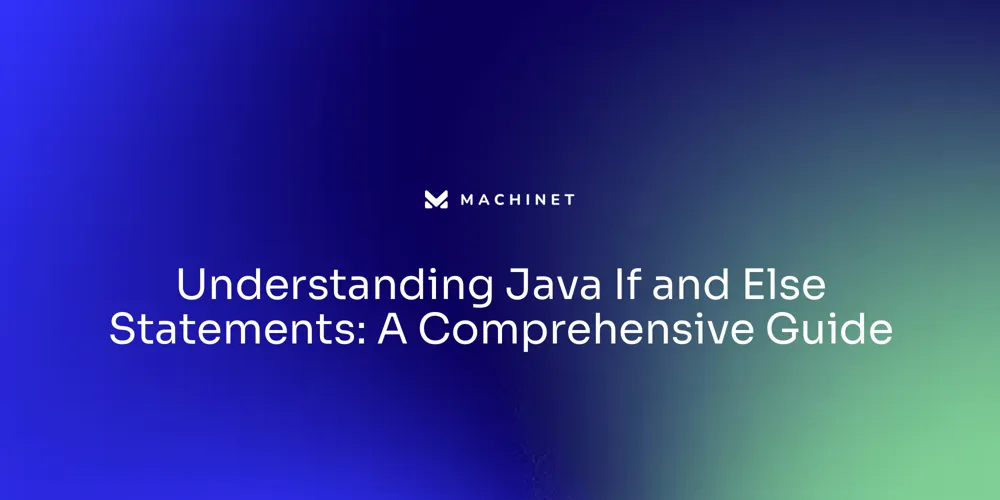
Table of Contents
- What is an If Statement in Java?
- Syntax of the If Statement
- Understanding the Else Statement
- Syntax of the Else Statement
Introduction
The article introduces the concept of the if
and else
statements in Java, which are essential control structures for decision-making in code. It explains the syntax and semantics of these statements, highlighting their importance in creating flexible and adaptable applications.
The article also discusses the recent advancements in Java, such as pattern matching and sealed classes, and emphasizes the significance of staying up-to-date with the latest Java developments. Additionally, it touches on Java's exception handling mechanism and how the else
statement plays a role in error handling. Overall, this article provides clear and concise information to the target audience about the usage and benefits of if
and else
statements in Java programming.
What is an If Statement in Java?
In Java, the if
statement is a fundamental control structure that enables selective execution of code blocks based on boolean conditions. For instance, consider a scenario where you're enhancing your Java application with a new feature.
Instead of deploying two separate versions for testing, you could utilize a feature toggle—a runtime variable that activates or deactivates the new functionality. This approach streamlines the testing process without the need for multiple deployments.
Here is the basic syntax for an if
statement in Java:
if (condition) {
// Code to execute if the condition is true
}
The if
keyword initiates the statement, followed by a condition enclosed in parentheses, which should evaluate to either true or false. If the condition is met, the subsequent code block within curly braces {}
executes.
This structure is not only crucial for decision-making in code but also enhances readability and maintainability. Recent advancements in Java, like pattern matching and sealed classes, further simplify operations on data structures, making code more intuitive. In the context of the ever-evolving Java ecosystem, the Eclipse Foundation and Red Hat have released new versions of their libraries, incorporating improvements that affect how developers write and manage Java code. For example, Quarkus 3.5.3 introduces changes that maintain input order and ensure proper authentication sequences, showcasing the importance of keeping up-to-date with the latest Java developments.
Syntax of the If Statement
Understanding the semantics of Java's conditional statements is paramount for creating flexible and adaptable applications. Take, for example, the humble if
statement, which serves as a cornerstone for decision-making in code.
In its simplest form, the syntax is straightforward:
java
if (condition) {
// code to be executed if the condition is true
}
The condition within the parentheses must result in a boolean value, either true
or false
, to determine the execution path. This binary decision-making enables applications to react dynamically to varying scenarios.
Consider a weather application that suggests different outdoor activities based on the current temperature. By utilizing an if
statement, the program can decide to recommend a warm jacket for chilly days or sunscreen for sunny weather, thereby enhancing user experience through conditional execution.
In the evolving landscape of Java, it's important to note that features and syntax may undergo changes across versions. For instance, when Java 12 introduced Switch Expressions in preview, it used the keyword break
for returning values in cases, which was later replaced with yield
in subsequent versions.
Such changes exemplify the ongoing refinement of Java's syntax and semantics, ensuring that developers have access to more expressive and concise language constructs. As highlighted by the release of Java 20, the language continues to evolve with features like pattern matching for switch expressions, underscoring the importance of staying current with Java's latest capabilities. By embracing these syntactical tools, developers can write code that is not only functional but also clear and maintainable. Replacing magic numbers and strings with named constants, for example, final String ADMIN_ROLE = "admin";
, greatly improves readability and self-documents the code. This practice ensures that when values need updating, the change is centralized, minimizing the risk of inconsistencies and bugs. Such attention to detail in writing code reflects the findings that refactoring is a prevalent and impactful practice, as it enhances code quality and simplifies the introduction of new features, contributing to a more agile development process.
Understanding the Else Statement
Java's robust exception handling is a cornerstone of its syntax and semantics, allowing developers to manage runtime errors effectively. When an unexpected event, known as an exception, occurs, it disrupts the normal flow of a program. These exceptions are objects thrown at runtime and can be intercepted by catch blocks.
Without proper management, exceptions can lead to the abrupt termination of applications. Java categorizes exceptions into checked and unchecked exceptions, each requiring different handling strategies. This mechanism not only addresses runtime issues but also enhances the application's reliability.
For instance, if an else statement is used in conjunction with an if statement, it executes a block of code when the if condition evaluates to false. This conditional logic is fundamental in error handling where the program has to decide between a normal operation and an exception handling routine. Understanding the interplay between if-else statements and exception handling is crucial for junior developers to debug effectively and maintain a smooth application flow.
Recent advancements in Java, like the anticipated Jakarta Data 1.0.0 in Jakarta EE 11, and updates to libraries such as Helidon 2.6.2 and Hibernate Reactive 2.0.3, continue to refine these aspects. With the release of Java 20, developers are introduced to features like structured concurrency and pattern matching for switch statements, which further empower them to write cleaner, more intelligent code. These enhancements demonstrate Java's commitment to evolving its syntax and semantics to meet modern development challenges, making it a language that not only copes with the present needs but also anticipates future requirements.
Syntax of the Else Statement
In Java, conditional logic is fundamental, and the else
statement is a key part of this. It allows programmers to execute a block of code only when a certain condition is not met. Here's a closer look at its structure:
java
if (condition) {
// code to be executed if the condition is true
} else {
// code to be executed if the condition is false
}
When the condition in the if
statement evaluates to true, the code within the first pair of curly braces runs.
Conversely, if the condition is false, the code inside the else
block is executed instead. This binary decision-making aligns with real-world scenarios, such as determining the type of vehicle for deliveries based on the city's size, or sorting vehicles into appropriate parking slots in a parking lot system. Pattern matching in Java further enhances this by allowing values to be matched against specific patterns, making code more intuitive and less cluttered with manual checks and assignments.
It's a feature that has evolved over time and, as per the latest news, continues to be refined in Java Development Kit 23 (JDK 23). Pattern matching allows developers to work with complex data structures more efficiently, leading to cleaner and more maintainable code. As Java evolves, it remains a language of choice for many, with its popularity reflected in search engine queries and online resources, indicating its wide use and the value of understanding its syntax and semantics.
Conclusion
The article provides a comprehensive understanding of the if
and else
statements in Java programming. It explains the syntax and semantics of these control structures, highlighting their importance in decision-making and enhancing code readability and maintainability.
The article emphasizes the significance of staying up-to-date with the latest advancements in Java, such as pattern matching and sealed classes. It showcases how these advancements simplify operations on data structures and improve code intuitiveness.
Furthermore, the article discusses Java's exception handling mechanism and how the else
statement plays a role in error handling. It emphasizes the importance of understanding the interplay between if-else statements and exception handling for effective debugging and maintaining smooth application flow. Overall, this article reinforces the message that by utilizing if
and else
statements effectively, developers can create flexible and adaptable applications that are clear, maintainable, and reliable. Staying current with Java's latest capabilities is crucial to leverage new features and enhance code quality.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.