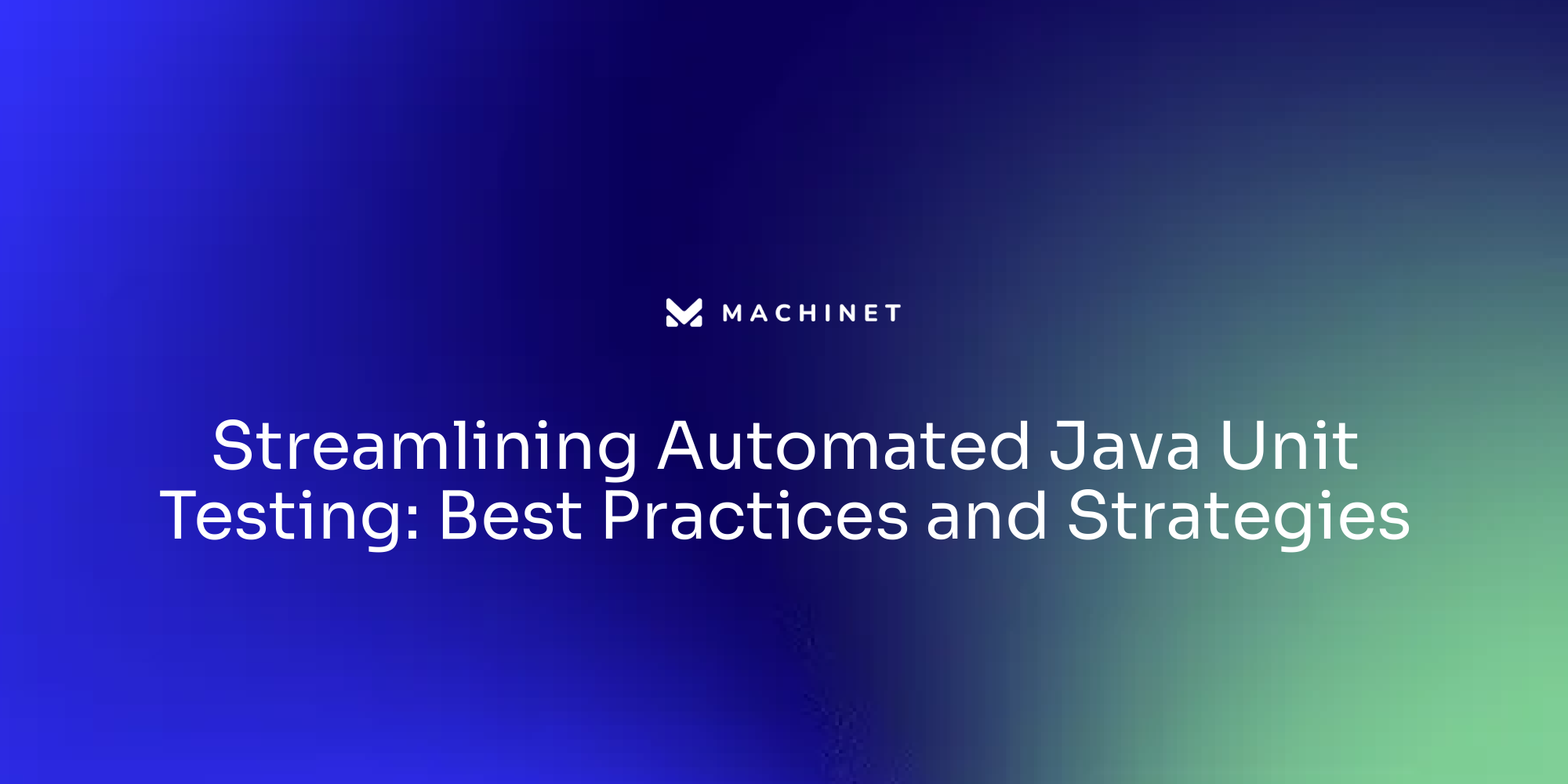
Table of Contents
- Understanding the Importance of Unit Testing in Java Development
- Setting Up and Running JUnit Tests in Java
- Utilizing JUnit Annotations and Assertions for Effective Testing
- Best Practices for Writing Effective Unit Tests in Java
- Automating Java Unit Tests: Tools, Frameworks, and Techniques
- Challenges and Limitations of Automatic Generation of Unit Tests in Java
- Strategies to Manage Technical Debt and Legacy Code in Unit Testing
- Case Study: Streamlining Automated Unit Testing in a Real-World Java Project
Introduction
Automated unit testing is a critical aspect of Java development, offering numerous benefits such as early bug detection, improved code quality, and increased software reliability. However, it is important to understand the challenges and limitations associated with automated unit testing to ensure its effectiveness. This article explores the importance of unit testing in Java development, the setup and execution of JUnit tests, strategies for writing effective unit tests, tools and frameworks for automating unit tests, and the management of technical debt and legacy code in unit testing. By following best practices and leveraging automation tools, developers can streamline the testing process, enhance code quality, and ensure the long-term success of their Java projects
1. Understanding the Importance of Unit Testing in Java Development
Unit testing is a fundamental aspect of Java development
Try Machinet to automate your unit testing process and save time!
, playing a key role in the verification of individual software components. It assists in early bug detection and resolution within the development cycle, thereby reducing debugging time, resources, and overall development cost.
Unit testing is also an invaluable documentation source, clarifying system behavior and making the code more understandable to developers. This is especially beneficial for large teams where code comprehension is crucial.
Unit testing in Java can be achieved using libraries such as JUnit and Mockito. These libraries enable the testing of each code component and isolate individual methods for testing, ensuring new functionality doesn't disrupt existing functionality and maintaining software stability and reliability.
Java project setup for unit testing requires the installation of IntelliJ IDE and project configuration with JUnit and Mockito dependencies. After the setup, test cases are written to check the expected output and compare it with the actual output. Assertions are used to pass or fail a test based on these value comparisons.
Unit testing also includes the creation of mock objects and method stubbing for code with external dependencies, such as model classes, database connections, or network requests. Libraries like Mockito are used for this purpose. This involves initializing necessary parameters, creating mock objects, stubbing methods, calling the method under test with initialized parameters, and using assertions to evaluate the test outcome.
Following a consistent methodology, breaking down test code into manageable units, and using effective naming conventions and structures are some of the best practices for writing effective unit tests. Tools like Jacoco can generate code coverage reports, which help measure progress in unit testing.
By adhering to these guidelines, test writing becomes simpler, quicker, and even enjoyable. These practices also lead to fewer bugs and facilitate future code refactoring. Ultimately, unit testing aids in ensuring that the code meets quality standards, making it an indispensable tool in the software development process.
To enhance the effectiveness of Java unit testing, it is crucial to follow certain best practices. These include proper code organization, achieving high test coverage, and effective test design. Test code should be structured for easy understanding and maintenance, tests should aim to cover as much code as possible, and tests should be designed to be independent of each other for easier debugging and maintenance.
Frameworks like Mockito can be used to isolate the code being tested from its dependencies, allowing for more focused and reliable tests. Automated test execution using build tools like Maven or Gradle ensures regular and consistent testing throughout the development process.
Moreover, following the AAA pattern (Arrange, Act, Assert) helps structure unit tests and improve their readability. Tests should cover different scenarios, both positive and negative cases, edge cases, and boundary conditions. Each unit test should be independent and not rely on the state or behavior of other tests. Test names should be descriptive and assertions should be used effectively to verify expected behavior.
Performance and scalability testing should also be considered alongside functional testing. Automated unit testing tools like JUnit, TestNG, Mockito, PowerMock, and EasyMock can simplify the unit testing process and improve the overall efficiency.
To integrate unit testing into the Java development workflow, a unit testing framework like JUnit should be chosen. Test methods covering different scenarios and testing the expected behavior of your code should be written and marked with annotations provided by the testing framework. These tests can be run using a build automation tool like Apache Maven or Gradle as part of the build process. Lastly, integrating unit testing into the continuous integration and delivery pipeline ensures automatic test execution whenever there is a change in the codebase.
While conducting Java unit testing, it is important to avoid common mistakes that can lead to inaccurate test results and hinder the effectiveness of the testing process. Mocking dependencies in Java unit tests can be achieved using a mocking framework like Mockito, which provides a simple and intuitive API for creating mock objects and specifying their behavior. Dependencies can be isolated, allowing for more reliable and deterministic unit tests
2. Setting Up and Running JUnit Tests in Java
The JUnit framework is a cornerstone of Java application development, renowned for its simplicity, ease of use, and seamless integration with most Java IDEs and build tools. It is a critical tool in the Test Driven Development (TDD) approach, where unit testing helps to identify potential issues early in the development cycle and guarantees code quality.
To begin with JUnit testing, the JUnit library should be integrated into your project. While some IDEs like Eclipse come with built-in support for JUnit, in other environments, you can add the JUnit library to your project by following a series of steps.
First, the JUnit library can be acquired either by downloading it from the official website or using a build management tool like Maven or Gradle to add it as a dependency. Once you have the library, it should be included in your project's classpath. In your Java project, create a new folder (for example, "lib") and place the JUnit library file (usually a JAR file) in this folder.
Next, navigate to the project properties or build path settings in your Java IDE, and locate the "Libraries" or "Dependencies" section. Click on the "Add JARs" or "Add External JARs" button and navigate to the location where you placed the JUnit library file. Select the JUnit library file and click "OK" to add it to your project's classpath. Once the JUnit library is added to your project, you can start using its classes and annotations to write and run unit tests.
Moving forward, the creation of a test class is the next step in the process, where each method within the class represents a unit test, focusing on a single functionality of the component being tested. The structure of a JUnit test class includes methods annotated with specific JUnit annotations. For example, JUnit 4 uses @BeforeClass, @Before, @Test, and @After annotations, while JUnit 5 employs @BeforeAll, @BeforeEach, @Test, and @AfterEach.
Test methods incorporate assert statements that compare the output of the code under test against expected results. JUnit provides a variety of assert methods including assertEquals, assertNotEquals, assertTrue, assertFalse, assertNull, assertNotNull, assertSame, and assertNotSame.
Executing test runs in Eclipse or any other IDE is straightforward. Whether it's an individual test method or the entire test class, simply right-click and choose "Run As" > "JUnit Test". The results of the test run, indicating pass or fail statuses, are displayed in the JUnit view in the IDE. Any tests can be rerun by right-clicking on the test class or test method and selecting "Run".
In summary, the setup and execution of JUnit tests not only aid in the early detection of bugs but also promote the delivery of high-quality software. For experienced Java programmers and software engineers, leveraging such tools can significantly enhance productivity and streamline the testing process
3. Utilizing JUnit Annotations and Assertions for Effective Testing
In the world of Java unit testing, JUnit stands out as a solid framework, utilizing annotations to discern and govern test methods. Annotations such as @Test, @Before, @After, and more notably, @BeforeClass are key in shaping the operational behaviour of your tests. The @BeforeClass annotation, in particular, is used to specify a method that is executed once before any test methods in the class, thereby enhancing the efficiency of tests that share common setup code.
Further, JUnit provides assert statements, such as the assertEquals() method, which checks the equality of two values. These assertions are pivotal in verifying the results, comparing the actual outcome with expected results. While the specific usage of assertEquals() method is not detailed in the context, it's worth noting that using it effectively is crucial for crafting precise tests that reflect your code's behavior accurately.
In addition to these, JUnit also focuses on naming conventions, ensuring that test classes are named in a way that makes them easy to identify and understand. Moreover, JUnit's compatibility with build management tools like Maven can simplify the testing process.
Beyond the standard annotations, JUnit offers the @Ignore annotation, useful for disabling tests or applying conditional assumptions. The feature of parameterized tests allows for testing with different input values, thereby improving the test coverage.
JUnit rules, a feature that permits the addition of behavior to tests, can be customized by implementing the TestRule interface. Test categorization, another valuable feature, allows inclusion or exclusion of tests based on annotations.
Moreover, JUnit supports the use of static imports, which can aid in writing more concise test statements. It integrates smoothly with Gradle and Maven, and can also be used independently in the Eclipse IDE.
The AssertJ library, which can be used alongside JUnit 5, provides a method called assertThat
for writing assertions and returns an assertion object. It supports assertions for a wide range of data types and structures, including boolean values, null objects, object equality and references, arrays, iterables, maps, exceptions, and optional objects.
A notable feature of AssertJ is 'Soft Assertions', which accumulate all assertion failures before reporting them, providing a comprehensive view of all failed assertions in a single report. This enhances the readability and understanding of test failures.
In essence, unit tests should be designed to cover a specific aspect of the code, testing a single behavior or functionality. Test names should be descriptive and expressive, clearly indicating what is being tested and what the expected outcome is. Unit tests should be independent of each other and not rely on the state or behavior of other tests. Choose assertions that clearly state the expected outcome and make the test results easier to understand. Select test data that covers a variety of scenarios and edge cases. Apply good coding practices to your test code, such as proper indentation, commenting, and avoiding duplication.
The extensive features of JUnit and AssertJ, when used effectively, can greatly enhance the efficiency and effectiveness of unit testing in Java
4. Best Practices for Writing Effective Unit Tests in Java
Unit testing in Java is a nuanced craft that demands a deep understanding of best practices. Each test should be self-reliant and independent, not reliant on the state of other tests. This can be achieved by using mocking frameworks like Mockito, which create mock objects that simulate the behavior of dependencies. These mock objects ensure that each unit test is insulated and does not depend on external resources or the state of other tests.
Each test must be precise and focused on a singular functionality, which allows for the accurate pinpointing of potential problems. Test data specific to each test case can be utilized to prevent test cases from interfering with one another, ensuring consistent test results.
Deterministic tests, which consistently yield the same results given the same input, foster a reliable and consistent testing environment. Comprehensive coverage of all conceivable scenarios and edge cases is critical. This includes testing both normal and exceptional scenarios, creating test cases with a variety of input data, and paying close attention to boundary conditions.
Automated tests are a crucial part of efficient testing. By writing tests that cover different aspects of your code, you can ensure that any changes or updates to your codebase do not introduce new bugs or issues. These tests can be run automatically as part of your build process, providing quick feedback on the stability of your code.
Adopting a systematic methodology can simplify the process of writing tests. Breaking down test code into manageable units of work, and utilizing clear naming conventions and structures, can add to the readability and maintainability of tests. A tool like Jacoco can be instrumental in generating code coverage reports, offering a quantitative measure of your progress.
Consider a simple customer class that needs to be tested. The constructor can be tested with both null and non-null parameters, and the getName method can be tested as well. Adopting a naming convention for test methods that encapsulates the given condition and the expected outcome can make the tests more self-explanatory.
Following the given-when-then style for test methods can be beneficial. The given step sets up the condition or creates the necessary objects for the test. The when step triggers the action that needs to be tested, followed by the then step that asserts that the application state is as expected. This logical sequence of steps makes each test an easy-to-follow unit of work.
Applying these conventions can result in concise, easy-to-read living documentation for your project. This practice not only makes the process of writing tests more efficient and enjoyable but also facilitates future code refactoring. As James Shvarts notes, "Writing unit tests is fun if you follow a consistent methodology which enables you to write tests faster, your test code is broken down into manageable small chunks (units of work), your test methods use effective, easy-to-follow naming convention and structure."
The value of adhering to these conventions in unit testing is immense. Once you adopt these guidelines, writing tests becomes easier, faster, and more enjoyable. Furthermore, the resulting code is backed by consistent, self-documenting tests, making future code refactoring significantly smoother
5. Automating Java Unit Tests: Tools, Frameworks, and Techniques
Java unit testing automation employs a variety of tools and frameworks designed to generate, execute, and report on tests automatically. JUnit, TestNG, and Mockito are popular choices for this, each offering functionality for creating test cases, running them, and presenting the results.
Test automation methodologies such as data-driven testing and behavior-driven testing are integral to this process. Data-driven testing executes a single test with multiple sets of data, while behavior-driven testing authors tests in a language that outlines the system's behavior. These tools and methods streamline testing processes and ensure comprehensive test coverage.
Consider a simple web application tested using JUnit. The application involved a Mule flow, taking a username as input and appending "hello" to it. The testing process required creating the Mule flow and the JUnit test, which were then run to verify the expected results.
In another instance, Mockito was used to mock a dependency in a Spring Boot application. The system under test was a class called "App", where the testing involved intercepting the 'sclient' in the app class and setting up the desired behavior of the mocked 'sclient'.
Logback is another tool used for reading the application log when testing a Spring application, further exemplifying the range of tools available for Java unit test automation.
The Awaitility library is particularly useful for asynchronous operations in Java integration tests. It allows you to poll for an expected test result until a condition is met, proving invaluable for asynchronous testing, especially when dealing with external systems in legacy systems.
JUnit 5 has brought significant improvements to parameterized tests. Now, individual test methods can be annotated as parameterized, with parameters supplied via annotations. This feature is especially useful for status validation testing using enum value source and exclude mode.
Hamcrest collections matchers, included in the Hamcrest library, can also be used in unit tests. These matchers allow for more readable syntax and direct comparison with Java Number objects without the need for explicit casting.
However, to optimize Java unit testing automation, it is crucial to adhere to best practices. This includes designing testable code, choosing an appropriate testing framework such as JUnit or TestNG, writing clear and concise tests, using test doubles for code dependent on external resources, testing in isolation, automating the testing process, and measuring code coverage. By following these practices, you can ensure the efficiency, accuracy, and scalability of your testing process.
Automated unit testing tools further streamline the testing process. These tools efficiently write, execute, and manage unit tests, saving time and effort while ensuring thorough testing for bugs and errors. Features such as test case generation, test execution, code coverage analysis, and test result reporting make it easier for developers to identify and fix issues in their code.
In summary, automating Java unit tests involves various tools and techniques that can enhance efficiency and effectiveness. By harnessing these resources, you can streamline your testing process and ensure comprehensive test coverage
6. Challenges and Limitations of Automatic Generation of Unit Tests in Java
Automated unit testing in Java development offers the promise of a productivity surge, but it's important to acknowledge its associated challenges and limitations. A significant concern is the inability of these auto-generated tests to cover all possible scenarios, especially edge cases. The lack of context can lead to tests that don't accurately reflect the intended behavior of the system.
To illustrate this, consider the use of Gson in end-to-end tests in Java. Its imprecise deserialization of objects from JSON can result in tests passing even when they shouldn't, creating false positive test results. This is because Gson does not throw exceptions when it fails to deserialize JSON. As a result, a string may pass as a number, or an enum may be mistaken for a string. Grasping the subtleties of the tools used, like understanding the difference between an enum and a string, or a string and a number, is key to sidestep unforeseen issues that can lead to potential bugs in the application.
The maintenance of these automatically generated tests can also be a formidable task, especially when dealing with a large and complex codebase. However, cutting-edge AI tools like Diffblue Cover can mitigate some of these challenges. Diffblue Cover utilizes machine learning to swiftly increase code coverage and generate human-readable tests without needing developer intervention. It boasts features such as automated unit test writing and automated software testing that can significantly enhance the quality of Java unit tests.
While automated testing comes with its own set of limitations, the benefits often outweigh the drawbacks, particularly when used alongside manual testing. When considering generative AI for code tools, it's crucial to keep pace with AI for code advancements and understand the behavior of the tools used. This is where resources and support from software development companies like Diffblue Ltd become valuable. Diffblue not only offers solutions for legacy code and software development but also provides a community forum for user interaction and support, making the journey of automated unit testing less daunting.
In response to the limitations of automatically generated unit tests in Java, developers can consider supplementing these with manual test cases. Manually written test cases can cover specific scenarios and edge cases that may be overlooked by automated testing tools. Techniques such as code reviews and pair programming can enhance the quality of automatically generated tests by involving multiple team members in the testing process, making it easier to identify and address any limitations or shortcomings in the generated tests.
Furthermore, managing complexity in Java automated testing requires adhering to best practices and employing proper techniques. These include writing modular and reusable code, using appropriate design patterns like the Page Object Model (POM), implementing clear and concise test cases, using assertions effectively, using parameterization techniques to run the same test with different input values, and maintaining good documentation.
In essence, while automated unit testing has its challenges, the right tools, understanding, and support can make it a valuable addition to the Java development process
7. Strategies to Manage Technical Debt and Legacy Code in Unit Testing
Addressing technical debt, an integral part of software development, is essential for the health and longevity of any software project. It represents the future costs that might arise from optimizing the codebase to assure its maintainability. This technical debt not only pertains to the code but also extends to the system and its architecture, accumulating over time due to suboptimal decisions and time constraints that inhibit the execution of more efficient solutions.
External factors, such as the advancement of libraries and frameworks, can also amplify the growth of technical debt. It's paramount to manage this diligently, particularly for prolonged projects, to avoid reaching a standstill where options for progression are non-existent. The process of addressing technical debt, often termed as 'paying' it, involves refactoring the code to improve its maintainability.
Refactoring is a demanding process that requires careful budgeting and justification to stakeholders. It encompasses various actions like cleaning up the code, enhancing its readability, breaking it down into smaller, more manageable components, and incorporating tests. Identifying technical debt can be aided by noticing difficulties in upgrading dependencies, scaling, and bug resolution.
An essential part of managing technical debt involves the proactive role of product management. Early monitoring and addressing of technical debt, recognizing and eliminating unnecessary features can alleviate some of the debt. The management of technical debt also involves early rectification of issues and bugs, promoting clean code, and integrating testing and refactoring into the development process.
Legacy code, another concept in software development, refers to code used in specific software that has accumulated a significant amount of technical debt. Developers might be reluctant to work with legacy code, but continuous education and training can help overcome this hesitation. The agreed approach is to gradually introduce tests as modifications are made to the code, ensuring that new bugs aren't introduced and that the code remains functional as it's updated.
One of the best practices to manage technical debt in unit testing involves regular review and refactoring of unit tests. This includes identifying any duplicated or unnecessary code and removing it, as well as enhancing the overall structure and organization of the tests. Prioritizing the most critical tests and ensuring they are up-to-date, while also gradually improving and expanding the test coverage over time. By continually investing in the maintenance and improvement of your unit tests, you can effectively manage technical debt, ensuring the long-term stability and quality of your codebase.
Automated testing tools can also be valuable in managing technical debt and legacy code, streamlining the testing process and making it easier to identify issues. A survey conducted at SymfonyLive Berlin 2019 revealed that only 20% of the participants rarely worked with legacy code, and about two-thirds of them would rather not work on it at all. This underscores the importance of strategies and tools that can make managing legacy code and technical debt less daunting and more efficient.
Improving code quality and maintainability is crucial and can be achieved by following best practices. These practices include writing clean and readable code, using meaningful variable and function names, modularizing code into smaller functions or classes, and implementing proper error handling. Additionally, using version control systems and conducting code reviews can also contribute to improving code quality and maintainability.
Managing technical debt and legacy code is a vital, ongoing process in software development that requires a proactive approach, continuous learning, and the right tools. It's a challenge that every developer faces, but with the right strategies and mindset, it can be effectively managed to ensure the long-term success of a software project
8. Case Study: Streamlining Automated Unit Testing in a Real-World Java Project
As seen in several large-scale Java projects, the need for optimizing unit testing processes is crucial. Teams often grapple with the complexities of their codebase and limited test coverage. To overcome these challenges, automation of Java unit tests becomes a pivotal strategy. By leveraging JUnit for writing and running tests, along with its annotations and assertions, teams can create effective tests. This approach of automated unit testing in Java greatly enhances code quality by verifying the functionality of individual units of code, catching bugs early, and making software more reliable and maintainable.
The practice of automated testing also facilitates easier refactoring and code changes, as any regressions can be quickly identified through the test suite. This is evident in the case of Dropbox's Android app, which faced challenges as their mobile engineering team and codebase grew. The time taken for their automated suite of unit tests increased from 30 to 75 minutes, leading to issues in their development infrastructure, notably in their testing pipeline. To combat this, they decided to reduce the continuous integration (CI) execution time and flakiness by selectively scaling their pipeline and utilizing industry-standard tools.
To write independent and comprehensive unit tests, it's crucial to follow some best practices. These include testing one thing at a time, using descriptive test names, structuring tests using the Arrange-Act-Assert (AAA) pattern, testing boundary conditions, using mocking and stubbing, testing failure scenarios, keeping tests independent, and testing performance. Following these practices helps in creating reliable, maintainable tests that provide good coverage of the code being tested.
The benefits of automated unit testing in Java development are numerous. It aids in identifying errors or defects early in the development process, thus allowing developers to rectify them before they escalate into larger issues. Automated unit testing also provides a safety net during code refactoring or modifications, ensuring that existing functionality remains intact. It also enhances code quality by enforcing good coding practices and reducing the occurrence of bugs. With automation, developers can save time and effort in manual testing, allowing them to focus on other important tasks.
However, managing flaky tests can also be a challenge. GitHub, for instance, implemented a system to manage flaky tests and saw an 18x improvement in reducing flaky builds. The system inspects failing tests, determines if they are flaky, and keeps the build green if the test passes when run again. This system helps prioritize and fix flaky tests, making CI more trustworthy and reducing the number of flaky builds. Developers can thus be confident that their builds won't fail due to flaky tests, allowing them to maintain momentum. This example from GitHub underscores the importance of managing flaky tests effectively to maintain a reliable CI pipeline
Conclusion
Automated unit testing plays a critical role in Java development, offering numerous benefits such as early bug detection, improved code quality, and increased software reliability. By following best practices and leveraging automation tools like JUnit and Mockito, developers can streamline the testing process and enhance the overall efficiency of their Java projects. Unit testing helps verify individual software components, aids in documenting system behavior, and ensures that code meets quality standards.
The importance of unit testing cannot be overstated. It not only helps catch bugs early in the development cycle but also promotes code readability and maintainability. By using effective naming conventions, breaking down test code into manageable units, and ensuring high test coverage, developers can create robust tests that accurately reflect the behavior of their code. Automation tools like JUnit make it easier to execute tests regularly and consistently throughout the development process.
To boost your productivity with Machinet, experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.