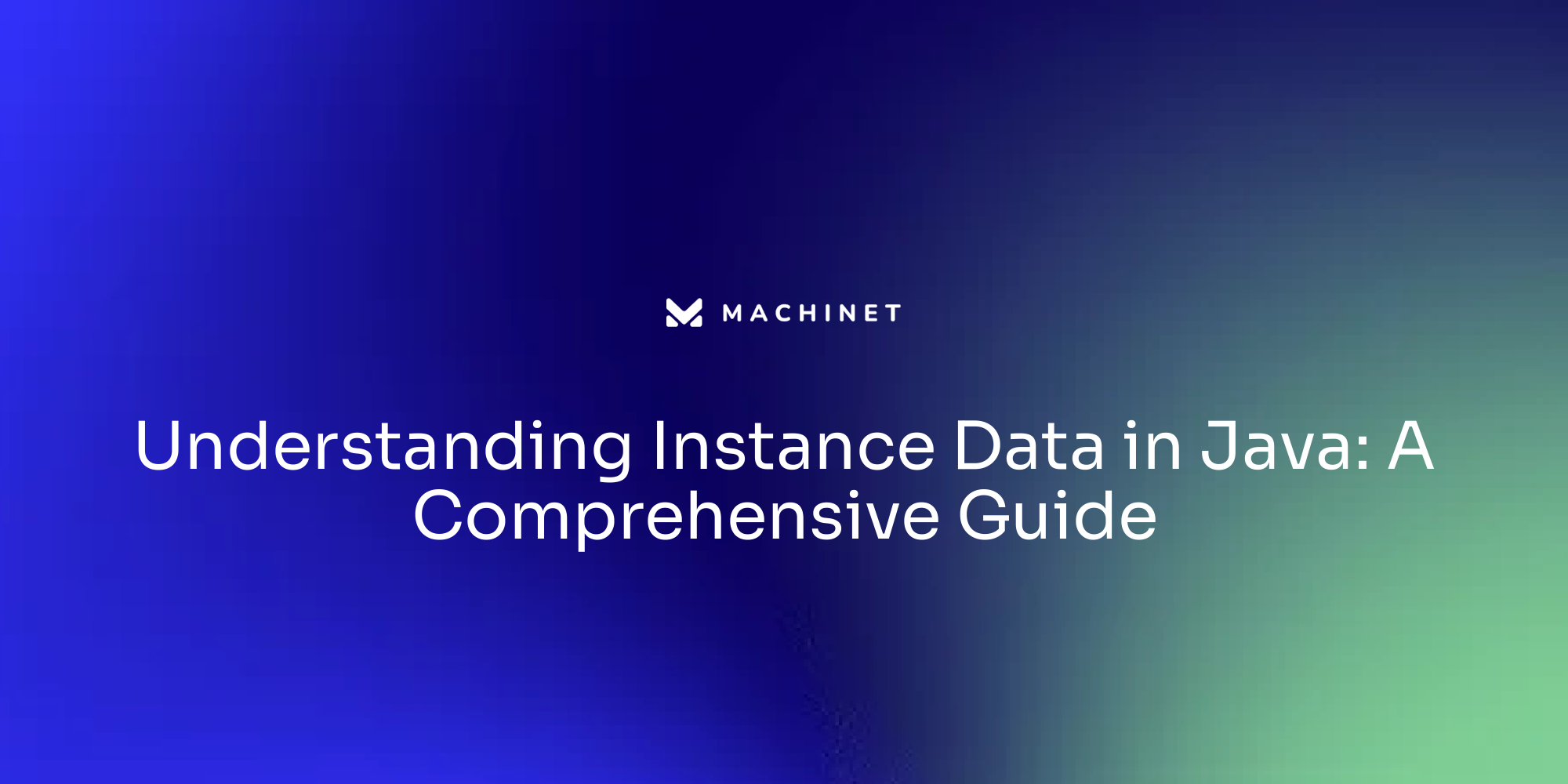
Introduction
In Java programming, the concept of an instance is fundamental, serving as the cornerstone for creating and managing objects. An instance, derived from a class, embodies a specific realization of that class, encapsulating its state and behavior. This instantiation process allocates memory for the object, allowing it to maintain its unique set of values for instance variables.
Unlike class variables, which are shared across all instances, each instance variable holds distinct data, enabling objects created from the same class to operate independently. This mechanism underpins the object-oriented nature of Java, fostering organized and modular code structures essential for developing complex applications. Understanding how instances and their associated variables and methods function is crucial for mastering Java, a language widely adopted in the industry and valued for its robustness and versatility.
What is an Instance in Java?
In Java, an object is a particular implementation of any type. When you define a type, it serves as a blueprint, but no memory is allocated until you create an object of that type. This example, commonly known as an entity, encompasses the state and behavior specified by its type. Each object keeps its own collection of values for attributes, guaranteeing that even if several objects are generated from the same type, they can store different data. This characteristic makes Java a powerful language for developing complex applications, as it allows for clear and organized code structure. Grasping how occurrences function is vital, particularly given that Java is extensively utilized in numerous leading firms and excelling in it can greatly enhance your career opportunities.
Instance Variables: Definition and Usage
Instance variables, also referred to as fields, are established within a structure but outside any method. Every entity created from the class has its own version of these attributes, which are essential for maintaining the condition of that particular entity. These variables store attributes distinct to each occurrence, allowing each entity to maintain its individual data. For example, in a banking application, a variable could be the account balance, with each bank account object having its own balance. This ensures that actions on one account do not affect the state of another.
Accessing Instance Variables: Using 'this' Keyword and Class Objects
The this
keyword in Java is a powerful tool that refers to the current object of a class. It's particularly helpful in distinguishing between object variables and parameters that share the same name, providing clarity and avoiding confusion. For example, in a constructor or function, when a parameter has the same name as a variable of the object, this
ensures that the variable of the object is accessed correctly. Instance functions, which are non-static, are invoked on instances of the class and can alter the state of the entity they belong to. These approaches are defined without the static
keyword and are called on an object using dot notation, such as my Dog.bark()
. By using this
, developers can write more readable and maintainable code, ensuring that each reference to a variable or method is clearly understood in the context of the current instance.
Differences Between Instance Variables and Class Variables
Object-specific variables are unique to each entity, ensuring that every object maintains its own state. In contrast, class variables, marked with the static keyword, are shared across all occurrences of a category. When a class variable is modified, the alteration is shown in all objects, creating a shared state. This distinction is crucial in object-oriented programming as it dictates how data is managed and accessed. Excessive use of static functions and properties can result in closely linked code, complicating unit testing and upkeep. 'Static attributes can be beneficial for constants or fixed values, but it's typically recommended to favor object functions to encourage a more maintainable code structure.'.
Understanding Instance Methods in Java
Object functions are crucial elements of a structure, created to work on object attributes. These techniques are called on an instance of the class, enabling them to reach and alter the instance's state directly. One of the essential characteristics of these functions is their capability to engage with object-specific variables, which signify the distinct traits of an object. By utilizing the this
keyword, object functions can effortlessly differentiate between object attributes and parameters that have the same name.
For example, consider a Dog
class with instance functions bark
and ageOneYear
. These techniques can be applied to separate Dog
instances, like my Dog
and another Dog
, to alter their corresponding name
and age
attributes. This interaction is crucial for encapsulating behavior related to an entity's state and providing a means to modify that state.
The arrangement of a specific function generally comprises an access specifier, a return type, a function name, and parameters. Together, these components define the visibility, functionality, and inputs of the approach, enabling it to perform specific tasks within the context of an object. This non-static context differs from static functions, which can be invoked without an object of the type.
How to Create and Use Instance Methods
To create a function in Java, define it within a type without using the static keyword. After being specified, these techniques can be invoked on an instance of the category using the dot operator. This enables the approach to function on object data, executing actions or calculations based on the particular condition of the entity.
For example, consider the following class:
```java public class Dog { private String name; private int age;
public Dog(String name, int age) {
this.name = name;
this.age = age;
}
public void bark() {
System.out.println(name + " is barking");
}
public void age one year() {
age++;
}
public int getAge() {
return age;
}
} ```
In this example, bark
and age one year
are instance methods of the Dog
class. They are called on examples of the Dog
class, such as my Dog
and another Dog
. These techniques can reach and modify the attributes (name
and age
) of their corresponding entities. This encapsulation of behavior associated with an object's state is a fundamental benefit of object-specific functions, as it allows interaction with and alteration of that state.
Object functions have access to the object attributes of the class and can use the this
keyword to refer to the current object. This is especially helpful when distinguishing between class variables and parameters with the same name. The non-static environment of class functions guarantees they are invoked on particular entities, rendering the code more modular and simpler to manage.
Key Differences Between Instance Methods and Static Methods
Functions linked to specific entities enable them to access variable data. In contrast, static functions are associated with the class itself and cannot access object variables directly. This distinction makes fixed functions perfect for utility tasks that do not rely on instance state. Additionally, static functions can act as factory functions, improving flexibility and encapsulation during object creation. They also offer performance benefits by avoiding the overhead of object instantiation, though this advantage is generally marginal in modern programming practices. Furthermore, static methods and properties allow namespace scoping, indicating their importance to the whole class rather than separate objects.
Practical Examples of Using Instance Data in Java
Utilizing example data in Java significantly enhances the flexibility and functionality of applications. Think of a banking application where each account can be represented as a structure containing attributes such as balance and account number. This enables instance functions to manage operations like deposits and withdrawals, thus guaranteeing clear and structured oversight of account states. This approach aligns with Object-Oriented Programming (OOP) principles, which advocate for structuring code in a modular and organized manner. According to specialists, classes in OOP serve as templates, outlining characteristics and functions that entities will have, thereby encouraging code reusability and maintainability. Additionally, utilizing automated test data generation tools like Instance can streamline testing processes, reducing boilerplate code and simplifying the creation of complex objects with multiple fields and relationships.
Conclusion
Understanding instances in Java is essential for grasping the language's object-oriented nature. Instances, or objects, are created from classes, which serve as blueprints for defining state and behavior. Each instance possesses its own set of instance variables, allowing for independent data management across multiple objects.
This encapsulation is vital for developing organized and maintainable code, particularly in complex applications.
Instance variables and methods play a crucial role in the functionality of Java applications. Instance variables maintain the unique state of each object, while instance methods operate on these variables, enabling the manipulation of object states. The this
keyword enhances clarity when referencing instance variables, especially when their names conflict with method parameters.
Understanding the distinction between instance and class variables, as well as between instance and static methods, is fundamental for effective programming in Java.
By leveraging instance data, developers can create modular and reusable code structures aligned with object-oriented programming principles. Practical applications, such as banking systems, illustrate the power of instances in managing complex state and behavior. Overall, a solid grasp of instances, variables, and methods in Java is crucial for building robust applications and advancing career prospects in the software development industry.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.