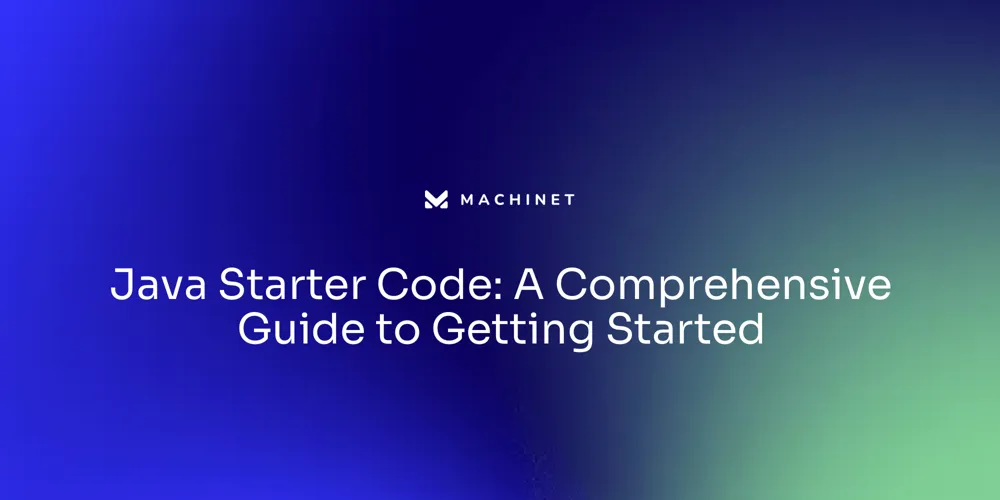
Table of Contents
- Setting Up the Development Environment
- Basic Syntax and Data Types
- Control Structures and Functions
- Object-Oriented Programming in Java
- Common Java Libraries and Frameworks
- Best Practices for Writing Efficient Java Code
- Troubleshooting and Debugging Techniques
Introduction
Embarking on Java development begins with understanding that Java is a high-level, class-based, object-oriented language known for its "Write Once, Run Anywhere" capability. This article will guide you through the process of setting up the development environment, mastering the basic syntax and data types, understanding control structures and functions, exploring object-oriented programming in Java, delving into common Java libraries and frameworks, learning best practices for writing efficient Java code, and acquiring troubleshooting and debugging techniques.
By the end of this article, you'll have a solid foundation in Java development and be equipped with the knowledge and tools to create versatile and secure applications. So let's dive in and unlock the full potential of Java programming.
Setting Up the Development Environment
Embarking on Java development begins with understanding that Java is a high-level, class-based, object-oriented language known for its "Write Once, Run Anywhere" capability. This means Java programs can run across different platforms without recompilation. Beginning with the installation of the Java Development Kit (JDK), which includes essential tools for Java programming, is the first step.
After installing the JDK, select an Integrated Development Environment (IDE) that suits your preferences, such as Eclipse or IntelliJ IDEA, to streamline your coding experience. Configure your IDE to recognize the JDK, setting the stage for a robust development environment. With these tools in place, you're equipped to dive into Java coding, crafting applications that can operate seamlessly across various systems.
Embrace the potential of Java to create versatile and secure applications, from mobile to enterprise solutions, as you join the global community of developers harnessing this powerful platform. To further enhance your development process, consider integrating Machine, an AI-powered plugin that accelerates writing code and unit tests. By simply describing the desired outcome, Machinet's context-aware AI chat can automatically generate code, while its AI unit test agent allows for the creation of unit tests by selecting the method.
This plugin simplifies test regeneration, saving time and effort. Moreover, Machinet's codebase-aware intelligence offers the unique ability to ask questions about general programming topics or your specific codebase directly from your IDE. It understands your local code, learning from the code graph, and utilizes the documentation within your organization to provide precise answers, streamlining your development workflow in Java.
Basic Syntax and Data Types
Diving into the core of Java, we begin with its syntax, heavily influenced by C and C++, to provide a familiar ground for those versed in these languages. Java emphasizes readability and a clean coding style, which is evident in its use of classes, methods, and statementsβkey components that form the skeleton of a Java application. The Java Development Kit (JDK) is indispensable, offering an extensive suite of tools and libraries for Java application construction.
Variables in Java serve as the foundational elements for storing data. They come in various types, each with a specific purpose: integers for whole numbers, floating-point numbers for decimals, characters for single symbols, and booleans for true/false values. Understanding these data types is crucial as they dictate the kind of operations you can perform and the nature of data you can store.
Java's operators are the building blocks that allow you to perform arithmetic, compare values, and execute logical operations. They are the tools that enable you to manipulate data and make decisions within your program. Control flow is the compass that guides the execution of your program.
Through if-else statements, loops, and switch statements, Java offers you the mechanisms to direct how and when certain blocks of code are executed, providing the flexibility to handle complex decision-making processes. By mastering these fundamental concepts, you lay the groundwork for writing robust and efficient Java applications, much like the transition from bicycles to more advanced transportation methods in a growing delivery company. It's about choosing the right tool for the task at hand, and in Java, variables, operators, and control flow structures are exactly thatβtools that, when used wisely, lead to successful and maintainable software development.
Control Structures and Functions
Java, an object-oriented language established by James Gosling in 1990, is structured with classes, methods, and statements that foster a clean coding style. The Java Development Kit (JDK) is essential, providing the necessary tools and libraries for Java application development.
Delving into Java means mastering its control structures, such as conditional statements and loops, which guide the program flow, much like choosing the right vehicle for different delivery distances, a concept seen in delivery business expansions. Java functions, or methods, are the building blocks that break down complex tasks.
They adhere to the Single Responsibility Principle (SRP), ensuring each method addresses a specific task, thereby avoiding complexity and maintaining focus. Meanwhile, exception handling with try-catch blocks in Java is akin to preparing for potential delivery issues, ensuring smooth operation even when unexpected situations arise. Clear commenting practices are also pivotal, providing explanations, documentation, and structure to the code, which, like clear requirements in software development, are the blueprints for successful execution and collaboration. As experts suggest, the realm of software development is limitless, and with well-defined methods and effective communication, developers can navigate the complexities of Java with precision and clarity.
Object-Oriented Programming in Java
Java, as an object-oriented programming language, embodies principles that are foundational to creating robust and maintainable software. The metaphor of a house made from different blocks is apt for understanding Java's OOP; classes serve as blueprints for objects, much like architectural plans for bricks in a building. Each class encapsulates specific attributes and behaviors, analogous to the distinct parts and functions of a human being, as described in object-oriented literature.
To illustrate, consider an object as a noun, such as a car or a book. In Java, an object represents an entity you wish to manipulate data for. For instance, a 'Person' class may contain attributes like name and age, and behaviors like walking or talking.
Inheritance and polymorphism in Java allow for the creation of a hierarchical structure where classes inherit properties and methods from their parent classes, much like a new model of a car inherits the basic design of its predecessor but with enhancements. Encapsulation and abstraction are two fundamental principles that promote a modular approach to programming. Encapsulation shields the internal workings of an object, just as the methods act as building blocks, allowing complex tasks to be broken down into simpler units.
Abstraction, on the other hand, enables developers to focus on essential qualities rather than specific details, streamlining the development process. The Single Responsibility Principle further refines this approach by advocating that each method should perform a single well-defined task, preventing complexity and fostering maintainability. Understanding these principles is not just theoretical but practical, as they guide the creation of software solutions that can be configured by technically skilled users, reflecting the evolving landscape of technology where adaptability and user-centric design are paramount.
Common Java Libraries and Frameworks
Java's extensive ecosystem is a boon for developers, offering a wide range of libraries and frameworks that are pivotal in enhancing development workflows. Delving into these tools not only boosts productivity but also maximizes the potential of the Java language.
- Java Standard Library: At the core of Java's ecosystem, this library hosts essential packages like
java.util
,java.io
, andjava.lang
.java.util
brings together collections and date-time utilities, whilejava.io
is dedicated to input/output operations.
java.lang
provides the fundamental classes necessary for any Java program. - Java Collections Framework: This framework is integral for working with groups of objects, featuring classes and interfaces such as ArrayList
, LinkedList
, HashMap
, and HashSet
.
Efficient data organization and manipulation hinge on these collections. - Spring Framework: Tailored for enterprise applications, Spring offers a robust programming and configuration model that streamlines the development of high-performance, testable, and reusable code.
In the realm of Java development, the Machine plugin emerges as a game-changer. Compatible exclusively with JetBrains, Machine harnesses the power of OpenAI, coupled with in-house validation models trained on high-quality, community-approved source code and natural language. This AI plugin allows developers to swiftly generate code and unit tests. By simply describing the desired functionality, Machinet's context-aware AI chat can automatically write code, while its AI unit test agent can craft unit tests when a method is selected. This innovative tool is designed to make coding and testing in Java faster and simpler, perfectly aligning with the needs of modern tech professionals who are expanding their expertise beyond traditional roles.
Best Practices for Writing Efficient Java Code
Improving Java code efficiency is key to successful software development. To ensure clarity in your code, use clear naming conventions.
For example, the term 'Customer' is far more understandable than 'Ctr', which could be mistaken for other terms. When creating methods, follow the Single Responsibility Principle to make them perform just one function, which makes them easier to manage and understand.
Selecting the appropriate data structures and algorithms optimizes Java performance, enhancing both the neatness of the code and its execution speed. Familiarize yourself with the 'Gang of Four' design patterns, which provide a blueprint for addressing common coding issues, helping you to write code that's both maintainable and scalable.
Logging and effective error handling are essential for developing sturdy Java applications. Use comments to clarify complex parts of your code and to outline the general structure, which will assist others in navigating through your codebase.
Clear and concise requirements are fundamental to crafting software that meets its intended goals. For Java developers looking to further streamline their coding process, the Machine plugin for JetBrains is a valuable tool. It leverages AI, powered by OpenAI and proprietary validation models, to assist in generating high-quality code and unit tests. The AI is trained on well-regarded source code and can generate code snippets or unit tests through a context-aware chat or by selecting a method. This plugin is designed to help developers write code and unit tests more quickly and with greater ease, making it an excellent addition to any Java developer's toolkit.
Troubleshooting and Debugging Techniques
Welcome to the intricate world of debugging, a skill indispensable for programmers. It involves a systematic approach to identifying and rectifying bugs in code. To commence, let's delve into the realm of debugging tools.
Integrated Development Environments (IDEs) offer a plethora of tools, like breakpoints, step-through debugging, and variable inspection, which are instrumental in pinpointing errors. Next, we have logging and error messages. These are not mere notifications; they serve as a compass guiding developers through the maze of code, revealing where things have gone awry.
By harnessing logging effectively, you can trace the execution path and understand the state of your application at the moment of failure. Interpreting stack traces and exceptions is akin to deciphering a map of your code's execution journey. It's essential to comprehend these artifacts to diagnose problems accurately.
Stack traces outline the call sequence that led to an error, while exceptions signal violations that need to be handled gracefully. In the context of software development, risks lurk at every corner, from technical complexities to changing requirements. Software Risk Analysis is a beacon for navigating these uncertainties.
It entails identifying potential risks, assessing their impact, and strategizing for mitigation. By doing so, you not only prevent project derailment but also ensure that your code is robust, maintainable, and scalable. Machinet, available exclusively as a JetBrains plugin, integrates seamlessly into this process, offering an AI-powered advantage.
It employs OpenAI and in-house validation models to generate code and unit tests, streamlining the development workflow. With Machine, developers can automatically create high-quality code and tests by simply describing the desired outcome or selecting a method, thus enhancing code reliability and accelerating the debugging phase. In summary, by mastering debugging techniques and incorporating risk analysis and Machine into your development process, you elevate your coding prowess, leading to high-quality software that aligns with user expectations and business needs.
Conclusion
In conclusion, this article provided a comprehensive guide to Java development. It covered setting up the development environment, mastering syntax and data types, understanding control structures and functions, exploring object-oriented programming, delving into common libraries and frameworks, learning best practices for efficient code, and acquiring troubleshooting and debugging techniques. Java's "Write Once, Run Anywhere" capability makes it a versatile language for creating applications that can run on different platforms without recompilation.
Installing the Java Development Kit (JDK) and selecting an Integrated Development Environment (IDE) are crucial steps in setting up a robust coding environment. The article emphasized the importance of variables, operators, and control flow structures in writing efficient code. It also highlighted the significance of clear commenting practices and effective communication for maintaining code quality.
Object-oriented programming principles such as classes, objects, inheritance, polymorphism, encapsulation, and abstraction were discussed as essential components of modular software solutions. The article also introduced common Java libraries and frameworks that enhance development workflows. Best practices for writing efficient code were shared, including clear naming conventions, selecting appropriate data structures and algorithms, familiarizing oneself with design patterns like the Gang of Four patterns, implementing logging and error handling techniques effectively, and ensuring clear requirements.
Troubleshooting and debugging techniques were discussed as essential skills for programmers. The article introduced debugging tools available in IDEs, emphasized the importance of logging and error messages in identifying errors accurately, discussed interpreting stack traces and exceptions for problem diagnosis, and introduced software risk analysis as a means to mitigate uncertainties. By following this guide to Java development with its practical tips and insights along with utilizing innovative tools like the Machinet plugin for code generation and testing processes developers can acquire a solid foundation in Java programming.
They will be equipped with knowledge about setting up their development environment efficiently while mastering syntax,data types along with control structures ,functions & OOP principles. Additionally they will gain best practices knowledge that help them write efficient code along with troubleshooting techniques along with debugging skills while incorporating risk analysis into their development process. This will enable them to create versatile applications that meet user expectations while ensuring maintainability
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.