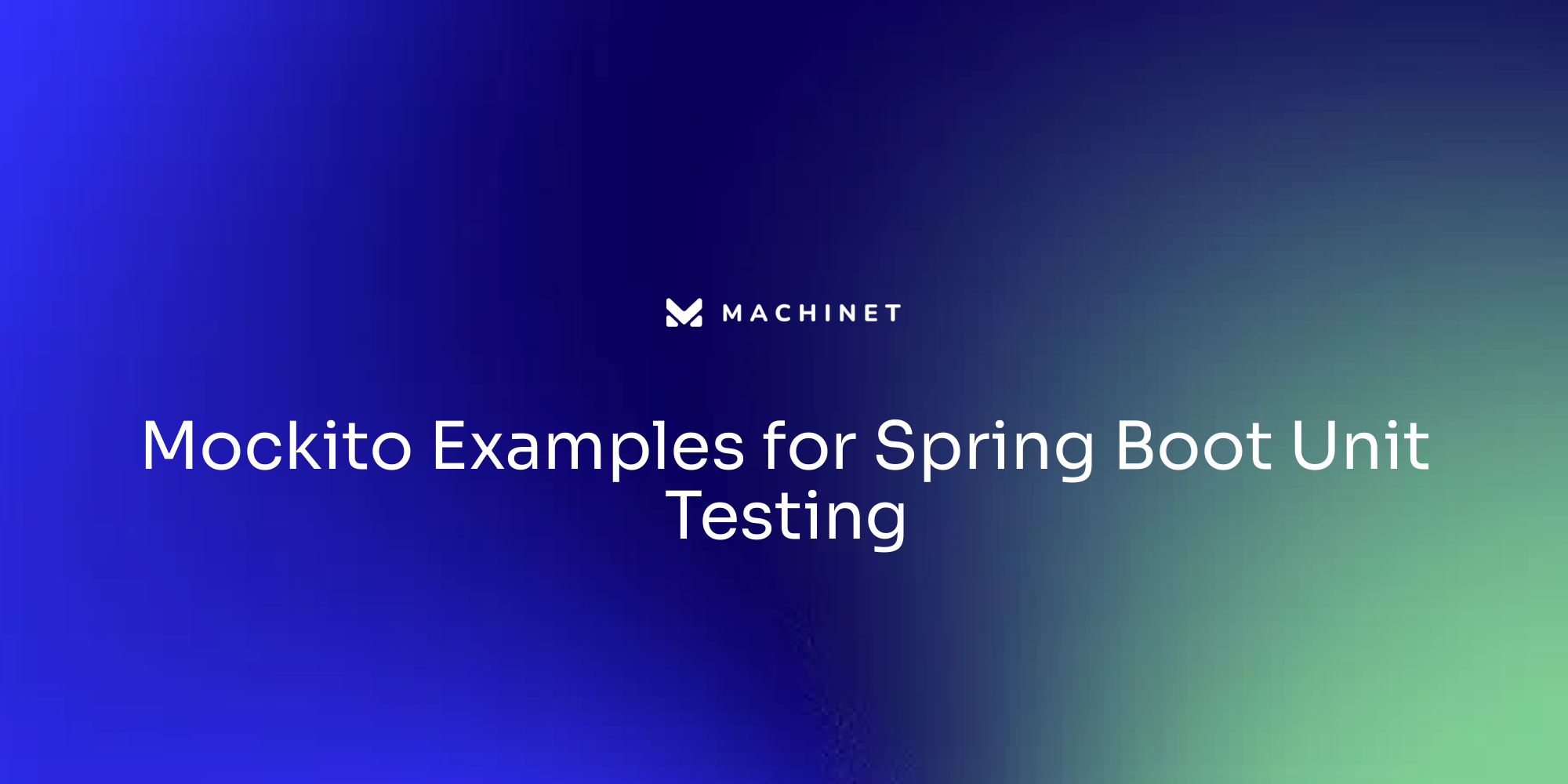
Table of Contents
- Understanding Mockito and Its Role in Unit Testing
- Setting Up Mockito for Spring Boot Unit Testing
- Mockito Tutorial: Basic Concepts and Terminology
- Writing Unit Tests Using Mockito and JUnit for Spring Boot Applications
- Advanced Techniques: Mocking Complex Scenarios with Mockito
- Refactoring Existing Test Suites with Mockito
- Strategies to Handle Changing Requirements in Unit Testing with Mockito
- Balancing Workload and Deadlines in Unit Testing using Mockito
Introduction
Unit testing is an essential part of software development, and Mockito is a powerful Java testing framework that enhances the efficiency and reliability of unit tests. By creating mock objects that mimic the behavior of real objects, Mockito allows developers to isolate and test specific components in isolation. This not only simplifies the testing process but also improves the quality and stability of the code.
In this article, we will explore the fundamentals of Mockito and its role in unit testing. We will discuss how to set up Mockito for unit testing, including adding dependencies and creating mock objects. Additionally, we will delve into advanced techniques such as mocking complex scenarios and refactoring existing test suites using Mockito. With Mockito's ability to handle changing requirements and balance workload and deadlines, developers can write more effective and efficient unit tests for their Java applications. So let's dive into the world of Mockito and discover how it can revolutionize your unit testing practices
1. Understanding Mockito and Its Role in Unit Testing
Mockito, an open-source testing framework for Java, is a crucial tool for the creation of mock objects, enhancing unit testing efficiency. When the need arises to isolate the component under test from its dependencies, Mockito shines. This framework allows developers to create and manage mock objects that mirror the behavior of complex real objects, simplifying testing of individual components in isolation. The result is more reliable code that operates predictably across various scenarios.
To utilize Mockito for unit testing, certain steps need to be followed.
Learn how to use Mockito for unit testing
The first step involves adding the Mockito dependency to your project. The creation of mock objects is then possible using the Mockito.mock()
method. These mock objects are used to simulate the behavior of dependencies in your unit tests. The next step is defining the expected behavior of these mock objects using Mockito's when()
and thenReturn()
methods. Finally, tests are executed and the interactions with the mock objects are verified using Mockito's verify()
method.
Mockito is a prominent mocking framework for Java unit testing.
Explore the benefits of using Mockito for Java unit testing
It aids developers in creating mock objects, simulating real object behavior, to isolate and test specific code portions. Mockito's simple and intuitive API for creating and configuring mock objects makes writing effective unit tests easier.
For best practices in using Mockito for unit testing in Java, several guidelines are recommended. These include using Mockito to create and configure mock objects for better control and isolation of the code under test. Mockito's verify() method should be used to ensure specific methods are called on the mock objects during the test. Mockito's when() method should be used to specify mock object behavior when certain methods are called. Argument matchers provided by Mockito can make test cases more flexible and reusable. Mockito's annotations, such as @Mock, @InjectMocks, and @Spy, simplify the setup of mock objects. Mockito's reset() method should be used to reset the state of mock objects between test cases. Mockito's annotations, such as @Before and @After, should be used to perform setup and teardown operations before and after each test case. Mockito should be integrated with the test framework using annotations like @RunWith(MockitoJUnitRunner.class) or @ExtendWith(MockitoExtension.class).
To mock dependencies in Java, Mockito can be used. Mockito, a popular Java framework, enables the creation of mock objects for testing purposes. By using Mockito, the behavior of dependencies can be simulated and the code to be tested can be isolated. The steps to mock a dependency using Mockito include creating a mock object using the Mockito.mock()
method, setting up the mock object using the Mockito.when()
method, and injecting the mock object into your test code instead of the real dependency.
The "ports and adapters" architecture, also known as the "hexagonal" architecture, is effective in managing external dependencies. A small exercise that explores refactoring and the "ports and adapters" architecture is the birthday greetings kata. This exercise is useful in understanding the practical application of these concepts. Notably, the authors Steve Freeman and Nat Pryce popularized the concept of mocks in their book "Growing Object-Oriented Software, Guided by Tests".
In summary, Mockito is a powerful tool for Java developers, enabling the creation of mock objects that mimic complex, real objects for testing purposes. Its proper use, understanding, and best practices can significantly enhance the reliability and efficiency of unit testing
2. Setting Up Mockito for Spring Boot Unit Testing
Integrating Mockito into a Spring Boot project commences with the inclusion of the Mockito dependency. This is achievable with Maven by adding the following dependency into your pom.xml file:
xml
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>3.11.2</version>
<scope>test</scope>
</dependency>
Alternatively, in Gradle, the Mockito dependency can be included by adding the following code snippet to the dependencies section of your build.gradle file:
java
testImplementation 'org.mockito:mockito-core:VERSION_NUMBER'
Remember to replace VERSION_NUMBER
with the desired version of Mockito. After integrating the Mockito dependency, Mockito can be utilized in your test suite. The @Mock
annotation can be utilized to generate a mock object, while the @InjectMocks
annotation can be used to inject the mock into the class under test. Also, you need to initialize the mocks by calling MockitoAnnotations.initMocks(this)
in the setup method of your test class. This will initialize all the mock objects annotated with @Mock
.
Unit testing is a method used to scrutinize specific, isolated portions of code, excluding any integration with other parts of the application. Automated tests, including unit tests, are executed automatically during the application's build phase. To write unit tests in Spring Boot, you require a testing library such as JUnit, an assertion library like AssertJ, and a mocking framework like Mockito.
Spring Boot provides a starter that includes all three libraries. Therefore, you only need to add the dependency to your project. When writing a unit test, a test class is created in the same package as the class you want to test. Annotations like @Test
and @ParameterizedTest
are used to define the test methods.
The assertThat
method from AssertJ can be used to make assertions in your tests. Parameterized tests allow for the execution of the same test with varying input parameters. Mockito can be used to mock dependencies and control their behavior in your tests. Mockito's when
method allows for the specification of the return value of a method call on a mock object. Mockito's verify
method enables the verification of a method on a mock object that was called with specific arguments. ArgumentCaptor can be used to capture arguments passed to a mock object and make assertions on them.
Mockito's thenAnswer
method allows for specifying a custom behavior for a mock object. AssertJ provides powerful assertions for collections, allowing for assertions on the contents of a collection. Spring provides built-in mock implementations for common classes like MockHttpServletRequest
and MockHttpServletResponse
. The choice of what to test will depend on your team's testing goals, but ideally, all business logic should be tested. Unit tests should focus on behavior and not implementation details. Configuration classes are better tested with integration tests or end-to-end tests. The number of unit, integration, and end-to-end tests you write will depend on your team's needs and priorities
3. Mockito Tutorial: Basic Concepts and Terminology
Mockito, a prevalent Java library, simplifies the creation and manipulation of mock objects by offering a host of annotations and methods. Let's explore some of its fundamental concepts:
@Mock
: This annotation is a convenient tool for generating a mock object, enabling isolated testing of class functionalities. According to the Solution Context, the@Mock
annotation is used to create a mock object of a class or interface, primarily in unit testing to simulate the behavior of dependencies. This eliminates the need for manual scripting of mock objects.
java
@Mock
SomeClass someObject;
@InjectMocks
: This annotation is used to inject mock objects into the class under test, negating the need for database connections or file reading, and simplifying the testing process. As per the Solution Context, the@InjectMocks
annotation works in conjunction with@Mock
or@Spy
annotations. Mockito automatically identifies the mocks/spies annotated with@Mock
or@Spy
and injects them into the target object or class.
java
@InjectMocks
SomeClassUnderTest someObjectUnderTest;
when()
: This method is employed to define the behavior of the mock object when a specific method is invoked. It enables the mock object to return dummy data corresponding to dummy input.
java
when(someObject.someMethod()).thenReturn(someValue);
verify()
: This method is used to validate that a specific method was indeed invoked on the mock object. It supports checking the order of method calls, enhancing the precision of the testing process. The Solution Context clarifies thatverify()
ensures the expected method calls are made on your mock objects during your unit tests, which is vital for verifying the behavior of your code under test and ensuring its correct interaction with its dependencies.
java
verify(someObject).someMethod();
thenReturn()
: This method is applied to determine the value that should be returned when a specific method is called on the mock object. It supports both return values and exceptions, providing a comprehensive testing scope. As per the Solution Context,thenReturn()
is a powerful tool in Mockito that allows you to define the behavior of a mock object and ensure that your unit tests are accurate and reliable.
java
when(someObject.someMethod()).thenReturn(someValue);
Through this brief overview, one can understand how Mockito uses Java reflection to create mock objects for a given interface seamlessly. Moreover, Mockito is refactoring-safe, meaning changes to interface method names or parameters will not break the test code.
To illustrate these concepts in action, consider a stock service scenario wherein a dummy implementation is required for testing. Mockito can be used to create a mock object of the stock service, simulate the behavior of the stock service to return the values of various stocks, and even verify the market value to be a specific value. This case study exemplifies how Mockito can enhance testing efficiency while ensuring accurate results
4. Writing Unit Tests Using Mockito and JUnit for Spring Boot Applications
Let's explore a hands-on example of applying Mockito within a Spring Boot application. We will use a UserService
class as an example, which has a dependency on a UserRepository
class. Mockito can be used here to create a mock UserRepository
, which can then be used within the UserService
for testing purposes.
Consider the following code snippet:
```java @RunWith(MockitoJUnitRunner.class) public class UserServiceTest {
@Mock
private UserRepository userRepository;
@InjectMocks
private UserService userService;
@Test
public void testFindUserByEmail() {
User user = new User("test@example.com");
when(userRepository.findByEmail("test@example.com")).thenReturn(user);
User result = userService.findUserByEmail("test@example.com");
assertEquals(user, result);
}
} ```
In this test scenario, Mockito is used in a specific way. It is instructed that whenever findByEmail()
is called on the userRepository
mock with the argument "test@example.com", it should return the user
object. Then, findUserByEmail()
is called on the userService
and it's checked that the returned value matches the user
object. This demonstrates Mockito's ability in managing dependencies during testing, allowing for precise and controlled test cases.
To use Mockito in Spring Boot, you can follow these steps:
- Add the Mockito dependency to your project's build configuration file (pom.xml for Maven or build.gradle for Gradle).
- Create a test class for your Spring Boot application or a specific component that you want to test.
- Import the necessary Mockito and Spring Boot test annotations in your test class, such as
@RunWith(MockitoJUnitRunner.class)
and@SpringBootTest
. - Use the Mockito framework to mock any dependencies that your Spring Boot component relies on. You can do this by using the
@Mock
annotation on the respective fields and initializing them with mock objects. - Set up the desired behavior of the mocked dependencies using Mockito's
when
andthenReturn
methods. This allows you to simulate specific responses or behaviors for testing purposes. - Write your test cases using the mocked dependencies and any other necessary assertions or verifications.
- Run the test cases using your preferred test framework, such as JUnit, and verify that the expected behavior is achieved.
By following these steps, you can effectively use Mockito in Spring Boot for unit testing your application or specific components. This helps in ensuring that your code is functioning as expected and producing the desired output
5. Advanced Techniques: Mocking Complex Scenarios with Mockito
Mockito, a leading framework for unit testing in Java, offers a rich set of features that cater to a multitude of complex mocking scenarios. One such feature is the creation of mock objects. Mockito enables you to form mock instances of classes or interfaces, which can then be manipulated using Mockito's API for specific behavioral outcomes during testing.
One of the powerful methods in Mockito's repertoire is doThrow()
. This method, when used in conjunction with when()
, enables the simulation of exceptions, enhancing the testing of error handling in your code.
java
// Simulate an exception
doThrow(new RuntimeException()).when(mockObject).someMethod();
In addition to this, Mockito provides the ArgumentCaptor
class, a feature that proves invaluable when you need to make assertions based on the arguments passed to a mock object. The ArgumentCaptor
captures these arguments, allowing you to verify their values or states later in your test code.
java
// Use ArgumentCaptor
ArgumentCaptor<String> argumentCaptor = ArgumentCaptor.forClass(String.class);
verify(mockObject).someMethod(argumentCaptor.capture());
One of Mockito's standout features is its ability to create 'partial mocks' using the spy()
method. A partial mock is a blend of a real object and a mock object, which is especially useful when the need arises to mock certain methods of an object while allowing other methods to retain their original behavior. This is achieved by creating a spy of a real object and then using the doReturn
or when
methods to define the behavior of specific methods.
java
// Create a partial mock
MyClass myObject = Mockito.spy(new MyClass());
// Define behavior of a specific method
Mockito.doReturn("Mocked Value").when(myObject).someMethod();
Furthermore, Mockito allows the mocking of final classes and methods through the PowerMock extension, although it's important to note that private methods and fields are beyond its reach. This proves particularly useful when dealing with legacy code or third-party libraries that may not be easily modified.
Mockito's when().thenReturn()
and doReturn().when()
methods allow for the configuration of return values of method calls on mock objects, giving you control over the test environment. The verify()
method further enriches the testing process by allowing for the verification of method calls on mock objects.
In summary, Mockito's advanced features, coupled with its support for spies and reflection to alter private fields, make it an indispensable tool for unit testing in Java, providing a controlled and flexible avenue for testing complex scenarios and code behavior
6. Refactoring Existing Test Suites with Mockito
Refactoring an existing test suite with Mockito can significantly improve its efficiency and functionality. The first step in this process is identifying the dependencies of the class under test. These dependencies can then be replaced with mock objects using the @Mock
annotation, which Mockito provides. The behavior of these mock objects can be specified using the when()
method. The verify()
method can then be used to ensure the interaction between the class under test and its dependencies is as expected. This approach not only simplifies your tests but also reduces the chance of test failures due to changes in unrelated sections of the code.
Mockito is a widely used Java mocking framework that enhances the quality of your tests by ensuring that your test code is concise and easily understandable. It's essential to avoid redundancy in tests and focus on evaluating the input and output. Aim to cover a wide range in your tests, including successful cases and error-prone code paths.
However, it's important to note that you should avoid mocking a type you don't own as it can lead to complications in design and integration. Overuse of mocking in your tests could potentially be an anti-pattern, as it might not effectively test the production code. Unless it's challenging to create new fixtures, value objects should not be mocked. Instead, builders or factory methods can be used.
Viacheslav Aksenov, a backend developer specializing in Java and Kotlin, emphasizes the importance of writing tests for your code as it leads to improved code quality, faster development, easier debugging, and increased confidence in your code. He further stresses the importance of Test-driven development (TDD), a methodology where tests are written before the implementation code.
Investing time in writing tests is a valuable practice. For further insights into testing, consider reading "Growing Object-Oriented Software Guided by Tests." You can also join the Mockito mailing list for additional discussions and support.
Refactoring your existing test suite to use Mockito gives you more control over the dependencies used in your tests, making them more focused and easier to maintain. Mockito's when()
method allows you to define what should happen when a specific method is called on the mock object. This helps in setting up the desired behavior of the mock object for your unit tests.
The verify()
method in Mockito is used to verify interactions with dependencies in unit testing. It allows you to check whether certain method calls or interactions with mocked objects have occurred during the execution of your test. By using the verify()
method, you can ensure that the expected interactions with your dependencies have taken place, providing a way to validate the behavior of your code.
By using Mockito, a mocking framework for Java unit testing, you can isolate the code being tested from its dependencies.
Discover how Mockito enhances the reliability of your unit tests
This can help reduce the number of test failures caused by changes in unrelated code.
To refactor test suites effectively using Mockito, it's important to clearly define the scope and purpose of each test case. Using Mockito's mocking capabilities effectively includes creating mock objects for any external dependencies that the code being tested relies on. These mock objects can be used to simulate the behavior of the real dependencies, allowing for more isolated and controlled testing.
Finally, it's important to keep the test suite well-organized and maintainable. This involves following naming conventions for test methods, grouping related test cases together, and regularly reviewing and refactoring the test suite as needed. By following these best practices, you can use Mockito effectively in test suite refactoring to improve the quality and maintainability of your code
7. Strategies to Handle Changing Requirements in Unit Testing with Mockito
In the dynamic realm of software development, evolving requirements and constant changes are the norm. A crucial aspect of navigating this fluid landscape is maintaining the adaptability and integrity of your codebase. A powerful ally in this endeavor is Mockito, a widely used Java testing framework.
Mockito empowers developers to create mock objects, acting as placeholders for real objects within a system. These mock objects can be manipulated to mimic varying behaviors, thereby enhancing the flexibility and adaptability of your tests. This adaptability is particularly beneficial when dealing with fluctuating requirements. Through Mockito, you can adjust the behavior of mock objects to mirror new requirements, while simultaneously isolating the component under test from its dependencies. This isolation safeguards your tests from any disruptions caused by changes in dependencies, thereby preserving their integrity.
The use of Mockito extends beyond merely creating mock objects. It fosters interaction-based testing, a cornerstone of object-oriented programming. This method prioritizes the interactions between objects over their state, offering more profound insights into your system's behavior.
While Mockito is a powerful tool for isolating components and managing dependencies, it does not address all testing challenges. For instance, when working with code dependent on external systems such as databases or web services, using an adapter is advisable. An adapter facilitates a connection between your code and the external system, offering an optimal API for interaction. Directly mocking the external API might complicate testing. However, utilizing an adapter can mitigate these complications, leading to simpler and more straightforward tests.
It's worth noting that Mockito's utility isn't exclusive to Java. It can also be applied with other languages such as Kotlin and Python, making it a versatile tool for a broad spectrum of developers. Regardless of whether you are a seasoned Java developer or a Python novice, Mockito can assist you in navigating the ever-evolving landscape of software development with ease and efficiency.
In essence, whether you're grappling with changing requirements, managing dependencies, or simply striving to write more reliable and maintainable code, Mockito provides a robust and flexible solution. From creating mock objects to promoting interaction-based testing, Mockito equips you with the tools required to confront the challenges of software development head-on
8. Balancing Workload and Deadlines in Unit Testing using Mockito
In the challenging sphere of software development, creating a balance between workload and deadlines is paramount. Mockito, a Java-based mocking framework, can be instrumental in striking this balance by enhancing the efficiency of your testing processes.
The ability to create mock objects using Mockito eliminates the necessity for intricate setup and teardown of test environments, which can be time-consuming. This aspect significantly quickens your testing process, thereby aiding in meeting project deadlines without compromising on quality.
The syntax of Mockito is not only clear but also succinct, making it easier to write and maintain tests and thus, reducing the workload for your team. This is akin to adhering to the principle of a "sustainable pace" in agile software development, which emphasizes consistent and frequent delivery of value to customers.
However, despite the proven success of these agile practices, many software organizations neglect the importance of working at a sustainable pace. This oversight often leads to unrealistic deadlines set by company leaders, resulting in overworked teams and a compromise on quality.
To avoid such scenarios, teams can assert their influence by setting limits on working hours and making overtime visible to management. They can also slice stories into small, consistently sized increments and limit work in progress to maintain a predictable and consistent cadence. This approach aligns with the use of Mockito, which allows for efficient and effective testing.
The importance of a learning culture cannot be overstated. Allowing time for continuous learning can help teams improve their performance and deliver small changes consistently. This culture can be fostered with tools like Mockito, which not only help reduce the testing time but also promote a better understanding of the codebase, leading to improved productivity.
However, it's important to remember that failing to maintain a sustainable pace can lead to burnout and high turnover. These are costly consequences for any organization. Thus, using tools like Mockito can help in maintaining this balance by making unit testing more efficient.
Mockito's ability to create mock objects and its clear, concise syntax can significantly reduce the time it takes to run your tests, thereby making it easier to meet your deadlines and reducing the workload on your team. Hence, Mockito is not just a tool but a catalyst for fostering a sustainable pace in software development
Conclusion
In conclusion, Mockito is a powerful Java testing framework that enhances the efficiency and reliability of unit tests. By creating mock objects that mimic the behavior of real objects, developers can isolate and test specific components in isolation. This simplifies the testing process and improves the quality and stability of the code. Mockito's ability to handle changing requirements and balance workload and deadlines makes it an invaluable tool for writing effective and efficient unit tests for Java applications.
Furthermore, Mockito provides advanced techniques such as mocking complex scenarios and refactoring existing test suites. These techniques allow developers to simulate exceptions, capture method arguments, create partial mocks, and even mock final classes and methods. By utilizing these features, developers can tackle complex testing scenarios with ease.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation. Start now
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.