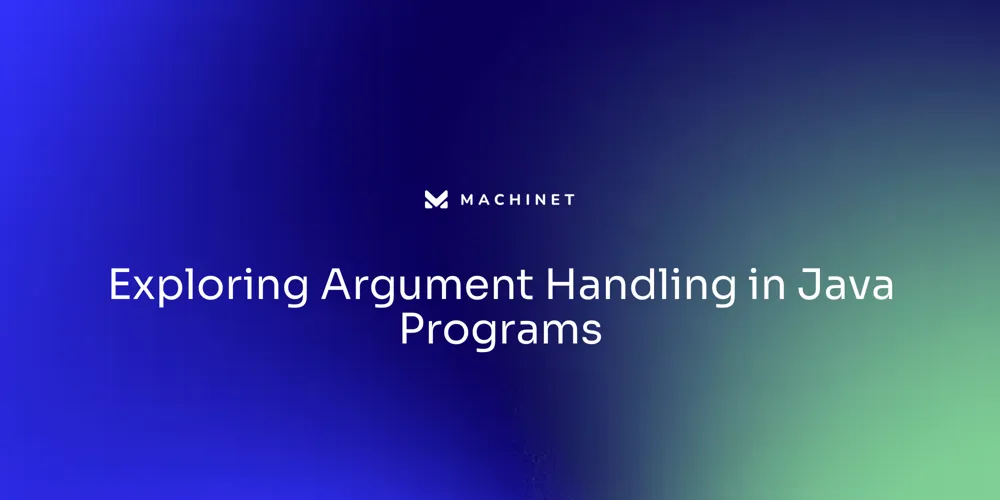
Table of Contents
- What are Command Line Arguments?
- How to Access Command Line Arguments in Java
- Parsing and Converting Command Line Arguments
- Handling Invalid or Missing Command Line Arguments
Introduction
Command line arguments in Java serve as a versatile conduit for passing parameters to a program at runtime. They enable developers to tailor the execution of an application dynamically, depending on the arguments received from the command line interface.
Each argument is a string, and together they form an array of strings known as 'args', which is typically processed in the 'main' method of the program. This array can hold a variety of inputs that the program can utilize to modify its behavior, from configuration settings to operational data.
In this article, we will explore what command line arguments are in Java and how they can be accessed and utilized in your programs. We will also discuss the importance of handling invalid or missing command line arguments and provide best practices for robust and user-friendly error handling. So, let's dive into the world of Java command line arguments and discover how they can enhance the flexibility and functionality of your applications.
What are Command Line Arguments?
Command line arguments in Java serve as a versatile conduit for passing parameters to a program at runtime. They enable developers to tailor the execution of an application dynamically, depending on the arguments received from the command line interface.
Each argument is a string, and together they form an array of strings known as 'args', which is typically processed in the 'main' method of the program. This array can hold a variety of inputs that the program can utilize to modify its behavior, from configuration settings to operational data.
The declaration of this string array can be done using either 'String[] args' or 'String args[]', with the former being the convention preferred by the majority of Java developers for its clarity and alignment with other array declarations in the language. Notably, these command line arguments are distinct from environment variables, which are set at the operating system level and can influence not just the Java application, but potentially other subprocesses as well.
Environment variables require careful management due to their broader scope and the possibility of unintended side effects, such as differences in case sensitivity across various operating systems. Java's evolution over nearly three decades has seen it incorporate features that maintain its modernity. With the release of JDK 23, Java continues to innovate, evidenced by the introduction of a Vector API that optimizes vector computations for different CPU architectures. This underscores Java's commitment to performance and its status as a mature yet continually evolving language, capable of supporting both traditional applications and modern development practices.
How to Access Command Line Arguments in Java
Command line arguments in Java offer a way to influence the behavior of an application at runtime. The args
parameter in the main
method is the gateway to accessing these arguments.
It is an array that captures the string values passed to the program. For instance, args[0]
holds the first argument passed.
Beyond simple access, the environment variables set prior to application startup are crucial for production-grade software. They can be set via command line or scripts, particularly in Unix systems, enhancing the program's flexibility to operate with external configurations.
Recent updates in the Java Development Kit, like JDK 23, have introduced new features to streamline coding practices further. This includes the Vector API, enhancing runtime compilation for vector computations across CPU architectures. While the core language provides powerful capabilities, the adoption of frameworks can elevate productivity. Practical examples demonstrate the value of such frameworks in managing complex projects, showcasing their strengths in real-world applications. As developers navigate the intricacies of Java, they learn to balance the native language's control with the frameworks' convenience, all while adhering to best practices for maintainable and clean code.
Parsing and Converting Command Line Arguments
In Java, command line arguments are a powerful way to pass information to your program at runtime, but handling them requires careful consideration. When you receive these arguments, they are typically in string format. To utilize them effectively within your Java application, you might need to convert them into specific data types.
Java is equipped with a set of tools for this task. For instance, you can employ the Integer.parseInt()
method to transform a string into an integer, or Double.parseDouble()
to change it into a double value. To navigate and manipulate command line arguments with more flexibility, the Scanner
class is a versatile tool that can parse strings into various types, extracting the data you need seamlessly.
These methods and classes are part of Java's standard libraries, which provide developers with the capability to craft tailored solutions and control over their codebases. Leveraging these features, you can efficiently process input data, ensuring your application handles command line arguments with precision and clarity, as emphasized by the importance of readability and maintainability in programming. Furthermore, the recent enhancements in JDK 11 and the introduction of new features in Java 21, like the ability to run .java source files directly, underscore Java's commitment to facilitating a developer's ability to tinker and iterate code effectively.
Handling Invalid or Missing Command Line Arguments
Handling command line arguments in Java requires careful consideration to ensure robustness and user-friendliness. When arguments are missing or cannot be parsed as expected, the application should not just crash; it should provide helpful feedback.
This can be achieved through a combination of conditional statements, which can check for the presence or format of arguments, and exception handling, which can gracefully manage errors during runtime. For example, if a user forgets to input a required argument, a clear error message should guide them towards correct usage.
Similarly, when an argument cannot be parsed, an exception can be caught, and a user-friendly message can be displayed, possibly along with a suggestion for valid input. It's also possible to define default values for certain arguments, ensuring the application can function even in the absence of input.
This approach to handling command line arguments enhances the maintainability of the code and ensures a consistent and professional user experience. As noted by experts, "The bug is most likely in the application," emphasizing the importance of thorough error handling within our code. With advancements in Java, such as the introduction of the Null-Safe Operator in Java 7, developers have even more tools at their disposal to write cleaner, more reliable command-line applications. It's these small, careful considerations in handling user input that contribute to the overall robustness and reliability of Java applications.
Conclusion
In conclusion, command line arguments in Java serve as a versatile way to pass parameters to a program at runtime, allowing developers to customize the behavior of their applications. These arguments are accessed through the 'args' array in the main method and can be used to modify the program's behavior based on user input or external configurations.
To effectively utilize command line arguments, developers can parse and convert them into specific data types using built-in tools like Integer.parseInt() and Double.parseDouble(). The Scanner class is also a valuable resource for navigating and manipulating command line arguments with flexibility.
Handling invalid or missing command line arguments is crucial for robustness and user-friendliness. By implementing conditional statements and exception handling, developers can provide helpful feedback when arguments are missing or cannot be parsed correctly.
Defining default values for certain arguments ensures that the application can still function even without input. Java's commitment to performance and evolution is evident with updates like JDK 23 and the introduction of new features such as the Vector API. These advancements, along with frameworks, enhance productivity while adhering to best practices for clean and maintainable code. Overall, by understanding how to access, parse, and handle command line arguments in Java, developers can create flexible and functional applications that meet user needs effectively.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.