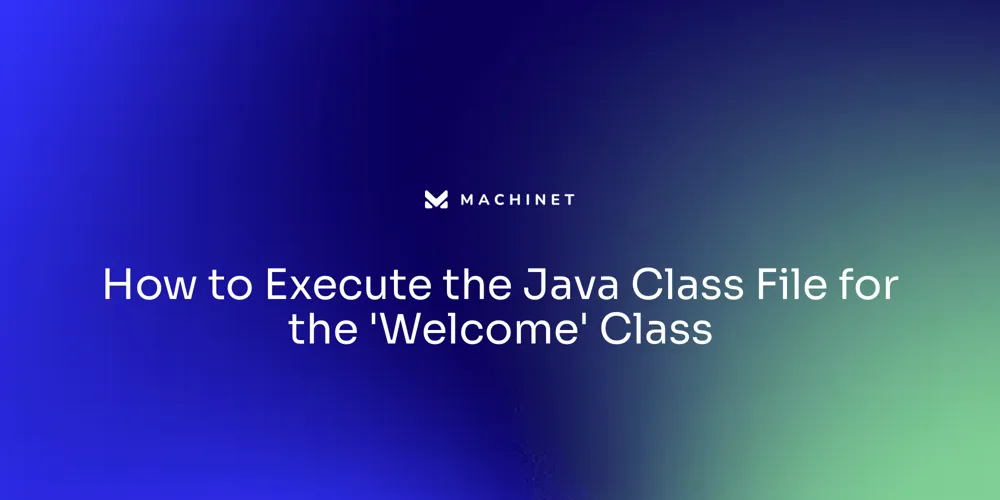
Introduction
Java is a powerful and widely-used programming language known for its performance, stability, and community support. In this article, we will explore the process of compiling and executing Java code, as well as understanding command line syntax and handling common errors. By gaining a deep understanding of these topics, developers can enhance their ability to create robust and efficient Java applications, while also leveraging the benefits of Java frameworks.
So, let's dive in and uncover the mechanics behind Java compilation, execution, and troubleshooting techniques.
Step 1: Compiling the Java Source File
Beginning with the fundamentals, in order to execute a class, it is necessary to initially convert the high-level source into a format that is compatible with machines. This is achieved through a process known as compilation, and it's facilitated by a tool called the Compiler (javac). During compilation, the source undergoes lexical analysis where it is broken down into tokens, representing the smallest units of meaning in the program, much like vocabulary words in a language.
To compile a Java source file, for instance, 'Welcome.java', the command is straightforward:
bash
javac Welcome.java
Executing this command prompts the Java Compiler to process the source code, handling any exceptions and converting it into bytecode, which is stored in a '.class' file bearing the same name as the source file. Bytecode serves as the intermediary representation of your program, a lower-level language that brings you one step closer to execution on the Java Virtual Machine (JVM).
The compilation of programming code is a crucial step in the lifecycle of an application's development, ensuring that developers can debug effectively with the help of tools like source maps, which map the compiled code back to the original source code, offering clarity and insight into the execution flow.
This process not only streamlines the debugging process but also plays a foundational role in the performance and stability of programming applications. As the programming language continues to evolve, enhancements in its toolchain aim to simplify the developer experience, such as the introduction of 'var' to infer types within methods, and improvements to the build process, allowing for a more gradual transition from small to larger projects without the immediate need for complex configuration.
The consistent updates to the programming language, including the release of new versions every six months and the regular introduction of long-term support (LTS) versions, underscore the language's commitment to stability, security, and performance. These updates are embraced by developers, as seen in the widespread adoption of new programming language versions in production environments.
By understanding the mechanics of compilation in the Java programming language and the available tools, developers can ensure robust application performance and a smoother development process, reflecting the dynamic and supportive nature of the Java community.
Step 2: Executing the Java Class File
After compiling a source code written in Java, the next step is to run the generated executable. To accomplish this, the 'java' command is utilized in combination with the name of the category without the '.class' extension. For instance, if you have a class file named 'Main.class', you would run the following command in your terminal:
bash
java Main
Executing this command initiates the Java Virtual Machine (JVM), which loads the class, verifies the bytecode, and executes the main method. This process outputs the result to the console, allowing you to see the program in action. It's crucial to keep in mind that the programming language's class name is case-sensitive, so the name specified in the command must match the class name precisely.
The execution of programming language programs is a pivotal moment where code springs to life, and understanding how the JVM operates under the hood can enhance your ability to troubleshoot and optimize your applications.
Understanding the Command Line Syntax
Becoming proficient in passing command line arguments can greatly enhance the interactivity and configurability of your applications. When you execute a class in Java, like the quintessential Hello World
, you can include command line arguments right after the class name. These arguments are then retrieved within the program through the args
array parameter of the main
method, a pivotal method that represents the starting point of execution.
For instance, to greet a user by name, your command might look like this:
bash
java HelloWorld John
Within the main
method, the argument can be accessed as:
java
public static void main(String[] args) {
String name = args[0];
// Additional code
}
This simple yet powerful technique can be a stepping stone to creating more dynamic Java applications. It's a well-tested practice, integrated in applications like XPipe, where configuration data or user preferences are often stored and managed through command line inputs. The benefits of using command line arguments are also evident in the context of desktop development, a domain that presents its own set of challenges, such as handling file system access restrictions imposed by antivirus software.
The joy and sense of accomplishment that come with successfully executing your first piece of code, such as HelloWorld
, is unforgettable. It's a fundamental experience shared by many developers, including those involved in the global programming language in Education initiative led by programming language User Groups (Lugs). This initiative underscores the importance of hands-on learning and the use of programming language in the educational journey of junior developers and students.
As we delve into the intricacies of programming in Java, it's important to remember the core strengths of Native Java in terms of performance and control, as well as the productivity gains brought by various programming frameworks. Our guide aims to equip you with a deep understanding of these topics, supported by real-world examples, best practices, and insights into the trade-offs of choosing between native programming language and its frameworks for your projects.
Through the lens of seasoned developers and educational efforts, we can appreciate the significance of mastering such command line techniques. They not only ensure ease of use but also contribute to the robustness and efficiency of software development, as echoed by the experiences and preferences of a diverse range of developers who specialize in the programming language of Java.
Common Errors and Solutions
During the execution of files written in the programming language, developers frequently come across various errors that can impede the process of execution. One specific mistake is the 'ClassNotFoundError', which usually happens when the runtime environment for Java fails to locate the desired program. To resolve this, ensure that the file is situated in the directory where the command is being run, or specify the file's path using the '-cp' option to set the classpath.
Another common stumbling block is the 'NoSuchMethodError'. When the runtime environment for the programming language can't find a specific method within the class, this error is thrown. It's essential to verify the accuracy of the method name and the parameters provided, as a discrepancy can lead to this error.
Lastly, the 'NullPointerException' is a frequent and tricky error that arises when trying to operate on a null reference. This metaphorically resembles attempting to utilize a blank sticky note instead of a meaningful reference. To steer clear of this issue, ensure all variables have been initialized and hold a valid object reference before being used.
Understanding and rectifying these errors is vital for smooth Java application execution. By keeping the nuances of test cases, code smells, and best practices in mind, programmers can mitigate these common errors and maintain a cleaner, more efficient codebase.
Conclusion
In conclusion, understanding Java compilation and execution is crucial for developers. Compiling source code into bytecode improves debugging and performance. Java's updates and long-term support versions highlight its commitment to stability, security, and performance.
Executing class files with the JVM enhances troubleshooting and optimization. Mastering command line syntax allows for interactive and configurable applications.
Awareness of common errors like "ClassNotFoundError," "NoSuchMethodError," and "NullPointerException" is vital. Resolving these errors involves proper file location, accurate method names, and variable initialization.
By understanding these topics and leveraging the Java community, developers can create robust applications. Java's performance, stability, and community support make it a powerful language.
Join the Java community and start creating robust applications today!
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.