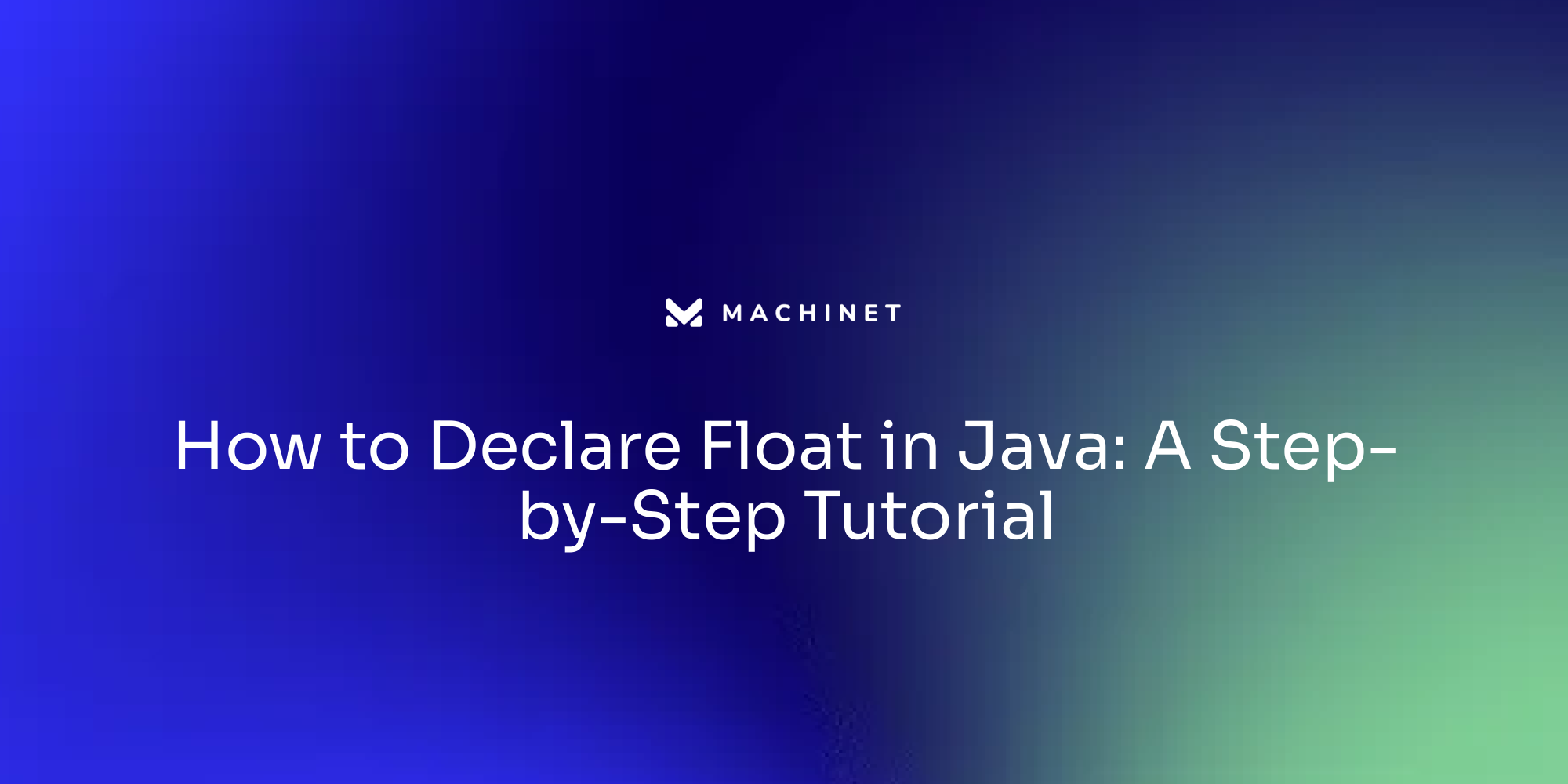
Introduction
Understanding the use of the float
data type in Java is crucial for developers working on applications that require precise decimal calculations. As a single-precision 32-bit IEEE 754 floating point, float
allows for the storage of fractional values, which is essential for scientific computations and financial applications. This article delves into the fundamentals of float
in Java, including how to declare and initialize float variables, the importance of the 'f' suffix, and common issues related to precision loss and rounding errors.
Additionally, the discussion extends to comparing float
with double
, and best practices for utilizing float
in various programming scenarios. Through practical examples and detailed explanations, this guide aims to equip developers with a comprehensive understanding of managing decimal numbers in Java effectively.
What is Float in Java?
In Java, the single-precision
data type is utilized to represent decimal numbers with 32-bit IEEE 754 floating point. This allows for the storage of fractional values, which is crucial for scientific computations and financial applications. For instance, in a case study on the SWIFT method for option pricing, the precision provided by a specific data type enables accurate calculations of complex financial derivatives, underscoring its importance in real-world financial modeling. The application of a floating-point data type in such scenarios ensures that developers can handle the nuanced demands of applications requiring detailed numerical analysis.
Declaring Float Variables in Java
To declare a decimal variable in Java, use the decimal
keyword followed by a meaningful variable name. For instance: java double productPrice;
This statement initializes a variable named product Price
that can hold a decimal value, which is a good practice as it clearly indicates the variable's purpose.
Initializing Float Variables with Decimal Values
To initialize a float variable during declaration, assign it a decimal value with the f
suffix. For example:java float myNumber = 5.75f;
The f
suffix is crucial because, by default, Java treats decimal numbers as doubles. This distinction connects to the IEEE 754 guideline, established by the Institute of Electrical and Electronics Engineers in 1985, which defines the representation of floating-point numbers. The standard ensures consistency and reliability across different computing systems.
The Importance of the 'f' Suffix
The 'f' suffix is essential when dealing with decimal literals in Java. Without it, such as in float Number = 5.75;
, the compiler will either throw an error or convert the value to a double. This conversion corresponds with the 754 specification, which regulates floating-point calculations. Established in 1985 by the Institute of Electrical and Electronics Engineers (IEEE), this standard ensures consistency in floating-point computations across different systems. Nevertheless, utilizing doubles instead of single-precision numbers can result in accuracy problems because of how decimal fractions are represented in binary format. To avoid these pitfalls and ensure accurate representation, always append 'f' to your decimal literals.
Examples of Declaring and Initializing Float Variables
Here are some examples of declaring and initializing float variables in Java:
java
float a = 4.5f;
float b = 3.14f;
float c = 0.001f;
These float variables, defined using the IEEE 754 standard, can be utilized in various mathematical operations and function calls, ensuring reliable and consistent calculations.
Common Issues with Java Float (Precision Loss and Rounding Errors)
The IEEE 754 guideline, established in 1985 by the Institute of Electrical and Electronics Engineers (IEEE), is crucial for representing floating-point numbers in computers. This guideline addresses issues found in various floating-point implementations, making computations more reliable and portable. However, using a single-precision type can lead to precision loss due to its limited accuracy compared to double-precision. For example, when performing arithmetic operations, you might encounter rounding errors. It's essential to be aware of these limitations, especially when accuracy is critical, such as in financial calculations. The 754 specification ensures that computers reliably produce the same outcomes for identical calculations, but grasping the inherent limitations of float
is crucial for creating precise software solutions.
Using Float for Mathematical Operations
Float variables are integral in performing various mathematical operations such as addition, subtraction, multiplication, and division. These operations are regulated by the 754 specification, which guarantees reliability and portability in floating-point calculations. For instance, consider the following code snippet:
java
float result = a + b;
This example calculates the sum of a
and b
, storing the result in the result
variable. 'The 754 specification separates floating-point values into three components: the sign bit, the exponent, and the fraction (mantissa).'. These components enable a wide range of numbers, though at the cost of precision. Understanding how decimal fractions like 0.1 and 0.2 are stored as binary numbers is crucial to grasping floating-point arithmetic's nuances. 'Founded in 1985 by the Institute of Electrical and Electronics Engineers, 754 addresses inconsistencies across floating-point implementations, ensuring that identical computations yield the same results consistently.'.
Float vs. Double: Understanding the Differences
Float and double are both used to represent decimal numbers, but they differ significantly in terms of precision and memory usage. Float is a single-precision 32-bit floating point, while double is a double-precision 64-bit floating point. This means that double can represent numbers with greater accuracy, which is crucial for many scientific and financial applications. The IEEE 754 standard, established in 1985, governs the representation of these floating-point numbers, ensuring that computations are consistent and reliable across different systems. For example, single precision can manage up to 7 decimal digits, whereas double precision can accommodate up to 15, making double precision more appropriate for complex calculations. However, the use of decimal numbers is often preferred in situations where memory is limited, such as in embedded systems or graphics processing. Understanding these differences is essential for making informed decisions about which data type to use in various programming scenarios.
Best Practices for Using Float in Java
When using float in Java, follow these best practices:
- Use
float
when memory usage is a priority or when interfacing with systems that require single precision. The IEEE 754 standard, established by the Institute of Electrical and Electronics Engineers in 1985, ensures consistent calculations across different systems but comes with a trade-off in precision. - Always append the 'f' suffix to decimal literals to avoid unintentional conversions. For example,
float value = 3.14f;
This practice prevents the code from inadvertently treating the literal as a double, which could lead to unexpected behavior. - Be aware of precision loss and rounding errors. A common issue with floating-point arithmetic is that decimal fractions like 0.1 and 0.2 cannot be represented exactly in binary form. Consequently, consider using
double
for calculations requiring higher precision. This approach is particularly important in applications like financial trading platforms, where precise calculations are crucial to avoid significant errors in transactions.
Conclusion
The exploration of the float
data type in Java highlights its essential role in handling decimal numbers within various applications, particularly in scientific and financial computations. By adhering to the IEEE 754 standard, float
provides a reliable means of storing fractional values, though it is important to recognize its limitations regarding precision. The significance of the 'f' suffix when declaring float variables further emphasizes the need for developers to ensure accurate data representation.
Declaring and initializing float variables in Java is straightforward, but it requires diligence to avoid common pitfalls such as precision loss and rounding errors. Understanding the differences between float
and double
is crucial for developers aiming to make informed decisions based on the specific requirements of their applications. While float
is beneficial for memory efficiency, especially in embedded systems, double
offers greater precision for more complex calculations.
In summary, effective use of the float
data type involves following best practices, including proper declaration, initialization, and awareness of its limitations. By leveraging the advantages of float
while also recognizing when to opt for double
, developers can enhance the reliability and accuracy of their applications, ultimately leading to better software solutions.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.