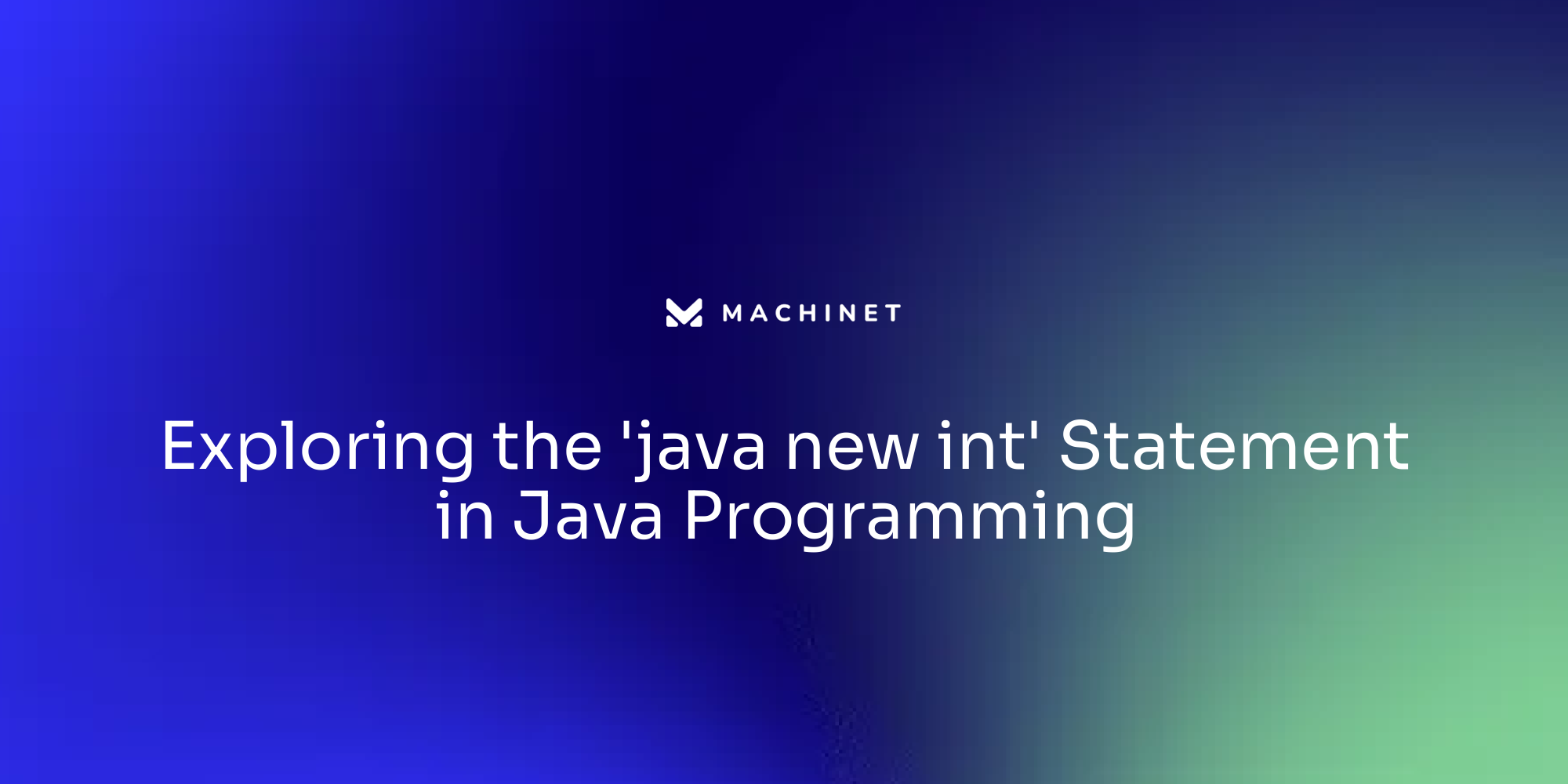
Introduction
Java, a widely-used programming language, provides a robust framework for data manipulation through its primitive types and array structures. This article delves into the fundamental aspects of Java's primitive types, such as byte, short, int, long, float, double, char, and boolean, which are pivotal for efficient memory management and performance optimization. It further explores the importance of declaring variables correctly, the role of the new
keyword in memory allocation, and the practical use of arrays in Java.
Additionally, the article addresses common mistakes and best practices, offering insights into efficient coding techniques and the effective use of data structures. Understanding these core concepts is essential for any Java developer aiming to write optimized and error-free code.
Understanding Primitive Types in Java
Java provides a set of primitive types that serve as the building blocks for data manipulation. These types include byte, short, int, long, float, double, char, and boolean. Each type has a specific size and range, which plays a critical role in resource management and optimizing performance. For instance, an 'int' is a 32-bit signed integer, allowing it to represent whole numbers within a certain range effectively. Grasping these basic types is crucial when dealing with structures such as lists or generating new instances using the 'new int' command, as it directly influences how information is stored and handled in storage. This foundational knowledge is crucial because Java's type-checking mechanisms align closely with these primitive types, fostering a more intuitive and efficient development experience.
Declaring Variables in Java
Declaring variables in Java involves specifying the type followed by the variable name, such as int myNumber;
. This informs the compiler about the variable's data type and its intended use. When you utilize the new int
statement, it not only declares the variable but also assigns space for it. For example, int[] myArray = new int[10];
declares an array that can hold ten integers, initializing the space required for those values. Effective resource management is essential in this context, as it prevents leaks and ensures optimal performance. For instance, Java's Garbage Collection (GC) plays a significant role in managing resources efficiently, by automatically reclaiming space that is no longer in use. This helps in maintaining the smooth running of applications and avoiding resource-related issues.
The 'new' Keyword in Java
The new
keyword in Java is essential for generating objects and reserving space for collections. When you use new
, you instruct the Java Virtual Machine (JVM) to allocate space on the heap. For example, new int[5]
reserves space for an array of five integers. Effective management of storage is crucial to prevent problems such as resource leaks, which happen when space assigned to objects is not freed after they are no longer required. This can lead to increased memory consumption and degraded performance. Understanding and utilizing the new
keyword properly helps ensure your Java programs run smoothly and efficiently handle dynamic data during runtime.
Arrays in Java: Using 'new int[]'
Arrays in Java are essential objects designed to store multiple values of the same type. Utilizing the syntax new int[]
, developers can dynamically create collections of integers. For instance, int[] numbers = new int[3];
initializes a collection that can hold three integer elements. A key feature of Java collections is their built-in support, which eliminates the need for additional imports, thereby simplifying coding.
However, collections have some limitations. One of the most significant aspects is their fixed size; once a collection is created, its size cannot be changed. If more elements need to be stored than the structure can hold, a new, larger structure must be created, and elements from the old structure need to be copied over—a process that can be inefficient. Additionally, all components in a newly created collection are initialized to zero by default, a characteristic inherent to Java.
Studies indicate that most Java collections are small, accessed by only one or two classes, and typically by a single thread. On average, 69.8% of data access patterns involve straightforward traversals. It's also significant that over 95% of classes in these studies did not use collections directly, highlighting that while collections are fundamental, their direct usage in complex systems might be limited.
Accessing and Modifying Array Elements
When you create a collection in Java using new int[]
, accessing and modifying its elements is straightforward. Each element is accessed via its index, starting from zero. For instance, using numbers[0] = 5;
assigns the value 5 to the first element of the collection. Modifying values is just as simple: you refer to the desired index and assign a new value. Ensuring that index values are within the collection’s bounds is crucial to avoid ArrayIndexOutOfBoundsException
errors.
Arrays allow for efficient data manipulation and analysis by providing methods for accessing, modifying, and iterating over elements. This makes it possible to perform complex operations such as searching, sorting, and filtering with ease. Grasping the fundamentals of collections, along with their classifications and applications, prepares you to utilize their strength in your coding assignments. One-dimensional collections, in particular, are fundamental in programming, enabling the storage and manipulation of elements in a linear fashion. Each component in such a collection can be accessed using a unique index, facilitating straightforward data management.
In the context of real-world applications, lists are indispensable. For example, in the area of finance, collections are utilized for financial modeling and risk assessment, where large datasets are handled to make informed decisions. Likewise, in image processing, collections are crucial for managing pixel data effectively. 'The versatility and efficiency of these structures make them a vital tool in various industries, reflecting their significance in both basic and advanced programming scenarios.'.
Common Mistakes and Best Practices
Working with collections and the new int
statement can lead to some common pitfalls. One frequent mistake is failing to specify the size of the collection during declaration, leading to compile-time errors. Developers should always be cautious about index bounds to avoid exceptions. Employing loops for initializing and accessing data elements enhances code readability and reduces redundancy.
Comprehending the distinctions between lists and ArrayLists is essential. Arrays offer optimal performance when the size is fixed and known, making them highly efficient due to their compact storage and constant-time random access. However, if the size of the data structure is dynamic or uncertain, ArrayLists or linked lists provide more flexibility and efficient memory management.
In practice, over 95% of instrumented classes do not use collections directly, highlighting the importance of selecting the appropriate data structure. For instance, a study tracked 3,803,043,390 data accesses made across 168,686 classes, revealing that 69.8% of access patterns comprise simple traversals. This data underscores that understanding array usage and alternatives like ArrayLists can significantly impact performance and memory management.
Conclusion
Java's primitive types and array structures form the backbone of effective data manipulation within the language. The significance of understanding types such as byte, short, int, long, float, double, char, and boolean cannot be overstated, as they are fundamental for efficient memory management and performance optimization. Proper variable declaration and memory allocation using the new
keyword are essential practices that contribute to writing robust and error-free code.
Arrays play a crucial role in Java programming by allowing developers to store multiple values of the same type. While they offer built-in support and straightforward access methods, developers must be mindful of their fixed size limitations and the potential for memory inefficiencies. Understanding how to access and modify array elements is key to utilizing their full potential while avoiding common pitfalls such as ArrayIndexOutOfBoundsException
.
Recognizing best practices and common mistakes when working with arrays enhances coding efficiency and reduces errors. The choice between arrays and more flexible data structures like ArrayLists should be informed by the specific requirements of the application, as each has its own strengths in terms of performance and memory management. Overall, a solid grasp of these concepts is indispensable for any Java developer aiming to craft optimized, high-performance applications.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.