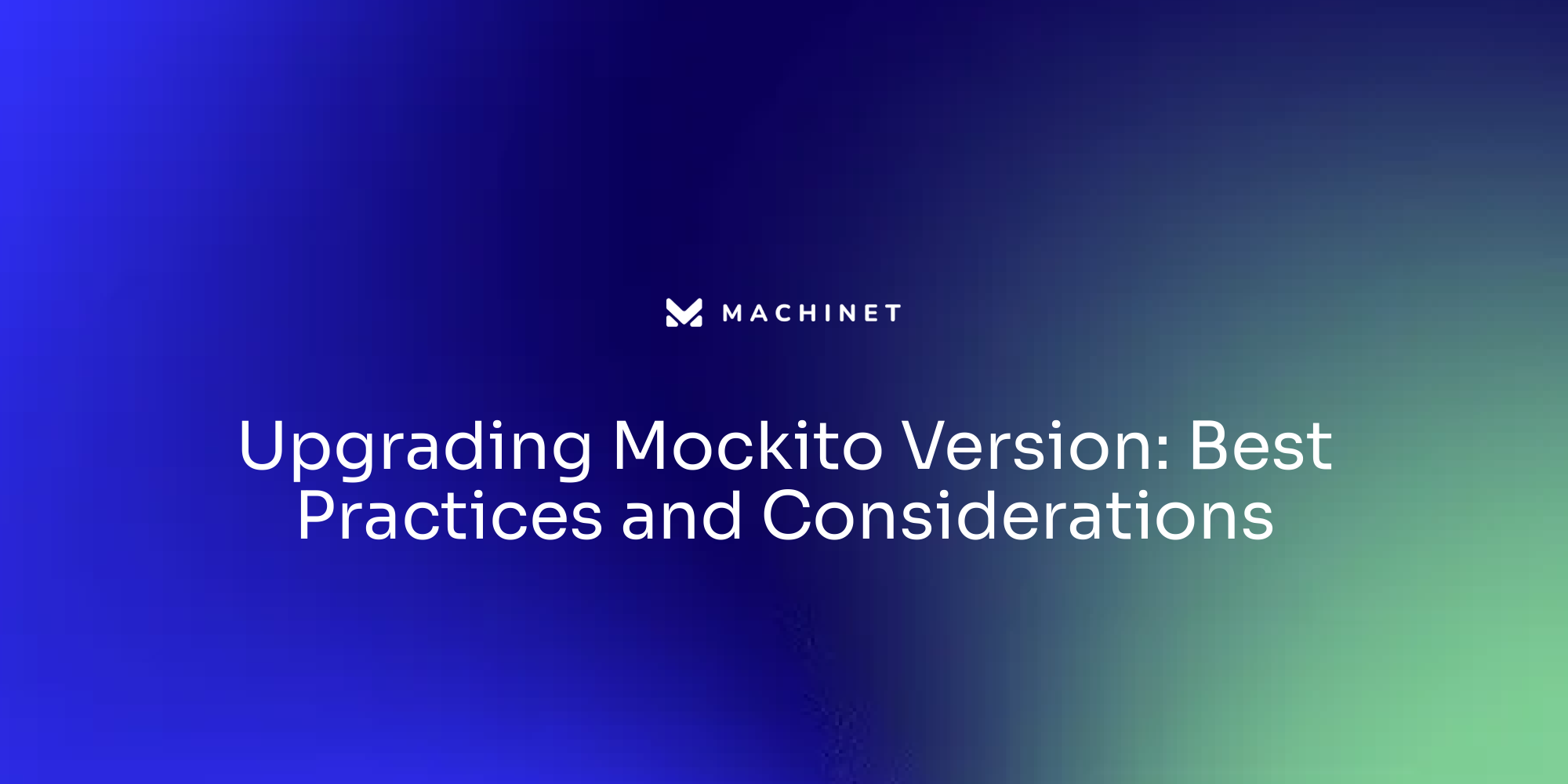
Table of Contents
- Understanding Mockito: An Overview
- Importance of Keeping Mockito Up-to-Date
- Preparing for Mockito Upgrade: Evaluating Current Version and Identifying Changes
- Steps to Upgrade Mockito in Your Java Unit Tests
- Addressing Potential Challenges During Mockito Upgrade
- Verifying the Successful Implementation of the New Mockito Version
- Best Practices for Maintaining and Updating Mockito Regularly
Introduction
Mockito is a powerful framework that simplifies the creation of mock objects for Java unit tests. It provides an intuitive API for mocking, verifying, and stubbing interactions, making it an essential tool for Java developers. Mockito is particularly useful when dealing with code that has complex dependencies, allowing for effective isolation during testing.
In this article, we will explore the advantages of using Mockito in Java unit testing and the best practices for upgrading and maintaining Mockito. We will discuss the importance of staying up-to-date with the latest version of Mockito, how to handle potential challenges during the upgrade process, and how to verify the successful implementation of the new version. Additionally, we will provide insights into maintaining and updating Mockito regularly, ensuring efficient and effective testing practices. By leveraging Mockito's capabilities and following best practices, developers can improve the quality and reliability of their unit tests in Java projects
1. Understanding Mockito: An Overview
Mockito is an influential framework that significantly streamlines the creation of mock objects for Java unit tests. This tool provides a clean, user-friendly API for mocking, verifying, and stubbing interactions, making it a vital instrument for any adept Java developer. Mockito is particularly useful when dealing with code that has complex dependencies, as it allows for the isolation of code behavior during testing.
This framework allows developers to focus on writing tests that accurately depict their code's behavior. Mockito aids in maintaining testing code that is concise and readable, thereby preventing coding redundancies and ensuring that as much of the code range as possible is covered.
However, it's important to be aware of the potential pitfalls of mocking. While Mockito is a powerful tool, it's prudent to avoid mocking types not owned by the developer. This practice can lead to design issues and complicate integration with third-party libraries. Instead, employing builders and factory methods for creating fixtures and value objects for testing is advised.
Despite the convenience of using mock objects as dependencies in unit tests, this method can lead to brittle tests. Mocking frameworks often assert each mock API call, which can break tests when the code is altered. Such tests are fine-grained and dependent on the current state of the device under test, making them fragile.
An alternative to using mocks is utilizing a "fake" implementation of the dependency. This method is less complicated and easier to set up in tests. Another option is to run integration and behavioral tests for multiple components instead of unit testing calls to complex dependencies. Stubs, or mocks without assertions, can be less fragile than mocks but may still exhibit brittleness issues.
It's also crucial to note that internal logic tests can disrupt tests even without observable external changes. Therefore, it's vital to concentrate on integration tests for complex interactions with dependencies, rather than testing the code of dependencies in unit tests.
Aspiring to expand their knowledge on testing and development practices, the book "Growing Object-Oriented Software Guided by Tests" is highly recommended. A mailing list is also available for further support and discussion.
Joe Blubaugh succinctly states, "Writing good unit tests is made much easier by dependency injection." He further advises, "Don't unit test calls to complex dependencies, write integration and behavioral tests for multiple components." And he reminds us, "A stub is like a mock that doesn't do assertions." These insights underline the importance of using Mockito judiciously and understanding its place within the broader context of Java unit testing.
To incorporate Mockito in Java, the first step is to include the Mockito library in your project's dependencies, either by adding the appropriate Maven or Gradle dependency to your build file. Once the library is added, Mockito can be utilized in your tests.
With the Mockito.mock()
method, a mock object can be created. This method takes a class or an interface as a parameter and returns a mock object of that type. This mock object can then be used to define the behavior of the methods you want to mock.
The behavior of a method in a mock object can be defined using the Mockito.when()
method. This method takes the mock object and the method you want to mock as parameters, and returns a MockitoStubber
object. The thenReturn()
method of the MockitoStubber
object can then be used to define the return value of the mocked method.
Once the behavior of the methods you want to mock has been defined, the mock object can be used in your tests. The mocked methods can be called and verified that they are called with the expected arguments and in the expected order using the various verification methods provided by Mockito.
Overall, using Mockito in Java enables the creation of mock objects and defines their behavior, which is beneficial for isolating and testing specific parts of your code. Mockito is a powerful tool for unit testing in Java, simplifying the process of creating and using mock objects, thereby allowing developers to write more effective and reliable tests.
Upgrade to the latest version of Mockito and supercharge your unit testing!
By using Mockito, developers can improve the quality of their code and ensure that it behaves as expected in different scenarios
2. Importance of Keeping Mockito Up-to-Date
As a Senior Software Engineer, the regular updating of Mockito, a widely-adopted mocking framework for Java unit tests, is crucial. With each new version, enhanced features, bug fixes, and performance improvements are introduced, which can significantly optimize your testing process.
Take Mockito 5, for example, which brought with it a change in the default mockmaker interface. The switch to Mockito Inline now enables mocking of constructors, static methods, and final classes, something that was not previously possible. This enhancement broadens your testing capabilities, allowing for a more extensive testing scope.
Moreover, Mockito 5 introduced the ArgumentMatcher interface, an instrumental feature that facilitates creating custom matchers for method arguments. This feature proves especially beneficial when dealing with varargs as it supports matching exactly one vararg argument or any number of arguments. By leveraging these enhancements, developers can construct more precise and flexible tests.
Another compelling reason for maintaining an up-to-date Mockito version is the compatibility it guarantees with other tools and frameworks in your tech stack. Mockito 5, for instance, aligns with the latest JDK versions, being compatible with Java 11 and above. This compatibility assures that your Mockito-based tests can integrate seamlessly with your current tech stack, ensuring efficient and uninterrupted testing workflows.
Keeping Mockito updated is also crucial for the security of your application. Each new version typically incorporates patches for any known vulnerabilities, contributing to the overall security posture of your application. Therefore, keeping Mockito updated not only furnishes you with the latest features but also bolsters your application against potential security threats.
To update Mockito to the latest version, a few simple steps can be followed:
- Check the current version of Mockito that you are using in your project.
- Visit the official Mockito website or the Maven repository to find the latest version available.
- Update the Mockito version in your project's build configuration file (such as pom.xml for Maven or build.gradle for Gradle) by changing the dependency declaration to the latest version.
- Save the changes and rebuild your project.
- Run your project's tests to ensure that everything is working correctly with the updated version of Mockito.
Through these steps, Mockito can be updated to the latest version in your project, ensuring you're leveraging the most up-to-date features and improvements. Regularly updating Mockito is an integral part of your software testing strategy, enabling you to harness the power of new features, ensure compatibility with your tech stack, and fortify your application's security.
Stay up-to-date with the latest version of Mockito and enhance your testing capabilities!
So, be it Mockito 5 or any future versions, staying updated is key to reap the full benefits of this robust testing framework
3. Preparing for Mockito Upgrade: Evaluating Current Version and Identifying Changes
When considering an upgrade to a newer version of Mockito, it's essential to first identify the version currently in use and understand the changes that will occur post-upgrade. This requires a thorough analysis of the release notes associated with the upcoming Mockito version. These notes offer a summary of new features, enhancements, bug fixes, and potential major changes. By understanding these changes, one can better anticipate any challenges that might arise during the upgrade, thus facilitating strategic planning.
To determine the current version of Mockito, you could refer to the official Mockito website or the Mockito GitHub repository. These resources offer details on the latest Mockito version, including release notes, documentation, and any modifications or updates made to the framework. This proactive approach ensures that you're using the most recent features and improvements in Mockito.
While contemplating an upgrade, it's also crucial to anticipate potential breaking changes. Mockito often provides guidance on migrating from one version to another, highlighting any breaking changes that could occur. It's recommended to thoroughly test your code base after upgrading Mockito to ensure that all unit tests pass and there are no unexpected issues. Paying attention to the Mockito user community and forums can provide insights into any challenges or workarounds faced by other users during the upgrade process, helping you proactively address potential breaking changes and minimize disruptions.
During the upgrade of Mockito, several issues might arise, including changes in the API, deprecated methods, or compatibility issues with other libraries or frameworks. A careful review of the release notes and documentation provided with the new Mockito version can help identify any potential issues. It's also recommended to thoroughly test the application after the upgrade to ensure that all mock objects and unit tests function correctly.
Preparing for a Mockito upgrade involves several steps. First, familiarize yourself with the latest version of Mockito, its new features, and improvements, by reading the release notes and documentation provided by the Mockito team. Next, review your existing unit tests and ensure they're written in a way that will be compatible with the new version of Mockito. This may involve making changes to your test code to accommodate any breaking changes or deprecated features in the new version. Also, run your existing unit tests with the new version of Mockito to ensure they still pass and function as expected. This will help identify any potential issues or incompatibilities that need to be addressed before fully upgrading. Finally, update any relevant documentation or guidelines for your development team to reflect the changes and improvements in the new version of Mockito. This will ensure that everyone is aware of the changes and can make the necessary adjustments to their code and testing practices
4. Steps to Upgrade Mockito in Your Java Unit Tests
In order to successfully upgrade Mockito in your Java unit tests, there are several steps that need to be followed. The first step is to identify the current version of Mockito that you are using in your project. This can be done by examining the dependencies in your project's build file or by checking the version in your project's configuration.
Next, you should visit the official Mockito website or the project's repository on GitHub to find the latest version of Mockito. Once you have identified the latest version, you can then update the Mockito dependency in your project's build file or configuration to this new version. This can be achieved by changing the version number in the dependency declaration.
After updating the dependency, it's time to rebuild your project. This ensures that the new version of Mockito is downloaded and used. Following this, you should review the Mockito documentation or release notes for any changes or new features introduced in the updated version. This will help you understand any breaking changes or additional configuration that may be required.
Your unit tests should then be updated accordingly. Depending on the changes introduced in the updated version, you may need to modify your existing code or configuration to align with the new version of Mockito. Finally, it's crucial to test your code thoroughly after the update to ensure that everything functions as expected.
Take note that when upgrading Mockito in Java unit tests, it's important to review the documentation, run your tests, and seek support from the community if needed to troubleshoot and resolve any issues that arise.
When upgrading Mockito in Java unit tests, there can be common issues that developers may encounter. These issues could be related to compatibility problems between the new version of Mockito and the existing codebase. It is important to ensure that the new version of Mockito is compatible with the other dependencies and frameworks used in the project. Additionally, there may be changes in the API or behavior of Mockito, which could require modifications to the existing test code.
Furthermore, it is possible that some tests may start to fail after the upgrade. This can happen due to changes in the behavior of the new version of Mockito. To handle failing tests, identify the failing tests, analyze the release notes and documentation of the new version of Mockito to understand the changes in behavior that might be causing the tests to fail, modify the failing tests to adapt to the changes in Mockito, rerun the tests after updating the failing tests, and run the entire test suite to ensure that the changes made in the failing tests did not introduce any new failures in other parts of the code.
Lastly, to verify test success after upgrading Mockito in Java unit tests, update the Mockito dependency in your project's build configuration to the latest version, recompile your project to ensure that the new version of Mockito is being used, review your existing unit tests and make any necessary updates to accommodate changes in the upgraded Mockito version, run your unit tests to verify that they still pass and that the mocking behavior is as expected, and if any tests fail, debug the failures and make any necessary adjustments to your test code or mocking setup.
This way, you can ensure that your unit tests continue to pass and that the upgraded Mockito version is working correctly in your Java project. Happy coding!
5. Addressing Potential Challenges During Mockito Upgrade
The challenge of upgrading Mockito can often be complex and multifaceted, with potential hurdles such as breaking changes and dependency conflicts. Breaking changes, modifications in the software that could require adjustments to your test code, are a common issue when dealing with software upgrades. Besides breaking changes, resolving dependencies with other libraries in your project can also become a stumbling block during the upgrade process.
To traverse these challenges effectively, having a comprehensive understanding of the changes associated with the new Mockito version is crucial. This knowledge allows you to anticipate possible issues and devise effective solutions in advance. Still, even with a thorough understanding of the changes, assistance may be required. In such scenarios, consider reaching out to online communities or professional consultants. These resources can offer valuable insights and guidance to aid in a successful Mockito upgrade.
In addition to the challenges mentioned above, users have reported multiple other issues during the Mockito upgrade process. These include problems with Mockito asynchronous verification, integration testing for asynchronous tasks, and errors when using static import of org.mockito.Mockito.
Some users have also encountered difficulties with Mockito BDD when mocking a method with a class return type, as well as issues with JDK not supplying a working agent attachment mechanism. Other common issues include trouble understanding how a mock is created in Mockito, unexpected behavior in multi-thread tests, and issues following a Mockito version upgrade.
Given the multitude of potential challenges, staying informed and updated about the latest issues and solutions is essential. Active participation in online communities, regular review of updates and pull requests, and maintaining open communication with other developers and Mockito users can help accomplish this. Dealing with these challenges effectively will ensure a smooth and successful Mockito upgrade process.
When upgrading Mockito, it's important to handle any breaking changes that may occur. A systematic approach is recommended, starting with reading the release notes of the new version. These notes provide information about any breaking changes and how to handle them. Next, update your project's dependencies to use the new version of Mockito and run your tests to identify any failures or errors.
If any tests fail after the upgrade, analyze the failures to determine if they are caused by breaking changes in Mockito. Look for error messages or stack traces that may indicate the cause of the failure. If you encounter any issues or are unsure how to handle a breaking change, consult the Mockito documentation. The documentation should provide guidance on how to handle specific breaking changes.
Based on the information gathered from the release notes, test failures, and documentation, make the necessary code changes to adapt to the breaking changes in Mockito. This could involve updating method signatures, changing method calls, or modifying test assertions. After making the necessary code changes, retest your application to ensure that it functions correctly with the upgraded version of Mockito. Verify that all tests pass and that the desired behavior is still achieved.
By following these steps, you can effectively handle breaking changes when upgrading Mockito and ensure the continued functionality of your tests. Remember to always keep your dependencies up to date to benefit from the latest features, bug fixes, and performance improvements
6. Verifying the Successful Implementation of the New Mockito Version
The process of upgrading Mockito and verifying its successful implementation is a multi-faceted one. It is not just about running tests and making sure they pass, but also involves a deep dive into the output of these tests to ensure Mockito is functioning as anticipated.
Firstly, identify the current version of Mockito used in your project. Check the official Mockito website or the project's documentation to find the latest version available. Update the version in your project's dependencies, either manually by editing the build file (like pom.xml
for Maven projects) or using a dependency management tool. After updating, rebuild your project to ensure all dependencies are resolved correctly.
Next, review the Mockito documentation or release notes for potential changes or deprecations in the new version. Update your test cases to use any new features or APIs introduced in the upgraded version, if applicable. Run your test suite and verify that all tests pass successfully. If you encounter any failures, debug and address them accordingly.
For instance, if you're testing a service class's login method and it's not validating credentials or handling exceptions as expected, you'll need to address this promptly. Debugging issues with Mockito after upgrading requires careful analysis of the changes in the new version and thorough testing of your code.
One common cause of issues after upgrading Mockito is changes in the API or behavior of certain methods. Review your code and make sure that you are using the correct method signatures and parameters according to the new version of Mockito. Mockito's verification features, such as the verify()
method, can help ensure that your mocks are being used correctly and the expected interactions are happening.
Moreover, if you're testing functionality that involves interaction with third-party libraries, such as an e-commerce application's login functionality which might need to interact with a data access object (DAO), be mindful of the principle that advises against mocking a type that you don't own. Instead, consider using wrappers or integration tests to verify such interactions.
Lastly, remember that the goal is not just to have a test suite that works but also one that adheres to principles of good testing - such as keeping the testing code compact and readable, avoiding coding a tautology, and covering as much of the range as possible.
As you work with Mockito in your unit tests, don't hesitate to further your understanding and mastery of this framework by leveraging additional resources. For instance, the book "Growing Object-Oriented Software, Guided by Tests" comes highly recommended. You can also engage in further discussion and support via the available mailing list.
In essence, upgrading Mockito to the latest version and verifying the successful implementation of the upgrade in your project involves not just running your tests, but also closely examining their output, promptly addressing any arising issues, and striving for excellence in your testing practices
7. Best Practices for Maintaining and Updating Mockito Regularly
Keeping Mockito updated and well-maintained is crucial to fully capitalize on the benefits this framework provides. Regularly updating Mockito to the latest version, closely reviewing and modifying your test code to align with any changes in Mockito, and incorporating a continuous integration server that autonomously builds and tests your code whenever modifications are made, all contribute to efficient and current use of Mockito.
To update Mockito to the latest version, the steps are straightforward. First, determine the current Mockito version in use in your project. Then, visit the official Mockito website or the Maven Central Repository to check for the latest available version. Update the version number in your project's build file, save the changes, and rebuild your project. The build tool will automatically download and update to the latest version of Mockito in your project. Don't forget to update any Mockito-related dependencies in your project, if required, to ensure compatibility with the latest version.
In line with this, consider some general best practices for maintaining and updating Mockito. Regularly updating to the latest version, referring to the official Mockito documentation for guidance, keeping track of release notes when updating, reviewing and updating existing test cases to align with any changes in Mockito, and staying informed about Mockito updates through official Mockito channels are all good habits to cultivate.
Let's illustrate this with a practical example of an ecommerce application. Here, the primary objective is to test if the customer's information is saved in the session post a successful login. With Mockito, focus shifts to verifying that the method responsible for saving customer data in the session is called. A fictitious data access object (DAO) named CustomerDao
has been introduced with a simple login
method that returns an empty Customer
object.
A service named LoginService
that employs CustomerDao
and possesses a login
method that delegates the login process to the DAO and invokes the saveInSession
method to save customer data in the session is introduced. The dependencies required for Mockito and JUnit are provided in the Maven pom.xml
file. The test code is displayed using a spy instead of a mock, and the verify
method from Mockito is utilized to check if the saveInSession
method is called. Note, the verify
method only checks if a method is called and does not verify returned values or object equality.
The method being verified cannot be private, hence a package-level modifier is used in this example. By using Mockito for method verification and regularly experimenting with the code in your own unit tests, your understanding of Mockito will enhance along with keeping your testing practices up-to-date and effective
Conclusion
In conclusion, Mockito is a powerful framework that simplifies the creation of mock objects for Java unit tests. It provides an intuitive API for mocking, verifying, and stubbing interactions, making it an essential tool for Java developers. Mockito allows developers to focus on writing tests that accurately depict their code's behavior and helps in maintaining concise and readable testing code. However, it's important to be aware of potential pitfalls such as design issues when mocking types not owned by the developer and the brittleness of tests using mock objects.
The broader significance of using Mockito lies in its ability to improve the quality and reliability of unit tests in Java projects. By leveraging Mockito's capabilities, developers can effectively isolate complex dependencies during testing and ensure that their code behaves as expected in different scenarios. Regularly updating and maintaining Mockito is also crucial, as it brings enhanced features, bug fixes, and performance improvements that optimize the testing process. Developers should stay up-to-date with the latest version of Mockito, handle potential challenges during the upgrade process, and verify the successful implementation of the new version to boost their productivity in Java unit testing.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.