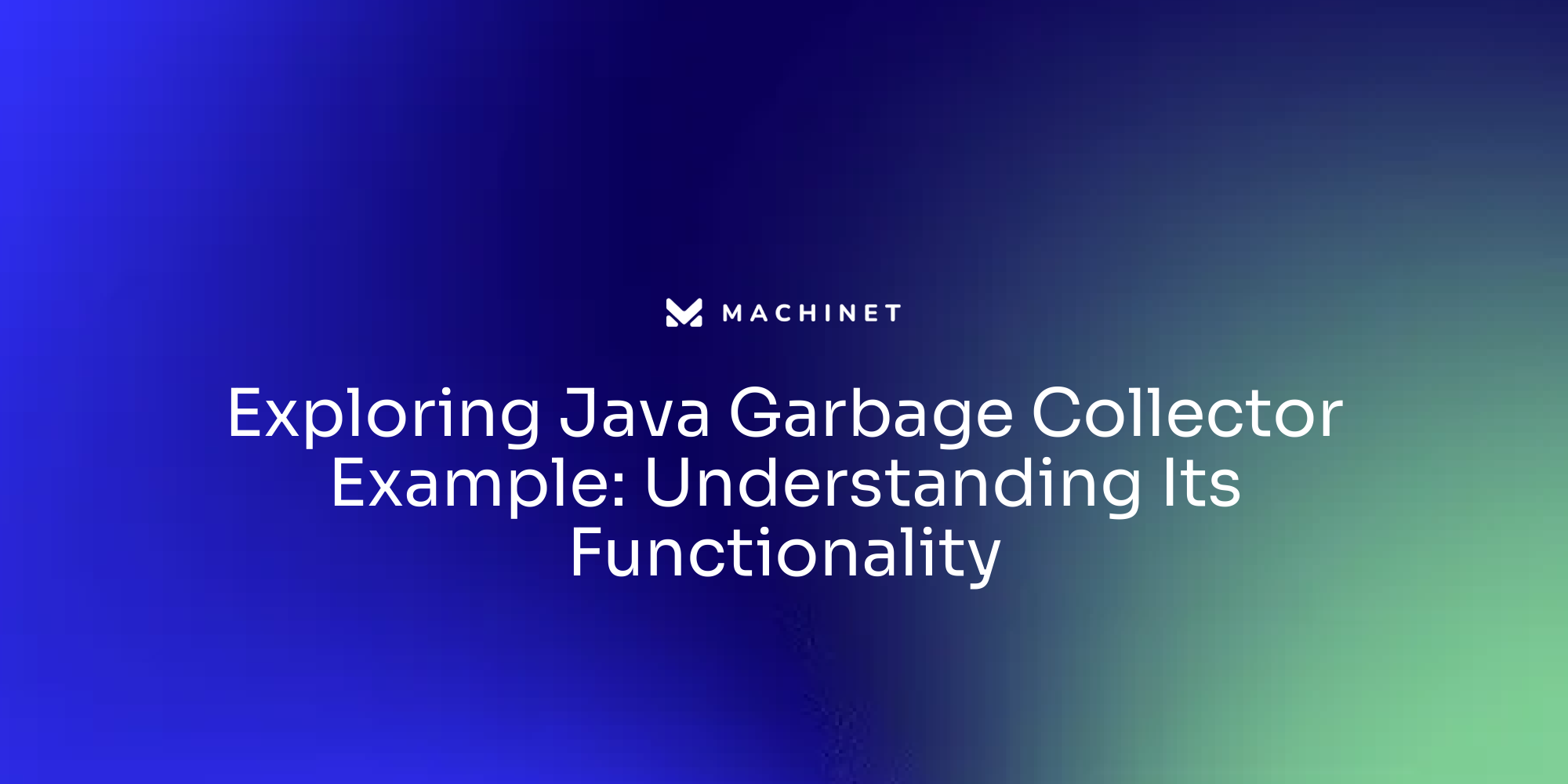
Introduction
Java's automatic memory management system, known as garbage collection (GC), plays a crucial role in enhancing application performance and efficiency. By identifying and disposing of objects no longer needed, GC prevents memory leaks and optimizes memory usage. This task, handled by the Java Virtual Machine (JVM), frees developers from the complexity of manual memory management, allowing them to focus on coding features.
The process involves several phases, including marking, deletion, and optional memory compaction, each contributing to efficient memory utilization. Additionally, Java employs generational garbage collection to further improve performance, dividing the heap into segments based on object lifespan. Various garbage collectors, such as Serial, Parallel, CMS, and G1, offer tailored solutions for different application needs.
Understanding and tuning garbage collection behavior, through profiling tools and JVM flags, is essential for maintaining optimal performance. Despite its benefits, GC can introduce performance issues, such as long pause times, which can be mitigated by best practices like minimizing object creation and selecting the appropriate garbage collector. Telemetry and observability tools provide real-time insights, enabling proactive performance management and elevating user experiences.
How Java Garbage Collection Works
Java employs an automatic resource management system known as garbage collection (GC) to enhance performance and efficiency. This process entails recognizing and eliminating items that are no longer required by the application, effectively preventing leaks and optimizing usage of resources. The Java Virtual Machine (JVM) manages this task by automatically allocating and freeing resources, eliminating the need for developers to handle resources directly. This method enables developers to concentrate on coding features instead of resource management, decreasing the intricacy and possible mistakes linked with manual resource handling.
The Garbage Collection Process
The waste removal procedure in Java comprises several unique stages that collaborate to handle resources effectively. These phases include identifying active objects and determining which ones are no longer needed and can be discarded. 'The significance of this process cannot be emphasized enough; it enables the Java Virtual Machine (JVM) to release resources, thereby enhancing performance.'.
Garbage collection automates resource management, a feature that significantly simplifies the development process. The JVM's waste collector assumes the duty of freeing up resources, which would otherwise necessitate meticulous manual oversight by programmers. This automatic data management is particularly crucial in large-scale distributed systems, where performance and resource optimization are essential.
A study titled 'The Cost of Waste Management for State Machine Replication' emphasizes that while waste management is resource-intensive, it is favored in contemporary programming languages because of its simplicity in development and scalability advantages. In spite of the expenses related to waste management, including memory and CPU utilization, its benefits render it a favored option for numerous businesses and startups.
Java’s method for memory management includes different tactics to reduce interruptions and latency. For instance, methods such as concurrent and parallel memory reclamation aim to minimize the effect on application performance. By managing memory automatically, Java allows developers to focus more on building features and less on manual memory management, making it a powerful tool in the software development lifecycle.
Marking: Identifying In-Use and Unused Objects
The first phase of waste collection is known as marking. During this stage, the collector (GC) traverses the entire object graph, beginning from root references such as local variables and active threads. The GC identifies and marks all reachable objects as in-use. Objects that cannot be reached are flagged for deletion in the subsequent phase. This process guarantees that only essential objects take up space, enhancing the overall effectiveness of the application.
Deletion: Removing Unused Objects
Once the marking phase is complete, the garbage collector moves on to deleting the unmarked objects. 'This phase is crucial as it frees up space occupied by objects that are no longer needed, ensuring that resources are available for new objects.'. Efficient resource management, such as this, is foundational for effective software development. It prevents resource leaks, which can result in increased usage, diminished system efficiency, and even application failures. By effectively overseeing data storage, the application can sustain peak functionality and prevent resource-related problems. As Zhiying Liang and other authors highlight in their paper, garbage collection costs resources but is essential for maintaining the health and performance of modern applications.
Compacting: Optional Memory Compaction
Fragmentation of storage can occur following the removal of objects, resulting in inefficient utilization of resources. The compacting phase addresses this by rearranging the remaining objects to fill in the gaps, thus enhancing allocation efficiency. This step not only prevents the fragmentation but also optimizes performance, reducing the time needed to allocate new objects. As mentioned in 'The Garbage Collection Handbook,' this compacting process is essential for maintaining optimal resource utilization and ensuring the system operates efficiently.
Generational Garbage Collection
Java utilizes an advanced generational memory reclamation strategy, which segments objects according to their lifespan to improve memory management efficiency. The heap is divided into three main areas: Young Generation, Old Generation, and Permanent Generation.
The Young Generation is where new objects are allocated and is further divided into Eden Space and two Survivor Spaces. Items that endure several cleanup processes in the Young Generation are elevated to the Old Generation, which contains long-lasting objects. The Permanent Generation, also known as Metaspace in newer Java versions, contains metadata required by the JVM, such as class definitions and method information.
This generational method enhances waste management by focusing efforts on the Young Generation, where most items are short-lived and rapidly become inaccessible. This emphasis on newly generated items greatly decreases the occurrence and length of memory cleanup interruptions, thus improving application efficiency.
Heap Structure: Young Generation, Old Generation, and Metaspace
Java's heap storage is divided into distinct sections that optimize performance and manage resources efficiently. The Young Generation is where new objects are initially allocated. This area is further split into Eden and two Survivor spaces. When items endure the initial cleanup rounds in the Young Generation, they are transferred to the Old Generation, which contains long-lived entities. Finally, Metaspace is used to store class metadata. This structured organization improves waste retrieval efficiency, particularly for short-lived objects, by concentrating on gathering and reclaiming memory from the Young Generation more frequently and swiftly.
Types of Garbage Collectors: Serial, Parallel, CMS, and G1
Java offers multiple memory management systems to address various application requirements, each with distinct advantages and compromises. The Serial GC is straightforward, making it suitable for single-threaded environments. 'Conversely, the Parallel GC utilizes several threads for memory management, improving efficiency in multi-threaded applications.'. The Concurrent Mark-Sweep (CMS) GC aims to reduce pause times, making it ideal for applications requiring low latency. Lastly, the Garbage-First (G1) GC provides a balanced approach, targeting both efficient operation and reasonable pause times.
Selecting the appropriate waste disposal system relies on the application's particular efficiency needs and resource utilization trends. For instance, real-time systems may benefit from the CMS GC due to its low-latency characteristics. Conversely, applications with high throughput demands might opt for the Parallel GC. The G1 GC is often favored for large heap sizes and when a balance between performance and pause times is necessary. Understanding these collectors' strengths and weaknesses allows developers to optimize memory management for their particular use case effectively.
Triggering Garbage Collection: System.gc(), Runtime.getRuntime().gc(), and Command-Line Options
Garbage disposal can be manually initiated using methods like System.gc()
and Runtime.getRuntime().gc()
. Furthermore, developers can adjust memory cleanup behavior using different command-line parameters when starting the JVM. For example, the -XX:+UseG1GC
option enables the G1 Garbage Collector, which is designed for applications that require low pause times. In spite of these features, it is typically advised to allow the JVM to manage waste removal automatically, since it is designed to make effective resource management choices on the fly. This automatic method assists in sustaining performance and minimizing the risk of leaks, ensuring that applications operate seamlessly without manual intervention.
Tuning Garbage Collection: Flags and Profiling Tools
Modifying different JVM settings and parameters is essential for enhancing memory management in Java applications. By adjusting these configurations, developers can affect the function of the waste collector, such as the size of various storage generations. 'Profiling tools are essential in this process, as they offer detailed insights into resource usage and garbage management efficacy.'. 'Java profiling, for instance, involves monitoring aspects like CPU usage, allocation of resources, method execution times, and thread behavior.'. This data helps identify potential bottlenecks, making it possible to make informed tuning decisions. Utilizing telemetry and observability can further improve management by providing real-time data gathering and a comprehensive understanding of system behaviors.
Common Issues and Best Practices
'Garbage collection, though a benefit for resource management, can sometimes lead to performance hiccups, notably long pause times.'. Developers can mitigate these issues by adopting several best practices. One effective strategy is to minimize object creation, thereby reducing the workload on the memory manager.
Utilizing weak references wisely can also assist, as they enable the cleanup process to recover resources more effectively. Selecting the right garbage collector for your application is crucial; different collectors have unique strengths and trade-offs, so choosing one that aligns with your application's needs can make a significant difference.
Regular monitoring and profiling of your Java application can provide invaluable insights into its runtime behavior. Profiling assists in examining CPU utilization, allocation of resources, method execution durations, and thread behavior. By identifying bottlenecks and inefficient memory usage, developers can make informed adjustments to improve efficiency.
Telemetry and observability, the dynamic duo of contemporary application management, provide profound insights into the internal workings of applications. Telemetry's real-time data gathering, coupled with observability's detailed grasp of system behaviors, guarantees not only swift identification and resolution of issues but also a proactive strategy to alleviating potential challenges. This synergy is transforming the landscape of application performance management, enabling developers to elevate user experiences to unprecedented heights.
Conclusion
Java's garbage collection system is a vital component in maintaining application performance and efficiency. By automating memory management, it alleviates the burden on developers, allowing them to concentrate on feature development rather than manual memory oversight. The garbage collection process is structured into distinct phases—marking, deletion, and optional compaction—each playing a crucial role in optimizing memory usage.
The implementation of generational garbage collection further enhances efficiency by categorizing objects based on their lifespan. This strategy minimizes interruptions and reduces the frequency of garbage collection pauses, which can be detrimental to application performance. Different types of garbage collectors, including Serial, Parallel, CMS, and G1, provide tailored solutions to meet various application needs, emphasizing the importance of selecting the right collector based on specific performance requirements.
While garbage collection offers significant benefits, it can also introduce challenges such as long pause times. Adopting best practices—like minimizing object creation and utilizing profiling tools—can help mitigate these issues. Additionally, proactive performance management through telemetry and observability ensures that applications run smoothly, elevating user experiences.
Understanding and tuning the garbage collection process is essential for developers aiming to achieve optimal application performance in a resource-efficient manner.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.