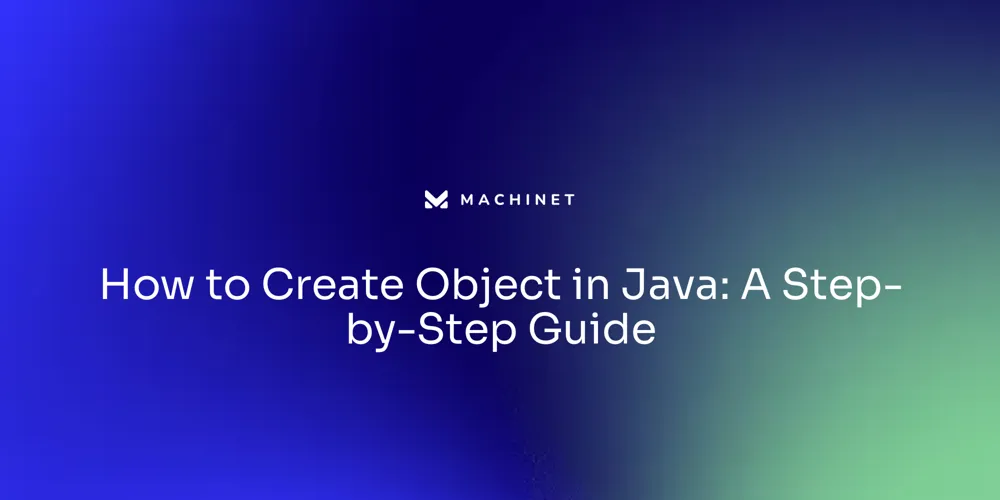
Table of Contents
- Understanding Classes and Objects in Java
- Different Ways to Create Objects in Java
- Example Programs for Object Creation
- Best Practices for Object Creation in Java
Introduction
Understanding Classes and Objects in Java
Java, an Object-Oriented Programming (OOP) language, relies heavily on classes and objects. Classes serve as user-defined data types, encapsulating data and behavior.
Objects, on the other hand, are instances of classes, embodying specific data and performing actions. This article explores the nature of classes and objects in Java, their relationship, and how they contribute to the functionality of a software program. Whether you're a beginner or an experienced Java programmer, understanding classes and objects is essential for building robust and maintainable code.
Understanding Classes and Objects in Java
Understanding the nature of classes and objects is fundamental in Java, an Object-Oriented Programming (OOP) language created by James Gosling in 1990. Classes in Java serve as user-defined data types that encapsulate data and behavior, similar to how a blueprint outlines the specifications for constructing a building.
For instance, envision a class as a category like 'Human', where each individual's traits and capabilities are akin to properties and methods of the class, respectively. When we talk about objects, we refer to them as instances of classes, embodying specific data and capable of performing actions.
These objects are like the building blocks of a software program, each with a unique state and behavior that contribute to the program's functionality. The process of creating an object from a class involves defining the class's variables and methods, which represent the object's state and behavior.
Following this blueprint, objects are instantiated, bringing the static class structure to life in a dynamic form. Java's syntax, influenced by C and C++, is designed with readability in mind, promoting a clean and organized coding style. This language not only simplifies software development but also its maintenance, thanks to the structured approach provided by classes and methods. The Java Development Kit (JDK) plays a pivotal role, offering the essential tools and libraries for Java application development. As a programmer, adhering to standard naming conventions is crucial for clarityβusing descriptive names like 'Customer' instead of ambiguous acronyms such as 'Ctr' makes the code more understandable and maintainable.
Different Ways to Create Objects in Java
Creating objects in Java is akin to assembling a structure with Lego blocks, where each block is a distinct entity with its own characteristics. For instance, consider a class as a blueprint for a house, with its properties being the rooms and its functions akin to the doors and windows that facilitate interaction. When you instantiate a class, you're essentially building a house with those specific features.
-
The
new
keyword is the standard tool for object creation, much like using a base Lego block. This approach involves invoking the constructor, which sets the foundation for your object, allocating memory for it in the process. -
Dynamic object creation can be achieved with the
Class.forName()
method, where you specify the class's full name. It's a bit like choosing a Lego block based on a picture; this method is particularly useful when you need to construct objects based on external inputs or settings. -
The
newInstance()
method of theConstructor
class allows for object creation without directly invoking the constructor, offering a more flexible building process, similar to using a special tool to modify your Lego block into the desired shape. 4.
To replicate an existing object, the clone()
method comes in handy, comparable to copying a Lego structure you've already built to create another one just like it, with the same initial state. 5. Lastly, deserialization is the method of reconstructing an object from its serialized state, akin to reassembling a Lego masterpiece from a set of stored instructions, allowing you to restore an object from a file or network stream.
Example Programs for Object Creation
In Java, the creation of objects is a cornerstone of Object-Oriented Programming (OOP), akin to assembling a structure using various blocks. Each object can be seen as an independent block, much like a Lego piece, with its own properties and behaviors. To instantiate these objects, different methods can be employed, each suited for specific scenarios.
Using the 'new' keyword is the most straightforward way to create an object. It's like saying, 'Here's a blueprint (class), now make me a house (object)!' For instance:
```java class Car { String color; int price; public Car(String color, int price) { this.color = color; this.price = price; } }
Car myCar = new Car("Red", 20000); ```
Alternatively, you can use 'Class.forName()' when the class name is dynamic or not known at compile time:
java
String className = "com.example.MyClass";
Class<?> myClass = Class.forName(className);
Object myObject = myClass.newInstance();
For cases where you need to specify constructor parameters, 'newInstance()' method of the 'Constructor' class can be utilized:
java
Constructor<Car> constructor = Car.class.getConstructor(String.class, int.class);
Car myCar = constructor.newInstance("Blue", 25000);
To create a duplicate of an existing object, 'clone()' method is used, which requires the class to implement 'Cloneable' interface:
```java class Person implements Cloneable { String name; public Person(String name) { this.name = name; } @Override protected Object clone() throws CloneNotSupportedException { return super.clone(); } }
Person person1 = new Person("John"); Person person2 = (Person) person1.clone(); ```
Lastly, deserialization is a technique to create objects from a stream of bytes, typically used when retrieving them from storage or over a network:
java
try (FileInputStream fileIn = new FileInputStream("object.ser");
ObjectInputStream in = new ObjectInputStream(fileIn)) {
Object myObject = in.readObject();
// Do something with the object
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
Best Practices for Object Creation in Java
In Java, crafting objects is akin to laying the foundation for a building; it requires precision, understanding, and adherence to best practices to ensure the edifice is sound. To begin with, employ the new
keyword for creating objects; it's the most uncomplicated and widely accepted technique.
However, exercise caution with the newInstance()
method of the Constructor
class; its complexity may invite errors. Ensure compliance with the Cloneable
interface and proper overriding of the clone()
method when using cloning.
When it comes to Deserialization
, tread carefully as it can be a gateway to security vulnerabilities. Naming conventions are your compass in the realm of code; they guide you through the labyrinth of your codebase.
Opt for simple, descriptive class and object names over enigmatic acronyms; 'Customer' is far more intuitive than 'Ctr', which could signify anything from 'contract' to 'control'. In the tapestry of software development, design patterns like Singleton or Factory are not mere threads but the weft that holds the fabric together. They are the embodiment of collective wisdom, distilled from years of problem-solving in the software design domain. Embrace these patterns judiciously to construct code that is not only maintainable but thrives on reusability and scalability. By observing these practices, you pave the way for your Java objects to be robust, maintainable, and a testament to high-quality software craftsmanship.
Conclusion
Understanding classes and objects in Java is crucial for building robust and maintainable code. Classes encapsulate data and behavior, while objects embody specific data and perform actions. Java's syntax promotes clean coding, making development and maintenance easier.
Different methods can be used to create objects in Java. The new
keyword is the standard tool, allowing you to invoke the constructor and allocate memory. Dynamic object creation can be achieved with the Class.forName()
method, while the newInstance()
method offers a flexible building process.
The clone()
method replicates existing objects, and deserialization reconstructs objects from serialized state. Following best practices is crucial when creating objects in Java. Use the new
keyword for simplicity, exercise caution with complex methods like newInstance()
, and ensure compliance with the Cloneable
interface when using cloning.
Naming conventions enhance code readability, favoring descriptive names over acronyms. Design patterns like Singleton or Factory promote maintainability and reusability. By understanding classes and objects in Java and following best practices, you can create robust code that showcases high-quality software craftsmanship.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.