns. The combined force of Chai and Mocha as testing tools enhances the robustness of Node.js projects, thereby improving the quality of software solutions.
While Chai itself does not include test runners or mocking utilities, there are several alternatives available for these functionalities. Libraries such as Jest, Sinon, and Jasmine are popular options that provide similar functionalities to Chai and can be used for test assertion and mocking in JavaScript unit testing
4. Detailed Analysis: Testdouble
The Testdouble library, designed with JavaScript and Node.js in mind, offers a user-friendly API for creating test doubles. These test doubles can be objects, functions, or modules that replace actual system components, making it easier to isolate the code being tested from the rest of the system. Additionally, Testdouble provides a variety of functions to create and manage these test doubles, such as spies, stubs, and mocks.
To create test doubles using Testdouble, you should first install the Testdouble library as a dependency in your project. Once installed, the necessary classes or functions from the Testdouble library can be imported into your test file. The library provides appropriate functions or methods to create the test double object, which could be a spy, stub, mock, or any other type of test double depending on your testing needs. Once the test double object is created, you can configure its behavior by setting up expectations or defining specific return values for method calls. This test double object can then be used in your test cases to simulate interactions with the real object being tested. Lastly, verify that the expected interactions with the test double object occurred during the test execution.
In the realm of unit testing, mocking dependencies is a common approach. TestDouble facilitates this through the use of stubs. Stubs are objects that provide predefined responses to method calls made during the test. Through the use of stubs in TestDouble, you can isolate the code under test from its dependencies, making the unit tests more focused and reliable. Stubs allow you to control the responses of the dependencies, making it easier to test different scenarios and edge cases.
Furthermore, TestDouble can be used in a variety of scenarios in JavaScript projects. For instance, in Stubbing, TestDouble can be used to create stubs, which are objects that provide predefined responses to method calls. This is useful when you want to simulate certain behaviors or control the output of a function during testing. It also allows for Spying, where TestDouble allows you to create spies, which are objects that record information about method calls, such as the number of times a method was called or the arguments passed to it. Spies are useful for verifying that certain methods were called or for gathering information about how methods are used. Lastly, Mocking is supported by TestDouble, which involves creating objects that mimic the behavior of real dependencies. Mocks can be used to simulate complex interactions or to test code that relies on external services or APIs.
When integrating TestDouble with popular testing frameworks, there are a few steps that need to be followed. Firstly, ensure that the testing framework you are using supports the use of TestDouble. Then, create your TestDouble objects and mock the necessary dependencies. Finally, integrate the TestDouble objects into your test cases and run your tests as usual. This approach allows you to isolate the code under test and control the behavior of the dependencies, making it easier to write thorough and reliable unit tests.
Beyond the technical aspects, understanding the purpose and functionality of a project is a crucial step before initiating it. Developers and end users often have varying perspectives and knowledge levels. Words can sometimes be inadequate to accurately convey ideas, especially in complex systems. This is where diagrams can provide clarity and answer questions more effectively. Simple diagrams can represent workflows and side effects in systems. They are useful for both builders and stakeholders of a system. As Pam Marie Guzzo, author and developer, eloquently states, "Creating a diagram doesn't just add clarity for the person doing the building, it's also an invaluable tool for the person asking for the system. A picture is worth a thousand words." This statement emphasizes the value of diagrams in the software development process
5. Detailed Analysis: Istanbul
Istanbul is an effective tool for analyzing code coverage in JavaScript. It integrates smoothly with various testing frameworks, offering a comprehensive view of how extensively your codebase is covered by tests. This tool is invaluable for identifying areas that may require further testing. Istanbul supports various coverage metrics, such as function, statement, branch, and line coverage, providing a nuanced view of code quality. However, it's worth noting that Istanbul alone cannot satisfy all testing needs and should be used in combination with other tools for test formulation and execution.
Software development often necessitates the use of multiple testing tools or frameworks to accommodate different types of tests within applications. IstanbulJS, a variant of Istanbul, enables the consolidation of test coverage data from various utilities into a single report. This provides a holistic view of your testing environment. The article outlines how to gather code coverage data from unit tests using Jest and from end-to-end tests using Cypress.
Collecting code coverage data from Cypress tests involves the use of IstanbulJS and the Cypress Code Coverage plugin. This process can encounter certain challenges. The article goes into detail about specific issues that can occur when trying to get the expected code coverage data from Cypress tests and the steps taken to troubleshoot and resolve these issues.
IstanbulJS has a CLI tool, NYC, that allows for the merging of code coverage data from Jest and Cypress into a single report. However, this process can run into problems, such as improper data merging, which can lead to inaccurate coverage percentages. To tackle this, the Lcov Viewer tool can be used as a reporter for NYC, generating a coverage report that is both accurate and easy to interpret.
To employ the Istanbul code coverage tool for JavaScript, you can follow these steps:
- Install Istanbul: Use a package manager like npm to install Istanbul globally by running the command
npm install -g istanbul
. - Instrument your code: Use the Istanbul command-line tool to instrument your JavaScript code. This will add tracking code to collect coverage information. Run the command
istanbul instrument [input files] --output [output directory]
to instrument your code. - Run your tests: Execute your JavaScript tests using a test runner like Mocha, Jasmine, or Karma. Make sure that your tests exercise all parts of your code.
- Generate coverage reports: After running your tests, use the Istanbul command-line tool to generate coverage reports. Run the command
istanbul report [report type]
to generate the desired type of report (e.g., HTML, text, or JSON). - Analyze the reports: Open the generated coverage reports to analyze the code coverage information. These reports will show you which parts of your code were covered by your tests and which parts were not.
The article concludes by emphasizing the importance of effectively using documentation, keeping third-party dependencies up-to-date, and proactively addressing updates. These practices are key to preventing potential issues in software projects and ensuring the delivery of high-quality software products. When it comes to troubleshooting issues with Istanbul, one possible solution is to check the configuration and setup of the tool. Make sure Istanbul is installed correctly and that you've followed the recommended guidelines for setting up code coverage in your JavaScript project. Additionally, consult the documentation and resources provided by the Istanbul team for troubleshooting common issues and resolving any compatibility issues with your specific JavaScript environment
6. Comparing Mocha, Ava and Jest for Unit Testing in Node.js
Selecting a suitable testing framework is a pivotal step in Node.js development. Mocha, Ava, and Jest are among the popular choices, each offering distinct advantages catering to diverse project requirements.
Mocha is a seasoned and adaptable framework, renowned for its abundant ecosystem of plugins and integrations. It endorses both Behavior-Driven Development (BDD) and Test-Driven Development (TDD), offering a synchronous API for script testing. With Mocha, the setup process is relatively straightforward and involves the installation of ts-node and configuration in the mocharc.json file. While it employs the assert assertion tool, developers seeking more versatility often pair it with Chai, an assertion library that extends test frameworks. To integrate Mocha with other plugins and tools, install the desired plugins or tools, and modify the Mocha configuration file to specify the required settings and dependencies for the integration.
Ava, a more recent framework, emphasizes simplicity and speed. Its standout feature is the ability to run tests concurrently, which can significantly expedite test times, particularly for larger codebases. Concurrent test execution in Java can enhance test times by enabling multiple tests to run simultaneously. This approach effectively utilizes available resources, thereby reducing the overall test execution time and providing quicker feedback on the quality of the tested code.
Jest, a product of Facebook, is an all-inclusive testing solution that bundles a test runner, assertion library, and mocking support. It seamlessly integrates code coverage reporting and a powerful watch mode for executing tests during coding. To get started with Jest, install it in your project's root directory, create a test file, and write your test cases using Jest syntax. Run the tests in your terminal, and Jest will automatically locate and execute all the test files in your project. While Jest offers its own matcher for added flexibility, it does not strictly adhere to semantic versioning, so updates should be undertaken with caution. Jest's code coverage reporting works by examining the executed code during test runs and offering a report showcasing which parts of the code were covered by the tests and which parts were not. This report aids developers in understanding how thoroughly their tests are examining their codebase. To enable code coverage reporting in Jest, include the --coverage
flag when running your tests. You can also configure Jest to include or exclude specific files or directories from the coverage analysis using configuration options in the jest.config.js
file. To effectively use Jest's mocking support, import the module or class you wish to mock in your test file, use the jest.mock()
function to mock the module or class, configure the behavior of the mock using the mockImplementation()
or mockReturnValue()
functions, write your test cases, and use the mocked module or class as needed. After running your test cases, use the jest.restoreAllMocks()
function to restore the original implementation.
Choosing amongst these frameworks requires careful consideration of your specific needs and preferences. For instance, Ava's concurrent testing might be beneficial for a large project that requires faster test execution. If you value a comprehensive solution with built-in features, Jest might be the better choice. If you prefer a framework that's easier to set up with a rich ecosystem, Mocha could be the right fit. Regardless of the framework you choose, it is essential to have a robust testing strategy in place, as testing forms an integral part of software development, ensuring program reliability and catching regressions
7. Factors to Consider When Choosing a Node.js Testing Framework
Determining an appropriate testing framework for Node.js can be a multifaceted task due to the plethora of options and varying perspectives within the developer community. The framework selection is largely contingent upon your codebase's scale and complexity, your favored testing paradigm (BDD or TDD), the need for features such as mocking and code coverage, and the framework's speed and efficacy.
Furthermore, the learning curve associated with the framework, coupled with the availability of a supportive community and accessible resources, are key factors to consider. An ideal testing framework is not a universally perfect solution, but one that meets your specific needs and aids in producing robust and dependable code.
The Node.js framework landscape is extensive, with options ranging from comprehensive, opinionated frameworks to minimalist ones that offer basic functionality while allowing for flexibility in structuring your application. Understanding the project's use case and requirements before evaluating frameworks is paramount. For instance, the framework choice may vary depending on whether you're developing a full-stack application or an API, focusing on server-side rendering, deploying serverless, or building real-time functionalities.
Modern Node.js frameworks should inherently support promises and async/await operations to ensure efficient and safe handling of asynchronous tasks. The quality and availability of documentation are significant as they can greatly influence your ability to navigate, search, and understand practical examples of the framework's usage.
The community and ecosystem associated with a framework are influential, providing support, resources, libraries, and plugins. A framework's popularity and package download counts can reflect its widespread adoption and usage within the community.
Assessing the health of a project is also important, looking at indicators such as regular releases, active issue responses, recent pull request activity, and multiple contributors. Tools like Snyk Open Source Advisor, GitHub Insights, and Moiva can be helpful in evaluating these aspects.
Lastly, while it may seem subjective, considering your personal enjoyment and satisfaction when using a framework can significantly impact your productivity and motivation. Selecting a Node.js framework can be complex, but with careful consideration of these factors, you can find the one that best suits your project's needs.
When it comes to improving the reliability of your Node.js code, testing frameworks play a critical role. They ensure that your code functions as expected and remains stable throughout its lifecycle. Numerous testing frameworks are available for Node.js, such as Mocha, Jest, and Jasmine, each with its own set of features and benefits. These frameworks provide tools for writing and running tests, making it easier to identify and rectify bugs before they impact your application. By integrating testing frameworks into your development process, you can enhance the overall quality and reliability of your Node.js code
8. The Role of Automated Unit Testing in Streamlining Development Process
Automated unit testing has become a cornerstone in the realm of software development, serving as a protective mechanism for developers as they refactor and alter code. With the assurance that any regressions will be promptly spotted by the tests, this methodology serves a dual purpose: it catches bugs and documents the expected behavior of the code. By integrating automated testing into the development cycle, teams can uncover and rectify bugs at an early stage, which helps to minimize technical debt and deliver high-quality software.
Speaking from personal experience, unit tests have proven their worth in preventing significant issues. A minor code modification that could have resulted in substantial production problems was avoided thanks to well-placed unit tests. This experience highlighted the preventive capabilities of unit tests and also demonstrated the time and effort saved by writing unit tests as opposed to manual testing and bug fixing. Unit tests provide a reliable method for verifying that new code is finished and functional, acting as a regression test to prevent the introduction of new bugs when modifying the code.
Unit tests also have an often overlooked role in documentation. They provide examples and instructions on how to use the code. Reviewing unit tests can offer insights into the understanding of the code and how it handles errors and edge cases. This encourages developers to think about the design and manage both positive and negative paths. While unit tests do not guarantee perfect code, they are a crucial tool in ensuring code quality and can reduce time and effort spent troubleshooting and fixing bugs.
However, unit tests can add complexity to the production code; this can be mitigated with proper design and dependency injection. Even writing unit tests for seemingly simple code can prove valuable as it can catch unexpected issues. Despite potential downsides, such as slower development and the need for maintenance, the return on investment for unit tests is high. The time saved by unit tests in identifying and fixing a race condition is invaluable.
Unit tests are not a cure-all solution, but they are a critical practice that enhances engineering performance in the long term. Tools like Machinet can enhance this process by automatically generating unit tests, saving developers' time and effort. This aligns with the Pareto principle in software engineering, where 80% of the results come from 20% of the efforts. The time and effort saved by automated testing can then be redirected towards delivering value to the end-users.
To automate unit testing with Machinet, the process begins with setting up a test suite that includes all the unit tests to be automated. Machinet's testing framework is then utilized to define and run unit tests. Individual test cases are written for each unit of code to be tested, covering all possible scenarios and edge cases. The tests are run using Machinet's testing framework, saving time and effort compared to manually running the tests. The results are analyzed to identify any failures or errors, which helps identify and fix any issues in the code. To further automate the unit testing process, Machinet is integrated with a continuous integration (CI) system, allowing the tests to run automatically whenever changes are made to the codebase.
Machinet also provides various resources related to unit testing, including blog posts on topics such as demystifying unit testing basics and benefits, as well as best practices for Java unit testing tips and techniques. Using Machinet for automated unit testing can lead to improved code quality and reliability, as bugs and errors can be identified early in the development process. Automated unit testing also aids with code refactoring and maintenance, providing a safety net to catch any regressions that may occur when making changes to the codebase. By using Machinet for automated unit testing, developers can save time and effort, ensuring the overall quality of their code
Conclusion
In conclusion, automated unit testing is an essential practice in the Node.js ecosystem that offers numerous benefits for software development. Testing frameworks like Mocha, Ava, and Jest provide developers with the necessary tools to catch bugs, ensure code stability, and streamline their workflows. These frameworks offer a range of features, from assertion libraries to mocking utilities and code coverage reporting. By integrating testing frameworks into the development process, developers can enhance the reliability and efficiency of their Node.js applications.
Furthermore, selecting the right testing framework depends on factors such as project requirements, preferred testing paradigm, and the need for specific functionalities. Each framework has its own advantages and use cases. For example, Mocha is a mature framework with a rich ecosystem of plugins and integrations. Ava excels at concurrent testing, which can significantly speed up test execution for larger codebases. Jest offers an all-inclusive testing solution with built-in features like mocking support and code coverage reporting. By carefully considering these factors and choosing the appropriate testing framework, developers can improve code quality, minimize technical debt, and deliver high-quality software.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Start automating your unit tests today
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.