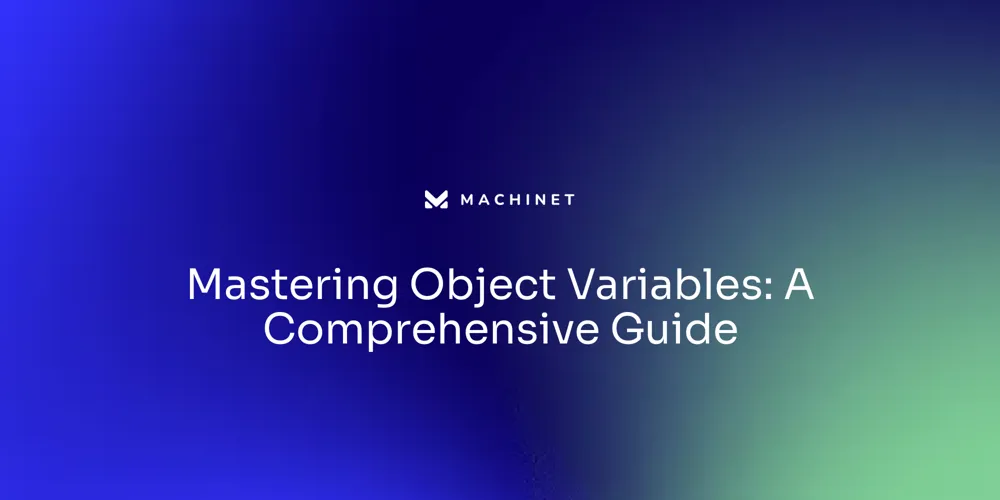
Table of Contents
- Understanding Object Variables
- Creating and Assigning Object Variables
- Accessing Object Variable Values
- Modifying Object Variable Values
Introduction
Understanding Object Variables
Bash scripting offers a versatile way to orchestrate various tasks and manage data through object variables, which are akin to containers that can hold different types of information. This article explores the concept of object variables in Bash, including their creation, assignment, and access. By understanding how to work with object variables, you can enhance your Bash scripts and efficiently manage data.
Understanding Object Variables
Bash scripting offers a versatile way to orchestrate various tasks and manage data through object variables, which are akin to containers that can hold different types of information. For instance, you can use object variables to store strings, integers, or arrays, and even create associative arraysβsimilar to hashmaps in other programming languages.
Associative arrays are particularly useful for storing key-value pairs, which can be declared and manipulated with built-in commands like 'declare -A'. This capability is demonstrated when engineers need to swiftly map data, such as GPU types to specific regions, under tight project timelines.
For example, in a high-pressure situation where requirements are fixed and time is of the essence, using Bash's associative arrays can be a lifesaver for quick data management and retrieval. Rapid prototyping and iteration are crucial in such scenarios, and Bash variables offer the flexibility needed to keep up with the pace. As technology evolves and the developer community continues to grow, tools like Bash remain integral for efficient software development. The ability to quickly define and access nested data structures within Bash scripts is a testament to the language's adaptability and enduring relevance in the fast-paced world of programming.
Creating and Assigning Object Variables
In Bash, variables are not just strings or numbers, they can also be more complex data structures like arrays and hashmaps. For instance, when you want to create a simple variable to store a name, you would do so with the syntax variable_name='value'
.
An example of this would be setting username='John'
, thereby defining a string variable. It's important to remember that these variables are case-sensitive, meaning username
and Username
are recognized as distinct entities by the shell.
To handle more complicated data structures, Bash provides hashmaps, which are incredibly useful for storing key-value pairs. For example, if you wanted to map a GPU kind to a region name, you'd begin by declaring the hashmap with the declare -A
command.
Then, you could add entries to this hashmap with a simple syntax, like gpu_map["nvidia"]="us-west"
. This allows for efficient storage and retrieval of associated data. Variables in Bash are versatile, and understanding how to create and manipulate them is essential for managing data within your scripts. Whether you're storing a single value or a collection of data points, Bash provides the tools necessary to keep your code organized and maintainable.
Accessing Object Variable Values
When working with Bash scripts, handling variables efficiently can greatly streamline your coding process. For instance, imagine you've set up a hashmap to pair GPU types with their corresponding regions.
To do this in Bash, you'd start by declaring the hashmap using the declare
built-in command. Once your hashmap is declared, you can dynamically add values as needed.
Here's an example from the fly. Io documentation that demonstrates adding values to a hashmap:
bash
declare -A gpu_region_map
gpu_region_map["NVIDIA"]="us-west"
gpu_region_map["AMD"]="eu-central"
You can even set up your hashmap as read-only by predeclaring it with values, ensuring that it remains unmodified during script execution.
Accessing the stored values is straightforward: use the same syntax you used to set them. For instance, to retrieve the region for an NVIDIA GPU, you'd use gpu_region_map["NVIDIA"]
.
Bash also allows you to iterate over hashmaps effectively. If you want to loop through all the keys, you can use a for loop with the '@' symbol:
bash
for key in "${!gpu_region_map[@]}"; do
echo "$key"
done
Similarly, to loop through all the values, replace '@' with '*':
bash
for value in "${gpu_region_map[*]}"; do
echo "$value"
done
Should you need to remove the entire hashmap, a simple unset
command will do the job. This level of control over data structures in Bash can be quite powerful, especially when dealing with complex tasks that involve managing a variety of data points. As a best practice, remember to comment your code to clarify the purpose of each variable and operation within your script. This not only aids others in understanding your logic but also serves as a reminder to your future self.
Modifying Object Variable Values
Bash, the ubiquitous shell on Linux systems, offers a plethora of methods for variable management. For instance, when scripting in Bash, it's common to encounter scenarios where you need to increment numerical variables.
You may start by initializing a variable, such as setting count
to 1 (count=1
), but there's no obligation to begin at this number. Following the initialization, you have various options to increment the variable.
You could use simple arithmetic like ((count++))
or let "count+=1"
to achieve this. Moreover, Bash allows for the creation and manipulation of associative arrays, often referred to as hashmaps.
To declare a hashmap, you can use the declare
command (declare -A map
) and then populate it with key-value pairs (map[GPU]='region1'
). Accessing stored values is straightforward, using the familiar syntax (${mymap[GPU]}
).
The versatility of Bash is further demonstrated in its handling of environment variables, which profoundly influence the shell's operation. For instance, modifying the PATH
variable in your .bash_profile
(export PATH=$PATH:/usr/local/bin
) extends the search path for executable binaries.
Variables in Bash are dynamic, allowing you to store and manipulate data, thereby granting your scripts the flexibility to adapt to various inputs and conditions. Additionally, expansions within Bash provide the capability to convert text into actionable data, unlocking the full potential of your scripts. As you navigate through the nuances of .bashrc
, .bash_profile
, and the distinctions between single ('
) and double ("
) quotes, you'll gain a deeper understanding of the shell's mechanics and how they affect subprocesses and the execution environment. These features of Bash are not just theoretical but have practical implications, as seen at the Linux Plumbers Conference and in the continuous evolution of scripting languages like Perl, which now supports Unicode 15.0. Developers must remain vigilant, however, as the discovery of malware in expired S3 buckets highlights the importance of secure coding practices. The bash shell, a critical tool for developers, is a testament to the power and responsibility that comes with scripting in the Linux environment.
Conclusion
In conclusion, understanding object variables in Bash scripting is crucial for efficient data management and manipulation. Object variables act as containers that can hold different types of information, such as strings, integers, arrays, and associative arrays.
Associative arrays, in particular, are useful for storing key-value pairs and can be declared and manipulated using built-in commands like 'declare -A'. Creating and assigning object variables in Bash is straightforward.
Simple variables can be created by using the syntax variable_name='value'
, while hashmaps can be declared with the declare -A
command and populated with key-value pairs. Accessing object variable values is done using the same syntax used for assignment.
For example, to retrieve a value from a hashmap, you would use hashmap_name["key"]
. Additionally, Bash allows for effective iteration over hashmaps using for loops.
Modifying object variable values in Bash offers various options. Numerical variables can be incremented using arithmetic operations like ((count++))
or let "count+=1"
.
Hashmaps can be created and manipulated using the declare
command and accessed with the familiar syntax. The versatility of Bash extends to handling environment variables, which greatly influence the shell's operation. Modifying environment variables like PATH
allows for extending the search path for executable binaries. Overall, Bash provides powerful tools for managing and manipulating data within scripts. Its adaptability and relevance make it an essential tool for efficient software development. By mastering object variables in Bash scripting, developers can enhance their coding process and effectively manage data within their scripts.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.