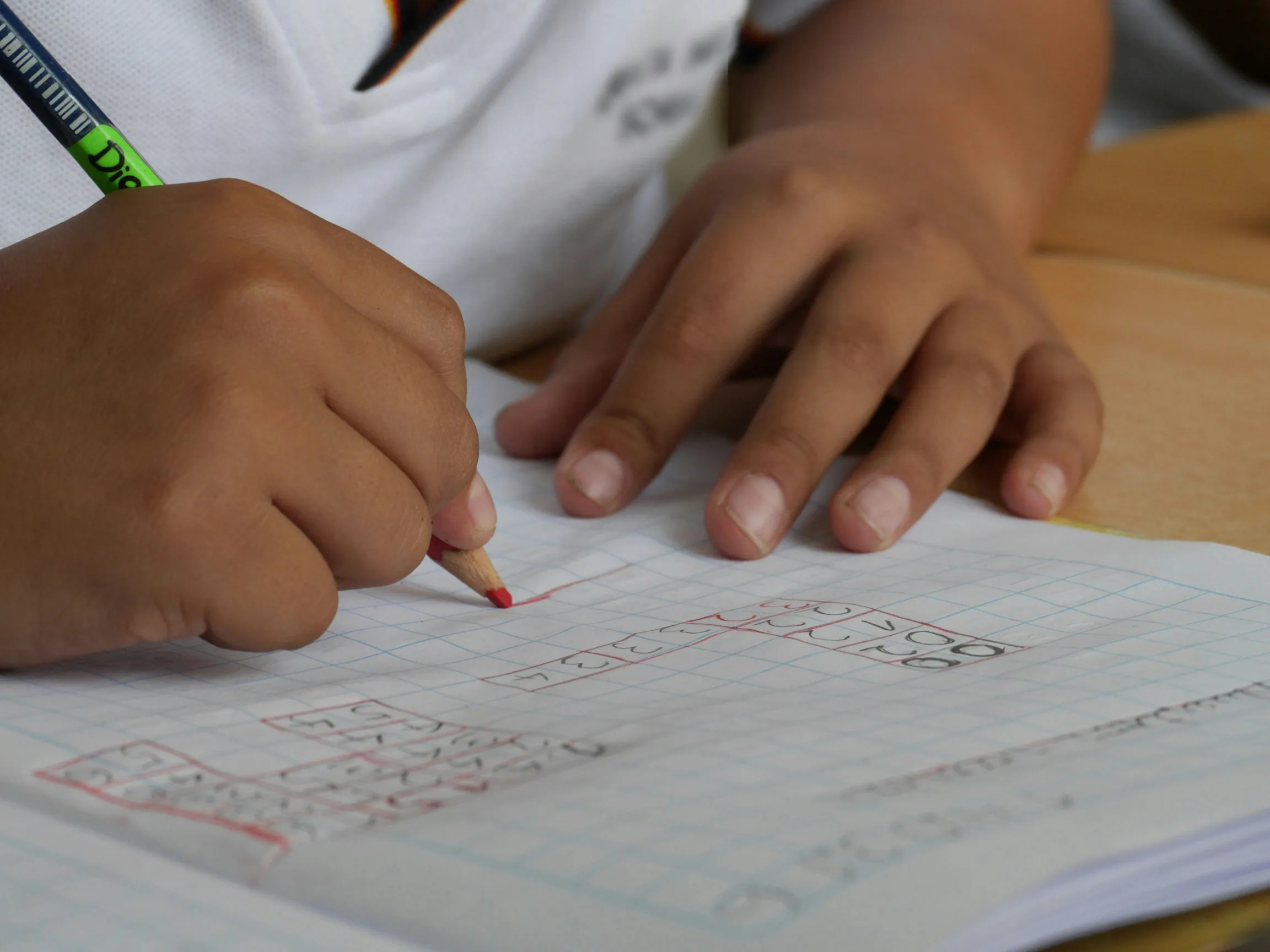
Table of Contents
- Understanding Java Object Types
- Primitive Types
- Reference Types
- Arrays in Java
Introduction
Understanding Java Object Types
In Java, discerning the type of an object is crucial for harnessing the language's object-oriented nature, allowing for sophisticated operations like pattern matching and the use of sealed classes. This article explores how pattern matching and sealed classes enhance Java's type system, promoting code readability and predictability.
It also delves into the principles of object-oriented programming embedded in Java, such as inheritance, encapsulation, and abstraction. Additionally, the article highlights the importance of mutable and immutable objects, design patterns, and the regular updates that keep Java developers equipped with the latest tools for efficient and scalable applications. Stay tuned to unravel the complexities of Java's object types and enhance your programming skills.
Understanding Java Object Types
In Java, discerning the type of an object is crucial for harnessing the language's object-oriented nature, allowing for sophisticated operations like pattern matching and the use of sealed classes. Pattern matching, for instance, elegantly matches a value against a pattern comprising variables and conditions, binding parts of the value to these variables upon a match. This not only yields more intuitive code but also enhances readability.
Sealed classes further enrich Java's type system by controlling which other classes may extend them, thereby introducing more predictability into your code. As Java evolves, new features continue to streamline and secure the development process. For example, JDK 21 introduced virtual threads and a generational Z garbage collector, reflecting Java's commitment to innovation.
Java's object properties, encapsulated within instance variables, define an object's state, while methods encapsulate code blocks to execute tasks, promoting organized, modular design. Object-oriented principles such as inheritance, encapsulation, and abstraction are embedded in Java, fostering modularity and ease of maintenance. Objects in Java can be mutable or immutable, with primitive types being immutable.
This distinction is vital for creating robust applications. By leveraging design patterns, Java developers can apply community-tested solutions to common problems, enhancing code quality. As a dynamic language, Java's regular updates, such as the recent JDK 21 release, ensure developers have access to the latest tools for writing efficient and scalable applications.
Primitive Types
Java's primitive types are the backbone of its type system, representing fundamental data like integers, floating-point numbers, characters, and booleans. Each type comes with a well-defined range and default value, crucial for efficient memory management and performance.
For instance, an 'int' in Java can store values from -2,147,483,648 to 2,147,483,647, while a 'boolean' simply holds 'true' or 'false'. These types are indispensable for developers, whether they're manipulating numbers in calculations or checking conditions in code.
As Java inherits its syntax from C and C++, those with experience in these languages will find Java's primitive types familiar and the transition smoother. This consistency is part of Java's design to be readable and writable, fostering a coding style that is both clean and organized.
Moreover, the Java Development Kit (JDK), essential for Java development, provides a comprehensive suite of tools that assist in writing, compiling, and debugging code that deals with these primitive types. Understanding the properties and behaviors of these types is pivotal in object-oriented programming (OOP), which Java robustly supports. In OOP, objects—instances of classes—encapsulate data, and Java's primitive types often define the state of these objects through their instance variables. This encapsulation is part of the larger OOP goal to bind data and functions together, promoting code reuse and modular design. Methods in Java, which are blocks of code that execute specific tasks, are often designed to operate on these primitive types, enhancing the language's capability to model real-world scenarios where data types and their operations are closely knit.
Reference Types
In Java, reference types go beyond the simplicity of primitives, encapsulating complex objects crafted from classes and interfaces. These types empower developers to forge custom data structures and tailor behavior specific to their application's needs.
Delving into pattern matching, a feature introduced in recent Java releases, we see how it streamlines operations on these data structures, offering a more readable approach to extracting and manipulating data. For instance, pattern matching allows for binding parts of a value to variables when a match is found, simplifying code and enhancing its intuitiveness.
The introduction of sealed classes further refines the control over inheritance, ensuring that only the specified classes can extend from a sealed class, thus providing a more robust and maintainable codebase. The nuances of Java's object references are intriguing; when an object reference is passed to a method, it's the memory address that is copied, not the object itself, which allows for the modification of object properties within the method.
However, the reference itself remains immutable, a concept vital for clear communication and understanding in Java programming. Moreover, the Foreign Function & Memory API brings advancements with the notion of restricted methods, signifying a cautious approach towards binding native functions and data, hinting at the evolving nature of Java's interaction with native resources. When creating new objects, the ubiquitous pattern of new/dup/invokespecial
Arrays in Java
In Java, arrays are versatile structures that enable developers to store multiple values in a contiguous block of memory. These structures are particularly efficient for operations like searching, sorting, and filtering data.
Arrays in Java are objects, and as a result, they are allocated on the heap. This allocation is dynamic, occurring at runtime, which provides flexibility in memory usage.
The elements within an array are stored sequentially, with each element's index beginning at zero. Given that arrays are static in size, their length is fixed upon creation, which means that developers must be mindful of the array's capacity when storing elements.
One-dimensional arrays, which consist of a single row or column, are a fundamental aspect of Java programming. They are suitable for storing and accessing lists of values and are widely employed across various programming languages.
However, arrays come with limitations, such as the inability to change their size once established. To add more elements than the array can accommodate, a new, larger array must be created, and the elements must be copied over, which can be inefficient. Despite these constraints, arrays offer direct access to their elements due to the contiguous nature of their storage in memory. They can hold various data types, from primitives like integers, which occupy 4 bytes each, to strings and objects. This inherent capability makes arrays a critical tool in a Java developer's arsenal, especially when considering the language's built-in support for arrays, eliminating the need for external dependencies.
Conclusion
In conclusion, understanding Java's object types is crucial for harnessing the language's object-oriented nature. Pattern matching and sealed classes enhance Java's type system, promoting code readability and predictability.
Pattern matching allows for elegant value matching against patterns, while sealed classes control which classes can extend them, introducing more predictability into the code. Java's object-oriented principles, such as inheritance, encapsulation, and abstraction, are embedded in the language, fostering modularity and ease of maintenance.
The distinction between mutable and immutable objects is vital for creating robust applications. By leveraging design patterns and regular updates like JDK 21, Java developers have access to community-tested solutions and the latest tools for efficient and scalable applications.
Java's primitive types are fundamental to its type system and offer well-defined ranges and default values. They are indispensable for efficient memory management and performance.
Understanding their properties and behaviors is pivotal in object-oriented programming (OOP), which Java robustly supports. Reference types in Java go beyond primitives and allow for complex objects crafted from classes and interfaces.
Pattern matching simplifies operations on these data structures, enhancing readability. Sealed classes provide control over inheritance, ensuring a more robust codebase. Arrays in Java are versatile structures that enable storing multiple values in a contiguous block of memory. They are efficient for operations like searching, sorting, and filtering data. While arrays have limitations regarding size change, they offer direct access to elements. Overall, mastering Java's object types empowers developers to create organized, modular designs while leveraging the language's features for efficient and scalable applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.