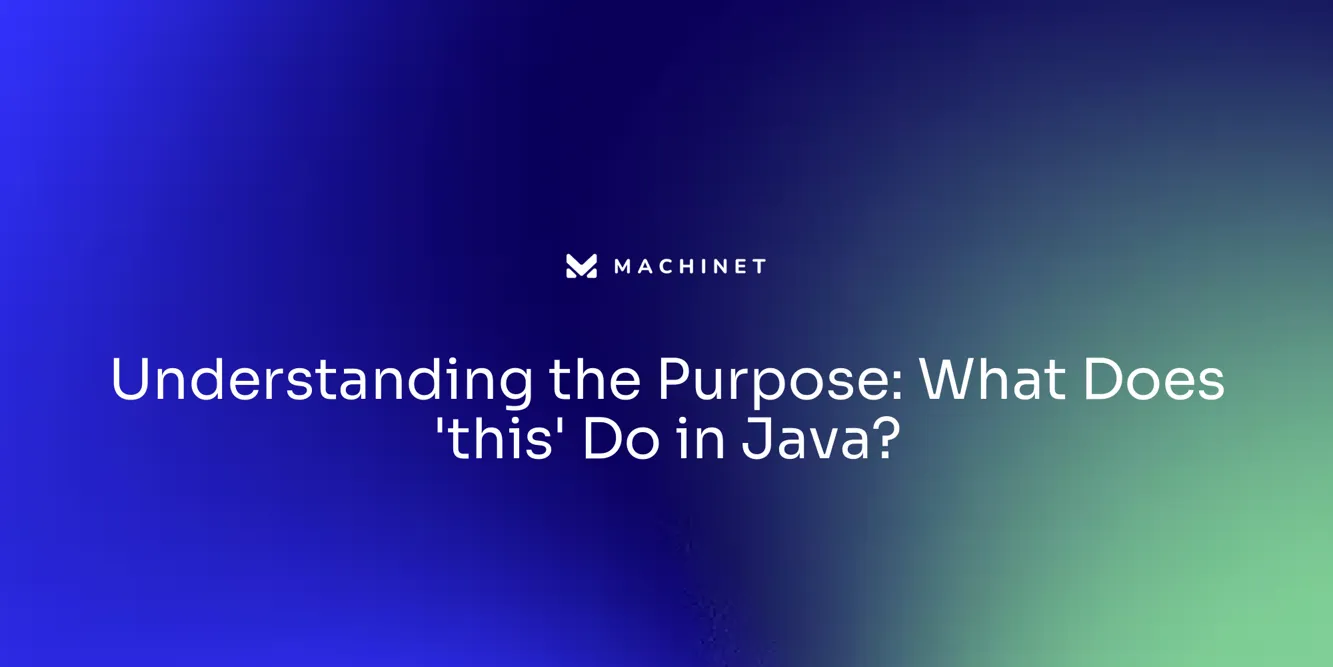
Table of Contents
- The 'this' Keyword in Java
- Accessing Instance Variables
- Invoking Methods
- Passing the Current Object as a Parameter
Introduction
The 'this' keyword in Java serves as a self-reference to the object currently under operation. It allows for differentiation between instance variables and local variables with the same name.
This article explores the role of the 'this' keyword in Java, its importance in writing efficient code, and its application in accessing instance variables and invoking methods. Understanding the use of the 'this' keyword is fundamental to mastering Java syntax and semantics, enabling developers to write more efficient and correct code.
The 'this' Keyword in Java
In Java, the keyword 'this' serves as a self-reference to the object currently under operation. It comes into play when we need to distinguish between instance variables of the object and local variables sharing the same name.
Every object created from a class has its unique set of instance variables, and 'this' allows differentiation among them. Think of 'this' as a GPS inside your code, pointing to the current object's location.
Its value may change dynamically, depending on the context it is used in. For instance, within a method, 'this' refers to the object that owns the method.
The 'this' keyword, although a cornerstone of Java, might initially seem confusing to developers, especially those new to the language. However, its understanding is crucial in writing efficient and clear code. It's noteworthy that Java, since its inception in 1995, remains one of the top three most-demanded programming languages. Its versatility across various applications, from web and mobile app development to enterprise systems, continues to win hearts of developers worldwide.
Accessing Instance Variables
The 'this' keyword in Java serves a crucial role in distinguishing between instance variables and parameters or local variables that share the same name. It points to the current object instance within an instance method.
For example, in the Dog class, instance methods like bark and age One Year can manipulate the instance variables name and age of the Dog objects they are invoked on, such as my Dog and another Dog. This encapsulation of behavior related to an object's state is a powerful feature of Object-Oriented Programming (OOP) in Java.
To illustrate, consider a Person class defined by properties like name and age along with methods such as walk() and talk(). This class acts as a blueprint for creating objects or instances of people like John and Mary.
The 'this' keyword helps these instance methods to interact with and modify the state of their respective objects. In the context of non-static methods, 'this' is used to invoke instance methods on an object of the class. For instance, if an instance method is declared without the static keyword, it can only be invoked on an instance of the class using dot notation. The 'this' keyword, therefore, plays a pivotal role in managing the state of an object and its behavior within the class it belongs to.
Invoking Methods
The 'this' keyword in Java is a reference variable that refers to the current object, providing a way to access the object's variables and methods. It's particularly useful when you need to call a method from another method within the same class. For instance, 'this.methodName()' can be used to invoke a method defined in the current class.
Consider the 'HelloWorld' code, a simple yet crucial execution for beginners, which prints 'Hello World' on the console. In Java, this involves writing a class with a main() method, marking the entry point of execution. The 'this' keyword can be used in this context to refer to the current instance of the class, distinguishing between instance variables and parameters with the same name.
Interestingly, with the introduction of Java 21, the process of printing a message to the console has been simplified. Now, you can define a source code file without having to write an explicit class, bringing a sense of simplicity and conciseness to the code. This change can help developers focus more on essential coding aspects rather than unnecessary details.
When it comes to naming conventions, it's worth noting that it's best to use verbs for methods (e.g., calculate Total Price()) and nouns for variables (e.g., totalPrice). Avoiding cryptic abbreviations and overly short names can also help prevent confusion and make the code more understandable. In conclusion, understanding the use of the 'this' keyword is fundamental to mastering Java syntax and semantics, enabling you to write more efficient and correct code.
Passing the Current Object as a Parameter
The 'this' keyword in Java is a powerful tool, often utilized to refer to the current instance of a class. Its use becomes particularly critical when differentiating between instance variables and parameters sharing identical names.
Instance methods, essentially non-static, are typically invoked within the context of an object and are incapable of being called without creating a class instance. A fascinating application of the 'this' keyword is when it's used as an argument to invoke another method, consequently supplying the current object as a parameter.
This practice enables the method to access and manipulate the object's instance variables and methods. However, it's crucial to remember how references are passed in Java.
When a primitive type is passed, a copy of its value is transferred to the method, leaving the original variable unaffected. But when an object reference is passed, the reference's value (memory address) is duplicated, not the object itself.
Consequently, while the object's properties can be modified within the method, the reference itself remains unaltered. In the interest of accurate communication, understanding the difference between 'passing by value' and 'passing by reference' is essential. Furthermore, when passing objects, it might be wise to consider making them immutable to avoid unintended modifications. Java's parameter passing behavior is often a topic of debate, but understanding these nuances is fundamental to mastering the language. Remember, the 'this' keyword isn't static and its value depends on the context in which the function is called. Using 'strict mode' can help catch potential issues and make the behavior of the 'this' keyword more predictable.
Conclusion
The 'this' keyword in Java is essential for distinguishing between instance variables and local variables, enabling efficient code writing. It acts as a GPS within the code, pointing to the current object's location.
Understanding 'this' is fundamental for mastering Java syntax and semantics. In accessing instance variables, 'this' allows instance methods to interact with and modify the state of their respective objects.
This encapsulation of behavior is a powerful feature of OOP in Java. When invoking methods, 'this' simplifies calling a method from another method within the same class, improving code organization.
Furthermore, when passing the current object as a parameter, 'this' enables methods to manipulate an object's instance variables and methods. Understanding how references are passed in Java is crucial here.
Mastering the use of 'this' is essential for writing efficient and correct Java code. By utilizing it effectively, developers can write maintainable and robust applications. In conclusion, the 'this' keyword plays a pivotal role in Java programming. Its proper utilization leads to efficient and correct code writing. By understanding its importance in accessing instance variables and invoking methods, developers can become proficient in Java syntax and semantics, enabling them to write high-quality code.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.