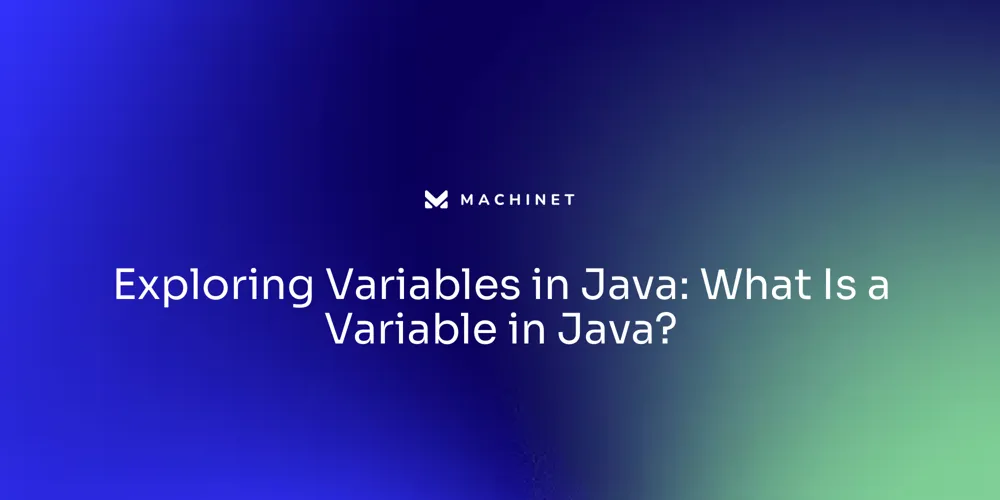
Table of Contents
- Definition of a Variable in Java
- Declaring Variables in Java
- Initializing Variables in Java
- Variable Types in Java
Introduction
In the realm of Java programming, variables are the cornerstone of data manipulation and storage. They are essentially named locations in memory where values can be stored for later retrieval and use throughout a program's lifecycle.
Each variable in Java is associated with a specific type, such as int for integers, double for decimal numbers, or String for text, which dictates the nature of data it can contain. Moreover, Java's object-oriented nature means that variables can also refer to objects, encapsulating both data and behaviors.
But what exactly is the process of declaring and initializing variables in Java? And how do different variable types in Java affect the way values are passed to methods?
In this article, we will explore these fundamental concepts of variable handling in Java. We will delve into the steps of declaring and initializing variables, discuss the various variable types in Java, and examine how they impact method parameter passing. By understanding these core concepts, you'll be equipped with the knowledge to effectively work with variables in your Java programs. So let's dive in and explore the world of Java variables!
Definition of a Variable in Java
In the realm of Java programming, variables are the cornerstone of data manipulation and storage. They are essentially named locations in memory where values can be stored for later retrieval and use throughout a program's lifecycle.
Each variable in Java is associated with a specific type, such as int for integers, double for decimal numbers, or String for text, which dictates the nature of data it can contain. Moreover, Java's object-oriented nature means that variables can also refer to objects, encapsulating both data and behaviors.
For instance, when dealing with environmental variables, developers can set these up through the operating system or scripts, providing flexibility in configuring Java applications before launch. The ability to toggle features at runtime through feature toggles is another powerful aspect of Java.
This empowers developers to seamlessly test new functionalities with select user groups without the need for multiple application deployments. Furthermore, Java's method of parameter passing is often misunderstood.
It operates on a "pass-by-value" basis, meaning it passes a copy of the value for primitive types or the value of the reference for objects, not the object itself. This distinction is crucial when modifying an object within a method, as the original reference remains unaffected. These concepts are not just academic; they are reflected in the continuous evolution of the Java Development Kit (JDK). For example, JDK 23 introduces new features enhancing vector computations and pattern matching, underscoring Java's commitment to developer productivity and performance optimization. The structured nature of Java, with its classes and methods, underpins the language's readability and maintainability, making it a steadfast choice for developers globally.
Declaring Variables in Java
In Java, declaring a variable is a fundamental step that sets aside memory for storing values. For instance, to declare an integer variable named total Apples
, one would use the syntax int total Apples;
. This tells the Java compiler that total Apples
will hold integer data.
Interestingly, Java's evolution has introduced features like pattern matching, which simplifies operations on data, such as extracting from data structures. This makes code more intuitive and readable. Java 20 and 21 have brought significant enhancements, including virtual threads and record patterns.
Virtual threads simplify concurrent application development, while record patterns in switch blocks, introduced in Java 21, mark a significant step towards functional programming patterns in Java. These advancements indicate Java's rapid evolution since Java 9, with releases now every six months. Notably, variable naming conventions in Java play a crucial role.
They should be clear and meaningful, avoiding abbreviations and generic names. For example, using total Price
instead of temp
makes the code more understandable and maintainable. As Java grows, such practices become even more vital for writing clean, maintainable code that shines in the collaborative and fast-paced world of software development.
Initializing Variables in Java
In Java programming, initializing a variable is a fundamental step that assigns an initial value to the variable after its declaration. This crucial action ensures that the variable holds a defined, valid value before it's employed in the code. For instance, to declare and initialize an integer variable named age
with a value of 25, the appropriate syntax would be:
java
int age = 25;
The concept of initialization aligns with Java's strong type system and its emphasis on ensuring type safety and reducing errors.
By initializing variables, Java developers prevent the occurrence of undefined states within their programs, which is especially important when dealing with complex data structures, such as those involving generics. Generics in Java bolster type safety by enforcing strict type checks at compile time, thus preventing runtime errors and class cast exceptions. The versatility of Java is further exemplified by its ability to adapt to new features and improvements.
For example, the recent Java 20 release introduces enhancements like virtual threads, which simplify concurrent programming, and various other updates that continue to evolve Java's capabilities. These advancements, along with Java's inherent flexibility and control, empower developers to write robust, maintainable, and efficient applications. Understanding and applying proper variable initialization is a stepping stone towards leveraging Java's full potential, enabling developers to create high-quality software that stands the test of time.
Variable Types in Java
Java's robust type system includes both primitive types like int, double, boolean, and char, as well as reference types such as String, arrays, and user-defined classes. The distinction between these variable types is foundational for Java programmers, as it influences how values are passed to methods.
When a primitive type is passed to a method, Java creates a copy of the actual value, which means the original value remains intact outside the method. In contrast, passing a reference type involves copying the reference's memory address, allowing methods to alter the object's attributes while the reference itself remains the same.
This concept is at the heart of Java's 'pass-by-value' mechanism, a critical understanding for any developer working with the language. Moreover, the static typing system in Java mandates that variable types be declared at compile time, which cannot be altered during runtime.
This approach, while seemingly inflexible, provides performance benefits and aids in the early detection of errors. It also complements Java's strong emphasis on Object-Oriented Programming (OOP), which is integral for constructing complex, large-scale enterprise applications. Recent updates in Java, like the introduction of the Vector API in JDK 23 and enhancements to virtual threads in Java 20, continue to evolve the language's capabilities. These features, including pattern matching for switch statements and expressions, are shaping the future of Java, enabling developers to write more efficient and scalable code. As the language progresses, understanding these core concepts and staying updated with the latest features is indispensable for developers to leverage Java's full potential.
Conclusion
Variables are fundamental to Java programming, providing named memory locations for storing and accessing values. Each variable is associated with a specific type, dictating the kind of data it can hold.
Java's object-oriented nature allows variables to refer to objects, encapsulating both data and behaviors. Declaring variables involves setting aside memory, while proper naming conventions enhance code readability.
Java's evolution introduces features like pattern matching and record patterns, improving code intuitiveness. Initializing variables assigns an initial value after declaration, ensuring defined and valid values.
This aligns with Java's strong type system, promoting type safety and reducing errors. Java's robust type system includes primitive types like int and reference types like String.
Understanding the distinction is crucial for method parameter passing. Java operates on a "pass-by-value" basis, creating copies for primitives and copying memory addresses for references. The static typing system in Java requires declaring variable types at compile time, providing performance benefits and error detection. Recent updates enhance capabilities, such as the Vector API in JDK 23 and improvements to virtual threads. In conclusion, understanding variable handling in Java is essential for effective programming. By mastering declaring and initializing variables, comprehending different types and their impact on method parameter passing, developers can write clean code that harnesses the power of Java for building robust applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.