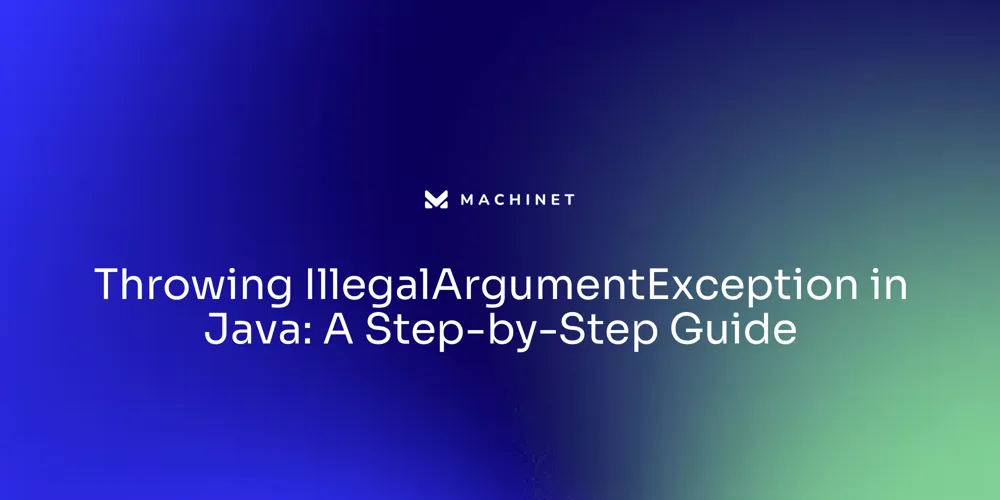
Table of Contents
- Understanding IllegalArgumentException
- When to Throw an IllegalArgumentException
- How to Throw an IllegalArgumentException
- Best Practices for Throwing Exceptions
Introduction
Exceptions are a critical aspect of programming in Java, allowing developers to handle unforeseen circumstances and maintain the stability of their applications. One such exception, the IllegalArgumentException, is often used to indicate that a method has received an illegal or inappropriate argument.
Understanding when and how to throw this exception is crucial for creating robust and reliable Java code. In this article, we will explore the concept of IllegalArgumentException, its use cases, and best practices for handling it effectively. Whether you're a beginner or an experienced Java developer, mastering exceptions like IllegalArgumentException is essential for creating high-quality applications.
Understanding IllegalArgumentException
In Java, exceptions are not merely roadblocks but also critical tools for managing the flow of a program during unforeseen circumstances. Among them, the NullPointerException
is a notorious bugbear for developers, often causing runtime errors if not handled with care.
It arises when code attempts to use a reference that points to no location in memory (null), usually due to the absence of object instantiation. When the Java Virtual Machine (JVM) encounters such a scenario, it halts the operation and raises a NullPointerException
, signaling that it stumbled upon an inappropriate use of a null reference.
This is a clear sign that the object you are trying to access or modify doesn't exist, and any such operation is beyond the bounds of acceptable behavior, and you must guard against it to ensure the stability of your application. In the realm of Java's exception hierarchy, the act of 'throwing' an exception is a key concept.
It is how a program reports an error condition and initiates exception handling. You can throw an exception using the throw
keyword, which effectively creates an exception object that encapsulates details about the erroneous situation, including a message that can offer insights into what went wrong.
For example, while an IllegalArgumentException
is typically thrown to indicate that a method has been passed an illegal or inappropriate argument, understanding the underlying cause, such as a NullPointerException
, is crucial to preventing such exceptions and maintaining robust code. Statistics reflect Java's enduring popularity, with a significant proportion of developers working with Java alongside other languages like Python. Despite newer languages entering the scene, Java's use in frameworks like Spring ensures its relevance in the programming world. The language's design, which encourages catching and carefully handling exceptions like Null Pointer Exception
, is part of what makes Java a resilient choice for various applications, from web to mobile platforms. Thus, mastering the nuances of Java exceptions is not just good practice but a necessity for developers who aspire to create stable, reliable, and efficient applications.
When to Throw an IllegalArgumentException
In the realm of Java development, handling exceptions is a critical skill that ensures the robustness and reliability of applications. One must be particularly vigilant about the infamous NullPointerException (NPE), a common but preventable source of runtime errors. When it comes to using exceptions such as IllegalArgumentException, there are several best practices to follow.
Firstly, if a method parameter is outside the expected range, throwing an IllegalArgumentException is prudent. For instance, if a positive integer is anticipated but a negative one is provided, this exception should be used to signal the error. Additionally, when null values are passed to methods that require non-null arguments, IllegalArgumentExceptions serve to maintain the method's contract, reminding developers that null values are often the culprits behind NPEs.
Lastly, IllegalArgumentExceptions are useful when a method receives argument combinations that are not supported or are invalid, like two boolean parameters that should not be the same but are. Understanding and preventing such issues is part of developing more secure and stable Java applications, as NPEs can arise when an object reference with a null value is accessed or modified, indicating the absence of the expected object. It's essential to recognize that exceptions like NPEs and IllegalArgumentExceptions are not just language features; they are signals of deeper issues that require attention to ensure the integrity of your code.
How to Throw an IllegalArgumentException
When developing in Java, understanding the proper use of exceptions is paramount to maintaining a robust application. Exceptions serve as a signal for anomalies that occur during runtime.
One particular exception that you might encounter is the IllegalArgumentException
. This exception should be thrown when a method receives an argument formatted incorrectly or otherwise inappropriate.
To throw this exception effectively, you should first determine the specific condition that would deem an argument illegal. Once identified, you can use a conditional statement like an if
to verify when this condition is true.
Upon confirmation, instantiate the IllegalArgumentException
and include a descriptive message that clearly outlines why the exception is being thrown. The final step involves using the throw
keyword to actively throw the exception, which then engages the Java Virtual Machine's exception handling machinery. Consider the following code snippet for a practical illustration java public void validate age int age { if { throw new (age < 0 | age > 120) IllegalArgumentException("Invalid age: " + age);
}
}
In this example, the validation logic checks whether the age
parameter falls outside an acceptable range. If it does, the method throws an IllegalArgumentException
with a message indicating the specific cause of the error. This practice not only prevents the method from proceeding with an invalid argument but also aids in debugging by providing a clear explanation of the fault directly in the exception's message.
Best Practices for Throwing Exceptions
In Java, exception handling is a cornerstone of writing robust applications. When raising an IllegalArgumentException, it is crucial to provide a clear and specific error message to pinpoint the cause of the issue.
This enhances the clarity of your code and aids in troubleshooting. Input validation should take place at the earliest opportunity, preventing the propagation of invalid values that could lead to more complex errors.
Consistency in exception handling practices, such as error logging and clear user instructions, is vital for maintainability across your codebase. Moreover, documentation of method expectations, including parameter restrictions, is essential for other developers to use your code correctly without triggering exceptions.
NullPointerExceptions, in particular, are a frequent source of runtime errors. They occur when a null reference is used in a way that requires an actual object, leading to a disruption in the application's flow. Preventing these exceptions is as important as handling them, requiring careful code practices to maintain application stability. By understanding the intricacies of Java exceptions, from their types to custom creation, developers can better manage the error flow within their applications. Java's exception mechanism, when used effectively, not only addresses runtime errors but also contributes to the reliability and fault tolerance of the application.
Conclusion
In conclusion, understanding and effectively handling the IllegalArgumentException is crucial for creating robust and reliable Java code. By mastering this exception, developers can maintain application stability and handle unforeseen circumstances.
Best practices for throwing this exception include validating input, maintaining consistency in exception handling, and documenting method expectations. Overall, mastering the IllegalArgumentException is essential for creating high-quality Java applications that are stable, reliable, and efficient.
Master the IllegalArgumentException and create high-quality Java applications!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.