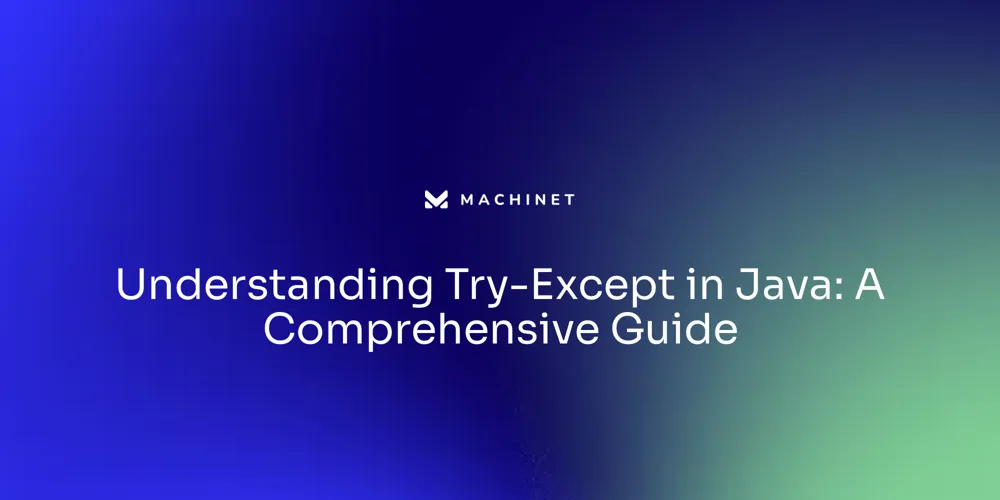
Table of Contents
- What is Try-Except in Java?
- Syntax of Try-Except
- Types of Exceptions in Java
- Handling Exceptions with Try-Except
Introduction
The try-except block in Java is a powerful mechanism that enhances the reliability of applications by effectively managing runtime exceptions. Exceptions, which are events that interrupt the normal program flow, can be caught and handled by catch blocks, allowing developers to mitigate potential disruptions.
In this article, we will explore the syntax of the try-except block, the types of exceptions in Java, and best practices for handling exceptions. By understanding and implementing proper exception handling, developers can build robust and fault-tolerant Java applications.
What is Try-Except in Java?
In Java, the try-except block is a sophisticated mechanism that enhances an application's reliability by managing runtime exceptions effectively. An exception, which is essentially an event that interrupts the normal program flow, is an object that can be thrown at runtime.
These exceptions can be meticulously caught by catch blocks, enabling developers to mitigate potential disruptions. Implementing such exception handling is not just about avoiding abrupt program terminations; it's about fortifying the application against unforeseen errors, thus making Java applications more robust and fault-tolerant.
Exceptions in Java are broadly classified into two categories, which allow developers to distinguish between recoverable errors and more critical issues. For example, a NumberFormatException
could be thrown by Integer.parseInt
, indicating a recoverable error that can be gracefully handled within the code.
As James Gosling highlighted in an interview, Java was designed with a strong emphasis on robustness, especially in networking environments, where handling errors efficiently is paramount. Recent Java versions have seen significant adoption, reflecting the language's ongoing importance and the developer community's efforts to keep it vibrant. Within six months of its release, Java 21 was being used by 1.4% of applications monitored by New Relic, a substantial increase compared to the 0.37% adoption seen with Java 17 in a similar timeframe post-release. This trend underscores the growing reliance on Java's stability and its advanced features like exception handling, as nearly 35% of applications in 2023 were using Java 17 in production, indicating a nearly 300% growth rate within a year.
Syntax of Try-Except
In Java, the try-except block, also referred to as try-catch, is critical for managing exceptions that can disrupt the normal flow of an application. The structure of this block is intuitive yet powerful, encompassing a 'try' section where code susceptible to exceptions is placed. Following this, one or more 'catch' blocks trail to capture and handle specific exception types.
Optionally, a 'finally' block can be added to execute code regardless of the occurrence of an exception, ensuring that certain actions are performed, such as resource cleanup. Here's a basic example:
java
try {
// code that may throw an exception
int result = Integer.parseInt("123");
} catch (NumberFormatException e) {
// NumberFormatException is a runtime exception
// code to handle the exception
System.out.println("Parsing error: " + e.getMessage());
} finally {
// code that will always run
System.out.println("The 'try catch' block has been executed. ");
}
This code demonstrates the handling of a NumberFormatException
, which is a runtime exception that can arise from invoking Integer.parseInt
with an invalid string.
If the string cannot be parsed as an integer, the exception is caught, and a user-friendly error message is printed, rather than allowing the program to terminate abruptly. Finally, a message indicating the completion of the try-catch block is printed, showcasing the use of the 'finally' block. It is essential to note that exceptions in Java can include both compile-time and runtime errors, and handling them effectively is crucial for building robust and fault-tolerant applications.
Types of Exceptions in Java
In Java, exception handling is a robust mechanism that enhances the reliability of applications. When an exception, which is an object indicating a disruption in the program flow, is thrown at runtime, it can be caught by catch blocks.
This prevents the program from terminating abruptly, thereby improving fault tolerance. There are two main types of exceptions in Java: checked and unchecked.
Checked exceptions, such as IOExceptions, are verified during compile-time and must be either caught or declared in the method's signature. Unchecked exceptions, like IndexOutOfBoundsException or NullPointerException, inherit from RuntimeException and do not require explicit handling.
These often indicate incorrect API usage and are not checked at compile-time. Errors represent serious issues that usually cannot be handled, as they are often related to system-level problems or resource constraints.
For example, a NumberFormatException, which may arise from calling Integer. ParseInt with an invalid string, is a runtime exception that can be handled, but is not required to be caught at compile-time. The recent release of Spring Integration 6.2.0 and Spring Session 3.2.0, with their host of improvements and new features, showcases the ongoing momentum in the Java community, as noted by Sharat Chander of Oracle. These enhancements continue to keep Java vibrant, with a strong focus on robustness and networking, as was the intention since its creation. As Java technology advances, understanding and implementing proper exception handling remains crucial for developers to ensure robust, fault-tolerant Java applications.
Handling Exceptions with Try-Except
In Java, the try-except block is a foundational construct for managing exceptions, which are events that disrupt the normal operation of a program. When coding, it's essential to anticipate and handle these exceptions to prevent abrupt program termination.
Here's an approach to refining exception handling for robust Java applications:
-
Specificity in Catching Exceptions: It's crucial to catch specific exceptions to avoid masking other errors. Catch blocks should be designed to handle only the exceptions they are intended for, providing clarity and precision in error handling.
-
Structured Catch Blocks: Employ multiple catch blocks for different types of exceptions. This structure allows for tailored responses to each exception type, enhancing the program's resilience.
-
Graceful Exception Handling: When an exception occurs, provide meaningful feedback to the user. This can involve logging the exception details or offering alternative solutions, rather than allowing the program to crash.
-
Cleanup with Finally: Use the finally block to perform necessary cleanup tasks. This block executes regardless of whether an exception was thrown, ensuring resources are properly released. Java's exception handling mechanism is a testament to its reliability as a programming language, as it empowers developers to craft fault-tolerant applications. With the recent updates in Java libraries like JobRunr and Spring Integration, the language continues to evolve, improving compatibility and functionality for developers worldwide. As such, understanding and implementing these best practices in exception handling is more than just error managementβit's about contributing to the ongoing vibrancy and robustness of Java applications.
Conclusion
In conclusion, the try-except block in Java is a powerful mechanism for managing runtime exceptions and enhancing the reliability of applications. By catching and handling exceptions with catch blocks, developers can mitigate potential disruptions and build robust and fault-tolerant Java applications. The syntax of the try-except block is intuitive yet powerful, with the ability to include a 'finally' block for executing code regardless of an exception occurrence.
Understanding the types of exceptions in Java, such as checked and unchecked exceptions, is crucial for effective exception handling. When handling exceptions in Java, it is important to be specific in catching exceptions, use structured catch blocks for tailored responses, provide meaningful feedback to users, and perform necessary cleanup tasks using the finally block. Java's ongoing updates and improvements in libraries like JobRunr and Spring Integration showcase its vibrancy and robustness.
By implementing best practices in exception handling, developers contribute to the reliability and success of Java applications. Overall, proper exception handling is essential for building fault-tolerant Java applications that can withstand unforeseen errors and disruptions. With its advanced features and strong emphasis on robustness, Java continues to be a reliable programming language for developers worldwide.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.