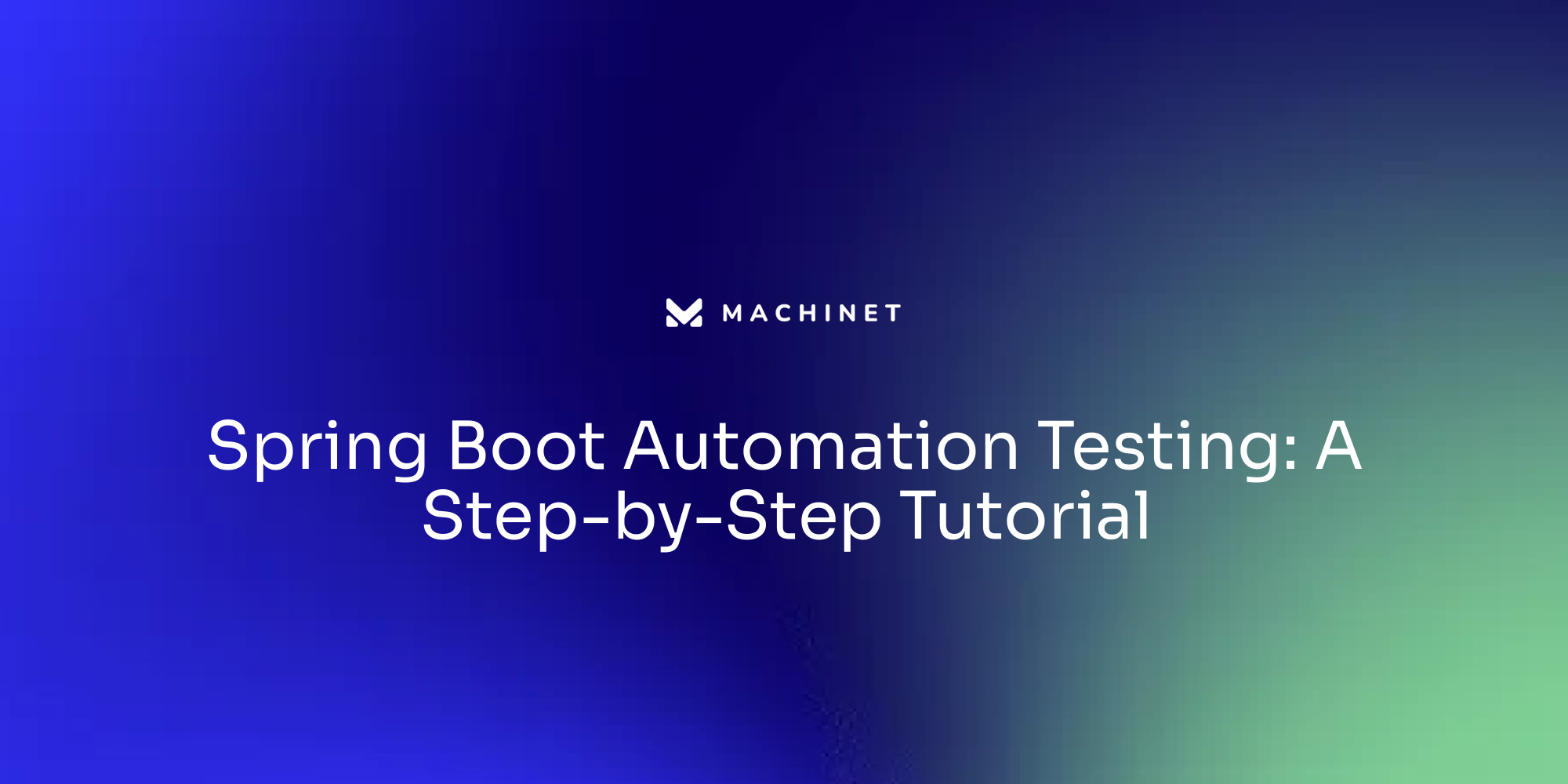
Introduction
In the fast-paced world of software development, ensuring the reliability and maintainability of applications is paramount. For developers working with Spring Boot, a robust testing strategy is essential to achieve these goals. This article delves into the comprehensive setup and execution of testing in Spring Boot projects, covering everything from the initial configuration to advanced testing techniques.
Readers will discover the importance of integrating essential libraries, configuring application properties for isolated testing environments, and the different types of tests crucial for maintaining application quality. Additionally, the article explores the use of popular tools like JUnit, Mockito, Selenium, and Cucumber, providing practical insights into unit, integration, and UI testing. The value of Continuous Integration and Continuous Deployment (CI/CD) pipelines is also highlighted, demonstrating how tools such as Jenkins, GitLab CI, and GitHub Actions can streamline and automate the testing and deployment processes.
By adhering to best practices and leveraging the powerful features of Spring Boot and its ecosystem, developers can ensure their applications are both scalable and reliable.
Setting Up a Spring Boot Project for Testing
To start automated evaluation in a Boot project, first ensure that you have the required dependencies in your pom.xml
or build.gradle
file. Essential libraries such as JUnit, Mockito, and Spring Test play a crucial role in this setup. JUnit, for example, is a widely used framework that enables developers to write repeatable assessments, ensuring code reliability and accuracy. Include these dependencies to harness the full potential of automated evaluation.
Next, configure your application properties for testing. This involves setting up test-specific configurations to isolate tests from production data. The Boot framework simplifies this process with its convention-over-configuration approach, reducing the amount of boilerplate code required. The auto-configuration feature further streamlines the setup, making the development experience more intuitive and efficient.
The modular structure of Boot and its vast array of libraries and integrations render it ideal for creating scalable and adaptable systems. Whether you're working on a monolithic software solution or a distributed microservices structure, Boot adapts easily to changing needs. This flexibility is bolstered by a large and active community of developers, providing ample resources, tutorials, and third-party integrations to support your development journey.
By following these procedures, you guarantee your Boot programs are strong, dependable, and easy to manage, facilitating swift development and effective evaluation methods.
Types of Tests in Spring Boot
Spring Boot supports various types of evaluations, each playing a crucial role in ensuring application quality. Unit evaluations concentrate on separate elements, assessing functions, methods, or classes in isolation to confirm they operate as expected. Integration evaluations confirm the interaction between various modules, ensuring they operate together as anticipated. Lastly, end-to-end (E2E) tests ensure the entire system works as intended, covering multiple layers of the application stack. Popular tools for E2E evaluation include Selenium, Cypress, and TestCafe. Comprehending these categories enables you to select the appropriate method for your evaluation requirements, contributing to a balanced and effective assessment strategy.
Unit Testing with JUnit 5 and Mockito
JUnit 5 offers a modular and adaptable framework for creating unit evaluations in Java, featuring advanced capabilities to enhance your assessment process. To incorporate Mockito for mocking dependencies, annotate your classes with @ExtendWith(MockitoExtension.class)
. Within these classes, define examination methods using the @Test
annotation and employ Mockito.when()
for specifying behaviors of mocked objects. Utilize assertions from the Assertions
class to validate that your code performs as expected. This method aids in guaranteeing the strength and dependability of your software.
Integration Testing with @SpringBootTest
For integration evaluation, the @SpringBootTest
annotation is essential as it loads the complete context of the system, enabling you to examine the integration of various components and services comprehensively. This practice ensures that your software behaves correctly under real-world conditions, interacting with actual databases rather than in-memory alternatives. Utilizing @MockBean
assists in simulating external services, enabling isolated evaluations, and ensuring that various components of your application function smoothly together. This methodology aligns with the principle that integration evaluation should verify the interfaces between different components, ensuring they function cohesively when combined. Employing tools such as Testcontainers can further improve your integration examinations by running actual services inside containers, offering consistency and isolation between examination runs.
Using Selenium with Spring Boot for UI Testing
Selenium is a robust instrument for automating websites, rendering it essential for user interface evaluation in Spring Boot projects. To integrate Selenium, start by setting up Selenium WebDriver within your project. This setup enables the creation of test cases that simulate real user interactions, ensuring your web application's interface functions correctly across various browsers.
Selenium's integration with Continuous Integration (CI) tools such as Jenkins, Travis CI, and CircleCI enables smooth automated evaluation as part of the development lifecycle. This approach enhances the efficiency and effectiveness of your evaluation process. Additionally, incorporating Java with Selenium leverages Java's simplicity, robustness, and platform independence, empowering developers to build sophisticated automation frameworks with ease.
For more advanced evaluation, consider using the Page Object Model (POM) design pattern. POM separates test scripts from page-specific code, making it easier to manage and update tests as your website evolves. Furthermore, Selenium WebDriver's ability to interact with various web elements and execute JavaScript actions greatly enhances your ability to handle complex web pages.
As one expert noted, 'Selenium WebDriver is more than just a tool—it's a key driver of reliability, stability, and performance in the digital landscapes we create.' This sentiment highlights the importance of mastering Selenium to achieve exceptional outcomes in automation. By combining Selenium with your Boot project, you guarantee a strong and dependable UI testing framework that can adjust and expand with your software's growth.
Integrating Cucumber for BDD Testing
Behavior-Driven Development (BDD) can be seamlessly integrated into a Spring Boot project using Cucumber, enhancing collaboration between developers, testers, and business stakeholders. Begin by creating feature files in Gherkin syntax, which outline the intended behavior of the software in a human-readable format. This ensures clear communication and serves as both documentation and test cases. Implement step definitions in Java to connect these scenarios to your code, allowing for the reuse of steps across different scenarios and early defect detection. This approach not only ensures that the application meets business requirements but also helps in maintaining clean, scalable code, leveraging Spring Boot's robust ecosystem and resources.
CI/CD Pipelines for Spring Boot Applications
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for automating your evaluation and deployment procedures, ensuring a smooth and dependable software development lifecycle. Tools like Jenkins, GitLab CI, and GitHub Actions stand out for their ability to integrate effortlessly with various development tools, providing a robust framework for CI/CD implementation.
Jenkins, one of the most widely used open-source automation servers, offers a vast ecosystem of plugins, allowing integration with different version control systems, build tools, and deployment platforms. This flexibility makes Jenkins an ideal choice for setting up CI/CD pipelines that automatically run tests on every code commit, catching potential issues early and promoting a smoother deployment process. As noted, “Jenkins automates parts of the software development process, including building, testing, and deploying code.”
Similarly, GitLab CI and GitHub Actions provide comprehensive solutions for automating workflows, with features tailored to meet diverse project requirements. These tools not only streamline the automation process but also offer continuous monitoring, providing feedback on performance, errors, and user behavior. This feedback loop is essential for maintaining the stability and reliability of releases, as highlighted by the insight, “Continuous monitoring tools monitor deployed applications, providing feedback on performance, errors, and user behavior.”
The adoption of CI/CD practices fosters a culture of collaboration and efficiency, significantly enhancing the quality of software. Automated evaluations and integration processes instill confidence in the stability and reliability of software releases, reducing the risk of post-release issues. This approach is transformative but not without its challenges, such as slow build times and integration issues, which require a strategic mindset to navigate successfully.
In summary, leveraging CI/CD tools like Jenkins, GitLab CI, and GitHub Actions can dramatically improve the software development process, ensuring timely and reliable software updates while overcoming common challenges associated with CI implementation.
Best Practices for Spring Boot Testing
Adhering to best practices in testing can significantly improve the quality of your Spring Boot applications. Compose clear and concise evaluations, ensuring that each assessment has a single responsibility and employs meaningful names for your case studies. Consistently examine and improve your examination code to remove redundancy and guarantee maintainability.
Key components to focus on include:
- Test Case ID: A unique identifier for easy reference and tracking.
- Case Title: A concise and descriptive title that reflects the purpose of the evaluation.
- Preconditions: The necessary conditions that must be satisfied before executing the evaluation case.
- Input Data: The specific data inputs or conditions needed for the evaluation.
- Steps to Reproduce: A step-by-step guide on how to carry out the case.
- Expected Results: The anticipated outcomes based on the specified inputs and conditions.
Understanding these components is crucial for ensuring the robustness and effectiveness of the testing process. Each test case should have specific objectives, outlining what aspect of the software's functionality or performance it aims to evaluate. This structured approach not only enhances the quality of your code but also helps in maintaining a healthy codebase, reducing the implementation time for new features and bug fixes.
Conclusion
In the realm of software development, particularly with Spring Boot, a comprehensive testing strategy is essential for delivering reliable and maintainable applications. The initial setup, involving the integration of key libraries such as JUnit and Mockito, lays the foundation for effective automated testing. Properly configuring application properties for isolated testing environments further enhances the robustness of the testing process, allowing developers to focus on writing high-quality, scalable code.
Understanding the different types of tests—unit, integration, and end-to-end—enables developers to adopt a balanced approach to quality assurance. Each test type serves a specific purpose, ensuring that individual components work correctly, that modules interact seamlessly, and that the entire application functions as intended. Utilizing tools like Selenium for UI testing and Cucumber for Behavior-Driven Development (BDD) enhances the testing strategy, promoting collaboration and ensuring that the application meets both technical and business requirements.
The integration of Continuous Integration and Continuous Deployment (CI/CD) pipelines, facilitated by tools such as Jenkins, GitLab CI, and GitHub Actions, automates testing and deployment processes, thereby improving the overall software development lifecycle. This automation not only reduces the likelihood of post-release issues but also fosters a culture of collaboration and efficiency among development teams.
By adhering to best practices in testing, developers can significantly elevate the quality of their Spring Boot applications. Clear, concise tests with well-defined objectives and structured components will ensure that the testing process is effective and maintainable. Ultimately, leveraging the powerful features of Spring Boot and its ecosystem equips developers to build applications that are not only functional but also resilient and adaptable to future challenges.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.