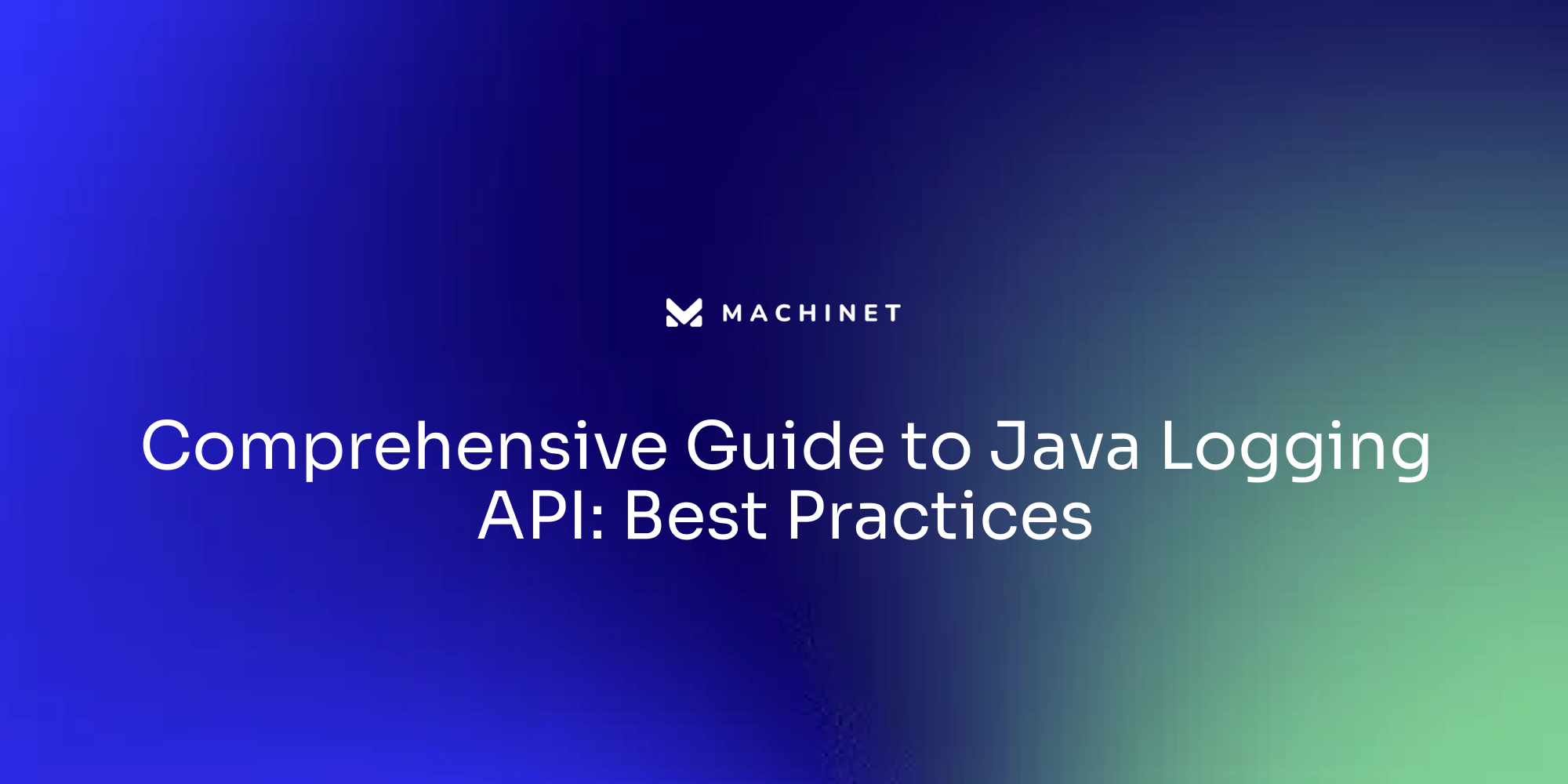
Introduction
The Java Logging API offers a comprehensive framework for managing log messages in applications, providing developers with essential tools to enhance observability, troubleshooting, and software reliability. This article delves into the core components of the Java Logging API, including loggers, handlers, formatters, and log records, explaining how each contributes to an efficient logging system. Furthermore, it explores the different log levels supported by the API, guiding developers on their strategic use for effective log management.
Additionally, the article provides step-by-step instructions for configuring the Java Logging API, emphasizing the importance of setting log levels, defining handlers, specifying formatters, and loading configurations. Best practices for Java logging are also discussed, highlighting the need for meaningful log messages, avoiding sensitive information, and centralizing logging configurations. The article concludes by comparing various logging frameworks, such as Log4j, SLF4J, and Logback, and introducing advanced logging techniques and troubleshooting strategies to optimize logging performance and make log data actionable.
Components of Java Logging API
The Java Logging API, located in the java.util. Logging package, provides a strong framework for handling log entries in applications. It comprises several essential components:
-
Logger: This is the core interface for recording information. Loggers capture log entries, which can be configured to log at various levels such as INFO, WARNING, or SEVERE. Effective use of log levels aids in filtering and prioritizing log entries.
-
Handler: Handlers are responsible for sending log entries to particular outputs, such as the console, a file, or a remote server. Personalizing handlers enables you to structure content in various formats, such as XML, HTML, or plain text, offering versatility in how records are displayed and saved.
-
Formatter: Formatters determine the appearance of log entries. They can be tailored to meet different formatting requirements, helping in better readability and analysis of log data. Common formats include XML, HTML, and simple text, ensuring that logs are both human-readable and machine-processable.
-
Log Record: Log records encapsulate detailed information about a log entry, including the entry content, timestamp, and severity level. This granularity is crucial for effective debugging and auditing, as it allows developers to pinpoint issues accurately.
Understanding these components and their interactions is key to leveraging the full potential of the Java Logging API. By configuring loggers, handlers, and formatters appropriately, developers can create a logging system that enhances observability, aids in troubleshooting, and improves overall software reliability.
Log Levels and Their Usage
Java Logging API supports multiple log levels that denote the severity and type of log messages, allowing developers to manage and filter logs effectively. Here are the primary log levels:
- SEVERE: Indicates a critical failure that demands immediate attention. It's crucial for identifying and addressing serious issues that could stop the program.
- WARNING: Highlights potential issues that might impact the system but do not halt its execution. These logs help in preemptively identifying issues that could escalate.
- INFO: Offers general information about the program's progress and operations. This level is useful for understanding the flow and state of the system during normal conditions.
- CONFIG: Used specifically for configuration messages, offering insights into the system's configuration state. This helps in verifying and troubleshooting setup-related aspects.
- FINE, FINER, FINEST: These levels are designed for detailed tracing and debugging. They capture granular information about the application's behavior, which is invaluable during development and for tracking down complex issues.
Selecting the appropriate log level is crucial for sustaining an effective recording system. It ensures that critical events are not missed while avoiding cluttering logs with too much information. As one expert notes, "Consider the importance of each log statement in troubleshooting and monitoring." By strategically using these levels, developers can create a balanced and informative record-keeping system that supports both operational monitoring and debugging needs.
Configuring Java Logging
Configuring the Java Logging API can be done programmatically or via configuration files, typically in XML or properties format. Here are the steps for configuration:
-
Set Log Level: Define the default log level for both loggers and handlers to manage which notifications are captured. This is essential for controlling the verbosity of the logs and ensuring that critical information is not missed while avoiding excessive logging that could obscure important details.
-
Define Handlers: Configure the destinations for log entries, such as the console or a file. Each handler can have its own log level, allowing detailed control over which entries are written to each destination. This setup is particularly useful for applications needing to handle multiple requests concurrently, such as those built with Spring WebFlux, which is designed for high scalability and efficient data handling.
-
Specify Formatters: Choose formatters to format log entries according to your needs. Different handlers can use different formatters, enabling you to present log messages in the most useful format for each context. This can significantly enhance the readability and usefulness of logs for debugging and monitoring purposes.
-
Load Configuration: Use the
LogManager
to load the configuration file at runtime. Proper configuration ensures that your recording framework captures the right information without overwhelming you with unnecessary details. This is crucial for maintaining performance and observability, especially in reactive applications where handling multiple requests efficiently is key.
Incorporating best practices and leveraging advanced features like the LocationAwareLogger
can transform your record-keeping system from a source of confusion into a precise diagnostic tool. 'Improved recording practices not only streamline debugging but also enhance operational efficiency and software reliability by providing clearer and more accurate entries.'.
Best Practices for Java Logging
To maximize the effectiveness of Java logging, consider the following best practices:
-
Log Meaningful Messages: Ensure that log messages provide context and are easy to understand. This aids significantly in troubleshooting by replicating the conditions under which the log was generated, making it easier to identify and resolve issues. As quoted, 'Context helps you understand the conditions under which the log was generated, aiding in replicating and fixing issues.'
-
Avoid Recording Sensitive Information: Be cautious about documenting personal or sensitive data to prevent security risks. This is crucial for compliance with data protection regulations such as GDPR.
-
Use Parameterized Recording: Instead of string concatenation, utilize parameterized recording to enhance performance and maintainability. This practice guarantees that record-keeping does not disrupt the software's flow, preserving efficiency even under significant load.
-
Centralize Log Configuration: Maintain log configurations in a central file to facilitate changes and ensure consistency across the system. This method streamlines upkeep and guarantees that recording standards are consistently implemented.
-
Monitor Log Files: Regularly review log files for signs of issues or abnormal patterns that could indicate problems. 'Enhanced observability through detailed log monitoring improves the ability to detect and troubleshoot issues in reactive systems, as noted by experts, 'By capturing detailed information about HTTP interactions, Logbook enhances the ability to monitor and troubleshoot reactive systems.'' Applying these methods improves the dependability and effectiveness of your record-keeping approach, ultimately resulting in a more resilient and manageable system.
Choosing the Right Logging Framework
While the Java Logging API is a solid option, there are several alternative frameworks for recording events available, such as Log4j, SLF4J, and Logback. These frameworks offer unique advantages that can be crucial for different use cases. When choosing the right logging framework, consider the following factors:
- Performance: Evaluate how the logging framework impacts application performance, especially under high load. For example, Logbook's non-blocking design ensures that record-keeping does not interfere with the reactive flow of the application, maintaining performance even under heavy load.
- Flexibility: Look for a framework that allows for easy configuration and supports various output destinations. SLF4J, for instance, provides a common interface to register logs and allows you to replace the final output with different implementations, whether it's a console, file, or even a remote service.
- Community Support: A well-supported framework with an active community can offer better resources and help when needed. The ongoing contributions from the Java community, as highlighted by Oracle, reflect the strong support and continuous improvements in these tracking frameworks.
- Integration: Ensure that the tracking framework integrates well with your existing tools and libraries. Frameworks like Logbook integrate seamlessly with popular frameworks such as Spring Boot and Spring WebFlux, making it easy to add tracking features to existing software.
Choosing the suitable tracking framework can greatly influence your software's maintainability and performance, offering improved visibility and simpler problem-solving.
Advanced Logging Techniques
For applications that require more sophisticated logging strategies, consider these advanced techniques:
-
Asynchronous Logging: Implement asynchronous logging to enhance application performance by offloading logging activities to separate threads. This can significantly reduce latency, especially under high-load conditions. For instance, a case study highlighted the adoption of multi-cluster architecture to manage data spikes, ensuring fault isolation and minimizing instability.
-
Structured Logging: Use structured logging to capture data in a structured format, which is easily consumable by log analysis tools. This enhances searchability and analytics, making it simpler to identify patterns and troubleshoot issues. An example includes setting up a notification flow with clearly defined events, facilitating better log management and analysis.
-
Log Aggregation: Utilize tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk for aggregating logs from multiple sources. This provides a centralized view for monitoring and analysis, critical for maintaining operational insights. Despite the challenges, smaller companies have found the standard ELK stack sufficient for their needs, demonstrating its practicality and efficiency.
-
Dynamic Log Levels: Implement functionality to change log levels at runtime without restarting the software. This permits more detailed control over output, enabling dynamic adjustments based on the application's current state. This capability can drastically reduce the total cost of record-keeping by managing verbosity and log frequency more effectively.
These techniques not only enhance data recording performance but also make log information more actionable, supporting improved decision-making and quicker incident resolution.
Troubleshooting and Debugging with Logging
Efficient record-keeping is essential for diagnosing and fixing software issues. To maximize the benefits of logging, consider the following practices:
-
Trace Errors: Use detailed logs to trace the origin of errors. This includes capturing stack traces and contextual information that can reveal the error's source. A case study emphasized that Eylon, a developer, encountered challenges in tracking request flows across microservices because of the intricacy of contemporary systems. Thorough records can alleviate such challenges by offering a clear trail of events.
-
Log Contextual Data: Capture important contextual data such as user IDs, session information, and transaction details. This data helps in understanding the application's state during an event, making it easier to diagnose issues. As noted, capturing the behavior of asynchronous events is crucial, especially in complex systems where events might be pending or scheduled for future execution.
-
Regularly Review Records: Establish a routine for examining records to identify patterns or recurring issues. Logs offer a historical record of events, making it easier to trace and analyze production issues. A study involving over 750,000 crash reports showed that developers do not solely rely on the volume of records but also consider other factors like the difficulty of solving the issue and customer priorities.
-
Implement Alerts: Set up alerts for severe log entries to promptly notify relevant teams. This enables quick reactions to critical issues, minimizing downtime. For instance, tools like Fluent Bit, which handle large volumes of log data, are designed to be highly scalable and easy to use, making them ideal for cloud-based environments.
By effectively utilizing these logging practices, you can enhance the reliability of your application and reduce downtime. As one expert suggested, turning logs into a powerful tool can significantly improve software maintenance and performance.
Conclusion
The Java Logging API serves as a vital framework for managing log messages, enabling developers to enhance observability and troubleshoot issues effectively. By understanding its core components—loggers, handlers, formatters, and log records—developers can create a robust logging system tailored to their application's needs. Each component plays a significant role in ensuring that logs are both informative and actionable, facilitating better debugging and operational monitoring.
Utilizing the various log levels strategically is crucial for maintaining an efficient logging system. By differentiating between SEVERE, WARNING, INFO, and other levels, developers can prioritize log messages and filter out noise, ensuring that critical information is readily available when needed. Proper configuration of the Java Logging API, whether programmatically or via configuration files, further enhances the logging process, allowing for customized handlers and formatters that improve log readability and management.
Implementing best practices, such as logging meaningful messages, avoiding sensitive information, and centralizing configurations, can significantly improve the effectiveness of logging efforts. Moreover, choosing the right logging framework based on performance, flexibility, and community support can have a profound impact on application maintainability and observability.
Advanced logging techniques, including asynchronous logging, structured logging, and log aggregation, provide additional layers of sophistication, optimizing performance and making log data more actionable. By employing effective troubleshooting strategies, such as tracing errors and capturing contextual data, developers can turn logs into powerful diagnostic tools that enhance software reliability and reduce downtime. Embracing these practices ultimately leads to a more robust and maintainable application, paving the way for improved performance and user satisfaction.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.