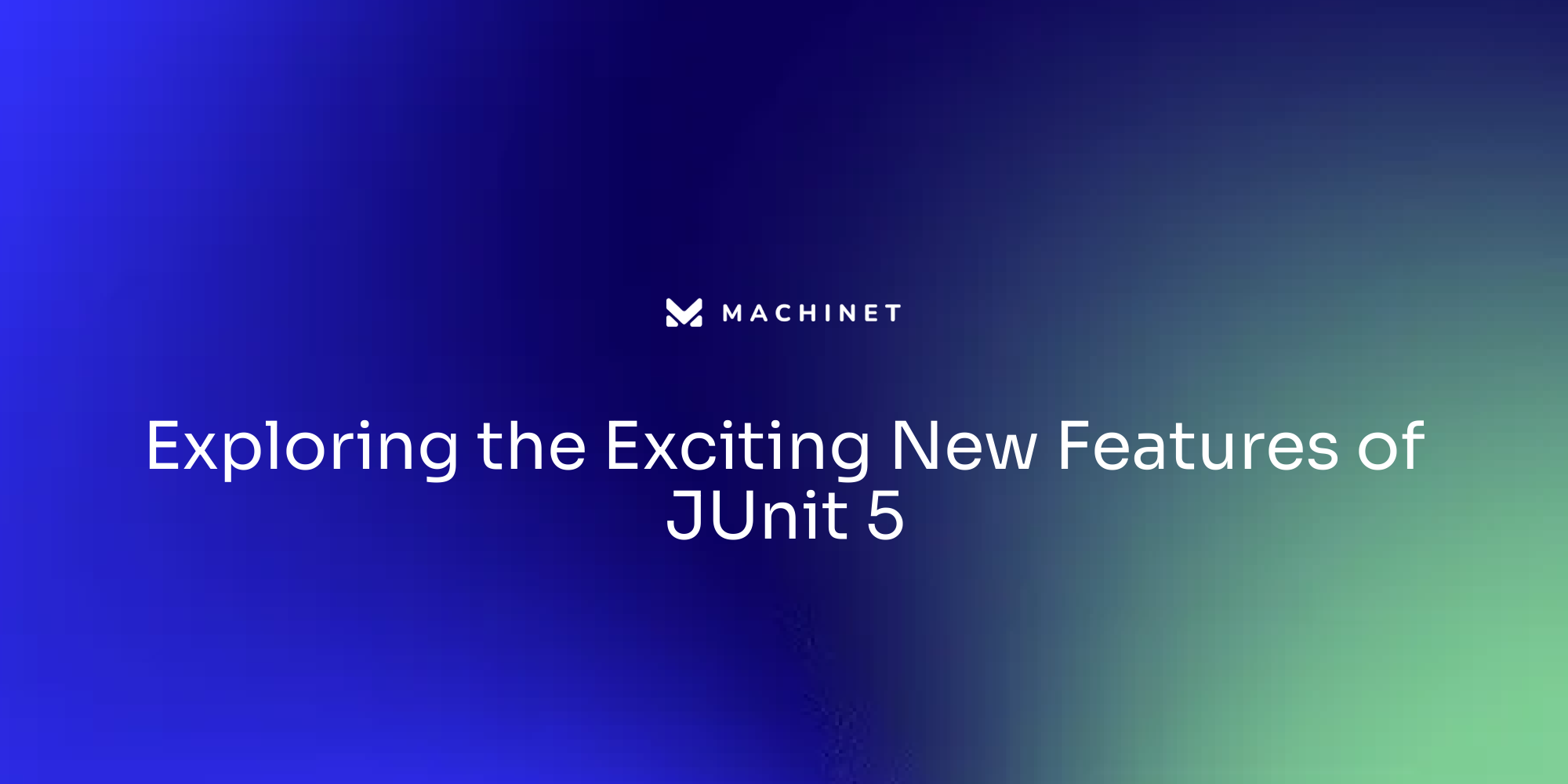
Table of Contents
- Understanding the Basics of JUnit 5
- Transitioning from JUnit 4 to JUnit 5: Key Differences
- Leveraging New Annotations and Assertions in JUnit 5
- Implementing Dynamic Tests in JUnit 5
- Utilizing Test Interfaces and Default Methods in JUnit 5
- Enhancing Test Suites with Tagging and Filtering in JUnit 5
- Managing Test Lifecycle with Extensions in JUnit 5
- Navigating Potential Challenges While Porting Tests to JUnit 5
Introduction
JUnit 5, the latest iteration of the renowned testing framework, introduces a range of upgrades and novel features that make it an essential tool for developers. With its modular architecture, support for Java 8 and beyond, enhanced assertions and assumptions, and dynamic tests, JUnit 5 offers a more contemporary and flexible choice for testing. In this article, we will explore the basics of JUnit 5, the key differences from JUnit 4, the new annotations and assertions, implementing dynamic tests, utilizing test interfaces and default methods, enhancing test suites with tagging and filtering, managing test lifecycle with extensions, and navigating potential challenges while porting tests to JUnit 5. Whether you're new to JUnit or looking to transition from JUnit 4, this article will provide you with a comprehensive understanding of JUnit 5 and its capabilities. Get ready to enhance your testing practices with JUnit 5!
1. Understanding the Basics of JUnit 5
JUnit 5.4, the latest iteration of the esteemed testing framework, showcases a plethora of upgrades and novel features that render it a must-have tool for developers. This version pioneers a newfangled architecture that distinguishes the JUnit platform, JUnit Jupiter, and JUnit Vintage, laying the groundwork for a robust foundation for launching testing frameworks on JVM.
This version's salient features encompass support for Java 8 and subsequent versions, the debut of a new extension model, and the enhancement of assertions and assumptions. Furthermore, it brings to light dynamic tests, tests that are produced during runtime by a factory method.
When juxtaposing the modernized JUnit 5.4 with its predecessor, JUnit 4, the former stands out with its support for lambda functions and test method parameter injection, placing it as a more contemporary choice. The setup with JUnit 5.4 is straightforward as it only necessitates the inclusion of the single junit-jupiter dependency.
Moreover, JUnit 5.4 introduces the @TempDir annotation, which streamlines the creation and cleanup of temporary files during testing. It also extends support for parameterized tests, including null and empty values. The @DisplayName annotation permits more dynamic and readable test display names, thereby enhancing readability.
JUnit 5.4 also heralds the @OrderAnnotation and @TestMethodOrder annotations, which enable the specification of the execution order of test methods. The release notes of JUnit 5.4 provide more information about additional features and improvements, making it a recommended upgrade given the plethora of new features and enhancements it brings.
It's crucial to note that JUnit 5.4 is an evolution of JUnit 5, with a focus on Java 8 and beyond, and enables various testing styles. This version originated from the JUnit Lambda project and its crowdfunding campaign on Indiegogo.
To utilize JUnit 5.4 with Java 8, be sure to add the necessary dependencies to your project. For instance, by using a build tool like Maven or Gradle, you can add the JUnit 5 dependencies to your project's build file. In Maven, the dependencies can be added to your pom.xml file as follows:
xml
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>5.8.1</version>
<scope>test</scope>
</dependency>
</dependencies>
Once the dependencies are added, you can write JUnit 5 tests using the new features and annotations provided by JUnit 5. For instance, you can use the @Test
annotation to mark a method as a test, use the @BeforeEach
and @AfterEach
annotations to set up and tear down test fixtures, and use the assert
methods from the Assertions
class to make assertions in your tests.
Ensure that your IDE supports JUnit 5, as some older versions may not fully support it. Once everything is set up, you should be able to run your JUnit 5 tests with Java 8 seamlessly.
JUnit 5.4 offers numerous other features, which can be found in the release notes. JUnit artifacts are deployed to Maven Central and can be downloaded. The JUnit team uses GitHub for version control, project management, and CI. They rely on Develocity to analyze and speed up their builds. The JUnit team can be followed on Mastodon and Twitter.
The copyright for JUnit belongs to the JUnit team
2. Transitioning from JUnit 4 to JUnit 5: Key Differences
Transitioning from JUnit 4 to JUnit 5 requires a thorough understanding of the major differences between these two frameworks. A significant enhancement in JUnit 5 is the introduction of a modular architecture, which enables software developers to use only the components they need, thus avoiding unnecessary dependencies.
In JUnit 5, the previously intertwined test runner and test classes have been separated. This means tests are implemented as methods and classes annotated with @Test, simplifying the structure and readability of the tests, resulting in improved manageability.
Another significant improvement in JUnit 5 is the enhanced assertion API. With robust support for lambda expressions and a more fluent API, assertions have become more expressive and readable, thereby enhancing the overall testing experience.
One of the groundbreaking features of JUnit 5 is dynamic tests, which are generated at runtime and provide an unprecedented level of flexibility in test writing. This feature is particularly useful when the input data is not known in advance or varies between test runs.
The latest iteration, JUnit 5.4, brought several improvements, including the "TempDirectory" extension, which simplifies the creation and cleanup of temporary files during testing. Parameterized tests in JUnit 5.4 now support null and empty input values, adding to the flexibility of test writing. The "DisplayName" annotation allows for smarter generation of test method and class names, providing clearer test results.
JUnit 5.4 also introduces the "OrderAnnotation" and "TestMethodOrder" annotations, which allow for specifying the execution order of test methods. This can be particularly useful when the order of execution can affect the outcome of the tests.
To integrate JUnit 5.4 into a project, you only need to include the single dependency of "junit-jupiter" in the build.gradle file. The transition from JUnit 4 to JUnit 5 can be facilitated by following a step-by-step guide, which includes understanding the differences between the two versions, updating project dependencies, migrating and refactoring tests, and running the tests to ensure successful transition[^4^].
To explore the modular architecture of JUnit 5, you may refer to resources like the blog post titled "JUnit Framework: Understanding Annotations and Assertions for Java Unit Testing" on the Machinet website[^5^]. The setup of the JUnit 5 test runner can be simplified by following steps like importing necessary dependencies, creating a new test class and annotating it with @RunWith(Machinet.class), setting up necessary configurations or resources before running the tests, defining test methods using the @Test annotation, and cleaning up any resources or configurations after running the tests[^8^].
With its modern features and robust capabilities, JUnit 5 is primed to become the preferred testing framework for Java and JVM developers. The JUnit team, backed by a community of sponsors and backers, continues to innovate and improve the framework, ensuring it remains at the forefront of software testing technology
3. Leveraging New Annotations and Assertions in JUnit 5
JUnit 5 has introduced a range of improvements to the field of test writing, largely due to the advent of new annotations and assertions. The @DisplayName annotation, for example, can be used to provide a test with a custom, human-readable name. This not only enhances code readability but also helps in better test management.
```java import org.junit.jupiter.api.DisplayName; import org.junit.jupiter.api.Test;
public class MyTest {
@Test
@DisplayName("Custom test name")
public void testMethod() {
// Test logic goes here
}
}
``
In the example above,
@DisplayName("Custom test name")` gives a custom name to the test, which will be shown in the test results instead of the default method name.
Another notable addition is the @Nested annotation, which enables the creation of nested test classes. This proves especially useful when we want to group related tests together for improved organization and readability.
Beyond these annotations, JUnit 5 has also introduced a more fluent interface for assertions and assumptions, making the process of writing and reading tests significantly smoother. One of the highlights of the new assertions API is the enhanced assertEquals assertion. Unlike its predecessor, the new assertEquals assertion shows both the expected and actual values in its failure message. This small but meaningful improvement makes it much easier to identify what went wrong in a test case.
java
assertEquals(expected, actual, "Custom failure message");
In this case, if the expected and actual values are not equal, the test will fail and the custom failure message will be displayed, providing additional context or information about the failure.
Moreover, JUnit 5 comes with a comprehensive set of features such as parameterized tests, lifecycle methods, test run order, assumptions, disabling and repeating tests, tags, and use of external libraries like Hamcrest. Understanding and leveraging these features can turn users into test automation superstars.
Moving on to the Assertion API, it provides a variety of methods for writing assertions. It is important to note that to write assertions with JUnit 5, the org.junit.jupiter.api.Assertions class must be invoked. This class provides static methods for writing assertions, such as assertTrue, assertFalse, assertNull, assertNotNull, assertEquals, assertNotEquals, assertSame, assertNotSame, assertArrayEquals, and assertIterableEquals.
Furthermore, complex error messages with parameters can be created using a message supplier, and assertions can be grouped using the assertAll method of the Assertions class. This method takes an optional heading and an array, collection, or stream of executable objects that invoke the assertions. Custom error messages can be specified by passing a string object as the last parameter of the invoked assertion method. This does not override the default error message but is prepended to it, providing additional context when an assertion fails.
In the realm of exception testing, the assertThrows method can be used to write assertions for exceptions and verify the type of the expected exception and the error message. In contrast, the assertDoesNotThrow method is used to assert that no exception is thrown by the system under test.
Lastly, JUnit 5 provides the assertTimeout and assertTimeoutPreemptively methods to write assertions for the execution time of the system under test. The former executes the provided executable or throwing supplier in the same thread and does not abort the execution if the timeout is exceeded. In contrast, the latter executes the provided executable or throwing supplier in a different thread and aborts the execution if the timeout is exceeded.
In sum, JUnit 5 has brought a plethora of improvements and new features to the table, making it a powerful tool for writing and managing tests in the Java ecosystem.
By understanding and effectively leveraging these features, developers can significantly enhance their testing capabilities and deliver high-quality software products
4. Implementing Dynamic Tests in JUnit 5
JUnit 5 introduces a flexible approach to test creation that can be particularly beneficial when dealing with parameterized tests or situations that demand adaptability in testing. This adaptability is achieved through dynamic tests, which are generated at runtime by a factory method. The @TestFactory
annotation is used to mark a method as a test factory.
Methods marked with this annotation are expected to produce a Stream, Collection, Iterable, or Iterator of DynamicTest
instances. Each DynamicTest
instance represents a single test case and consists of two parts: the display name, which acts as an identifier for the test case, and the TestExecutionBody
, a functional interface embodying the logic of the test case.
The dynamic nature of these tests provides a notable enhancement in flexibility over traditional static tests. For example, in the fast-paced field of software development, testing requirements can often change. Dynamic tests allow for tests that can efficiently adapt to these changes, minimizing the time and effort needed to revamp test cases.
Dynamic tests also prove beneficial in data-driven testing scenarios, such as parameterized tests. For instance, you might have a set of data inputs that you wish to apply the same test logic to. Instead of creating separate static tests for each data input, you can use a dynamic test to generate these tests at runtime.
A crucial feature of dynamic tests in JUnit 5 is the utilization of the TestExecutionBody
. This functional interface is a straightforward yet potent tool that allows you to specify the behavior of each dynamic test. It offers a clear and succinct way of defining your test logic, making your tests easier to read and maintain.
In summary, the introduction of dynamic tests in JUnit 5 offers a more adaptable and flexible approach to writing tests. This can result in more efficient and maintainable test suites, which can significantly contribute to the delivery of high-quality software applications
5. Utilizing Test Interfaces and Default Methods in JUnit 5
JUnit 5 brings to the table the unique ability to define tests within interfaces, enhancing code reusability and offering a flexible structure for testing. These test interfaces can be leveraged to create a contract that a multitude of test classes can conform to, thereby ensuring a consistent standard across all tests.
Moreover, JUnit 5 extends its capabilities by allowing the use of default methods to offer concrete implementations of tests. This feature can be particularly advantageous when you are contending with complex test hierarchies or wish to disseminate common test logic across multiple classes. Default methods provide a baseline implementation of a test that can be overridden if necessary.
In JUnit 5, code reuse in test structure can be achieved through various mechanisms. One common approach is using parameterized tests, where a single test method is executed multiple times with different sets of input parameters. This allows for testing different scenarios with minimal code duplication. Additionally, JUnit 5 provides support for test interfaces, which allows multiple test classes to implement a common interface and share test methods. This promotes code reuse and ensures consistent testing across different test classes.
To share common test logic with JUnit 5 default methods, you can leverage the concept of interfaces and default methods in JUnit 5. By defining an interface with default methods, you can provide common test logic that can be shared across multiple test classes.
Further, JUnit 5 introduces a new annotation, @DisplayName
, which permits you to configure the display name of your test classes and test methods, enhancing the readability of your test results. This feature underscores the importance of clear communication in test results, making it easier for the entire team to comprehend the outcome of the tests.
JUnit 5 also provides @BeforeAll
, @BeforeEach
, @AfterEach
, and @AfterAll
annotations for setup and teardown methods. These methods should not return any value and should not be declared as private.
Moreover, JUnit 5 brings support for parameterized tests, a feature that can drastically cut down the amount of boilerplate code in your tests. This is achieved through the use of the @ParameterizedTest
and @ValueSource
annotations among others. These annotations, in conjunction with custom argument providers, enable you to create dynamic, data-driven tests with ease.
To sum up, the shift to JUnit 5 brings along a wealth of new features and improvements, making it a potent tool for writing and managing tests in the Java ecosystem. Whether you are grappling with complex test hierarchies, striving for better code reusability, or looking to create more readable and dynamic tests, JUnit 5 has you covered
6. Enhancing Test Suites with Tagging and Filtering in JUnit 5
JUnit 5's tagging and filtering capabilities provide a robust mechanism to govern the execution of specific tests. By attaching simple string labels or 'tags' to tests using the @Tag annotation, you can categorize your tests according to your needs. For instance, you can tag a test method with the "smoke" tag as follows:
java
@Test
@Tag("smoke")
public void testMethod() {
// Test logic here
}
You can also assign multiple tags to a test method or class by providing a comma-separated list of tag names:
java
@Test
@Tag({"smoke", "regression"})
public void testMethod() {
// Test logic here
}
This proves incredibly beneficial in larger projects where running specific subsets of tests becomes a necessity. Once you have assigned tags to your test methods or classes, you can use the tags to filter the execution of tests. This can be done through IDEs, build tools, or the JUnit 5 Console Launcher. By specifying the tags you want to include or exclude, you can run only the tests that match the specified criteria.
Furthermore, JUnit 5 extends the control over test execution with its filtering feature. This allows you to include or exclude tests based on various parameters such as tags, class names, or even custom filters. For example, to include only the tests with the tag "integration", you can use the following command:
java
mvn test -Dgroups="integration"
Similarly, to exclude tests with the tag "performance", you can use the following command:
java
mvn test -DexcludedGroups="performance"
This enriches the granularity of test execution, enabling you to manage your tests more efficiently.
However, as you leverage these features, it's crucial to remember that the number of sequences can increase rapidly with the increase in the number of set members. This can potentially impact the time required for test execution.
In addition to JUnit 5, the Chronicle Test Framework, an open-source library, offers support classes for writing JUnit tests. It is equipped with features that support combinations, permutations, and products, allowing you to create exhaustive tests. For instance, it can generate streams of collection elements, making it easy to adapt to other frameworks like JUnit 5. Furthermore, it can be used to compare the behavior of different list implementations against each other.
For instance, Chronicle Queue and Chronicle Map, two libraries, utilize the Chronicle Test Framework to enhance their testing capabilities. In a code example from Chronicle Map, five map operations were exhaustively tested, resulting in 326 dynamic tests.
In closing, both JUnit 5 and the Chronicle Test Framework offer advanced features that can significantly improve your testing capabilities. However, one must be mindful of the potential increase in test time if the number of dynamic tests is not kept under control
7. Managing Test Lifecycle with Extensions in JUnit 5
JUnit 5 has ushered in an innovative extension model that has supplanted the runner classes of JUnit 4. This model allows for a new level of flexibility and composability. It includes a variety of extension points such as beforeAll, beforeEach, afterEach, and afterAll, which are instrumental in performing actions at specific stages of test execution.
For instance, JUnit 5 allows you to use the @ExtendWith
annotation to register extensions that provide additional functionality to your tests. This includes popular extensions like @BeforeEach
and @AfterEach
annotations used for defining methods that will run before and after each test method. For resources that are shared across multiple tests, @BeforeAll
and @AfterAll
annotations can be utilized. These annotations can be used to set up or tear down expensive or time-consuming resources that are shared across multiple tests.
Moreover, the extension model of JUnit 5 allows for the integration of additional information into test methods by utilizing built-in extensions like TestInfoParameterResolver and TestReporterParameterResolver. The ease with which extensions can be created is another notable feature of JUnit 5. Developers can create extensions by implementing one or more extension APIs, including TestInstancePostProcessor, ExecutionCondition, ParameterResolver, or TestExecutionExceptionHandler.
The registration process of extensions is simplified by using the @ExtendWith
annotation. This annotation allows you to specify multiple extensions for a test class or a test method. Additionally, extensions can also be registered via a configuration file, providing more flexibility.
The integration of third-party frameworks such as Mockito, Selenium, and Spring TestContext with JUnit 5 has broadened the scope of testing. For instance, with the LambdaTest platform, Selenium automation tests can be executed on a scalable, cloud-based infrastructure. This platform supports testing on a wide array of devices and browsers, thereby facilitating comprehensive browser compatibility testing.
JUnit 5 also offers advanced annotations for testing reactive applications, which are particularly useful in handling exceptions and customizing the behavior of test cases when exceptions occur. The extensible architecture and the new extension model of JUnit 5 make it easy to implement custom features and handle exceptions with the TestExecutionExceptionHandler interface.
For further learning and assistance, JUnit 5 provides resources including the official documentation, user guide, and online tutorials. For a deep dive into the different extension APIs in JUnit 5, you can refer to the official JUnit 5 documentation that provides comprehensive information about the various extension points and APIs available. By studying the documentation, you can gain a better understanding of how to leverage these extension APIs in JUnit 5 to enhance your test suite. The transition from JUnit 4 to JUnit 5 has indeed been a significant leap towards more efficient and comprehensive testing in the Java ecosystem
8. Navigating Potential Challenges While Porting Tests to JUnit 5
Shifting from JUnit 4 to JUnit 5 isn't without its challenges, given the differences in functionalities like parameterized tests and rules. However, it's essential to remember that JUnit 5 introduces a fresh programming model and several new features that make the migration worthwhile. The steps to migrate your JUnit 4 tests to JUnit 5 include updating the JUnit dependency in your project's build file, updating test annotations, assertions, test runner, test lifecycle methods, and test naming conventions.
For example, replace @Test
with @org.junit.jupiter.api.Test
, and assertEquals()
with Assertions.assertEquals()
. Instead of using the test runner from JUnit 4, you now annotate your test class with @RunWith(JUnitPlatform.class)
. The test lifecycle methods also need updating, such as replacing @BeforeClass
with @BeforeAll
. As for test naming conventions, JUnit 5 allows you to use any name you prefer for your test methods, provided you annotate them with @DisplayName
for a meaningful name.
Despite the initial hurdles, JUnit 5.4 has made significant strides to justify the transition. It simplifies usage with Gradle, introduces temporary directories for testing, and even allows dynamic generation of the display name of test methods based on the nested class or method name. A new test method order annotation has been introduced, enabling the execution of test methods in a specific order.
Notably, JUnit 5.4, released on October 19, 2021, presents several improvements over the previous 5.3.2 release, elevating JUnit 5 as a more contemporary version compared to JUnit 4. It incorporates features like lambda support and test method parameter injection. The inclusion of dependencies has been simplified, allowing the use of just the single junit-jupiter dependency. High-quality documentation for JUnit 5 provides illustrative code examples, which further assist in the transition from JUnit 4 to JUnit 5.
Tools like IntelliJ IDEA offer built-in support for migrating tests from JUnit 4 to JUnit 5, easing the transition process. However, it's important to note that the current migration action does not support exception tests in JUnit 4, requiring manual migration of these tests. JUnit 5 also enables the reduction of accessibility of test classes and methods to package default scope, achieved by changing the modifier. This minimizes the risk of accidentally selecting wrong types and decreases suggestion candidates in the IDE.
In summary, the transition to JUnit 5 is highly encouraged due to the numerous new features and improvements it brings. Despite the initial challenges, the benefits of adopting JUnit 5, such as improved test organization and increased flexibility in test execution, far outweigh the effort required for migration
Conclusion
In conclusion, JUnit 5 is a significant upgrade to the renowned testing framework, offering a range of new features and improvements. With its modular architecture, support for Java 8 and beyond, enhanced assertions and assumptions, dynamic tests, and test interfaces with default methods, JUnit 5 provides developers with a more modern and flexible choice for testing. The transition from JUnit 4 to JUnit 5 brings numerous benefits, including improved code readability, easier test management, and enhanced test organization. By leveraging the new annotations and assertions in JUnit 5, developers can write more expressive and readable tests. Additionally, the tagging and filtering capabilities in JUnit 5 allow for better control over test execution, making it easier to run specific subsets of tests. The extension model in JUnit 5 provides a flexible way to manage the test lifecycle and integrate additional functionality into tests. Overall, JUnit 5 empowers developers to enhance their testing practices and deliver high-quality software products.
Boost your productivity with Machinet. Experience the power of AI-assisted coding and automated unit test generation.
AI agent for developers
Boost your productivity with Mate. Easily connect your project, generate code, and debug smarter - all powered by AI.
Do you want to solve problems like this faster? Download Mate for free now.