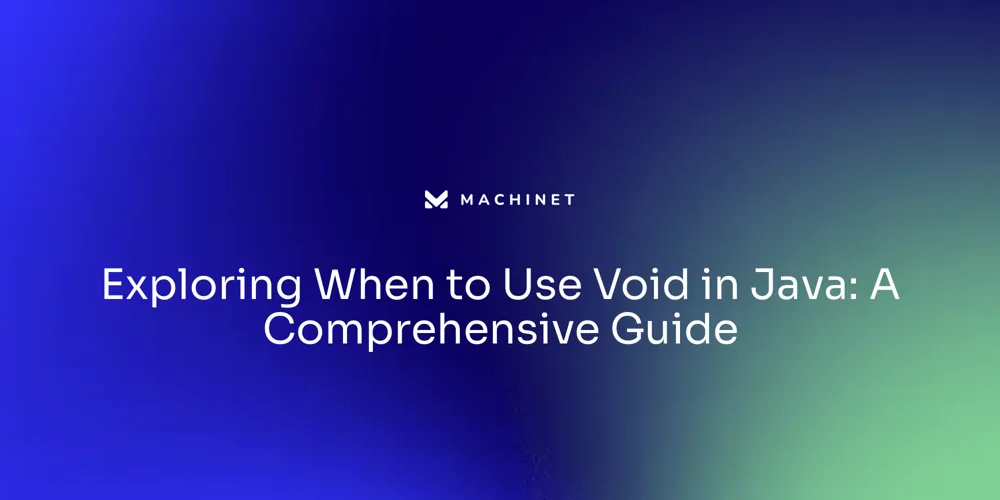
Table of Contents
- Understanding Void Methods
- When to Use Void Methods
- When to Use Return Methods
- Performance Considerations
- Best Practices for Using Void Methods
- Common Use Cases for Void Methods
Introduction
Void methods in Java play a crucial role in code organization and simplification. These methods encapsulate a set of related instructions without the need to return a value, making code more modular and easier to read.
By adhering to best practices such as the Single Responsibility Principle, developers can create focused and maintainable segments of code. In this article, we will explore the importance of void methods, when to use them, considerations for performance, and best practices for their implementation in Java development.
Understanding Void Methods
Void methods in Java serve a key role by encapsulating a set of related instructions into a cohesive unit without the need to return any value. These methods, acting as building blocks within your code, allow for the simplification of complex tasks into smaller, more digestible segments.
Adherence to the Single Responsibility Principle (SRP), a core component of software design, is crucial; it requires a method to embody a singular, clear-cut function. By ensuring your void methods are aligned with SRP, you create focused segments of code that avoid unnecessary intricacies.
Moreover, embracing clean Java code principles, such as using straightforward and descriptive names for methods, substantially aids in rendering code that is not only operationally sound but effortless to read, comprehend, and adjust. This contributes to a healthier, sustainable codebase that stands the test of time, reflective of Eleftheria's insights on relaying intricate technical concepts in an approachable manner. In the broader spectrum, while other technology stacks like JavaScript, SQL, Python, and HTML/CSS are frequently utilized, the disciplined application of Java best practices is a testament to the language's enduring relevance and the commitment of its developers to maintainable and quality code.
When to Use Void Methods
In the dynamic landscape of Java software development, certain programming practices stand out for their efficiency and robustness. Among these are the use of void methods in Java code, which are instrumental in various scenarios without the need for returning any values.
Void methods shine in event handling within GUI applications. Take, for example, a void method that springs into action when a user clicks a button, unleashing a predetermined sequence of operations.
Similarly, interfacing with external devices is streamlined through void methods, which facilitate actions like sending a print job to a printer or issuing commands to external hardware. Furthermore, void methods are the backbone of callback functions.
These special methods are triggered in response to certain events, exemplifying the asynchronous nature of today's software interactions, as they allow other tasks to proceed without interruption. In a world where writing clean code equates to a commitment to software craftsmanship, adherence to principles such as the Single Responsibility Principle (SRP) is non-negotiable.
By assigning a single, focused task to each method, developers can avoid complexities and enhance the clarity and maintainability of their code. This ideal is echoed in joint research and product development.
According to a renowned tech mindset, the journey from an exploratory 'tech debt' phase towards a robust product requires optimized software practices unique to each stage. This bifurcation manifests in specialized research and applications divisions within companies. A recent survey underscores the predominance of Java and its development ecosystem. IntelliJ IDEA by JetBrains stands out as a leading Java and Kotlin IDE amidst the fast-evolving technology landscape. The Java developer community, brimming with youthful energy, remains large and active, with 66% employed full-time and a significant 23% comprising students and working students. Therefore, mastering Java's best practices, like the effective use of void methods, is more pertinent than ever for those starting their careers in this vibrant field.
When to Use Return Methods
In Java programming, the significance of return methods goes beyond merely sending back a value or object from a function. These methods embody the principles of clean code; they serve as building blocks that distill complex operations into concise units of logic that are easy to read, understand, and maintain.
Such methods adhere to the Single Responsibility Principle (SRP), which advocates for methods to have only one reason to change, ensuring their coherence and simplifying debugging. Adopting a meticulous approach to method creation contributes to the overall health and sustainability of software.
This is evidenced by practices like using descriptive names instead of obscure acronyms—for example, preferring to use 'Customer' over the ambiguous 'Ctr'. According to a report involving programming practices, technologies like Java face the challenge of staying relevant amidst constantly evolving languages. Nevertheless, Java continues to be a foundation for many developers, with languages such as JavaScript, SQL, Python, and HTML/CSS often used in conjunction with Java. This interoperability, combined with a dedication to principles of clean code, empowers programmers to write Java methods that are not just functional, but exemplary in their clarity and elegance.
Performance Considerations
In Java development, the debate between using void methods versus those with return values is a subtle yet important consideration. Void methods don't pass back data, which can slightly streamline performance as they eschew the need for creating a new object or managing a return value.
Despite this, the real-world impact on performance is often minuscule, especially given modern Java Virtual Machine (JVM) optimizations. Additionally, the decision should align with clean code principals, prioritizing readability, maintainability, and adherence to the Single Responsibility Principle (SRP).
SRP advocates for methods to perform a single task or action, enhancing focus and minimizing complexity. As the adage goes, methods should act as building blocks, breaking down intricate operations into simpler, more comprehensible segments. When coding in Java, it's not just about efficiency but also about the clarity and quality of the code, ensuring it's straightforward to manage and evolve over time. Therefore, while performance considerations have their place, the ultimate choice between void and return methods should also contemplate the principles of clean code practice.
Best Practices for Using Void Methods
When crafting Java methods, it's essential to hinge on the Single Responsibility Principle, ensuring each void method is driven by one sole task. This adherence translates to clearer, more maintainable code.
The naming of methods demands equal attention—opt for lucidity and expressiveness that directly relate to the method's function, thereby simplifying the comprehension and collaboration process among developers. It's also critical to minimize side effects, a rule that underscores the advocacy for simplicity in code construction.
Should side effects become complex, breaking the method into smaller, more defined segments is beneficial. This approach not only aligns with clean code philosophies but also mirrors the elegance of simplicity as detailed by Robert C. Martin in 'Clean Code: A Handbook of Agile Software Craftsmanship.' By applying these guidelines, your methods contribute to a codebase that is not just functional, but also a testament to thoughtful software craftsmanship.
Common Use Cases for Void Methods
In the domain of Java development, void methods serve as essential building blocks, streamlining complex functionalities into neat, efficient packages. Handling user interface events is one of their primary roles.
Consider a button within a graphical user interface; when clicked, it activates a void method meticulously crafted to execute a predetermined action, in line with the Single Responsibility Principle (SRP). This principle dictates that a method should be narrowly focused on a single task, avoiding the pitfalls of complexity and enhancing readability and maintenance.
Similarly, void methods prove indispensable in validating user input. They diligently check whether the provided data adheres to the specified criteria and promptly communicate any discrepancies through clear error messages.
Furthermore, void methods play a crucial role in the background operations of logging and error management—quietly documenting the system's activities and elegantly handling any unforeseen errors to maintain the software's overall robustness. Lastly, when an operation requires execution but not a response, void methods step in to bridge the gap. They efficiently carry out tasks like updating databases or interfacing with external devices, leaving no need for a return value. This demonstrates the power of methods in Java, underpinning the clean code philosophy where each line serves its purpose with precision, ensuring the code is not just operative, but also a paragon of software craftsmanship.
Conclusion
Void methods in Java are crucial for code organization and simplification. They encapsulate related instructions, making code more modular and readable.
Adhering to the Single Responsibility Principle ensures that void methods have a clear and focused function, avoiding unnecessary complexities. Void methods excel in event handling for GUI applications, interfacing with external devices, and as callback functions.
They allow for seamless task execution and asynchronous interactions. Prioritizing readability, maintainability, and adherence to the Single Responsibility Principle is crucial when choosing between void and return methods.
Performance considerations between void and return methods are often minimal due to JVM optimizations. The key is to prioritize clean and maintainable code.
Following best practices such as clear naming conventions, minimizing side effects, and embracing simplicity contribute to code quality. In conclusion, void methods in Java play a vital role in code organization and readability. They are versatile in handling events, interfacing with external devices, and facilitating asynchronous interactions. Prioritizing clean code practices and the Single Responsibility Principle is crucial for creating maintainable code. By leveraging the power of void methods and considering performance, developers can write code that is not only functional but also exemplary in its simplicity and elegance.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.