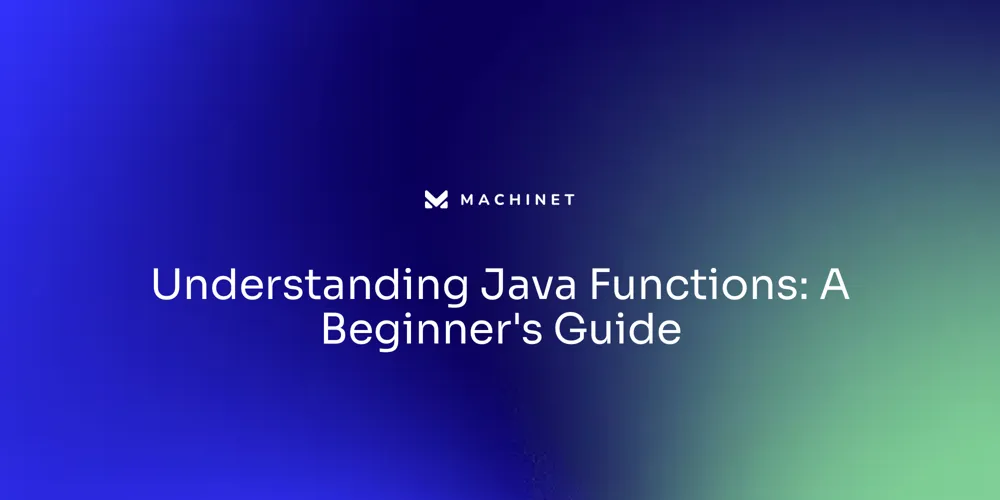
Table of Contents
- Defining Functions in Java
- Calling Functions in Java
- Common Types of Java Functions
Introduction
Java, a language renowned for its robustness and versatility, is used extensively across various applications. A core aspect of Java programming is defining functions within a class.
This article will explore the syntax and components of function declaration in Java, as well as the role of lambda expressions in functional programming. Additionally, it will discuss the importance of calling functions in Java and the different types of functions that can be created. Whether you are a beginner learning Java or an experienced developer looking to deepen your understanding, this article will provide clear and concise information to help you master the art of defining and calling functions in Java.
Defining Functions in Java
Java, a language renowned for its robustness and versatility, is used extensively across various applications. A core aspect of Java programming is defining functions within a class. The general form for declaring a function is as follows:
java
<access_modifier> <return_type> <function_name>(<parameter_list>) {
// Implementation code
return <return_value>;
}
Each part of this syntax plays a vital role:
<access_modifier>
controls the visibility scope of the function, with options like public, private, or protected.
For instance, public methods are accessible from any part of the program, much like a public building's open entrance, whereas private methods are akin to a private club, restricted to the class they belong to. - <return_type>
indicates the data type of the result the function will return, or void
if there is no return value. - <function_name>
should be descriptive, conveying the function's purpose.
<parameter_list>
includes input parameters the function accepts, with each parameter having a specified type and name. - The implementation code contains the logic to be executed by the function. -return <return_value>;
is used to output the result if the function is not of typevoid
.
In the context of functional programming, Java's introduction of lambda expressions since Java 8 has significantly streamlined the creation of functions. Lambdas act as concise anonymous functions, adhering to the principles of functional interfaces like Consumer or Function, which facilitate behaviors like callbacks or function chaining. This evolution of Java syntax and semantics, alongside upcoming enhancements like record patterns in Java 21, reflects the language's continuous adaptation to modern programming paradigms.
Calling Functions in Java
Invoking a function in Java is a fundamental operation that breathes life into code, allowing for modular, reusable, and organized programming. For instance, consider the function calculate
, designed to process numerical inputs.
When you wish to leverage this function, you invoke it with the required parameters like this:
java
int result = calculateSum(5, 3);
The calculateSum
function is called upon with two arguments, 5
and 3
, which are processed to produce a sum. The function then diligently returns the computed value, assigned here to the variable result
.
This encapsulation of functionality not only reduces code redundancy but also enhances clarity and maintenance. Functions are akin to machines in a factory line, each with a distinct role, working in unison to deliver a desired outcome. As Oracle updates Java biannually, with the latest being JDK 23, the language continues to evolve, embracing new features such as the Vector API to optimize its performance across diverse CPU architectures. This relentless innovation, dating back to Java's inception in 1995, ensures that functions and method calls remain integral to Java's syntax and semantics, facilitating developers in crafting efficient and robust applications.
Common Types of Java Functions
Java, a language at the forefront of innovation, allows developers to create a variety of function types, each suited to specific use cases. Functions in Java can be categorized mainly based on their parameter and return value characteristics.
- Functions without parameters or return values are often used for simple tasks such as displaying messages. An example is a straightforward function that prints a greeting to the console:
```java public void sayHello() { System.out.println("Hello!
"); } ```
- Functions that accept parameters but do not return values can perform actions using the provided inputs. For instance, greeting a user by name would be handled by a function like:
```java public void greet(String name) { System.out.println("Hello, " + name + "!
"); } ```
- Functions that take parameters and also return a value enable complex calculations and data processing. An example is a function designed to calculate the sum of two integers:
java
public int calculateSum(int a, int b) {
return a + b;
}
- Functions with multiple parameters are capable of dealing with more complex data structures, such as arrays. A function to calculate the average of an array of numbers demonstrates this capability:
java
public double calculateAverage(double[] numbers) {
double sum = 0;
for (double num : numbers) {
sum += num;
}
return sum / numbers.length;
}
These diverse function types are essential tools in a Java developer's arsenal, enabling the creation of robust and efficient applications. They serve as building blocks for complex operations and are integral to solving algorithmic problems, such as determining if a number is a 'Happy Number'—a concept that, while seemingly abstract, has practical applications in computer science and Java programming.
Conclusion
In conclusion, defining functions in Java is crucial for creating efficient and robust applications. The syntax for declaring functions includes components such as access modifiers, return types, function names, parameter lists, implementation code, and return values.
This allows for control over visibility, data types, purpose conveyance, input parameters, logic execution, and result output. The introduction of lambda expressions in Java 8 has streamlined the creation of functions in functional programming.
Lambdas act as concise anonymous functions adhering to functional interfaces like Consumer or Function. This reflects Java's continuous adaptation to modern programming paradigms.
Calling functions in Java is fundamental for modular and organized programming. By invoking functions with parameters and capturing return values, developers can encapsulate functionality and improve code clarity and maintenance.
Functions remain integral to Java's syntax and semantics as the language evolves with biannual updates. Java supports various function types based on parameter and return value characteristics. These include functions without parameters or return values for simple tasks, functions with parameters for actions using inputs, functions with parameters and return values for complex calculations, and functions with multiple parameters for dealing with complex data structures. Mastering the art of defining and calling functions in Java is crucial for creating efficient applications. Functions serve as essential building blocks for solving algorithmic problems and facilitating operations in Java programming. With continuous innovation and adaptation to new features, Java remains at the forefront of programming languages.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.